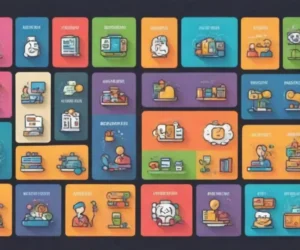
Are Neural Networks a Type of Machine Learning?
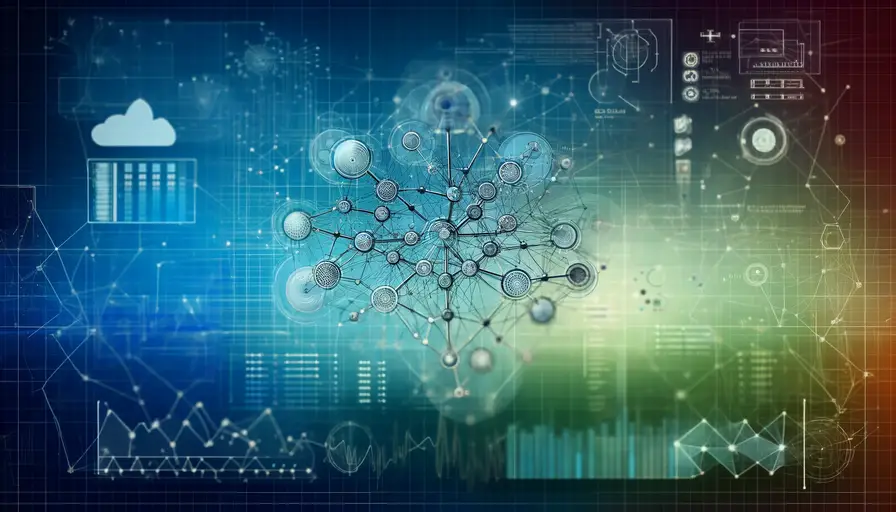
Neural networks have become a cornerstone of modern artificial intelligence (AI) and machine learning (ML), driving advancements in various fields from image recognition to natural language processing. This article explores whether neural networks are indeed a type of machine learning, delving into their principles, how they differ from other ML algorithms, and their applications.
The Relationship Between Neural Networks and Machine Learning
Defining Neural Networks
Neural networks are a subset of machine learning algorithms inspired by the structure and function of the human brain. They consist of interconnected layers of nodes, or neurons, where each neuron processes input data and passes it through an activation function to produce an output. The connections between neurons, known as weights, are adjusted during training to minimize the difference between predicted and actual outcomes.
Neural networks can learn complex patterns and relationships in data, making them particularly effective for tasks involving large and unstructured datasets. They are the foundation of deep learning, a subset of ML that focuses on training neural networks with many layers, known as deep neural networks.
The ability of neural networks to model non-linear relationships and their flexibility in handling various types of data have contributed to their widespread adoption in fields such as computer vision, speech recognition, and autonomous systems.
Pros and Cons of Various Machine Learning Models: A ComparisonComparing Neural Networks to Traditional ML Algorithms
While neural networks are a type of machine learning, they differ significantly from traditional ML algorithms in several ways. Traditional algorithms like linear regression, decision trees, and support vector machines (SVMs) are often based on statistical methods and rely on predefined rules and assumptions about the data.
In contrast, neural networks do not require explicit feature engineering or manual intervention. Instead, they automatically learn features and representations from raw data through multiple layers of abstraction. This makes neural networks particularly powerful for tasks where feature extraction is complex or not well understood.
Traditional ML algorithms typically perform well on structured data with clear patterns, while neural networks excel at handling unstructured data, such as images, audio, and text. However, neural networks require large amounts of data and computational resources for training, which can be a limitation compared to traditional ML methods.
Here’s an example of implementing a simple neural network using TensorFlow:
Exploring the Latest Breakthroughs in Modern Machine Learning Modelsimport tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# Load the iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Build a simple neural network model
model = Sequential([
Dense(64, activation='relu', input_shape=(4,)),
Dense(64, activation='relu'),
Dense(3, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
The Evolution of Neural Networks in Machine Learning
Neural networks have evolved significantly since their inception, driven by advancements in computational power, data availability, and algorithmic innovations. The concept of neural networks dates back to the 1940s with the development of the McCulloch-Pitts neuron, but practical applications were limited due to computational constraints.
The resurgence of neural networks in the 1980s, marked by the development of the backpropagation algorithm, allowed for the training of multi-layer networks. This breakthrough, combined with the advent of powerful GPUs, enabled the rise of deep learning in the 2010s. Deep learning has since revolutionized many fields, demonstrating state-of-the-art performance in tasks such as image classification, language translation, and game playing.
The ongoing evolution of neural networks continues to push the boundaries of what is possible in machine learning, with innovations such as convolutional neural networks (CNNs), recurrent neural networks (RNNs), and transformer models further enhancing their capabilities.
Key Components of Neural Networks
Neurons and Activation Functions
Neurons are the fundamental units of a neural network, responsible for processing input data and generating output. Each neuron receives inputs, applies a weighted sum, and passes the result through an activation function to introduce non-linearity. This non-linearity allows neural networks to model complex relationships in the data.
Is NLP: A Form of Machine Learning or AI?Common activation functions include the sigmoid, tanh, and rectified linear unit (ReLU). The sigmoid function maps inputs to a range between 0 and 1, making it suitable for binary classification tasks. The tanh function, which outputs values between -1 and 1, is often used in hidden layers. ReLU, defined as the maximum of zero and the input, has become the default activation function for hidden layers due to its simplicity and effectiveness in mitigating the vanishing gradient problem.
Here’s an example of implementing a neuron with a ReLU activation function using NumPy:
import numpy as np
# Input and weights
inputs = np.array([1.0, 2.0, 3.0])
weights = np.array([0.2, 0.8, -0.5])
bias = 2.0
# Weighted sum
weighted_sum = np.dot(inputs, weights) + bias
# ReLU activation function
output = np.maximum(0, weighted_sum)
print(output)
Network Architectures and Layers
Neural networks consist of multiple layers of neurons, including input, hidden, and output layers. The input layer receives raw data, the hidden layers perform feature extraction and transformation, and the output layer produces the final prediction. The architecture of a neural network, including the number of layers and neurons, significantly influences its performance.
Feedforward neural networks (FNNs), also known as multi-layer perceptrons (MLPs), are the simplest type of neural network where information flows in one direction from input to output. Convolutional neural networks (CNNs) are specialized for processing grid-like data, such as images, and utilize convolutional layers to detect spatial features. Recurrent neural networks (RNNs) are designed for sequential data and use recurrent connections to capture temporal dependencies.
Decoding Machine Learning Models: Deterministic or Probabilistic?The choice of network architecture depends on the specific task and data characteristics. For instance, CNNs are well-suited for image recognition, while RNNs excel in natural language processing. Experimenting with different architectures and hyperparameters is essential for optimizing model performance.
Training and Optimization
Training a neural network involves optimizing its weights to minimize a loss function, which measures the discrepancy between predicted and actual outputs. The backpropagation algorithm, combined with gradient descent optimization, is the standard method for training neural networks. During backpropagation, gradients of the loss function with respect to the weights are computed and used to update the weights iteratively.
Various optimization techniques have been developed to improve the efficiency and convergence of training. Stochastic Gradient Descent (SGD) updates the weights using a small batch of data, providing faster convergence and reduced memory usage. Advanced optimizers, such as Adam and RMSprop, adapt the learning rate for each parameter, further enhancing training performance.
Regularization techniques, such as dropout and weight decay, help prevent overfitting by adding noise to the training process or penalizing large weights. These techniques improve the generalization of the model, ensuring it performs well on unseen data.
Understanding the Concept of Epochs in Machine LearningHere’s an example of training a simple neural network using the Keras library in TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Load and preprocess the iris dataset
iris = load_iris()
X = iris.data
y = iris.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Build a neural network model
model = Sequential([
Dense(64, activation='relu', input_shape=(4,)),
Dense(64, activation='relu'),
Dense(3, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
Applications of Neural Networks
Image Recognition
Neural networks, particularly convolutional neural networks (CNNs), have revolutionized image recognition. CNNs are designed to automatically and adaptively learn spatial hierarchies of features from images through backpropagation. This capability has led to significant improvements in tasks such as object detection, image classification, and facial recognition.
For instance, deep learning models like AlexNet, VGGNet, and ResNet have achieved remarkable accuracy on image recognition benchmarks like ImageNet. These models use multiple layers of convolutions, pooling, and fully connected layers to learn intricate patterns in images, enabling them to classify objects with high precision.
The success of CNNs in image recognition has numerous practical applications, including autonomous driving, medical imaging, and security surveillance. Companies like Google, Facebook, and Tesla have integrated CNNs into their products to enhance image processing capabilities.
Unit Testing for Machine Learning ModelsNatural Language Processing
Natural language processing (NLP) is another domain where neural networks, especially recurrent neural networks (RNNs) and transformer models, have made significant strides. RNNs are designed to handle sequential data, making them suitable for tasks like language modeling, machine translation, and sentiment analysis.
Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) are variants of RNNs that address the issue of long-term dependencies in sequences. These models have been widely used in applications such as speech recognition, text generation, and chatbots.
Transformer models, like BERT and GPT-3, have further advanced NLP by leveraging self-attention mechanisms to capture long-range dependencies and context. These models have achieved state-of-the-art performance in various NLP tasks, including question answering, text summarization, and language translation.
Companies like OpenAI and Microsoft have developed advanced NLP systems based on neural networks, enabling applications that enhance communication and information processing.
Autonomous Systems
Autonomous systems, such as self-driving cars and drones, rely heavily on neural networks to perceive and navigate their environments. These systems use a combination of CNNs, RNNs, and other deep learning models to process sensor data, recognize objects, and make real-time decisions.
In autonomous driving, neural networks are used to interpret data from cameras, lidar, radar, and other sensors to understand the vehicle's surroundings. This involves detecting and classifying objects, predicting the movements of pedestrians and other vehicles, and planning safe paths.
Neural networks also play a crucial role in reinforcement learning, where autonomous agents learn to perform tasks by interacting with their environment. Reinforcement learning models, such as Deep Q-Networks (DQNs) and Proximal Policy Optimization (PPO), have been used to train robots, drones, and game-playing AI.
Companies like Tesla, Waymo, and Amazon are at the forefront of developing autonomous systems that leverage neural networks to achieve high levels of autonomy and safety.
Advances in Neural Network Research
Convolutional Neural Networks (CNNs)
Convolutional Neural Networks (CNNs) are specialized neural networks designed for processing structured grid data, such as images. CNNs use convolutional layers to automatically learn spatial hierarchies of features from input data, making them highly effective for image-related tasks.
CNNs consist of multiple types of layers, including convolutional layers, pooling layers, and fully connected layers. Convolutional layers apply filters to the input data to detect features like edges, textures, and shapes. Pooling layers reduce the spatial dimensions of the data, making the model more computationally efficient and robust to variations.
Innovations in CNN architectures, such as ResNet with its residual connections and Inception with its multi-scale processing, have significantly improved performance and efficiency. These advancements have made CNNs the go-to choice for image recognition, object detection, and other computer vision tasks.
Here’s an example of implementing a CNN for image classification using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
from tensorflow.keras.datasets import mnist
from tensorflow.keras.utils import to_categorical
# Load and preprocess the MNIST dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train = X_train.reshape((X_train.shape[0], 28, 28, 1)).astype('float32') / 255
X_test = X_test.reshape((X_test.shape[0], 28, 28, 1)).astype('float32') / 255
y_train = to_categorical(y_train)
y_test = to_categorical(y_test)
# Build a CNN model
model = Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(64, kernel_size=(3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
Recurrent Neural Networks (RNNs) and Transformers
Recurrent Neural Networks (RNNs) are designed for sequential data, where the order of the data points is important. RNNs maintain an internal state that captures information from previous time steps, making them suitable for tasks such as time series forecasting, language modeling, and speech recognition.
Despite their advantages, traditional RNNs suffer from the vanishing gradient problem, which makes it difficult to learn long-term dependencies. Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) networks address this issue by incorporating gating mechanisms that control the flow of information.
Transformer models, introduced by Vaswani et al., have revolutionized NLP by leveraging self-attention mechanisms to capture long-range dependencies without the need for recurrent connections. Transformers have led to state-of-the-art models like BERT, GPT-3, and T5, which excel in various NLP tasks.
Transformers have also been adapted for other domains, such as computer vision and reinforcement learning, demonstrating their versatility and effectiveness.
Here’s an example of implementing a simple RNN using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import SimpleRNN, Dense
from sklearn.preprocessing import MinMaxScaler
import numpy as np
# Generating sample time series data
data = np.sin(np.arange(0, 100, 0.1))
scaler = MinMaxScaler()
data = scaler.fit_transform(data.reshape(-1, 1))
# Preparing the data for RNN
X = []
y = []
for i in range(len(data) - 10):
X.append(data[i:i+10])
y.append(data[i+10])
X = np.array(X)
y = np.array(y)
# Build an RNN model
model = Sequential([
SimpleRNN(50, activation='relu', input_shape=(10, 1)),
Dense(1)
])
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Train the model
model.fit(X, y, epochs=10, batch_size=32)
# Make predictions
predictions = model.predict(X)
print(predictions)
Advancements in Transfer Learning and Pre-trained Models
Transfer learning is a technique where a model trained on a large dataset is fine-tuned on a smaller, task-specific dataset. This approach leverages the knowledge learned from the larger dataset, improving performance and reducing training time for the target task.
Pre-trained models, such as VGG, ResNet, BERT, and GPT-3, have been widely used in transfer learning. These models provide a robust starting point for various applications, from image classification to text generation, allowing researchers and practitioners to achieve high accuracy with limited data and computational resources.
Transfer learning has democratized access to advanced ML models, enabling smaller organizations and individuals to leverage state-of-the-art models without extensive resources. It has also accelerated the development and deployment of ML solutions across diverse domains.
Here’s an example of using a pre-trained BERT model for text classification with Hugging Face Transformers:
from transformers import BertTokenizer, TFBertForSequenceClassification
import tensorflow as tf
# Load pre-trained BERT model and tokenizer
model = TFBertForSequenceClassification.from_pretrained('bert-base-uncased')
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
# Sample text data
texts = ["This is a positive review.", "This is a negative review."]
labels = [1, 0]
# Tokenize the text data
inputs = tokenizer(texts, return_tensors='tf', padding=True, truncation=True, max_length=128)
inputs['labels'] = tf.convert_to_tensor(labels)
# Train the model
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=5e-5), loss=model.compute_loss, metrics=['accuracy'])
model.fit(inputs, labels, epochs=2, batch_size=1)
# Make predictions
predictions = model.predict(inputs)
print(predictions)
Neural networks are indeed a type of machine learning, distinguished by their ability to model complex relationships and handle unstructured data. Their evolution and advancements have transformed various fields, from image recognition and natural language processing to autonomous systems and beyond. By leveraging the power of neural networks and staying abreast of the latest innovations, researchers and practitioners can continue to push the boundaries of what is possible in machine learning. Using tools like TensorFlow, Keras, and Hugging Face Transformers.
If you want to read more articles similar to Are Neural Networks a Type of Machine Learning?, you can visit the Artificial Intelligence category.
You Must Read