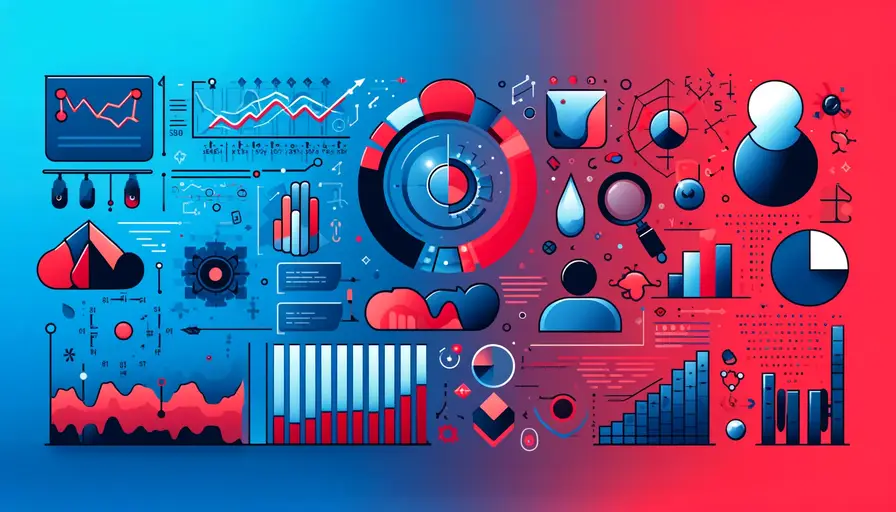
Are Machine Learning Models Statistically Valid?

Machine learning (ML) models have become integral to various fields, from healthcare to finance, and their statistical validity is crucial to ensure reliable and actionable results. This article explores the concept of statistical validity in machine learning models, examining the methods to evaluate it, the challenges faced, and the best practices to ensure robust models.
Evaluating Statistical Validity in Machine Learning Models
Understanding Statistical Validity
Statistical validity refers to the extent to which the results of a model accurately reflect the data and can be generalized to new, unseen data. Ensuring statistical validity involves verifying that the model captures the true underlying patterns in the data rather than noise or artifacts. This is essential for making reliable predictions and informed decisions.
There are various aspects of statistical validity, including internal validity, which measures how well the model fits the training data, and external validity, which assesses the model's generalizability to new data. Additionally, construct validity evaluates whether the model accurately measures the concept it is intended to, while criterion validity compares the model's predictions to an external standard.
Ensuring statistical validity involves a combination of theoretical knowledge and practical techniques. This includes using appropriate validation methods, understanding the assumptions behind the models, and performing rigorous testing.

Cross-Validation Techniques
Cross-validation is a critical technique for assessing the statistical validity of machine learning models. It involves dividing the dataset into multiple folds and training the model on some folds while testing it on others. This process is repeated multiple times, and the results are averaged to provide a robust estimate of model performance.
K-fold cross-validation is one of the most commonly used methods. In K-fold cross-validation, the data is split into K equal-sized folds. The model is trained on K-1 folds and tested on the remaining fold. This process is repeated K times, with each fold serving as the test set once. The final performance metric is the average of the results from all folds.
Here’s an example of performing K-fold cross-validation using Scikit-learn:
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
import numpy as np
# Generating sample data
X = np.random.rand(100, 5)
y = np.random.randint(2, size=100)
# Defining the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
# Performing K-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
print(f'Cross-validation scores: {scores}')
print(f'Mean cross-validation score: {scores.mean()}')
Bootstrapping Methods
Bootstrapping is another powerful technique for evaluating the statistical validity of machine learning models. It involves repeatedly sampling from the dataset with replacement to create multiple bootstrap samples. The model is trained and evaluated on these samples to provide estimates of its performance and variability.

Bootstrapping helps assess the stability and robustness of the model by providing insights into how it performs on different subsets of the data. It is particularly useful when the dataset is small or when traditional cross-validation is not feasible.
Here’s an example of performing bootstrapping using Scikit-learn:
from sklearn.utils import resample
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
import numpy as np
# Generating sample data
X = np.random.rand(100, 5)
y = np.random.randint(2, size=100)
# Defining the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
# Bootstrapping
n_iterations = 1000
scores = np.zeros(n_iterations)
for i in range(n_iterations):
X_resampled, y_resampled = resample(X, y)
model.fit(X_resampled, y_resampled)
y_pred = model.predict(X)
scores[i] = accuracy_score(y, y_pred)
print(f'Bootstrapped accuracy: {np.mean(scores)} ± {np.std(scores)}')
Challenges in Ensuring Statistical Validity
Overfitting and Underfitting
Overfitting and underfitting are common challenges that affect the statistical validity of machine learning models. Overfitting occurs when the model learns the noise in the training data, leading to high accuracy on the training set but poor generalization to new data. Underfitting happens when the model is too simple to capture the underlying patterns, resulting in poor performance on both training and test data.
Detecting and addressing overfitting and underfitting is crucial for ensuring statistical validity. Techniques such as cross-validation, regularization, and pruning can help mitigate these issues. Additionally, choosing the right model complexity and monitoring performance metrics on both training and validation sets are essential.

Here’s an example of addressing overfitting using regularization with Scikit-learn:
from sklearn.linear_model import Ridge
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import numpy as np
# Generating sample data
X = np.random.rand(100, 5)
y = np.random.rand(100)
# Splitting the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Defining and training the model with regularization
model = Ridge(alpha=1.0)
model.fit(X_train, y_train)
# Making predictions
y_pred = model.predict(X_test)
# Evaluating the model
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
Data Quality and Preprocessing
The quality of the data used for training and testing machine learning models significantly impacts their statistical validity. Poor data quality, including missing values, outliers, and noisy data, can lead to biased and unreliable models. Proper data preprocessing, including cleaning, normalization, and feature engineering, is essential to ensure data quality.
Handling missing values involves techniques such as imputation, where missing data is replaced with estimated values, or removing records with missing data. Normalization ensures that features are on a comparable scale, preventing certain features from dominating the model. Feature engineering involves creating new features or transforming existing ones to better capture the underlying patterns in the data.
Here’s an example of data preprocessing using Pandas and Scikit-learn:

import pandas as pd
from sklearn.preprocessing import StandardScaler
from sklearn.impute import SimpleImputer
# Generating sample data
data = {'feature1': [1, 2, np.nan, 4, 5], 'feature2': [10, 20, 30, np.nan, 50], 'target': [0, 1, 0, 1, 0]}
df = pd.DataFrame(data)
# Handling missing values
imputer = SimpleImputer(strategy='mean')
df_imputed = pd.DataFrame(imputer.fit_transform(df), columns=df.columns)
# Normalizing features
scaler = StandardScaler()
df_scaled = pd.DataFrame(scaler.fit_transform(df_imputed.drop('target', axis=1)), columns=df_imputed.drop('target', axis=1).columns)
print(df_scaled)
Model Selection and Hyperparameter Tuning
Choosing the right model and tuning its hyperparameters are critical for ensuring the statistical validity of machine learning models. Different models have varying assumptions and strengths, making it essential to select the model that best fits the data and the problem at hand. Hyperparameter tuning involves finding the optimal settings for a model's parameters to achieve the best performance.
Grid search and random search are common techniques for hyperparameter tuning. Grid search exhaustively evaluates all combinations of hyperparameters, while random search randomly samples from the hyperparameter space. Advanced methods like Bayesian optimization use probabilistic models to guide the search for optimal hyperparameters.
Here’s an example of hyperparameter tuning using grid search with Scikit-learn:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
import numpy as np
# Generating sample data
X = np.random.rand(100, 5)
y = np.random.randint(2, size=100)
# Defining the model and hyperparameter grid
model = RandomForestClassifier(random_state=42)
param_grid = {'n_estimators': [50, 100, 200], 'max_depth': [None, 10, 20]}
# Performing grid search
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
print(f'Best parameters: {grid_search.best_params_}')
print(f'Best cross-validation score: {grid_search.best_score_}')
Best Practices for Ensuring Statistical Validity
Using Proper Validation Techniques
Proper validation techniques are essential for ensuring the statistical validity of machine learning models. Cross-validation, as discussed earlier, is a fundamental technique that provides a robust estimate of model performance. Stratified cross-validation, where the folds are created to preserve the class distribution, is particularly useful for imbalanced datasets.

Holdout validation, where a portion of the data is set aside as a test set, is another common method. Ensuring that the test set is representative of the real-world data is crucial for obtaining an accurate estimate of model performance. Additionally, using a validation set to tune hyperparameters and a separate test set for final evaluation helps prevent data leakage and overfitting.
Ensemble methods, such as bagging and boosting, can also enhance the statistical validity of models by combining the predictions of multiple models to reduce variance and bias. These methods often lead to more robust and reliable models.
Here’s an example of using ensemble methods with Scikit-learn:
from sklearn.ensemble import BaggingClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import numpy as np
# Generating sample data
X = np.random.rand(100, 5)
y = np.random.randint(2, size=100)
# Splitting the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Defining and training the ensemble model
model = BaggingClassifier(base_estimator=DecisionTreeClassifier(), n_estimators=10, random_state=42)
model.fit(X_train, y_train)
# Making predictions
y_pred = model.predict(X_test)
# Evaluating the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Monitoring and Addressing Bias
Bias in machine learning models can arise from various sources, including biased training data, model assumptions, and feature selection. Ensuring statistical validity involves monitoring and addressing bias to prevent discriminatory outcomes and ensure fairness.

Techniques for mitigating bias include re-sampling the data to balance class distributions, using fairness-aware algorithms, and auditing models for biased predictions. Additionally, transparency and accountability in the modeling process help identify and address potential biases.
Fairness metrics, such as demographic parity, equalized odds, and disparate impact, can be used to evaluate the fairness of machine learning models. Implementing these metrics and monitoring them throughout the model development process is crucial for ensuring ethical and unbiased models.
Here’s an example of evaluating fairness using demographic parity with Scikit-learn:
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import numpy as np
import pandas as pd
# Generating sample data
data = {'feature1': np.random.rand(100), 'feature2': np.random.rand(100), 'protected': np.random.randint(2, size=100), 'target': np.random.randint(2, size=100)}
df = pd.DataFrame(data)
# Splitting the data into training and testing sets
X = df[['feature1', 'feature2']]
y = df['target']
protected = df['protected']
X_train, X_test, y_train, y_test, protected_train, protected_test = train_test_split(X, y, protected, test_size=0.2, random_state=42)
# Defining and training the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Making predictions
y_pred = model.predict(X_test)
# Evaluating fairness
accuracy = accuracy_score(y_test, y_pred)
demographic_parity = np.mean(y_pred[protected_test == 1]) - np.mean(y_pred[protected_test == 0])
print(f'Accuracy: {accuracy}')
print(f'Demographic Parity: {demographic_parity}')
Ensuring Reproducibility
Reproducibility is a fundamental aspect of ensuring the statistical validity of machine learning models. It involves documenting the entire modeling process, including data preprocessing, model selection, hyperparameter tuning, and evaluation. Reproducibility ensures that the results can be independently verified and reproduced by other researchers or practitioners.
Version control systems, such as Git, help track changes to the code and data, enabling collaboration and transparency. Using containerization tools like Docker ensures that the model environment, including dependencies and configurations, can be consistently replicated across different systems.
Automating the workflow using scripts and notebooks, such as Jupyter notebooks, helps document and reproduce the entire process. Additionally, sharing the code and data through repositories like GitHub or Kaggle facilitates collaboration and transparency.
Here’s an example of using a Jupyter notebook to document the modeling process:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Generating sample data
data = {'feature1': np.random.rand(100), 'feature2': np.random.rand(100), 'target': np.random.randint(2, size=100)}
df = pd.DataFrame(data)
# Splitting the data into training and testing sets
X = df[['feature1', 'feature2']]
y = df['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Defining and training the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Making predictions
y_pred = model.predict(X_test)
# Evaluating the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
The statistical validity of machine learning models is crucial for ensuring reliable and actionable results. By understanding the concept of statistical validity, employing proper validation techniques, addressing common challenges, and following best practices, practitioners can develop robust and trustworthy models. Leveraging tools and frameworks like Scikit-learn, Pandas, Jupyter, and Git helps streamline the process and ensure that models are statistically valid, reproducible, and ethically sound.
If you want to read more articles similar to Are Machine Learning Models Statistically Valid?, you can visit the Performance category.
You Must Read