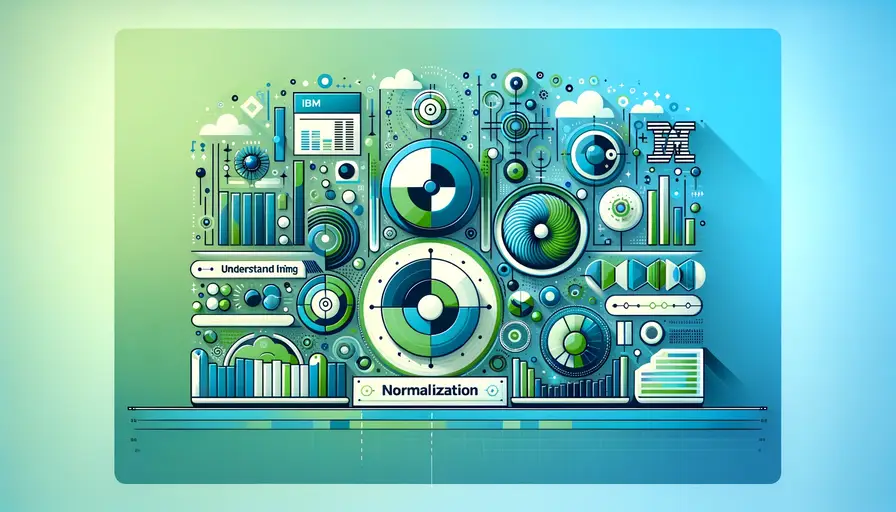
Beginner's Guide to Machine Learning in R

Machine learning is an exciting field that combines statistics, computer science, and domain knowledge to create predictive models. For beginners, R is a fantastic programming language to start with, given its rich ecosystem of packages and tools designed specifically for data analysis and machine learning. This guide will take you through the steps of getting started with machine learning in R, from understanding the basics to deploying your models.
- Understand the Basics of Machine Learning
- Learn the R Programming Language
- Install Necessary Packages in R
- Load and Preprocess Data
- Choose a Machine Learning Algorithm
- Train the Model Using the Chosen Algorithm
- Evaluate the Model's Performance
- Fine-tune the Model for Better Results
- Use the Trained Model to Make Predictions
- Deploy the Machine Learning Model in Real-world Applications
Understand the Basics of Machine Learning
Machine learning involves training algorithms to recognize patterns in data and make predictions or decisions based on new data. The key concepts include supervised learning (where the model learns from labeled data), unsupervised learning (where the model identifies patterns without labeled data), and reinforcement learning (where the model learns by interacting with its environment).
Supervised learning tasks include regression (predicting continuous values) and classification (predicting categorical values). Unsupervised learning includes clustering (grouping similar data points) and association (finding rules that describe large portions of data). Understanding these fundamentals will help you choose the right algorithm and approach for your specific problem.
Learn the R Programming Language
R is a powerful language for statistical computing and graphics. It is widely used for data analysis, visualization, and machine learning. Learning R will enable you to leverage its extensive libraries and tools to build machine learning models efficiently.

To get started with R, you should familiarize yourself with its syntax, data structures (such as vectors, lists, and data frames), and basic functions for data manipulation and visualization. There are many online resources, tutorials, and books available to help you learn R.
Install Necessary Packages in R
Installing necessary packages is crucial for performing machine learning tasks in R. R has a vast repository of packages that simplify the implementation of various machine learning algorithms and techniques.
Commonly Used Packages for Machine Learning in R
Commonly used packages for machine learning in R include caret
for training and evaluating models, randomForest
for building random forest models, e1071
for support vector machines, and nnet
for neural networks. You can install these packages using the install.packages()
function in R.
# Install commonly used packages
install.packages(c("caret", "randomForest", "e1071", "nnet"))
Load and Preprocess Data
Loading and preprocessing data are essential steps before training a machine learning model. Proper data preparation ensures that your model performs well and generalizes to new data.

Loading the Data
Loading the data involves reading data from various sources such as CSV files, databases, or online repositories. In R, you can use functions like read.csv()
to load data from CSV files.
# Load data from a CSV file
data <- read.csv("data.csv")
Preprocessing the Data
Preprocessing the data includes handling missing values, normalizing numerical features, and encoding categorical variables. This step ensures that the data is in a suitable format for modeling.
Feature Engineering
Feature engineering involves creating new features from existing data to improve model performance. This can include deriving new variables, combining features, or creating interaction terms.
Data Splitting
Data splitting is the process of dividing the dataset into training and testing sets. The training set is used to train the model, while the testing set is used to evaluate its performance. This helps prevent overfitting and ensures that the model generalizes well to new data.

# Split the data into training and testing sets
library(caret)
set.seed(123)
trainIndex <- createDataPartition(data$Target, p = .8, list = FALSE, times = 1)
trainData <- data[trainIndex,]
testData <- data[-trainIndex,]
Choose a Machine Learning Algorithm
Choosing a machine learning algorithm depends on the problem you're trying to solve and the nature of your data. Each algorithm has its strengths and weaknesses, and some are better suited for specific types of problems.
Linear Regression
Linear regression is used for predicting continuous values. It assumes a linear relationship between the input features and the target variable.
Logistic Regression
Logistic regression is used for binary classification problems. It models the probability of the target variable belonging to a particular class.
Decision Trees
Decision trees are used for both classification and regression tasks. They split the data into subsets based on feature values, creating a tree-like model of decisions.

Random Forest
Random forest is an ensemble method that builds multiple decision trees and combines their predictions. It improves accuracy and reduces overfitting.
Support Vector Machines
Support vector machines (SVM) are used for classification tasks. They find the hyperplane that best separates the classes in the feature space.
Neural Networks
Neural networks are powerful models for both regression and classification tasks. They consist of interconnected layers of neurons that learn complex patterns in the data.
Train the Model Using the Chosen Algorithm
Training the model involves using the training data to teach the algorithm to recognize patterns and make predictions. This step requires selecting the appropriate algorithm and configuring its parameters.

# Train a random forest model
library(randomForest)
model <- randomForest(Target ~ ., data = trainData, ntree = 100)
Evaluate the Model's Performance
Evaluating the model's performance is crucial to ensure that it generalizes well to new data. This step involves using various metrics to assess how well the model performs on the testing set.
Metrics for Model Evaluation
Metrics for model evaluation include accuracy, precision, recall, F1 score, and area under the ROC curve (AUC-ROC) for classification tasks, and mean squared error (MSE) and R-squared for regression tasks.
Cross-validation
Cross-validation is a technique used to assess the model's performance by splitting the data into multiple subsets and training and testing the model on different combinations of these subsets.
Visualizing Model Performance
Visualizing model performance can help you understand how well the model is performing and identify areas for improvement. Common visualizations include confusion matrices, ROC curves, and residual plots.

# Evaluate model performance
predictions <- predict(model, testData)
confusionMatrix(predictions, testData$Target)
Fine-tune the Model for Better Results
Fine-tuning the model involves adjusting its parameters to improve performance. This step can include hyperparameter tuning, feature selection, and model ensembling.
Hyperparameter tuning can be done using techniques like grid search or random search, which test different combinations of parameters to find the best configuration.
Use the Trained Model to Make Predictions
Using the trained model involves applying it to new data to make predictions. This step can be done in batch mode or in real-time, depending on the application.
# Make predictions on new data
newData <- read.csv("new_data.csv")
newPredictions <- predict(model, newData)
Deploy the Machine Learning Model in Real-world Applications
Deploying the machine learning model involves integrating it into a real-world application where it can provide value. This step can include building web applications, creating APIs, or performing batch processing.
Web Applications
Web applications allow users to interact with the model through a user-friendly interface. R Shiny is a popular framework for building interactive web applications in R.
APIs
APIs enable other systems to interact with the model programmatically. This approach is useful for integrating machine learning models into existing software systems.
Batch Processing
Batch processing involves applying the model to large datasets in bulk. This approach is suitable for scenarios where real-time predictions are not required.
Integration with Existing Systems
Integration with existing systems ensures that the model can be seamlessly incorporated into the current workflow. This can include automating predictions, generating reports, and providing insights to decision-makers.
# Deploy the model as a web application using R Shiny
library(shiny)
ui <- fluidPage(
titlePanel("Machine Learning Model Deployment"),
sidebarLayout(
sidebarPanel(
fileInput("file", "Choose CSV File", accept = ".csv"),
actionButton("predict", "Predict")
),
mainPanel(
tableOutput("predictions")
)
)
)
server <- function(input, output) {
predictions <- eventReactive(input$predict, {
req(input$file)
newData <- read.csv(input$file$datapath)
predict(model, newData)
})
output$predictions <- renderTable({
predictions()
})
}
shinyApp(ui, server)
Getting started with machine learning in R involves understanding the basics, learning the R programming language, and using the right tools and packages. By following the steps outlined in this guide, you can load and preprocess data, choose and train machine learning algorithms, evaluate and fine-tune models, and deploy them in real-world applications. With practice and experience, you can leverage R to build powerful machine learning solutions that provide valuable insights and drive decision-making.
If you want to read more articles similar to Beginner's Guide to Machine Learning in R, you can visit the Artificial Intelligence category.
You Must Read