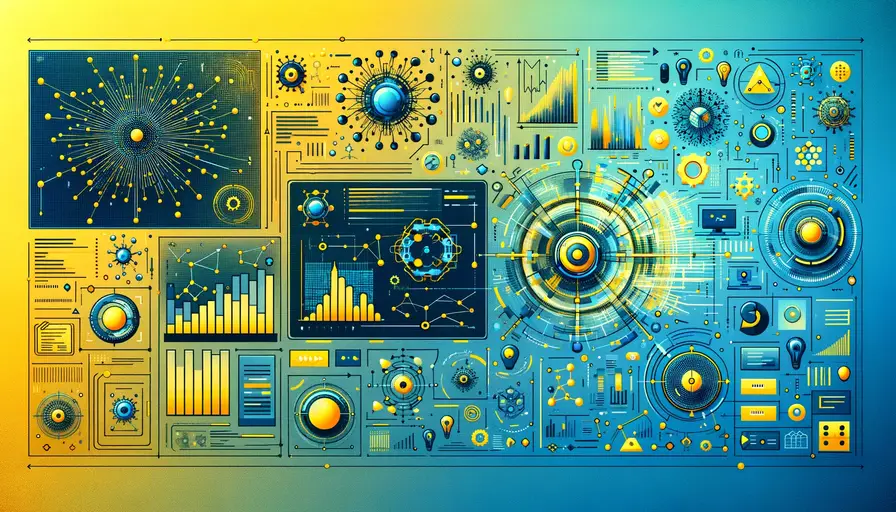
Predicting Categorical Variables with Linear Regression

- Appropriate Encoding Technique for Categorical Variables
- Include Interaction Terms
- Use Regularization Techniques
- Perform Feature Selection
- Use Cross-Validation
- Use Different Algorithms for Binary Variables
- Use Appropriate Evaluation Metrics
- Incorporate External Data and Domain Knowledge
- Continuously Update and Retrain
Appropriate Encoding Technique for Categorical Variables
Encoding Nominal Variables
Nominal variables are categorical variables without any inherent order. Encoding these variables is crucial when preparing data for linear regression, as the algorithm requires numerical input. One common method is one-hot encoding, which converts each category into a separate binary feature. For instance, a variable with categories "red," "blue," and "green" would be transformed into three binary features: "is_red", "is_blue", and "is_green."
One-hot encoding ensures that the encoded variables do not imply any ordinal relationship. This method is effective for nominal variables with a moderate number of categories. However, it can lead to a high-dimensional feature space if the variable has many categories, which might complicate the model.
Here’s an example of one-hot encoding in Python using pandas
:
import pandas as pd
# Sample data
data = pd.DataFrame({
'Color': ['Red', 'Blue', 'Green', 'Blue', 'Red']
})
# One-hot encoding
encoded_data = pd.get_dummies(data, columns=['Color'])
print(encoded_data)
This code demonstrates how to transform nominal variables into binary features suitable for linear regression.

Encoding Ordinal Variables
Ordinal variables have a defined order but no fixed interval between categories. Encoding these variables involves converting the categories into numerical values that reflect their order. Label encoding is a straightforward technique where each category is assigned a unique integer based on its rank.
For example, a variable with categories "low," "medium," and "high" could be encoded as 1, 2, and 3, respectively. This approach maintains the ordinal relationship between the categories. However, it’s essential to ensure that the encoding reflects the true ordinal nature of the variable to avoid misleading the regression model.
Here’s an example of label encoding in Python using pandas
:
import pandas as pd
# Sample data
data = pd.DataFrame({
'Size': ['Small', 'Medium', 'Large', 'Medium', 'Small']
})
# Label encoding
size_mapping = {'Small': 1, 'Medium': 2, 'Large': 3}
data['Size'] = data['Size'].map(size_mapping)
print(data)
This code shows how to encode ordinal variables while preserving their order.

Considerations and Precautions
Considerations and precautions are necessary when encoding categorical variables to ensure the quality and effectiveness of the regression model. One critical consideration is avoiding dummy variable trap, which occurs when one of the binary variables in one-hot encoding is perfectly collinear with the others. To prevent this, one category is usually dropped.
Another precaution is ensuring that the encoding reflects the actual data distribution and relationships. For instance, improper encoding of ordinal variables can mislead the model about the nature of the data. Additionally, when dealing with high-cardinality nominal variables, dimensionality reduction techniques or embedding methods might be required to manage the feature space effectively.
It’s also essential to consider the interpretability of the encoded variables. The chosen encoding technique should not only facilitate accurate predictions but also allow for meaningful interpretation of the model coefficients.
Include Interaction Terms
Interaction terms between categorical and continuous variables can capture complex relationships that linear regression alone might miss. Including these terms in the regression model allows it to account for the combined effect of variables on the outcome. For example, the effect of a continuous variable, such as income, might differ across categories of a variable like education level.

Creating interaction terms involves multiplying the categorical variable (often encoded) by the continuous variable. This process can be implemented in Python using the statsmodels
library, which provides an easy way to include interaction terms in the regression model.
Here’s an example:
import pandas as pd
import statsmodels.api as sm
# Sample data
data = pd.DataFrame({
'Income': [50000, 60000, 70000, 80000, 90000],
'Education': ['HighSchool', 'Bachelors', 'Masters', 'PhD', 'Bachelors']
})
# One-hot encoding
data = pd.get_dummies(data, columns=['Education'], drop_first=True)
# Create interaction term
data['Income_Education_Bachelors'] = data['Income'] * data['Education_Bachelors']
# Fit linear regression model
X = data[['Income', 'Education_Bachelors', 'Income_Education_Bachelors']]
X = sm.add_constant(X) # Add intercept
y = [1, 0, 0, 1, 0] # Sample target variable
model = sm.OLS(y, X).fit()
print(model.summary())
This example demonstrates how to include interaction terms in a linear regression model.
Use Regularization Techniques
Regularization techniques, such as Lasso or Ridge regression, are crucial for handling multicollinearity and improving model generalization. These techniques add a penalty to the model's complexity, discouraging it from fitting too closely to the training data and thus improving its performance on unseen data.

Lasso regression (Least Absolute Shrinkage and Selection Operator) adds a penalty equal to the absolute value of the magnitude of coefficients. This technique can shrink some coefficients to zero, effectively performing feature selection. Ridge regression, on the other hand, adds a penalty equal to the square of the magnitude of coefficients, which helps reduce the impact of multicollinearity without eliminating any variables.
Here’s an example of using Lasso regression in Python with sklearn
:
from sklearn.linear_model import Lasso
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# Sample data
X = data[['Income', 'Education_Bachelors', 'Income_Education_Bachelors']]
y = [1, 0, 0, 1, 0] # Sample target variable
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Fit Lasso regression model
lasso = Lasso(alpha=0.1)
lasso.fit(X_train, y_train)
# Predict and evaluate
y_pred = lasso.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print("Mean Squared Error:", mse)
print("Coefficients:", lasso.coef_)
This example shows how to apply Lasso regression to a dataset with interaction terms.
Perform Feature Selection
Feature selection is a crucial step in identifying the most important categorical variables for the regression model. By selecting relevant features, we can improve model accuracy and interpretability while reducing complexity. Techniques for feature selection include filter methods, wrapper methods, and embedded methods.

Filter methods rank features based on statistical tests such as chi-square or mutual information and select the top-ranked ones. Wrapper methods, like recursive feature elimination (RFE), iteratively train the model and remove the least important features. Embedded methods, such as those used in regularization techniques like Lasso, perform feature selection during model training.
Here’s an example of feature selection using RFE in Python:
from sklearn.feature_selection import RFE
from sklearn.linear_model import LinearRegression
# Sample data
X = data[['Income', 'Education_Bachelors', 'Income_Education_Bachelors']]
y = [1, 0, 0, 1, 0] # Sample target variable
# Fit linear regression model
model = LinearRegression()
rfe = RFE(model, n_features_to_select=2)
fit = rfe.fit(X, y)
# Print selected features
print("Selected Features:", fit.support_)
print("Feature Ranking:", fit.ranking_)
This code demonstrates how to use RFE for feature selection in a regression model.
Use Cross-Validation
Cross-validation is essential for evaluating the performance of the regression model and ensuring its generalizability to new data. By partitioning the dataset into multiple subsets and training the model on different folds, cross-validation provides a more reliable estimate of model performance.

K-fold cross-validation is a popular method where the dataset is divided into K equal parts. The model is trained on K-1 parts and tested on the remaining part. This process is repeated K times, with each part used as the test set once. The performance metrics are averaged across all folds to obtain a robust evaluation.
Here’s an example of implementing K-fold cross-validation in Python using sklearn
:
from sklearn.model_selection import cross_val_score
from sklearn.linear_model import LinearRegression
# Sample data
X = data[['Income', 'Education_Bachelors', 'Income_Education_Bachelors']]
y = [1, 0, 0, 1, 0] # Sample target variable
# Fit linear regression model
model = LinearRegression()
# Perform 5-fold cross-validation
scores = cross_val_score(model, X, y, cv=5, scoring='neg_mean_squared_error')
print("Cross-Validation Scores:", scores)
print("Mean Score:", scores.mean())
This code demonstrates how to evaluate the model using K-fold cross-validation.
Use Different Algorithms for Binary Variables
Logistic regression is often more appropriate for predicting binary categorical variables than linear regression. Logistic regression models the probability that a given input belongs to a particular category, making it suitable for classification tasks. Unlike linear regression, logistic regression outputs probabilities that are constrained between 0 and 1, ensuring meaningful predictions for binary outcomes.
In logistic regression, the relationship between the dependent variable and independent variables is modeled using the logistic function, which transforms the linear combination of inputs into a probability. This approach allows for effective handling of binary classification problems.
Here’s an example of logistic regression in Python using sklearn
:
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Sample data
X = data[['Income', 'Education_Bachelors', 'Income_Education_Bachelors']]
y = [1, 0, 0, 1, 0] # Sample target variable
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Fit logistic regression model
log_reg = LogisticRegression()
log_reg.fit(X_train, y_train)
# Predict and evaluate
y_pred = log_reg.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
print("Coefficients:", log_reg.coef_)
This example demonstrates how to use logistic regression for binary classification.
Use Appropriate Evaluation Metrics
Appropriate evaluation metrics are essential for assessing the performance of a regression model, especially when predicting categorical variables. Metrics such as accuracy, precision, recall, and F1-score provide a comprehensive view of model performance, particularly for classification tasks.
Accuracy measures the proportion of correct predictions out of the total predictions. However, it may not be sufficient for imbalanced datasets. Precision and recall provide deeper insights: precision measures the proportion of true positive predictions among all positive predictions, while recall measures the proportion of true positives among all actual positives. The F1-score is the harmonic mean of precision and recall, offering a balanced evaluation.
Here’s an example of calculating these metrics in Python using sklearn
:
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
# Sample predictions and true labels
y_true = [1, 0, 0, 1, 0]
y_pred = [1, 0, 1, 1, 0]
# Calculate evaluation metrics
accuracy = accuracy_score(y_true, y_pred)
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
print("Accuracy:", accuracy)
print("Precision:", precision)
print("Recall:", recall)
print("F1-Score:", f1)
This code demonstrates how to calculate and print key evaluation metrics for a classification model.
Incorporate External Data and Domain Knowledge
Incorporating external data sources and domain knowledge can significantly improve the predictions of a regression model. External data can provide additional context and features that enhance the model’s ability to capture relevant patterns. Domain knowledge helps in selecting meaningful features, engineering new ones, and interpreting the results.
For instance, in a retail sales prediction model, incorporating economic indicators, weather data, or social media sentiment can provide a more comprehensive view of the factors influencing sales. Domain expertise can guide the selection of these external data sources and ensure they are integrated effectively.
Here’s an example of incorporating external data in Python:
import pandas as pd
# Sample internal data
internal_data = pd.DataFrame({
'Sales': [200, 220, 250, 270, 300],
'Advertising': [20, 25, 30, 35, 40]
})
# Sample external data (e.g., economic indicators)
external_data = pd.DataFrame({
'Economic_Index': [1.2, 1.3, 1.5, 1.7, 1.8]
})
# Combine internal and external data
combined_data = pd.concat([internal_data, external_data], axis=1)
print(combined_data)
This example demonstrates how to combine internal and external data for model training.
Continuously Update and Retrain
Continuously updating and retraining the model as new data becomes available is crucial for maintaining its accuracy and relevance. The data distribution and relationships between variables can change over time, necessitating regular updates to the model. Continuous learning ensures that the model adapts to new patterns and remains effective in dynamic environments.
Retraining the model involves periodically retraining it on the latest available data, incorporating new features, and re-evaluating its performance. Automation of this process through pipelines can streamline the updating process, ensuring timely and efficient updates.
Here’s an example of automating model retraining in Python:
import pandas as pd
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
# Function to retrain the model
def retrain_model(data):
X = data[['Advertising', 'Economic_Index']]
y = data['Sales']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
return model
# Sample new data
new_data = pd.DataFrame({
'Sales': [310, 320, 330],
'Advertising': [45, 50, 55],
'Economic_Index': [1.9, 2.0, 2.1]
})
# Retrain the model
model = retrain_model(new_data)
print("Retrained Model Coefficients:", model.coef_)
This code demonstrates how to retrain a regression model with new data.
Predicting categorical variables with linear regression involves several key steps and considerations. From using appropriate encoding techniques and incorporating interaction terms to employing regularization and feature selection, each step enhances the model's performance. Cross-validation, logistic regression for binary variables, appropriate evaluation metrics, and incorporating external data are crucial for robust model evaluation and improvement. Continuous updates and retraining ensure the model remains effective over time, adapting to new data and evolving patterns. By following these practices, we can build accurate and reliable models for predicting categorical variables.
If you want to read more articles similar to Predicting Categorical Variables with Linear Regression, you can visit the Education category.
You Must Read