
Decoding Machine Learning Models: Deterministic or Probabilistic?
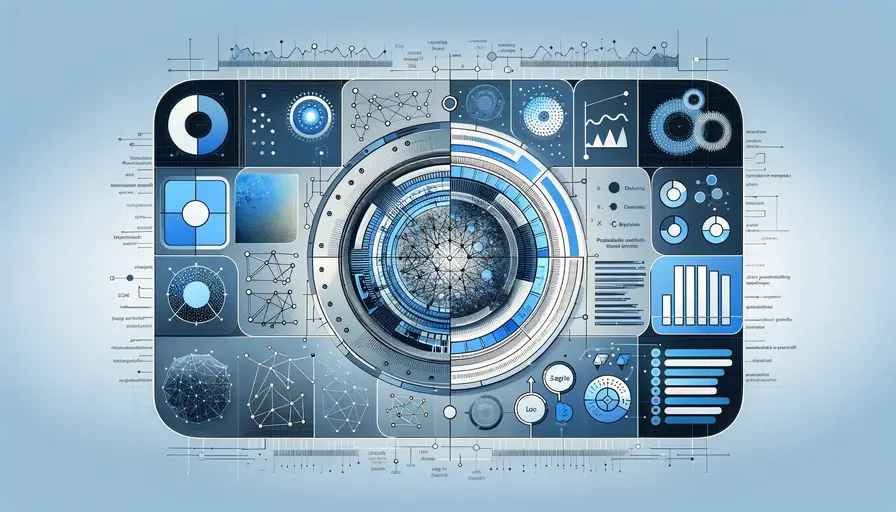
Machine learning models can be broadly categorized into deterministic and probabilistic models, each offering distinct advantages and applications. This article explores these two types of models, highlighting their key features, real-world applications, and practical examples to provide a comprehensive understanding of their differences and uses.
Key Features of Deterministic Models
Defining Deterministic Models
Deterministic models are characterized by their predictability and consistency. Given a specific set of inputs, a deterministic model will always produce the same output. This predictability makes deterministic models particularly useful in scenarios where consistency and reliability are crucial.
Deterministic models are typically used in applications where the relationships between variables are well understood and can be precisely modeled. For example, linear regression is a common deterministic model used to predict a dependent variable based on one or more independent variables.
The simplicity of deterministic models also makes them easier to interpret and explain. Because the output is a direct function of the input variables, it is straightforward to understand how changes in the input affect the output.
Understanding the Concept of Epochs in Machine LearningCommon Deterministic Algorithms
Several algorithms fall under the category of deterministic models. Linear regression is perhaps the most well-known, used to model the relationship between a dependent variable and one or more independent variables. The model assumes a linear relationship and aims to find the best-fitting line that minimizes the difference between observed and predicted values.
Decision trees are another example of deterministic models. These models split the data into branches based on certain conditions, creating a tree-like structure that can be used for both classification and regression tasks. The paths in the tree represent the decision rules, leading to a final output.
Support vector machines (SVMs) are deterministic models used for classification tasks. They work by finding the hyperplane that best separates different classes in the data. The position of the hyperplane is determined by the support vectors, which are the data points closest to the boundary.
Here is an example of a decision tree using scikit-learn:
Unit Testing for Machine Learning Modelsfrom sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn import tree
import matplotlib.pyplot as plt
# Load the dataset
data = load_iris()
X = data.data
y = data.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train a Decision Tree classifier
model = DecisionTreeClassifier(random_state=42)
model.fit(X_train, y_train)
# Plot the decision tree
plt.figure(figsize=(12,8))
tree.plot_tree(model, filled=True, feature_names=data.feature_names, class_names=data.target_names)
plt.show()
This code demonstrates how to train a decision tree classifier and visualize the resulting tree, illustrating the deterministic nature of the model.
Applications of Deterministic Models
Deterministic models are widely used in various applications due to their predictability and ease of interpretation. In finance, linear regression models are employed to predict stock prices, assess risk, and model economic relationships. These models provide straightforward and reliable predictions, making them valuable tools for financial analysis.
In healthcare, deterministic models like decision trees are used for diagnosing diseases, predicting patient outcomes, and optimizing treatment plans. The interpretability of these models allows healthcare professionals to understand the decision-making process, enhancing trust and transparency in medical decisions.
Manufacturing industries also benefit from deterministic models for quality control, process optimization, and predictive maintenance. By modeling the relationships between process variables, these models help in identifying factors that impact product quality and efficiency, leading to improved production processes.
Is Linear Regression Considered a Machine Learning Algorithm?Key Features of Probabilistic Models
Defining Probabilistic Models
Probabilistic models, unlike deterministic models, incorporate uncertainty and variability in their predictions. These models provide not only a prediction but also a probability distribution over possible outcomes. This characteristic makes probabilistic models particularly useful in scenarios where uncertainty and risk need to be quantified.
Probabilistic models are based on probability theory and statistics, allowing them to capture the inherent randomness in data. By modeling the probability distributions of variables, these models can provide insights into the likelihood of different outcomes, which is crucial in many decision-making processes.
The flexibility of probabilistic models also enables them to handle complex and uncertain environments more effectively. They can incorporate prior knowledge and update predictions as new data becomes available, making them adaptive and robust in dynamic settings.
Common Probabilistic Algorithms
Several algorithms are classified as probabilistic models. Naive Bayes is a popular probabilistic model used for classification tasks. It is based on Bayes' theorem and assumes that the features are conditionally independent given the class label. Despite this simplifying assumption, Naive Bayes often performs well in practice, especially for text classification tasks.
Supervised vs Unsupervised Learning: Understanding the DifferenceHidden Markov Models (HMMs) are probabilistic models used for sequence data, such as time series or speech signals. HMMs model the system as a Markov process with hidden states, allowing them to capture temporal dependencies and uncertainties in the data.
Gaussian Mixture Models (GMMs) are used for clustering and density estimation. They assume that the data is generated from a mixture of several Gaussian distributions, each representing a cluster. GMMs can capture the underlying structure of the data and provide probabilistic assignments of data points to clusters.
Here is an example of a Gaussian Mixture Model using scikit-learn:
from sklearn.datasets import make_blobs
from sklearn.mixture import GaussianMixture
import matplotlib.pyplot as plt
# Generate synthetic data
X, _ = make_blobs(n_samples=300, centers=3, cluster_std=0.60, random_state=42)
# Apply Gaussian Mixture Model
gmm = GaussianMixture(n_components=3, random_state=42)
gmm.fit(X)
labels = gmm.predict(X)
# Plot the clustered data
plt.scatter(X[:, 0], X[:, 1], c=labels, s=40, cmap='viridis')
plt.title('Gaussian Mixture Model Clustering')
plt.show()
This code demonstrates how to apply a Gaussian Mixture Model for clustering, showcasing the probabilistic nature of the model.
Is Deep Learning part of AI or ML?Applications of Probabilistic Models
Probabilistic models are widely used in applications that involve uncertainty and risk assessment. In finance, probabilistic models such as GARCH (Generalized Autoregressive Conditional Heteroskedasticity) are used to model and forecast financial volatility. These models help in assessing the risk of investment portfolios and making informed trading decisions.
In healthcare, probabilistic models like Bayesian networks are employed for disease diagnosis and prognosis. By incorporating prior knowledge and updating predictions based on new evidence, these models provide a flexible and robust framework for medical decision-making under uncertainty.
Natural language processing (NLP) also benefits from probabilistic models. Hidden Markov Models (HMMs) are used for tasks such as speech recognition and part-of-speech tagging. By modeling the probability distributions of sequences, HMMs can effectively handle the variability and ambiguity inherent in natural language.
Comparing Deterministic and Probabilistic Models
Interpretability and Transparency
One of the key differences between deterministic and probabilistic models is their interpretability and transparency. Deterministic models are generally easier to interpret because they provide a clear and direct mapping from inputs to outputs. The decision-making process is transparent, allowing users to understand how the model arrived at a particular prediction.
The Formula for Calculating Y Hat in Machine Learning ExplainedFor example, in a decision tree, the paths from the root to the leaf nodes represent the decision rules, making it straightforward to interpret and explain the model's predictions. This interpretability is particularly valuable in applications where transparency is crucial, such as healthcare and finance.
Probabilistic models, on the other hand, can be more complex and less transparent. They provide probability distributions over possible outcomes, which can be harder to interpret and explain. However, this probabilistic nature allows them to capture uncertainty and provide a more nuanced understanding of the predictions.
Handling Uncertainty
Another significant difference between deterministic and probabilistic models is their ability to handle uncertainty. Deterministic models do not account for uncertainty in their predictions. Given the same inputs, they always produce the same output, which can be a limitation in scenarios where uncertainty and variability are inherent.
Probabilistic models excel in handling uncertainty. They provide probability distributions over possible outcomes, quantifying the uncertainty in the predictions. This ability to capture and represent uncertainty is particularly valuable in decision-making processes where risk assessment is crucial.
For example, in financial forecasting, probabilistic models can provide a range of possible future scenarios with associated probabilities, allowing investors to assess the risk and make informed decisions. Similarly, in medical diagnosis, probabilistic models can quantify the likelihood of different diseases, helping doctors make more informed and confident decisions.
Computational Complexity
The computational complexity of deterministic and probabilistic models can also differ significantly. Deterministic models are often simpler and faster to train and evaluate. Their predictability and straightforward calculations make them suitable for applications where computational resources and time are limited.
Probabilistic models, on the other hand, can be more computationally intensive. The need to estimate probability distributions and handle uncertainty can increase the complexity of the models. This increased complexity can require more computational resources and longer training times.
However, advances in computing power and algorithms have made it increasingly feasible to use probabilistic models in practical applications. The trade-off between computational complexity and the ability to handle uncertainty and provide probabilistic insights often justifies the use of probabilistic models in many scenarios.
Practical Examples and Case Studies
Predicting Housing Prices with Linear Regression
Linear regression is a common deterministic model used for predicting continuous variables. In this example, we will use linear regression to predict housing prices based on features such as square footage, number of bedrooms, and age of the house.
from sklearn.datasets import fetch_california_housing
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Load the dataset
data = fetch_california_housing()
X = data.data
y = data.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train a Linear Regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions and evaluate the model
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
This code demonstrates how to use linear regression to predict housing prices, illustrating the deterministic nature of the model.
Spam Detection with Naive Bayes
Naive Bayes is a probabilistic model commonly used for classification tasks such as spam detection. In this example, we will use Naive Bayes to classify emails as spam or not spam based on their content.
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
# Load the dataset
emails = pd.read_csv('emails.csv')
X = emails['text']
y = emails['spam']
# Convert text data to a matrix of token counts
vectorizer = CountVectorizer(stop_words='english')
X = vectorizer.fit_transform(X)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train a Naive Bayes model
model = MultinomialNB()
model.fit(X_train, y_train)
# Make predictions and evaluate the model
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
This code demonstrates how to use Naive Bayes for spam detection, highlighting the probabilistic nature of the model.
Clustering Customer Data with Gaussian Mixture Models
Gaussian Mixture Models (GMMs) are probabilistic models used for clustering. In this example, we will use GMMs to cluster customer data based on purchasing behavior.
from sklearn.mixture import GaussianMixture
import pandas as pd
# Load the dataset
data = pd.read_csv('customer_data.csv')
X = data[['Annual Income', 'Spending Score']]
# Apply Gaussian Mixture Model
gmm = GaussianMixture(n_components=3, random_state=42)
gmm.fit(X)
labels = gmm.predict(X)
# Add cluster labels to the dataset
data['Cluster'] = labels
print(data.head())
This code demonstrates how to use Gaussian Mixture Models for clustering customer data, showcasing the probabilistic nature of the model.
Decoding machine learning models involves understanding the key differences between deterministic and probabilistic models. Deterministic models are characterized by their predictability, simplicity, and ease of interpretation, making them suitable for applications where consistency and transparency are crucial. Probabilistic models, on the other hand, excel in handling uncertainty and providing probabilistic insights, making them valuable in scenarios where risk assessment and flexibility are important.
By leveraging the strengths of both deterministic and probabilistic models, practitioners can tackle a wide range of data challenges and make informed decisions based on the specific requirements of their applications. Whether it is predicting housing prices, detecting spam, or clustering customer data, the choice of model depends on the nature of the problem and the desired outcomes.
If you want to read more articles similar to Decoding Machine Learning Models: Deterministic or Probabilistic?, you can visit the Artificial Intelligence category.
You Must Read