
Analyzing Accuracy of Loan Approval Prediction with Machine Learning
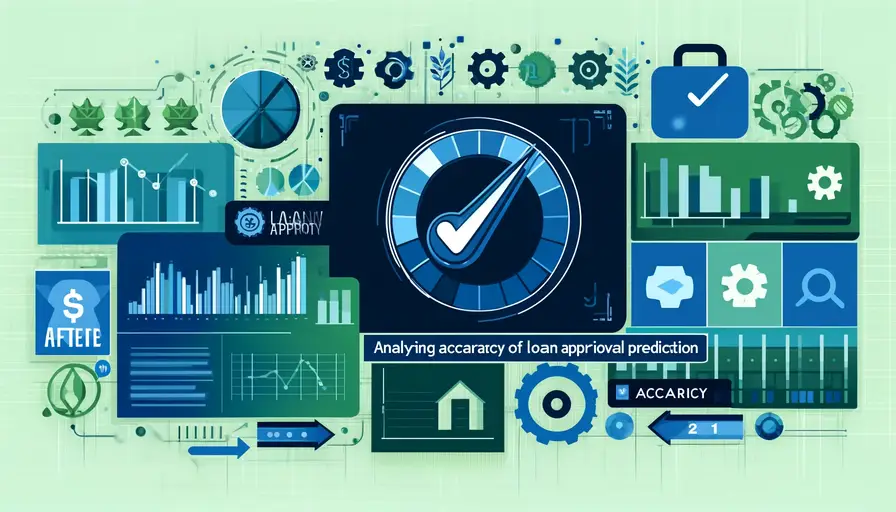
Content
Collect a Large Dataset of Loan Applications
Organize and Preprocess the Dataset
To start, it's essential to gather a substantial dataset of loan applications, including various attributes and their corresponding approval outcomes. This data should be meticulously organized to ensure that it covers a wide range of scenarios and variables affecting loan approvals. Proper organization involves categorizing data points, removing duplicates, and ensuring consistency across the dataset.
Preprocessing the dataset is a crucial step in preparing it for machine learning analysis. This involves handling missing values, normalizing numerical variables, and encoding categorical variables. These steps are vital to convert raw data into a format that machine learning algorithms can effectively process. Ensuring data integrity at this stage helps in building robust models.
Choose and Implement Machine Learning Algorithms
Once the dataset is organized and preprocessed, the next step is to choose appropriate machine learning algorithms. Common choices for loan approval prediction include logistic regression, decision trees, and random forests. Each algorithm has its strengths and can be selected based on the specific requirements and nature of the dataset.
Implementing these algorithms requires a good understanding of their mechanics and the ability to configure them for optimal performance. This might involve setting parameters, defining the structure of decision trees, or specifying the number of trees in a random forest. The goal is to apply these algorithms in a way that maximizes their predictive accuracy.
Step-by-Step Guide: Animated Visualizations for ML RegressionEvaluate and Interpret the Results
After implementing the machine learning algorithms, it's crucial to evaluate their performance. This involves comparing the predicted loan approval outcomes with the actual outcomes in the dataset. Evaluation metrics such as accuracy, precision, recall, and F1 score provide insights into how well the models are performing.
Interpreting the results helps in understanding the strengths and weaknesses of each model. It also offers a clear picture of which algorithm is the most reliable for predicting loan approvals. This step is fundamental in fine-tuning the models and improving their performance for more accurate predictions.
Preprocess the Data
Cleaning the Data
Data cleaning is a vital preprocessing step that involves removing or correcting inaccuracies, inconsistencies, and missing values in the dataset. This process ensures that the data is accurate and complete, which is critical for building reliable machine learning models. Techniques such as imputation, removing duplicates, and handling outliers are commonly used during this stage.
Clean data provides a solid foundation for model training and helps in achieving more accurate predictions. It's important to carefully review the dataset to identify and address any issues that could affect the performance of the machine learning models.
Hedge Fund Strategies: Machine Learning for InvestmentsTransforming Variables
Transforming variables involves converting data into formats that machine learning algorithms can process. This might include scaling numerical variables, encoding categorical variables into numerical formats, and normalizing data. Such transformations ensure that the variables are on a comparable scale and the models can interpret them correctly.
For example, categorical variables such as loan type or applicant's employment status need to be encoded into numerical values. Techniques like one-hot encoding or label encoding are often used to achieve this transformation. Properly transformed variables enhance the model's ability to learn from the data and make accurate predictions.
Feature Engineering
Feature engineering is the process of creating new features from existing data to improve the performance of machine learning models. This can involve generating interaction terms, polynomial features, or aggregating features over time. The goal is to create features that provide additional insights and capture underlying patterns in the data.
Effective feature engineering can significantly boost the predictive power of machine learning models. By creating features that better represent the underlying relationships in the data, models can achieve higher accuracy and more reliable predictions. This step requires creativity and domain knowledge to identify meaningful transformations.
Time Frame for Training and Implementing Machine LearningSplit the Data into Training and Testing Sets
Data Splitting
Splitting the dataset into training and testing sets is crucial for evaluating the performance of machine learning models. The training set is used to train the model, while the testing set is used to evaluate its performance on unseen data. This approach helps in assessing the generalizability of the model.
A common practice is to split the data into 70% for training and 30% for testing, though this ratio can be adjusted based on the dataset size and specific needs. Ensuring a representative split helps in building models that perform well on real-world data.
Ensuring Representative Splits
When splitting the data, it's important to ensure that both the training and testing sets are representative of the overall dataset. This involves maintaining the distribution of classes and other important characteristics in both sets. Stratified sampling can be used to achieve this, especially for datasets with imbalanced classes.
Representative splits help in training models that generalize well to new data. They prevent scenarios where the model performs well on the training data but poorly on the testing data due to a mismatch in data characteristics.
Building an Effective End-to-End Machine Learning PipelineTrain Various Machine Learning Algorithms
Logistic Regression
Logistic Regression is a simple yet effective algorithm for binary classification tasks like loan approval prediction. It models the probability that a given input belongs to a particular class using a logistic function. Logistic regression is easy to implement and interpret, making it a popular choice for initial model building.
In the context of loan approval, logistic regression can help identify the probability of loan approval based on variables X and Y. It provides a clear understanding of how each variable contributes to the prediction, aiding in feature selection and model tuning.
Here’s an example of using logistic regression for loan approval prediction:
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load the dataset (replace 'data' and 'target' with actual dataset variables)
X_train, X_test, y_train, y_test = train_test_split(data, target, test_size=0.3, random_state=42)
# Initialize the Logistic Regression model
model = LogisticRegression(random_state=42, max_iter=1000)
# Train the model
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Logistic Regression Accuracy: {accuracy}')
Decision Trees
Decision Trees are versatile algorithms that model decisions based on the features of the data. They work by recursively splitting the dataset into subsets based on the value of a selected feature. Decision trees are easy to visualize and interpret, making them useful for understanding complex relationships in the data.
The Significance of Machine Learning Applications for BusinessesIn loan approval prediction, decision trees can capture non-linear relationships between variables X and Y and the target variable. They provide insights into the decision-making process, highlighting the most important features influencing loan approvals.
Random Forests
Random Forests are an ensemble learning method that builds multiple decision trees and combines their predictions. This approach helps in reducing overfitting and improving model accuracy. Random forests are robust and can handle large datasets with many features, making them suitable for complex prediction tasks.
By using random forests for loan approval prediction, you can leverage the power of multiple decision trees to achieve more accurate and reliable predictions. The ensemble nature of random forests ensures that the model generalizes well to new data.
Evaluate Model Performance Using Metrics
Accuracy
Accuracy is a fundamental metric used to evaluate the performance of classification models. It is calculated as the ratio of correctly predicted instances to the total number of instances. While accuracy is a useful metric, it may not always provide a complete picture, especially in imbalanced datasets.
Easy Raspberry Pi Machine Learning Projects for BeginnersIn loan approval prediction, high accuracy indicates that the model correctly predicts loan approvals and rejections for most applications. However, it's important to consider other metrics to ensure a comprehensive evaluation of model performance.
Precision
Precision measures the proportion of true positive predictions out of all positive predictions made by the model. It is particularly important in scenarios where false positives are costly. High precision means that when the model predicts a loan approval, it is usually correct.
Precision is crucial in loan approval prediction as it helps in minimizing the risk of approving loans that are likely to default. A model with high precision ensures that approved loans have a higher likelihood of being repaid.
Recall
Recall measures the proportion of true positive predictions out of all actual positive instances. It indicates how well the model identifies positive cases. High recall means that the model successfully identifies most of the loans that should be approved.
In loan approval prediction, high recall ensures that potential loan approvals are not missed. This is important for maximizing the approval rate while maintaining acceptable risk levels.
F1 Score
F1 Score is the harmonic mean of precision and recall. It provides a balanced measure of a model's performance, especially in cases where there is a trade-off between precision and recall. The F1 score is useful for evaluating models on imbalanced datasets.
In loan approval prediction, the F1 score helps in balancing the need to approve as many good loans as possible (recall) while minimizing the approval of bad loans (precision). A high F1 score indicates a well-balanced model.
Fine-Tune the Models
Hyperparameter Tuning
Hyperparameter tuning involves adjusting the parameters of a machine learning model to improve its performance. This process is critical for optimizing models and achieving better accuracy. Techniques such as grid search and random search are commonly used for hyperparameter tuning.
By fine-tuning the hyperparameters of models used for loan approval prediction, you can enhance their predictive power and robustness. This involves experimenting with different parameter values to find the optimal configuration.
Cross-Validation
Cross-validation is a technique used to evaluate the performance of a model on different subsets of the data. It helps in assessing the generalizability of the model and reducing the risk of overfitting. K-fold cross-validation is a popular method where the data is divided into k subsets, and the model is trained and evaluated k times.
In loan approval prediction, cross-validation ensures that the model performs well on unseen data. It provides a more reliable estimate of model performance compared to a single train-test split.
Compare Different Models
Importance of Accuracy
Accuracy is a key metric in comparing different machine learning models for loan approval prediction. It provides a straightforward measure of how well each model performs on the task. However, it's important to consider other metrics as well to get a comprehensive evaluation.
Accuracy is crucial for understanding the overall effectiveness of a model. In loan approval prediction, high accuracy means that the model correctly classifies most loan applications, providing reliable predictions.
Comparing Models
Comparing different models involves evaluating them based on multiple metrics, such as accuracy, precision, recall, and F1 score. This comprehensive evaluation helps in identifying the strengths and weaknesses of each model. It also provides insights into which model is the most suitable for the specific task.
By comparing models, you can select the one that offers the best performance for loan approval prediction. This involves analyzing the trade-offs between different metrics and choosing the model that balances them effectively.
Validate the Selected Model
Generalizability
Validating the selected model involves assessing its performance on the testing set. This step ensures that the model generalizes well to new data and is not overfitted to the training set. A model that performs well on the testing set is likely to provide reliable predictions in real-world scenarios.
Generalizability is critical in loan approval prediction as it ensures that the model can accurately predict outcomes for new loan applications. This validation step provides confidence in the model's ability to perform consistently.
Deployment
Once the model is validated, it can be deployed to predict loan approval for new, unseen loan applications. Deployment involves saving the trained model, setting up a server or cloud-based environment, and exposing the model as an API. This allows the model to be integrated into existing systems and used in real-time decision-making.
Here's an example of how to save and deploy a trained model using Python:
import joblib
# Save the trained model
joblib.dump(model, 'loan_approval_model.pkl')
# Load the saved model
model = joblib.load('loan_approval_model.pkl')
# Example of using the loaded model for prediction
new_data = [...] # Replace with new data
prediction = model.predict(new_data)
print(f'Prediction: {prediction}')
Monitor and Update the Model
Updating the Model
Monitoring and updating the model is essential to ensure its continued accuracy and relevance. This involves regularly checking the model's performance and retraining it with new data as needed. Keeping the model updated helps in maintaining its predictive power and adapting to changes in the data.
In loan approval prediction, updating the model ensures that it remains effective in predicting outcomes as new trends and patterns emerge. This proactive approach helps in maintaining high accuracy and reliability.
Continuous Improvement
Continuous improvement involves regularly assessing the model's performance metrics and making necessary adjustments. This might include fine-tuning hyperparameters, adding new features, or retraining the model with additional data. The goal is to ensure that the model evolves and improves over time.
By continuously improving the model, you can achieve better performance and more accurate predictions. This ongoing process is crucial for maintaining the effectiveness of the model in a dynamic environment like loan approval prediction.
Analyzing the accuracy of loan approval prediction with machine learning involves multiple steps, including data collection, preprocessing, model training, evaluation, and deployment. By using various machine learning algorithms such as logistic regression, decision trees, and random forests, and evaluating them with metrics like accuracy, precision, recall, and F1 score, you can identify the most accurate model for predicting loan approvals. Fine-tuning the models, validating their performance, and continuously monitoring and updating them ensures their effectiveness and reliability. With the right approach and tools, machine learning can significantly enhance the accuracy of loan approval predictions, leading to better decision-making and improved financial outcomes.
If you want to read more articles similar to Analyzing Accuracy of Loan Approval Prediction with Machine Learning, you can visit the Applications category.
You Must Read