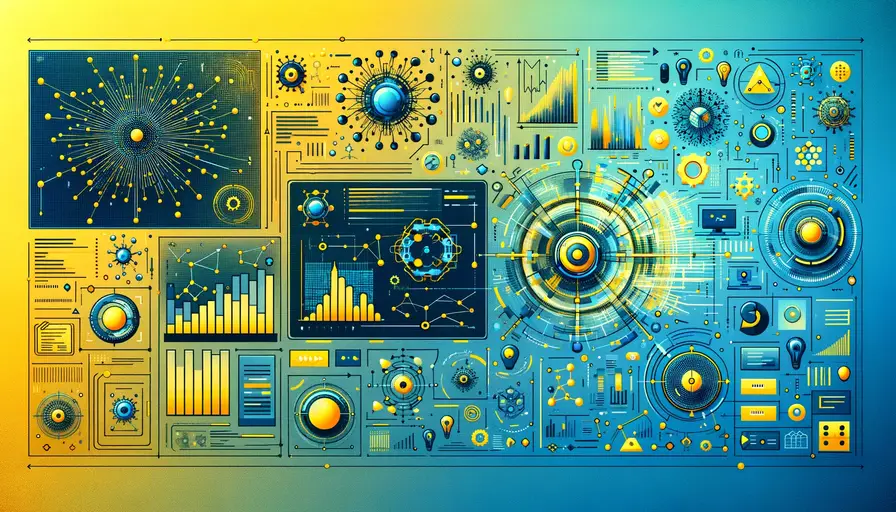
Mastering Robust and Efficient Machine Learning Systems
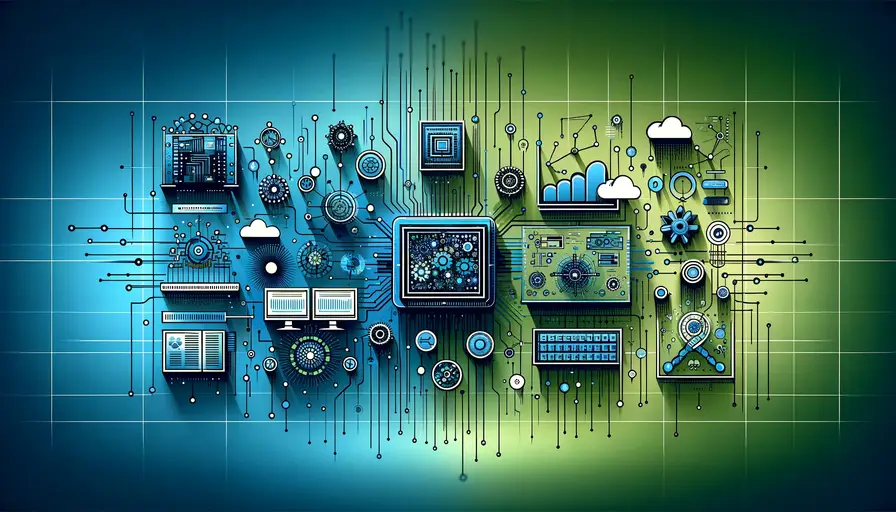
Developing robust and efficient machine learning systems is essential for leveraging the full potential of machine learning in various applications. This guide will cover key strategies to enhance the performance, reliability, and scalability of machine learning models, from using large datasets to optimizing hyperparameters and implementing distributed computing.
- Use Large and Diverse Datasets to Train Machine Learning Models
- Implement Regularized Models to Prevent Overfitting
- Optimize Hyperparameters to Improve Model Performance
- Use Model Compression Techniques to Reduce Model Size
- Implement Efficient Algorithms for Training and Inference
- Use Distributed Computing to Speed Up Model Training
Use Large and Diverse Datasets to Train Machine Learning Models
Benefits of Using Large and Diverse Datasets
Using large and diverse datasets significantly improves the performance and generalizability of machine learning models. Large datasets provide a comprehensive representation of the problem domain, capturing various patterns and nuances. This reduces the risk of overfitting, where the model performs well on training data but poorly on unseen data.
Diverse datasets ensure that the model is exposed to a wide range of scenarios and variations. This diversity helps the model learn more robust features and relationships, making it more resilient to changes and anomalies in new data. For instance, in image recognition, a dataset with images from different angles, lighting conditions, and backgrounds helps the model generalize better.
Moreover, large datasets enhance the model's ability to identify and learn subtle patterns that may not be evident in smaller datasets. This leads to improved accuracy and reliability. Additionally, more data allows for more effective use of complex models, such as deep neural networks, which require vast amounts of data to achieve their full potential.

import pandas as pd
from sklearn.model_selection import train_test_split
# Load a large and diverse dataset
data = pd.read_csv('large_dataset.csv')
# Preprocess the data
data.fillna(0, inplace=True)
X = data.drop('target', axis=1)
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Implement Regularized Models to Prevent Overfitting
Regularization is a technique used to prevent overfitting by adding a penalty to the model's complexity. Overfitting occurs when a model learns not only the underlying patterns but also the noise in the training data, leading to poor performance on new data. Regularized models constrain the model parameters, making them less sensitive to fluctuations in the training data.
There are several regularization techniques, including L1 (Lasso), L2 (Ridge), and Elastic Net. L1 regularization adds a penalty equal to the absolute value of the coefficients, promoting sparsity by shrinking some coefficients to zero. L2 regularization adds a penalty equal to the square of the coefficients, preventing any single coefficient from dominating. Elastic Net combines both L1 and L2 penalties.
Using regularized models helps maintain a balance between bias and variance, leading to more generalizable models. This is particularly important for high-dimensional datasets where the number of features is large compared to the number of observations.
from sklearn.linear_model import Ridge
# Implementing a regularized model
model = Ridge(alpha=1.0)
model.fit(X_train, y_train)
# Evaluate the model
y_pred = model.predict(X_test)
print(f'Model Performance: {model.score(X_test, y_test)}')
Optimize Hyperparameters to Improve Model Performance
Hyperparameter optimization involves finding the best set of parameters that maximizes the model's performance. Hyperparameters are external to the model and control the learning process, such as learning rate, number of trees in a forest, or the penalty in regularization.

There are several techniques for hyperparameter optimization, including grid search, random search, and Bayesian optimization. Grid search exhaustively searches through a specified parameter grid, while random search samples from the parameter grid randomly. Bayesian optimization uses probabilistic models to find the optimal parameters efficiently.
Optimizing hyperparameters can significantly enhance the performance of machine learning models by tuning them to the specific characteristics of the data. This process helps in finding a balance between underfitting and overfitting, leading to more accurate and reliable models.
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Define the parameter grid
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 10, 20, 30],
'min_samples_split': [2, 5, 10]
}
# Implement grid search for hyperparameter optimization
grid_search = GridSearchCV(RandomForestClassifier(), param_grid, cv=5)
grid_search.fit(X_train, y_train)
# Best parameters and model performance
print(f'Best Parameters: {grid_search.best_params_}')
print(f'Best Model Performance: {grid_search.best_score_}')
Use Model Compression Techniques to Reduce Model Size
Model compression techniques are essential for deploying machine learning models in resource-constrained environments, such as mobile devices or embedded systems. These techniques reduce the model size without significantly compromising performance, enabling faster inference and lower memory usage.
Common model compression techniques include quantization, pruning, and knowledge distillation. Quantization reduces the precision of the model's weights and activations, typically from 32-bit floating-point to 8-bit integer. Pruning removes less important weights, resulting in a sparser model. Knowledge distillation transfers knowledge from a large, complex model (teacher) to a smaller, simpler model (student).

Implementing model compression allows for the deployment of efficient and lightweight models, making machine learning accessible in various applications. These techniques help maintain high performance while optimizing resource usage.
import tensorflow as tf
# Example of model quantization
converter = tf.lite.TFLiteConverter.from_saved_model('saved_model_directory')
converter.optimizations = [tf.lite.Optimize.DEFAULT]
tflite_model = converter.convert()
# Save the compressed model
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
Implement Efficient Algorithms for Training and Inference
Training Algorithms
Efficient training algorithms are crucial for reducing training time and computational resources. Techniques such as stochastic gradient descent (SGD), mini-batch gradient descent, and adaptive learning rate methods (e.g., Adam, RMSprop) optimize the training process.
Stochastic gradient descent (SGD) updates the model parameters for each training example, while mini-batch gradient descent updates the parameters for a batch of examples. Adaptive learning rate methods adjust the learning rate based on the gradient, improving convergence speed and stability.
Inference Algorithms
Efficient inference algorithms are essential for real-time applications where quick predictions are required. Techniques such as early stopping, model pruning, and optimized data structures (e.g., KD-trees for nearest neighbor searches) enhance inference efficiency.

Optimizing training and inference algorithms ensures that machine learning models can be trained and deployed quickly, providing timely insights and predictions.
import tensorflow as tf
# Example of using Adam optimizer for training
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=0.001),
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Loss: {loss}, Accuracy: {accuracy}')
Use Distributed Computing to Speed Up Model Training
Distributed computing leverages multiple machines to parallelize the training process, significantly reducing training time for large datasets and complex models. Distributed computing frameworks, such as Apache Spark, TensorFlow's Distributed Strategy, and PyTorch's Distributed Data Parallel, enable efficient distribution of data and computation.
Implementing distributed computing involves dividing the dataset and model training across multiple nodes. Each node processes a subset of the data, and the results are aggregated to update the model. This approach allows for scaling machine learning tasks across clusters of machines, making it feasible to handle large-scale datasets and models.
The benefits of distributed computing include faster training times, the ability to process large datasets that do not fit into a single machine's memory, and improved model accuracy through the use of more extensive data. This approach is essential for organizations that require real-time insights and rapid model updates.

import tensorflow as tf
# Example of distributed training with TensorFlow
strategy = tf.distribute.MirroredStrategy()
with strategy.scope():
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(128, activation='relu', input_shape=(input_dim,)),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model using distributed strategy
model.fit(X_train, y_train, epochs=10, batch_size=32)
Mastering robust and efficient machine learning systems involves using large and diverse datasets, implementing regularized models, optimizing hyperparameters, compressing models, and employing efficient algorithms. Additionally, leveraging distributed computing can significantly speed up model training and improve overall performance. By following these best practices, you can develop machine learning models that are both powerful and efficient, capable of delivering accurate and timely insights across various applications.
If you want to read more articles similar to Mastering Robust and Efficient Machine Learning Systems, you can visit the Education category.
You Must Read