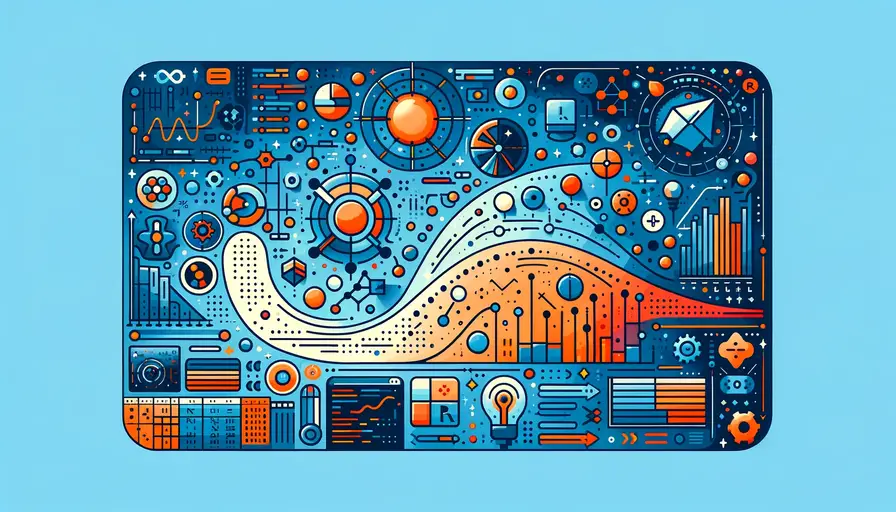
Essential Tips for Tackling Machine Learning Problems Successfully

- Understand the Problem Domain Thoroughly
- Gather and Prepare High-Quality Data
- Select the Appropriate Machine Learning Algorithm
- Properly Train and Validate the Model
- Regularly Monitor and Update the Model
- Optimize Hyperparameters for Better Performance
- Handle Missing or Noisy Data Effectively
- Feature Engineering for Better Representation
- Regularize the Model to Avoid Overfitting
- Interpret and Analyze Model Predictions
Understand the Problem Domain Thoroughly
Understanding the problem domain is crucial for the success of any machine learning project. A deep comprehension of the domain helps in defining the problem accurately and selecting the appropriate methods and algorithms.
Perform Comprehensive Data Preprocessing
Effective data preprocessing is vital for the accuracy of machine learning models. It involves cleaning the data, handling missing values, normalizing features, and encoding categorical variables. Proper preprocessing ensures that the data fed into the model is of high quality.
Choose the Right Evaluation Metrics
Choosing the correct evaluation metrics is essential for assessing the performance of your model. Metrics such as accuracy, precision, recall, F1-score, and ROC-AUC are commonly used, depending on the problem type. Selecting the right metric helps in making informed decisions about model improvements.
Here's an example of calculating evaluation metrics using Python and scikit-learn:

from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
# Sample data
y_true = [0, 1, 1, 0, 1]
y_pred = [0, 1, 0, 0, 1]
# Calculate metrics
accuracy = accuracy_score(y_true, y_pred)
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
print(f'Accuracy: {accuracy}, Precision: {precision}, Recall: {recall}, F1-Score: {f1}')
Gather and Prepare High-Quality Data
Gathering and preparing high-quality data is the foundation of any machine learning project. Accurate and relevant data is critical for training models that generalize well to new data.
Define Your Problem and Set Clear Goals
Clearly defining your problem and setting specific goals are the first steps in data preparation. This clarity helps in identifying the right data sources and determining the necessary preprocessing steps.
Identify and Collect Relevant Data
Collecting relevant data involves sourcing datasets that are pertinent to your problem. These datasets can come from internal databases, public repositories, APIs, or web scraping. Ensuring data relevance and diversity enhances the model's performance.
Here's an example of collecting data from a public API using Python:

import requests
# API endpoint
url = "https://api.example.com/data"
# Fetch data
response = requests.get(url)
data = response.json()
print(data)
Select the Appropriate Machine Learning Algorithm
Selecting the appropriate machine learning algorithm is crucial for the success of your project. The choice depends on the problem type, data characteristics, and performance requirements.
Understand the Problem
Understanding the problem helps in narrowing down the algorithm choices. For instance, classification problems might require decision trees, SVMs, or neural networks, while regression problems might use linear regression or random forests.
Evaluate Data Characteristics
Evaluating the characteristics of your data, such as size, dimensionality, and the presence of missing values, helps in selecting suitable algorithms. Some algorithms handle high-dimensional data better, while others are robust to missing values.
Here's an example of selecting an algorithm based on data characteristics using Python:

from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier
from sklearn.svm import SVC
# Load data
data = load_iris()
X, y = data.data, data.target
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Choose algorithm
algorithm = RandomForestClassifier() if X.shape[1] > 10 else DecisionTreeClassifier()
algorithm.fit(X_train, y_train)
print(f'Chosen Algorithm: {algorithm.__class__.__name__}')
Properly Train and Validate the Model
Properly training and validating the model ensures its effectiveness and reliability. This process involves splitting the data, selecting an algorithm, training the model, and evaluating its performance.
Collect and Preprocess the Data
Collecting and preprocessing the data is the first step in model training. This includes handling missing values, normalizing features, and encoding categorical variables.
Train and Validate the Model
Training the model involves feeding the preprocessed data into the chosen algorithm and fitting it. Validation is done by evaluating the model's performance on a separate test set to ensure it generalizes well to new data.
Here's an example of training and validating a model using Python and scikit-learn:

from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Sample data
X, y = load_iris(return_X_y=True)
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Validate model
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Regularly Monitor and Update the Model
Regularly monitoring and updating the model ensures it stays up-to-date and continues to perform well as new data becomes available.
Set Up Automated Monitoring
Setting up automated monitoring involves tracking the model's performance over time and detecting any decline. This can be achieved using tools and frameworks that provide real-time analytics.
Continuously Collect and Label New Data
Continuously collecting and labeling new data helps in keeping the model updated. This new data should be used to retrain the model periodically to improve its performance and adapt to changing trends.
Here's an example of setting up automated model monitoring using Python:

import time
import numpy as np
# Simulate monitoring
def monitor_model(model, X_test, y_test):
while True:
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f'Current Accuracy: {accuracy}')
time.sleep(3600) # Monitor every hour
# Load data and model
X, y = load_iris(return_X_y=True)
model = RandomForestClassifier().fit(X, y)
# Start monitoring
monitor_model(model, X, y)
Optimize Hyperparameters for Better Performance
Optimizing hyperparameters can significantly improve the performance of your model. This process involves tuning the model's parameters to find the best combination that maximizes performance.
Importance of Hyperparameter Tuning
Hyperparameter tuning is crucial because it can enhance the model's accuracy, reduce overfitting, and improve generalization. Techniques like grid search, random search, and Bayesian optimization are commonly used for this purpose.
Here's an example of hyperparameter optimization using Python and scikit-learn:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Sample data
X, y = load_iris(return_X_y=True)
# Define parameter grid
param_grid = {'n_estimators': [50, 100, 200], 'max_depth': [None, 10, 20, 30]}
# Perform grid search
grid_search = GridSearchCV(RandomForestClassifier(), param_grid, cv=3)
grid_search.fit(X, y)
# Best parameters
print(f'Best Parameters: {grid_search.best_params_}')
Handle Missing or Noisy Data Effectively
Handling missing or noisy data is important for accurate predictions. This involves identifying and addressing any gaps or errors in the dataset to ensure high-quality inputs.

Techniques for Handling Missing Data
Common techniques for handling missing data include imputation, where missing values are filled with mean, median, or mode, and deletion, where rows with missing values are removed. More advanced techniques involve using algorithms to predict missing values.
Here's an example of handling missing data using Python and pandas:
import pandas as pd
import numpy as np
# Sample data with missing values
data = {'A': [1, 2, np.nan, 4], 'B': [np.nan, 2, 3, 4]}
df = pd.DataFrame(data)
# Fill missing values with mean
df.fillna(df.mean(), inplace=True)
print(df)
Feature Engineering for Better Representation
Feature engineering involves creating new features or modifying existing ones to improve the model's performance. This process can significantly enhance the model's ability to capture the underlying patterns in the data.
Feature Selection
Feature selection involves identifying and using only the most relevant features for training the model. Techniques like recursive feature elimination (RFE) and feature importance from tree-based models are commonly used.
Here's an example of feature selection using Python and scikit-learn:
from sklearn.feature_selection import RFE
from sklearn.ensemble import RandomForestClassifier
# Sample data
X, y = load_iris(return_X_y=True)
# Perform feature selection
selector = RFE(RandomForestClassifier(), n_features_to_select=2)
selector = selector.fit(X, y)
# Selected features
print(f'Selected Features: {selector.support_}')
Regularize the Model to Avoid Overfitting
Regularizing the model helps prevent overfitting and improves generalization. Regularization techniques add a penalty to the model's complexity, discouraging it from fitting the noise in the training data.
L1 Regularization (Lasso)
L1 regularization, or Lasso, adds a penalty equal to the absolute value of the model coefficients. This can result in sparse models, where some coefficients are exactly zero, effectively performing feature selection.
Here's an example of applying L1 regularization using Python and scikit-learn:
from sklearn.linear_model import Lasso
from sklearn.model_selection import train_test_split
# Sample data
X, y = load_iris(return_X_y=True)
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model with L1 regularization
model = Lasso(alpha=0.1)
model.fit(X_train, y_train)
# Coefficients
print(f'Coefficients: {model.coef_}')
L2 Regularization (Ridge)
L2 regularization, or Ridge, adds a penalty equal to the square of the model coefficients. This discourages large coefficients, reducing model complexity and helping prevent overfitting.
Here's an example of applying L2 regularization using Python and scikit-learn:
from sklearn.linear_model import Ridge
from sklearn.model_selection import train_test_split
# Sample data
X, y = load_iris(return_X_y=True)
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model with L2 regularization
model = Ridge(alpha=1.0)
model.fit(X_train, y_train)
# Coefficients
print(f'Coefficients: {model.coef_}')
Interpret and Analyze Model Predictions
Interpreting and analyzing model predictions provides valuable insights into the model's behavior and helps identify areas for improvement.
Understanding Predictions
Understanding how the model makes predictions involves analyzing feature importance, decision paths, and model coefficients. This helps in explaining the model's behavior and making it more transparent.
Tools for Interpretation
Several tools and techniques, such as SHAP (SHapley Additive exPlanations) and LIME (Local Interpretable Model-agnostic Explanations), can be used to interpret complex models and understand their predictions.
Here's an example of interpreting model predictions using Python and SHAP:
import shap
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_iris
# Load data
X, y = load_iris(return_X_y=True)
# Train model
model = RandomForestClassifier()
model.fit(X, y)
# Interpret model predictions
explainer = shap.TreeExplainer(model)
shap_values = explainer.shap_values(X)
shap.summary_plot(shap_values, X, feature_names=load_iris().feature_names)
Tackling machine learning problems successfully requires a thorough understanding of the problem domain, high-quality data preparation, selecting the right algorithm, proper training and validation, regular monitoring and updating, effective hyperparameter optimization, handling missing or noisy data, feature engineering, regularization to avoid overfitting, and interpreting model predictions. By following these essential tips and leveraging the power of machine learning techniques, you can build robust, accurate, and reliable models that deliver valuable insights and predictions.
If you want to read more articles similar to Essential Tips for Tackling Machine Learning Problems Successfully, you can visit the Applications category.
You Must Read