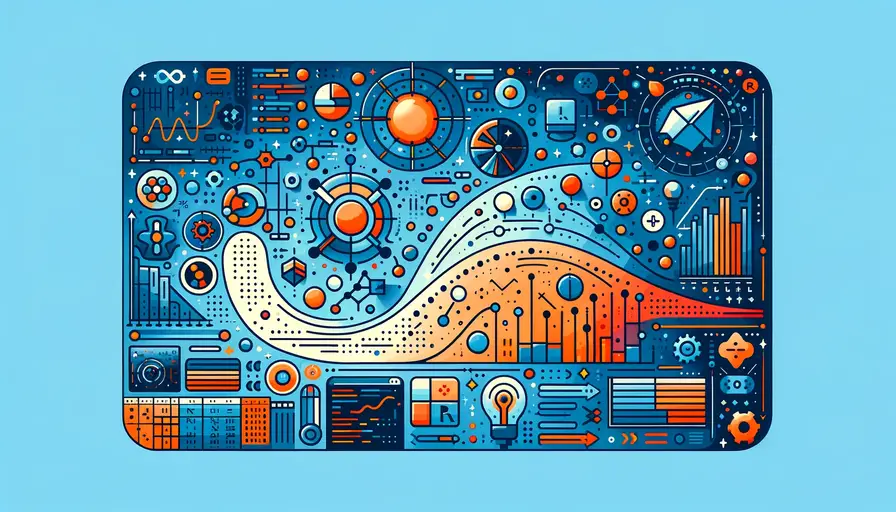
Best Websites With Extensive Reinforcement Learning Models Collection

Reinforcement Learning
Reinforcement Learning (RL) is a branch of machine learning where an agent learns to make decisions by performing actions in an environment to maximize cumulative reward. This learning paradigm is inspired by behavioral psychology and has applications in various fields, including robotics, game playing, and autonomous systems.
What Is Reinforcement Learning?
Reinforcement learning involves an agent that interacts with an environment through actions and receives feedback in the form of rewards or penalties. The agent's goal is to learn a policy that maximizes the long-term reward. Unlike supervised learning, RL does not rely on labeled data but rather on exploration and exploitation of the environment.
Key Concepts in Reinforcement Learning
Key concepts in RL include the agent, environment, state, action, reward, and policy. The agent is the learner or decision maker, the environment is the external system the agent interacts with, state represents the current situation, action is the decision made by the agent, reward is the feedback received, and policy is the strategy the agent follows to determine actions.
Example: Basic Q-Learning Algorithm in Python
Here’s an example of implementing a basic Q-Learning algorithm using Python:

import numpy as np
# Initialize parameters
num_states = 5
num_actions = 2
q_table = np.zeros((num_states, num_actions))
learning_rate = 0.1
discount_factor = 0.9
epsilon = 0.1
# Q-Learning algorithm
for episode in range(1000):
state = np.random.randint(0, num_states)
done = False
while not done:
if np.random.uniform(0, 1) < epsilon:
action = np.random.randint(0, num_actions) # Explore
else:
action = np.argmax(q_table[state, :]) # Exploit
next_state = (state + action) % num_states
reward = -1 if next_state == 0 else 1
q_table[state, action] = q_table[state, action] + learning_rate * (reward + discount_factor * np.max(q_table[next_state, :]) - q_table[state, action])
state = next_state
if state == 0:
done = True
print(q_table)
Websites With Reinforcement Learning Models
There are several excellent websites that provide extensive collections of reinforcement learning models, tutorials, and resources. These websites are valuable for both beginners and advanced practitioners looking to enhance their knowledge and skills in RL.
OpenAI
OpenAI is a leading research organization dedicated to developing and promoting friendly AI. OpenAI provides various RL models, research papers, and tutorials, making it an invaluable resource for anyone interested in reinforcement learning.
Key Features of OpenAI
OpenAI offers a range of environments and toolkits, such as OpenAI Gym, which provides a diverse set of environments for training RL agents. The platform also offers pre-trained models, detailed documentation, and a supportive community.
Example: Using OpenAI Gym in Python
Here’s an example of using OpenAI Gym to create an RL environment in Python:

import gym
# Create environment
env = gym.make('CartPole-v1')
# Reset environment
state = env.reset()
# Run an episode
done = False
while not done:
action = env.action_space.sample() # Random action
next_state, reward, done, info = env.step(action)
env.render()
env.close()
DeepMind
DeepMind, a subsidiary of Alphabet Inc., is a pioneer in AI research, particularly in reinforcement learning. DeepMind's work on RL has led to groundbreaking achievements, such as AlphaGo and AlphaZero. The website offers research papers, models, and tutorials on advanced RL techniques.
Key Features of DeepMind
DeepMind provides access to state-of-the-art RL research, including details on algorithms like Deep Q-Networks (DQN), AlphaGo, and AlphaZero. The website also offers educational resources and collaboration opportunities for researchers and practitioners.
Example: Training a Deep Q-Network
Here’s an example of implementing a basic Deep Q-Network (DQN) using TensorFlow:
import tensorflow as tf
from tensorflow.keras import layers
import numpy as np
import gym
# Create environment
env = gym.make('CartPole-v1')
# Define Q-network
def build_q_network(state_shape, action_shape):
model = tf.keras.Sequential([
layers.Dense(24, activation='relu', input_shape=state_shape),
layers.Dense(24, activation='relu'),
layers.Dense(action_shape, activation='linear')
])
model.compile(optimizer='adam', loss='mse')
return model
# Parameters
num_episodes = 1000
max_steps = 200
epsilon = 0.1
discount_factor = 0.99
# Initialize Q-network
q_network = build_q_network((4,), env.action_space.n)
# Training loop
for episode in range(num_episodes):
state = env.reset()
state = np.reshape(state, [1, 4])
for step in range(max_steps):
if np.random.uniform(0, 1) < epsilon:
action = env.action_space.sample()
else:
action = np.argmax(q_network.predict(state))
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, 4])
target = reward
if not done:
target += discount_factor * np.max(q_network.predict(next_state))
target_f = q_network.predict(state)
target_f[0][action] = target
q_network.fit(state, target_f, epochs=1, verbose=0)
state = next_state
if done:
break
print("Training completed.")
Educational Platforms for Reinforcement Learning
Educational platforms offer structured courses, tutorials, and projects on reinforcement learning. These platforms are ideal for learners seeking a guided and comprehensive approach to mastering RL.

Coursera
Coursera offers a wide range of courses on machine learning and reinforcement learning from top universities and institutions. These courses cover fundamental to advanced topics, providing hands-on projects and certifications.
Key Features of Coursera
Coursera provides courses from renowned institutions like Stanford University and the University of Alberta. It offers flexible learning schedules, peer-reviewed assignments, and real-world projects to enhance practical skills in RL.
Example: Enrolling in a Coursera Course
Here’s how to enroll in a reinforcement learning course on Coursera:
- Visit the Coursera website.
- Search for "reinforcement learning".
- Select a course and click "Enroll".
- Follow the prompts to complete the enrollment process.
edX
edX is another popular platform offering courses on reinforcement learning from prestigious universities like MIT and Harvard. edX provides both free and paid courses with certificates.

Key Features of edX
edX offers in-depth courses on RL, featuring video lectures, quizzes, and hands-on labs. The platform supports self-paced learning and provides access to course materials even after completion.
Example: Accessing a Course on edX
Here’s how to access a reinforcement learning course on edX:
- Visit the edX website.
- Search for "reinforcement learning".
- Choose a course and click "Enroll Now".
- Complete the registration to start learning.
Open-Source Repositories for Reinforcement Learning
Open-source repositories provide code implementations, pre-trained models, and extensive documentation for reinforcement learning projects. These repositories are valuable for experimentation and learning from existing work.
GitHub
GitHub is the largest platform for hosting open-source projects, including numerous repositories on reinforcement learning. Users can access code, contribute to projects, and collaborate with other developers.

Key Features of GitHub
GitHub offers version control, issue tracking, and collaborative tools. It hosts repositories for popular RL frameworks and projects, such as OpenAI Baselines, DQN, and PPO. Users can fork repositories, submit pull requests, and explore various implementations.
Example: Cloning a GitHub Repository
Here’s an example of cloning a reinforcement learning repository from GitHub:
# Clone repository
git clone https://github.com/openai/baselines.git
# Navigate to repository directory
cd baselines
# Install dependencies
pip install -r requirements.txt
Papers with Code
Papers with Code is a platform that links academic papers with their corresponding code implementations. It is an excellent resource for finding state-of-the-art reinforcement learning models and code.
Key Features of Papers with Code
Papers with Code categorizes research papers by task and model, providing links to GitHub repositories and performance metrics. Users can filter by dataset, task, and method to find relevant papers and code.

Example: Finding RL Papers and Code
Here’s how to find reinforcement learning papers and code on Papers with Code:
- Visit the Papers with Code website.
- Search for "reinforcement learning".
- Browse the list of papers and click on a paper title.
- Access the code implementation through the provided GitHub link.
Reinforcement Learning Competitions and Challenges
Participating in competitions and challenges can enhance your RL skills by providing practical experience and exposure to real-world problems.
Kaggle
Kaggle is a platform for data science competitions, offering challenges in various domains, including reinforcement learning. Competitions provide datasets, problem descriptions, and evaluation metrics.
Key Features of Kaggle
Kaggle offers a collaborative environment where participants can form teams, share code, and compete for prizes. It provides kernels (notebooks) for running code in the cloud and discussion forums for community support.
Example: Participating in a Kaggle Competition
Here’s how to participate in a Kaggle competition:
- Visit the Kaggle website.
- Search for "reinforcement learning" competitions.
- Select a competition and click "Join Competition".
- Follow the instructions to download data and submit predictions.
OpenAI Gym Challenges
OpenAI Gym offers various environments and challenges specifically designed for reinforcement learning. Users can test and improve their RL models on standardized tasks.
Key Features of OpenAI Gym Challenges
OpenAI Gym provides a variety of environments ranging from simple control tasks to complex robotics simulations. It supports benchmarking and comparison of different RL algorithms.
Example: Solving a Gym Challenge
Here’s how to solve a challenge in OpenAI Gym:
import gym
# Create environment
env = gym.make('MountainCar-v0')
# Reset environment
state = env.reset()
# Run an episode
done = False
while not done:
action = env.action_space.sample() # Random action
next_state, reward, done, info = env.step(action)
env.render()
env.close()
Learning and implementing reinforcement learning models can be significantly enhanced by leveraging the extensive resources available on various websites. Platforms like OpenAI, DeepMind, Coursera, edX, GitHub, and Papers with Code provide a wealth of knowledge, tutorials, and code implementations. Additionally, participating in competitions on platforms like Kaggle and OpenAI Gym can offer practical experience and exposure to real-world challenges. By utilizing these resources, you can deepen your understanding of RL, improve your skills, and contribute to the field of artificial intelligence.
If you want to read more articles similar to Best Websites With Extensive Reinforcement Learning Models Collection, you can visit the Applications category.
You Must Read