
Enhancing Data Mining Techniques with Machine Learning and AI
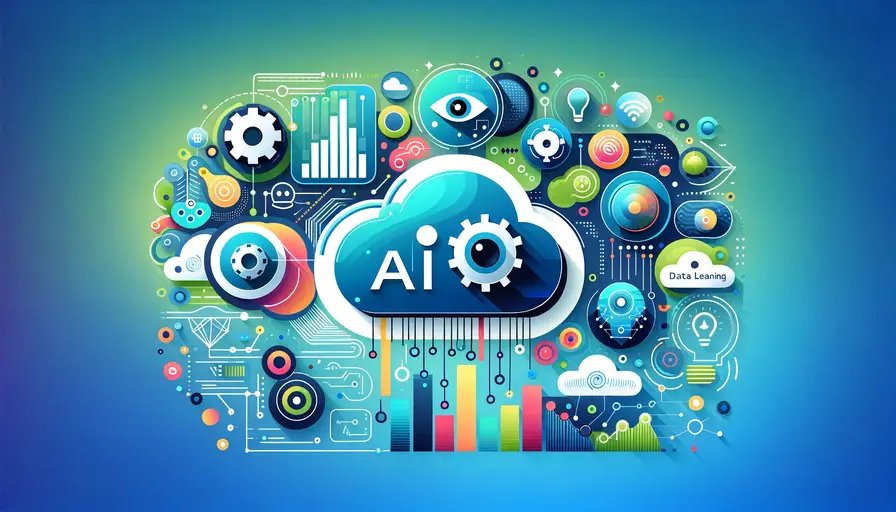
Content
Leveraging Machine Learning for Advanced Data Mining
Exploring Machine Learning Models in Data Mining
Machine learning models have become integral to enhancing data mining techniques, offering the ability to uncover patterns and insights from vast datasets. These models can handle various data types, including structured, unstructured, and semi-structured data, making them versatile tools for data mining tasks.
Decision trees, for instance, are highly effective in data mining due to their simplicity and interpretability. They can be used to classify data into distinct categories or predict outcomes based on historical data. Decision trees work by splitting the data into subsets based on feature values, forming a tree-like structure that is easy to understand and visualize.
Another powerful model for data mining is the Random Forest, an ensemble method that combines multiple decision trees to improve accuracy and robustness. By aggregating the predictions of several trees, Random Forests reduce the risk of overfitting and provide more reliable results. This approach is particularly useful when dealing with large, complex datasets where individual decision trees might struggle.
Deep Learning in Data Mining
Deep learning, a subset of machine learning, has revolutionized data mining with its ability to process and analyze high-dimensional data. Convolutional Neural Networks (CNNs) are particularly well-suited for image data, enabling tasks such as image classification, object detection, and image segmentation. These models automatically learn hierarchical features from raw data, eliminating the need for manual feature extraction.
Exploring Road-Related Machine Learning DatasetsRecurrent Neural Networks (RNNs), including Long Short-Term Memory (LSTM) networks, excel at handling sequential data, such as time series and natural language. RNNs can capture temporal dependencies and patterns in the data, making them ideal for tasks like stock price prediction, speech recognition, and language translation.
Generative models, such as Generative Adversarial Networks (GANs), have also made significant contributions to data mining. GANs consist of two neural networks—a generator and a discriminator—that compete to produce realistic data. This ability to generate high-quality synthetic data has applications in data augmentation, anomaly detection, and creative tasks like image synthesis.
Example: Implementing a Convolutional Neural Network for Image Classification in Python
import numpy as np
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
from keras.utils import to_categorical
# Load and preprocess MNIST dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train = X_train.reshape(X_train.shape[0, 28, 28, 1]).astype('float32') / 255
X_test = X_test.reshape(X_test.shape[0, 28, 28, 1]).astype('float32') / 255
y_train = to_categorical(y_train)
y_test = to_categorical(y_test)
# Create a CNN model
model = Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(64, kernel_size=(3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
# Compile and train the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
In this example, a Convolutional Neural Network (CNN) is implemented using Keras to classify images from the MNIST dataset. The model is trained on digit images, demonstrating the practical application of deep learning in data mining.
Enhancing Data Mining with Artificial Intelligence
Natural Language Processing for Text Data
Natural Language Processing (NLP) is a branch of AI that focuses on the interaction between computers and human language. NLP techniques are crucial for mining text data, enabling tasks such as text classification, sentiment analysis, and topic modeling. By processing and analyzing large volumes of text, NLP models can extract valuable insights and trends.
Enhancing Empirical Asset Pricing with Machine LearningText classification involves categorizing text into predefined categories. For example, an NLP model can classify customer reviews as positive, negative, or neutral. Sentiment analysis goes a step further by identifying the sentiment expressed in the text, helping businesses understand customer opinions and improve their services.
Topic modeling is another powerful NLP technique that identifies the underlying topics in a collection of documents. Models such as Latent Dirichlet Allocation (LDA) can uncover hidden themes and patterns in large text datasets, providing a deeper understanding of the content. These insights can be used for content recommendation, document clustering, and knowledge discovery.
AI-Driven Anomaly Detection
Anomaly detection is the process of identifying unusual patterns or outliers in data. AI-driven anomaly detection models can automatically detect anomalies in large datasets, making them invaluable for applications such as fraud detection, network security, and predictive maintenance. These models learn the normal behavior of the data and flag any deviations that indicate potential anomalies.
One common approach to anomaly detection is the use of autoencoders, a type of neural network that learns to reconstruct its input. During training, the autoencoder learns to compress the data into a lower-dimensional representation and then reconstruct it. Anomalies are detected based on the reconstruction error, with higher errors indicating deviations from the normal pattern.
Using Machine Learning for Mental Health Tracking and SupportAnother approach is the use of clustering algorithms, such as k-means or DBSCAN. These algorithms group similar data points together, and points that do not fit well into any cluster are considered anomalies. Clustering-based methods are effective for detecting anomalies in data with well-defined clusters.
Example: Implementing Anomaly Detection with Autoencoders in Python
import numpy as np
from keras.models import Model, Sequential
from keras.layers import Input, Dense
# Generate synthetic normal data
data = np.random.normal(size=(1000, 20))
# Create an autoencoder model
input_dim = data.shape[1]
encoding_dim = 10
input_layer = Input(shape=(input_dim,))
encoded = Dense(encoding_dim, activation='relu')(input_layer)
decoded = Dense(input_dim, activation='sigmoid')(encoded)
autoencoder = Model(input_layer, decoded)
autoencoder.compile(optimizer='adam', loss='mse')
# Train the autoencoder
autoencoder.fit(data, data, epochs=50, batch_size=32, shuffle=True)
# Detect anomalies
reconstructions = autoencoder.predict(data)
mse = np.mean(np.power(data - reconstructions, 2), axis=1)
threshold = np.percentile(mse, 95)
anomalies = data[mse > threshold]
print(f"Detected {len(anomalies)} anomalies")
In this example, an autoencoder is implemented using Keras for anomaly detection. The model is trained on synthetic normal data, and anomalies are detected based on the reconstruction error, showcasing the application of AI in identifying unusual patterns.
Integrating AI with Traditional Data Mining Techniques
Combining Clustering with AI
Clustering is a fundamental data mining technique that groups similar data points together. Integrating AI with clustering can enhance the accuracy and interpretability of the results. For example, clustering algorithms such as k-means can be combined with deep learning models to handle high-dimensional data more effectively.
Deep clustering is an approach that combines clustering with deep learning. In this method, deep neural networks are used to learn a lower-dimensional representation of the data, which is then clustered using traditional algorithms. This approach leverages the feature extraction capabilities of deep learning to improve clustering performance.
Effective Strategies for Machine Learning in Noisy Data EnvironmentsHierarchical clustering is another technique that benefits from AI integration. AI models can preprocess the data to highlight important features, making hierarchical clustering more effective. Additionally, AI-driven methods can help visualize and interpret the clustering results, providing deeper insights into the data.
Enhancing Association Rule Mining with AI
Association rule mining is a data mining technique used to discover interesting relationships between variables in large datasets. These relationships are often expressed as rules that capture associations, such as "If a customer buys bread, they are likely to buy butter." Integrating AI with association rule mining can enhance the discovery of complex patterns and improve rule accuracy.
AI-driven approaches can preprocess the data to identify relevant features and reduce noise, making association rule mining more effective. Additionally, machine learning models can be used to validate and refine the discovered rules, ensuring that they are accurate and actionable.
Deep learning models, such as recurrent neural networks (RNNs), can also be applied to sequential pattern mining. These models can capture temporal dependencies in the data, uncovering sequential patterns that traditional methods might miss. This integration of AI and association rule mining provides a more comprehensive understanding of the data and its relationships.
Machine Learning for RSS Feed Analysis in RExample: Implementing Apriori Algorithm for Association Rule Mining in Python
import pandas as pd
from mlxtend.frequent_patterns import apriori, association_rules
# Load and preprocess the data
data = pd.read_csv('path/to/transactions.csv')
basket = data.pivot_table(index='transaction_id', columns='item', aggfunc='size', fill_value=0)
# Apply Apriori algorithm to find frequent itemsets
frequent_itemsets = apriori(basket, min_support=0.01, use_colnames=True)
# Generate association rules
rules = association_rules(frequent_itemsets, metric='lift', min_threshold=1.0)
print(rules)
In this example, the Apriori algorithm is implemented using the mlxtend library in Python for association rule mining. The model identifies frequent itemsets and generates association rules, demonstrating the integration of AI in discovering meaningful relationships in data.
AI-Powered Data Preprocessing
Data preprocessing is a critical step in data mining that involves cleaning, transforming, and preparing data for analysis. AI-powered data preprocessing techniques can automate and enhance this process, improving data quality and making subsequent analysis more effective.
AI models can automatically detect and handle missing values, outliers, and inconsistencies in the data. For example, imputation algorithms can predict missing values based on the available data, ensuring that the dataset remains complete and usable. Similarly, AI-driven outlier detection can identify and remove anomalous data points that might skew the analysis.
Feature engineering, the process of creating new features from raw data, is another area where AI can make a significant impact. AI models can identify important features, create interactions between variables, and transform data into more meaningful representations. This automated feature engineering accelerates the data preprocessing process and enhances the performance of data mining models.
Machine Learning-Based Bitcoin Price PredictionsMachine learning and AI have transformed data mining techniques, providing powerful tools for uncovering insights and patterns in data. By leveraging advanced models, integrating AI with traditional methods, and enhancing data preprocessing, organizations can extract more value from their data and make informed decisions. As these technologies continue to evolve, the possibilities for data mining and AI applications will only expand, driving innovation and discovery across various fields.
If you want to read more articles similar to Enhancing Data Mining Techniques with Machine Learning and AI, you can visit the Applications category.
You Must Read