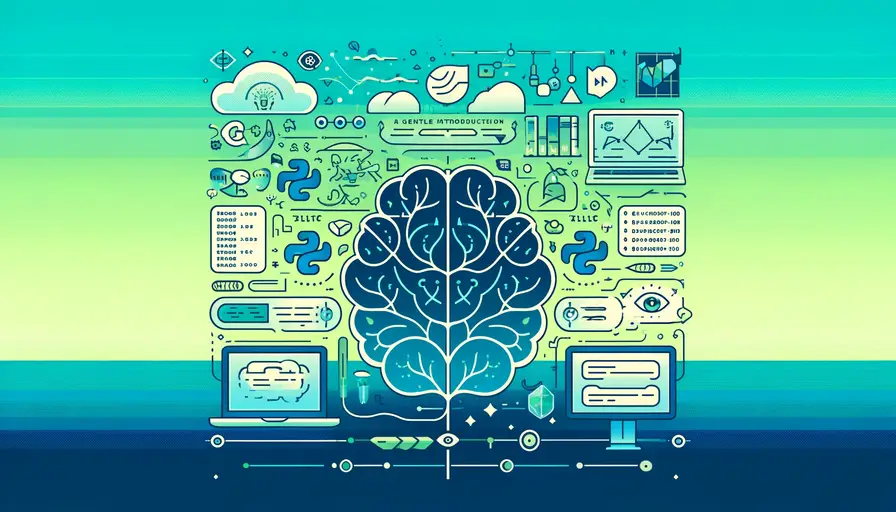
Extracting a Machine Learning Model: A Step-by-Step Guide
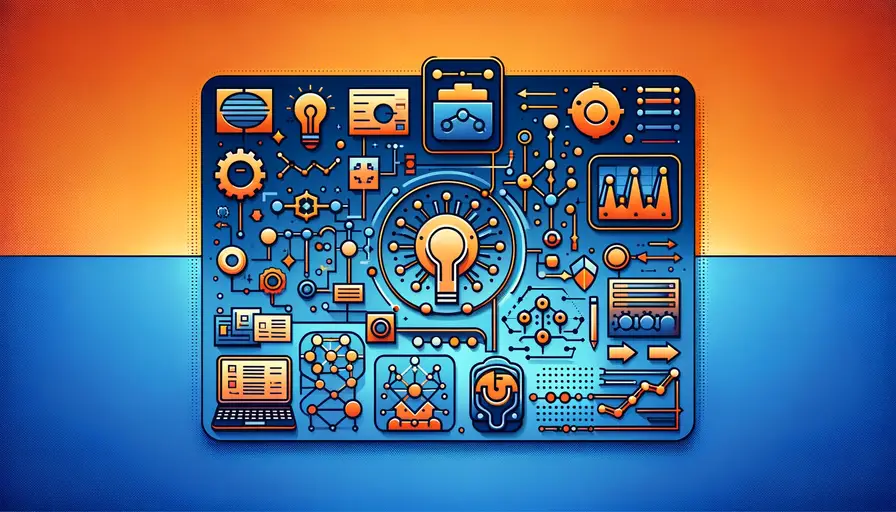
Creating and deploying a machine learning model involves several crucial steps, from data preprocessing to model evaluation and deployment. This guide provides a comprehensive, step-by-step process to help you extract and utilize a machine learning model effectively using popular libraries like Scikit-learn or TensorFlow.
- Scikit-learn or TensorFlow
- Train Your Machine Learning Model on a Suitable Dataset
- Evaluate the Performance of Your Trained Model Using Appropriate Metrics
- Save Your Trained Model to a File or Serialization Format
- Load the Saved Model Into Memory for Future Use
- Use the Loaded Model to Make Predictions on New Data
- Update and Iterate on Your Model as New Data Becomes Available or Requirements Change
Scikit-learn or TensorFlow
Choosing the right framework is essential for building your machine learning model. Scikit-learn is great for beginners and classical machine learning tasks, while TensorFlow is more suited for deep learning applications and complex neural networks.
Import the Necessary Libraries
Start by importing the necessary libraries for your project. This typically includes pandas for data manipulation, numpy for numerical operations, and the machine learning library you choose.
# Importing necessary libraries
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
import joblib
Load and Preprocess the Data
Loading and preprocessing your data is crucial for building an effective model. Ensure your data is clean and formatted correctly for the model.

# Loading the dataset
data = pd.read_csv('data.csv')
# Preprocessing the data
data.fillna(0, inplace=True) # Handling missing values
X = data.drop('target', axis=1) # Features
y = data['target'] # Target variable
Split the Data Into Training and Testing Sets
Splitting your data into training and testing sets helps evaluate your model's performance on unseen data.
# Splitting the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Build and Train the Machine Learning Model
Choose an appropriate machine learning algorithm and train your model.
# Building and training the model
model = LogisticRegression()
model.fit(X_train, y_train)
Evaluate and Fine-tune the Model
Evaluate the model using various metrics and fine-tune it to improve performance.
# Evaluating the model
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
precision = precision_score(y_test, y_pred)
recall = recall_score(y_test, y_pred)
f1 = f1_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
print(f'Precision: {precision}')
print(f'Recall: {recall}')
print(f'F1 Score: {f1}')
Train Your Machine Learning Model on a Suitable Dataset
Training your machine learning model involves feeding it with the right data and ensuring that it learns from the patterns within this data. This step is critical as the quality of the training data and the relevance of features significantly impact the model's performance.

Ensure your dataset is comprehensive and representative of the problem you're trying to solve. Preprocessing steps like normalization, handling missing values, and feature engineering can help improve the model's learning process.
Evaluate the Performance of Your Trained Model Using Appropriate Metrics
Evaluating the performance of your machine learning model involves using various metrics to understand how well it performs on the testing data. Different metrics provide different insights into the model's effectiveness.
Precision, Recall, and F1-Score
Precision, recall, and F1-score are crucial for classification tasks, especially when dealing with imbalanced datasets.
Accuracy
Accuracy measures the overall correctness of the model by calculating the ratio of correct predictions to total predictions.

Mean Squared Error (MSE)
Mean Squared Error (MSE) is commonly used for regression tasks to measure the average squared difference between the actual and predicted values.
Save Your Trained Model to a File or Serialization Format
Saving your trained model allows you to reuse it later without retraining, which saves time and computational resources.
Using Pickle
Pickle is a Python library for serializing and deserializing Python objects.
# Saving the model using pickle
import pickle
with open('model.pkl', 'wb') as file:
pickle.dump(model, file)
Using Joblib
Joblib is more efficient for storing large numpy arrays.

# Saving the model using joblib
joblib.dump(model, 'model.joblib')
Load the Saved Model Into Memory for Future Use
Loading the saved model allows you to make predictions on new data without retraining the model.
Import the Necessary Libraries
Ensure you have the necessary libraries to load the saved model.
import joblib
Load the Model
Load the model from the saved file.
# Loading the model
model = joblib.load('model.joblib')
Use the Loaded Model for Predictions
Utilize the loaded model to make predictions on new data.

# Making predictions with the loaded model
new_data = pd.read_csv('new_data.csv')
predictions = model.predict(new_data)
print(predictions)
Use the Loaded Model to Make Predictions on New Data
Using the loaded model, you can make predictions on new data, providing insights and aiding decision-making processes. Ensure the new data is preprocessed in the same way as the training data for consistent results.
Update and Iterate on Your Model as New Data Becomes Available or Requirements Change
Models need to be updated and iterated upon as new data becomes available or as the requirements change. This ensures that the model remains relevant and performs well over time.
Gather New Data
Collect new data regularly to keep the model updated and relevant.
Preprocess the Data
Preprocess the new data in the same manner as the initial data to maintain consistency.

Evaluate the Performance
Evaluate the model's performance with the new data to ensure it still meets the required standards.
Update the Model
Retrain or fine-tune the model with the new data to improve its performance and accuracy.
Test and Validate
Test the updated model thoroughly to ensure it works as expected and provides accurate predictions.
Monitor and Iterate
Continuously monitor the model's performance and iterate on it to address any issues or improvements needed.
Extracting a machine learning model involves several key steps: selecting the right framework, preprocessing data, training and evaluating the model, saving and loading the model, and continuously updating it. By following this comprehensive guide, you can effectively develop and deploy machine learning models that provide valuable insights and make accurate predictions.
If you want to read more articles similar to Extracting a Machine Learning Model: A Step-by-Step Guide, you can visit the Algorithms category.
You Must Read