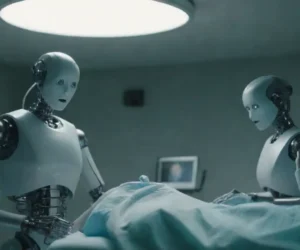
How to Build a Sentiment Analysis Model Using Python Libraries

Introduction
Sentiment analysis is a natural language processing (NLP) technique that aims to determine the emotional tone behind a series of words, often used to understand the sentiments expressed in digital communication. It can help uncover insights from social media, product reviews, and customer feedback by classifying the text as positive, negative, or neutral. As businesses face an overwhelming amount of data every day, sentiment analysis has become an invaluable tool in gauging consumer opinions, assessing brand reputation, and formulating marketing strategies.
In this article, we will explore the process of building a sentiment analysis model from scratch using Python libraries. The goal is not just to implement a straightforward approach but to provide insights into various aspects such as data collection, pre-processing, feature extraction, model selection, training, and evaluation. We will leverage powerful Python libraries like Pandas, NLTK, Scikit-learn, and TensorFlow, ensuring a well-rounded understanding of sentiment analysis concepts and practices.
Understanding the Basics of Sentiment Analysis
To start our journey, it's essential to grasp the foundational concepts of sentiment analysis. Sentiment analysis operates on the premise that language can convey emotion—be it joy, anger, surprise, or sadness. By analyzing text data, we can identify and classify emotional context. In general, sentiment analysis can be divided into three types:
Fine-grained Sentiment Analysis: This approach evaluates the sentiment at a granular level, typically using scales (e.g., 1 to 5 stars) to retrieve highly detailed emotional responses that allow for an in-depth analysis.
Recognizing and Mitigating Sentiment Analysis MisinterpretationsAspect-Based Sentiment Analysis: In this case, the analysis is performed on specific aspects or features of a product or service. For instance, one might evaluate customer opinions on the performance, aesthetics, or value of an electronic gadget.
Emotion Detection: This goes beyond simple positive or negative analysis, aiming to detect specific emotions such as joy, fear, or anger from the text.
Understanding these variants facilitates a more nuanced approach to building our sentiment analysis model. Depending on specific needs, businesses can employ different sentiment analysis models, either generic or tailored.
Collecting Data for Sentiment Analysis
The first step in constructing a sentiment analysis model is data collection. The success of any machine learning project heavily relies on the quality and quantity of data available. There are several sources where you can harvest textual data for sentiment analysis:
Sentiment Analysis APIs: Top Services for Developers to UseSocial Media: Platforms like Twitter, Facebook, and Instagram offer a wealth of user-generated content rich in sentiments. Tools or APIs like Tweepy can be utilized for Twitter to gather tweets. The use of hashtags can help target specific sentiment-related content.
Product Reviews: Websites like Amazon, Yelp, and TripAdvisor provide product and service reviews that are excellent sources for sentiment analysis. Scraping data from these websites using techniques like web scraping can yield diverse datasets.
News Articles: Articles from news sources can be another great resource, as they often convey public sentiment on trending topics. APIs such as the News API can streamline this data collection process.
However, it’s crucial to ensure that you're compliant with the terms of service and data usage policies for each platform. After determining your data source, use libraries such as BeautifulSoup for scraping, or Requests for API calls to collect the necessary data.
The Interplay Between Sentiment Analysis and User Experience DesignPre-processing the Text Data

Once you have gathered your data, the next important step is pre-processing. Text pre-processing is a critical part of any NLP task because the raw textual data often contains noise that can hinder the performance of your model. Common pre-processing techniques include:
Cleaning the Data: This involves removing unnecessary characters like HTML tags, punctuation, or special characters. You can use regular expressions in Python to achieve this.
Tokenization: Tokenization is the splitting of text into smaller units like words or sentences. This is key for further analysis, allowing the model to understand the relationships between different words.
Exploring the Use of Emojis in Sentiment Analysis ModelsNormalization: This includes converting all text to the same case (e.g., lowercasing), removing stop words (commonly used words that carry minimal information), and stemming or lemmatization (reducing words to their base forms). Using libraries like NLTK or spaCy, you can effectively handle these tasks.
```python
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
Sample text
text = "I love Python! It's amazing @2023."
Step 1: Clean the data
cleaned_text = re.sub(r'[^a-zA-Zs]', '', text).lower()
Analyzing Sentiment in Multilingual Text: Challenges and SolutionsStep 2: Tokenization
tokens = cleaned_text.split()
Step 3: Normalization
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
lemmatizer = WordNetLemmatizer()
normalizedtokens = [lemmatizer.lemmatize(word) for word in tokens if word not in stopwords]
```
Through pre-processing, the data will become more manageable for machines to evaluate, thus enhancing the efficiency and accuracy of the sentiment analysis model.
Feature Extraction Techniques
After pre-processing, the next task is feature extraction—the process of transforming the text data into a format that machine learning algorithms can work with. There are several methods to achieve this, including:
Bag of Words (BoW): The Bag of Words model simplifies corpus representation by counting the frequency of each word. While easy to implement, BoW doesn't convey information about word order, leading to a potential loss of context.
Term Frequency-Inverse Document Frequency (TF-IDF): TF-IDF improves upon BoW by adjusting word frequency based on how commonly a word appears across multiple documents. This means that while common words are filtered out, more unique indicators that justify sentiment are emphasized.
Word Embeddings: Techniques such as Word2Vec or GloVe create dense vector representations of words where similar words have similar representations. This technique retains semantic meanings and relationships between words.
Integrating these techniques allows us to generate feature sets that accurately represent the text for subsequent machine learning models. Here’s an example of using TF-IDF for feature extraction:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
Sample data
documents = ["I love Python", "Python is great at data science"]
TF-IDF Implementation
vectorizer = TfidfVectorizer()
tfidfmatrix = vectorizer.fittransform(documents).toarray()
```
By converting text data into numerical features, these methods help prepare the sentiment analysis model for training.
Selecting and Training the Model
Now that we have applied the prior steps to generate a feature set, we can proceed to select a machine learning model suitable for sentiment analysis. Popular algorithms include:
Logistic Regression: A great choice for binary classification tasks, logistic regression is easy to implement and understand.
Support Vector Machines (SVM): Known for their robustness, SVMs can effectively classify complex datasets and are especially powerful for high-dimensional datasets.
Deep Learning Models: More advanced models such as Recurrent Neural Networks (RNNs) and Long Short-Term Memory (LSTM) networks are extensively used for analyzing sequential data like text due to their ability to learn from the contextual relationships among words.
Once you decide on a model, the next step is to train it using the training data. Here’s a simple implementation of training a logistic regression model:
```python
from sklearn.modelselection import traintestsplit
from sklearn.linearmodel import LogisticRegression
from sklearn.metrics import accuracy_score
Sample feature data and labels
X = tfidf_matrix
y = [1, 1] # Example sentiment labels (1 for positive)
Split the dataset
Xtrain, Xtest, ytrain, ytest = traintestsplit(X, y, testsize=0.2, randomstate=42)
Training the Logistic Regression model
model = LogisticRegression()
model.fit(Xtrain, ytrain)
Predictions
ypred = model.predict(Xtest)
Evaluate the model
accuracy = accuracyscore(ytest, y_pred)
print(f'Accuracy: {accuracy * 100:.2f}%')
```
Training results can vary based on the size and quality of the dataset used, so experimenting with different models and tuning their parameters is vital to optimizing performance.
Evaluating Your Sentiment Analysis Model
After training your model, it's essential to evaluate its effectiveness using metrics like:
- Accuracy: The ratio of correctly predicted instances to the total instances.
- Precision: The ratio of true positive observations to the total predicted positives, reflecting how many selected items are relevant.
- Recall: The ratio of true positives to the actual number of relevant cases, providing insights on how well the model can find all the relevant instances.
- F1 Score: The weighted average of precision and recall, giving a balance between the two metrics.
You can leverage libraries like Scikit-learn to simplify this evaluation process:
```python
from sklearn.metrics import classification_report
Output evaluation metrics
print(classificationreport(ytest, y_pred))
```
Understanding these metrics allows you to pinpoint areas for improvement and refine your approach, whether by improving feature extraction techniques, adjusting your model's parameters, or augmenting your dataset.
Conclusion
In this article, we dove deep into the world of sentiment analysis, showcasing the entire process of building a sentiment analysis model using Python libraries. From understanding the basics of sentiment analysis to data collection, pre-processing, feature extraction, model selection, training, and evaluation, each step is crucial for achieving an effective outcome.
By utilizing tools like Pandas, NLTK, Scikit-learn, and TensorFlow, you are armed with the resources to tackle sentiment analysis effectively. As you experiment with different algorithms, datasets, and techniques, remember that the journey does not end with model building. Continuous refinement, evaluation, and adaptation to new data are essential for maintaining a robust sentiment analysis system.
From businesses analyzing customer feedback to marketers gauging public opinion, sentiment analysis remains a powerful ally in the decision-making process. By mastering the techniques outlined in this article, you can harness the power of text data and explore the emotions that drive consumer behavior. Happy coding!
If you want to read more articles similar to How to Build a Sentiment Analysis Model Using Python Libraries, you can visit the Sentiment Analysis category.
You Must Read