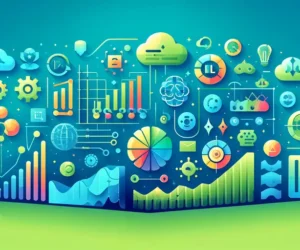
Best Deep Learning Software for NVIDIA GPUs: A Complete Guide
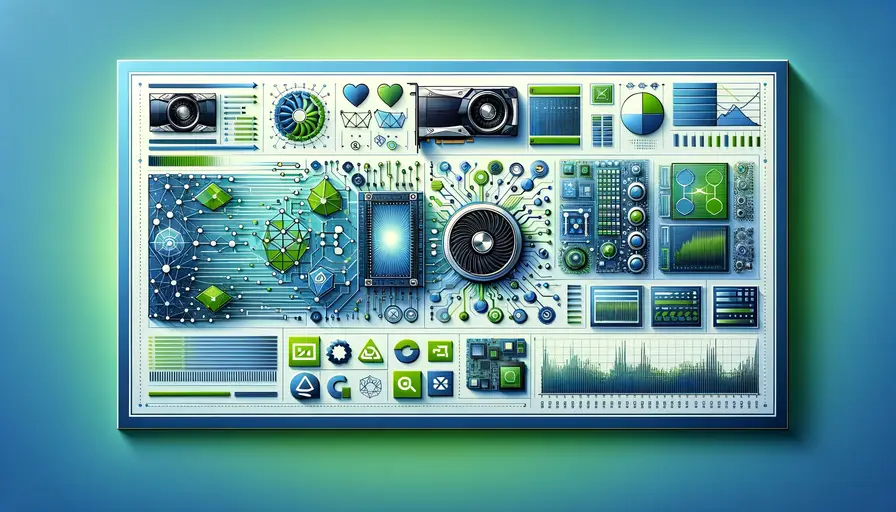
TensorFlow: Popular Deep Learning Software
Why TensorFlow?
TensorFlow is one of the most popular deep learning frameworks, known for its flexibility and scalability. Developed by Google, it is designed to run efficiently on NVIDIA GPUs, leveraging their power to accelerate deep learning tasks. TensorFlow supports a wide range of machine learning applications, from research to production.
Its extensive ecosystem includes tools for building, training, and deploying models, making it a comprehensive solution for deep learning projects. TensorFlow's support for multiple programming languages and its robust community make it a go-to choice for many developers.
TensorFlow on NVIDIA GPUs
TensorFlow optimizes its operations using CUDA, NVIDIA's parallel computing platform and application programming interface (API). This optimization allows TensorFlow to perform computations at high speed, taking full advantage of the parallel processing capabilities of NVIDIA GPUs. This makes TensorFlow ideal for training complex neural networks and handling large datasets.
Here's an example of a simple neural network in TensorFlow:
Quantum Machine Learning Tools for Advanced Data Analysisimport tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Load sample data
(X_train, y_train), (X_test, y_test) = tf.keras.datasets.mnist.load_data()
X_train, X_test = X_train / 255.0, X_test / 255.0
# Build the model
model = Sequential([
Dense(128, activation='relu', input_shape=(784,)),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=5)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
This code demonstrates how to build and train a neural network using TensorFlow.
PyTorch: Widely Used Software
Why PyTorch?
PyTorch is another leading deep learning framework known for its dynamic computation graph and ease of use. Developed by Facebook's AI Research lab, PyTorch is particularly favored in the research community for its flexibility and speed. Its dynamic nature allows developers to change the architecture of the network on the fly, making it easier to experiment and debug.
PyTorch's extensive library and community support, along with its seamless integration with Python, make it a powerful tool for developing deep learning models. Its support for CUDA ensures that PyTorch can efficiently utilize NVIDIA GPUs for faster computation.
PyTorch on NVIDIA GPUs
PyTorch efficiently leverages NVIDIA GPUs to accelerate deep learning computations. By using CUDA, PyTorch can perform operations on the GPU, significantly speeding up the training and inference processes. This capability makes PyTorch suitable for large-scale deep learning tasks and real-time applications.
Is CML the Ultimate Solution for Machine Learning Pipelines?Here’s an example of a simple neural network in PyTorch:
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
# Load sample data
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
trainset = datasets.MNIST('.', download=True, train=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=64, shuffle=True)
# Define the model
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(28 * 28, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = x.view(-1, 28 * 28)
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Instantiate and train the model
model = Net()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
for epoch in range(5):
for data, target in trainloader:
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
This code demonstrates how to build and train a neural network using PyTorch.
NVIDIA Deep Learning SDK
Key Features
NVIDIA's Deep Learning SDK provides a comprehensive set of tools and libraries designed to accelerate deep learning applications on NVIDIA GPUs. The SDK includes cuDNN, NCCL, and TensorRT, which offer optimized performance for training and inference. These tools ensure that deep learning frameworks like TensorFlow and PyTorch run efficiently on NVIDIA hardware.
cuDNN, for instance, provides highly optimized implementations of standard routines such as forward and backward convolution, pooling, normalization, and activation layers. TensorRT is a high-performance deep learning inference library that optimizes and deploys trained models, ensuring they run efficiently on NVIDIA GPUs.
Comparing On-Premise vs Cloud for ML Model DeploymentBenefits for Developers
Developers benefit from the NVIDIA Deep Learning SDK by gaining access to tools that enhance the performance and efficiency of their models. These tools streamline the development process, from model training to deployment, ensuring that applications can handle the demands of real-world data and computational loads.
Here’s an example of using TensorRT for model inference:
import tensorrt as trt
# Create a TensorRT logger and builder
logger = trt.Logger(trt.Logger.WARNING)
builder = trt.Builder(logger)
network = builder.create_network()
# Define the network (this part is usually exported from a trained model)
input_tensor = network.add_input(name="input", dtype=trt.float32, shape=(1, 28, 28))
# Add layers...
# Build the engine
builder.max_workspace_size = 1 << 20
engine = builder.build_cuda_engine(network)
# Perform inference
context = engine.create_execution_context()
# Allocate buffers and perform inference...
This code shows how to use TensorRT for efficient model inference on NVIDIA GPUs.
Caffe: Optimized for GPUs
Features of Caffe
Caffe is a deep learning framework that emphasizes speed and modularity. Developed by the Berkeley Vision and Learning Center (BVLC), Caffe is particularly optimized for image processing tasks and is widely used in computer vision applications. Its architecture encourages clean and modular design, making it easy to switch between CPU and GPU computations.
Machine Learning Studio vs. Service: Which is Better?Caffe's speed and efficiency make it an excellent choice for developing and deploying deep learning models that require real-time processing. Its extensive model zoo offers pre-trained models that can be readily used for various applications, further simplifying the development process.
Caffe on NVIDIA GPUs
Caffe leverages NVIDIA GPUs to accelerate deep learning computations, providing significant performance improvements over CPU-only implementations. The framework's integration with cuDNN ensures that it can utilize the optimized GPU kernels provided by NVIDIA, enhancing its computational efficiency.
Here’s an example of defining and training a simple neural network using Caffe:
import caffe
from caffe import layers as L, params as P
# Define the network
def simple_net():
n = caffe.NetSpec()
n.data, n.label = L.Data(source="mnist_train_lmdb", backend=P.Data.LMDB, batch_size=64, ntop=2)
n.fc1 = L.InnerProduct(n.data, num_output=128, weight_filler=dict(type='xavier'))
n.relu1 = L.ReLU(n.fc1, in_place=True)
n.fc2 = L.InnerProduct(n.relu1, num_output=10, weight_filler=dict(type='xavier'))
n.loss = L.SoftmaxWithLoss(n.fc2, n.label)
return n.to_proto()
# Write the network to a file
with open('simple_net.prototxt', 'w') as f:
f.write(str(simple_net()))
# Load and train the network
solver = caffe.SGDSolver('solver.prototxt')
solver.solve()
This code demonstrates how to define and train a simple neural network using Caffe.
Find the Ideal Platform for Your Machine Learning ProjectsCUDA Toolkit
Key Features
The NVIDIA CUDA Toolkit is a powerful platform for GPU-accelerated computing. It provides developers with a comprehensive development environment, including tools for debugging, optimization, and performance monitoring. The toolkit includes libraries such as cuBLAS, cuFFT, and cuSPARSE, which offer optimized routines for common mathematical operations.
CUDA enables developers to write parallel code that runs on NVIDIA GPUs, significantly accelerating computational tasks. This capability is essential for deep learning applications that require large-scale data processing and complex model training.
CUDA for Deep Learning
CUDA plays a crucial role in deep learning by providing the necessary infrastructure for running intensive computations on NVIDIA GPUs. By utilizing CUDA, developers can achieve significant speedups in both training and inference, making it possible to tackle more complex problems and larger datasets.
Here’s an example of a simple CUDA program:
Flask: Best Practices for Deploying ML Models#include <cuda_runtime.h>
#include <iostream>
__global__ void add(int *a, int *b, int *c) {
int index = threadIdx.x;
c[index] = a[index] + b[index];
}
int main() {
int a[5] = {1, 2, 3, 4, 5};
int b[5] = {10, 20, 30, 40, 50};
int c[5] = {0};
int *d_a, *d_b, *d_c;
cudaMalloc((void**)&d_a, 5 * sizeof(int));
cudaMalloc((void**)&d_b, 5 * sizeof(int));
cudaMalloc((void**)&d_c, 5 * sizeof(int));
cudaMemcpy(d_a, a, 5 * sizeof(int), cudaMemcpyHostToDevice);
cudaMemcpy(d_b, b, 5 * sizeof(int), cudaMemcpyHostTo
Device);
add<<<1, 5>>>(d_a, d_b, d_c);
cudaMemcpy(c, d_c, 5 * sizeof(int), cudaMemcpyDeviceToHost);
for (int i = 0; i < 5; i++) {
std::cout << c[i] << " ";
}
std::cout << std::endl;
cudaFree(d_a);
cudaFree(d_b);
cudaFree(d_c);
return 0;
}
This code shows a basic CUDA program for adding two arrays.
Microsoft Cognitive Toolkit (CNTK)
Features of CNTK
Microsoft Cognitive Toolkit (CNTK) is a deep learning framework developed by Microsoft. It is designed to be highly efficient and scalable, supporting both CPU and GPU computations. CNTK is particularly known for its ability to handle large-scale datasets and complex models, making it suitable for enterprise-level applications.
CNTK provides a flexible and extensible architecture, allowing developers to build custom models and algorithms. Its support for multiple languages, including Python and C++, ensures that developers can choose the best tools for their needs.
CNTK on NVIDIA GPUs
CNTK leverages NVIDIA GPUs to accelerate deep learning computations. By integrating with CUDA and cuDNN, CNTK ensures that models run efficiently on NVIDIA hardware. This capability is crucial for training deep learning models on large datasets and achieving high performance.
Here’s an example of a simple neural network using CNTK:
import cntk as C
# Create sample data
X = C.input_variable((2,), np.float32)
y = C.input_variable((1,), np.float32)
data = np.array([[1, 2], [2, 3], [3, 4], [4, 5]], dtype=np.float32)
labels = np.array([[1], [2], [3], [4]], dtype=np.float32)
# Define the model
model = C.layers.Dense(1)(X)
# Define the loss function and trainer
loss = C.losses.mean_squared_error(model, y)
learner = C.learners.sgd(model.parameters, lr=0.01)
trainer = C.Trainer(model, (loss, None), [learner])
# Train the model
for epoch in range(100):
trainer.train_minibatch({X: data, y: labels})
This code demonstrates how to build and train a simple neural network using CNTK.
MXNet
Features of MXNet
MXNet is a flexible and efficient deep learning framework that supports multiple programming languages, including Python, Scala, and Julia. Developed by the Apache Software Foundation, MXNet is designed for both efficiency and productivity, offering a hybrid programming model that combines symbolic and imperative programming.
MXNet's ability to scale across multiple GPUs and machines makes it suitable for large-scale deep learning applications. Its extensive library of pre-trained models and tools for deploying models on various platforms enhance its versatility and ease of use.
MXNet on NVIDIA GPUs
MXNet leverages NVIDIA GPUs to accelerate deep learning computations, ensuring high performance for both training and inference. The framework's integration with CUDA and cuDNN provides optimized GPU support, enabling developers to build and deploy efficient deep learning models.
Here’s an example of a simple neural network using MXNet:
import mxnet as mx
from mxnet import gluon, nd, autograd
# Load sample data
mnist = mx.test_utils.get_mnist()
train_data = gluon.data.DataLoader(gluon.data.ArrayDataset(mnist['train_data'], mnist['train_label']), batch_size=64, shuffle=True)
# Define the model
net = gluon.nn.Sequential()
net.add(gluon.nn.Dense(128, activation='relu'))
net.add(gluon.nn.Dense(10))
net.initialize(mx.init.Xavier())
# Define the trainer
trainer = gluon.Trainer(net.collect_params(), 'adam', {'learning_rate': 0.001})
loss_fn = gluon.loss.SoftmaxCrossEntropyLoss()
# Train the model
for epoch in range(5):
for data, label in train_data:
with autograd.record():
output = net(data)
loss = loss_fn(output, label)
loss.backward()
trainer.step(batch_size=64)
This code demonstrates how to build and train a simple neural network using MXNet.
Torch
Features of Torch
Torch is a scientific computing framework with wide support for machine learning algorithms. Known for its flexibility and speed, Torch is particularly popular in the research community for developing and experimenting with deep learning models. Its core library provides a variety of functions for tensor operations, which are fundamental for building neural networks.
Torch's simplicity and ease of use make it a preferred choice for rapid prototyping and research. Its Lua-based syntax is straightforward, allowing developers to focus on designing and testing models without getting bogged down by complex code structures.
Torch on NVIDIA GPUs
Torch efficiently utilizes NVIDIA GPUs through CUDA integration, enabling fast computations and efficient training of deep learning models. This capability is essential for handling large datasets and complex neural network architectures.
Here’s an example of a simple neural network using Torch:
require 'nn'
require 'cunn'
-- Load sample data
local mnist = require 'mnist'
local trainset = mnist.traindataset()
local inputs = torch.Tensor(trainset.size, 784)
local targets = torch.Tensor(trainset.size, 10)
for i=1, trainset.size do
inputs[i] = trainset.data[i]:view(784)
targets[i] = torch.zeros(10)
targets[i][trainset.label[i] + 1] = 1
end
-- Define the model
local model = nn.Sequential()
model:add(nn.Linear(784, 128))
model:add(nn.ReLU())
model:add(nn.Linear(128, 10))
model:add(nn.LogSoftMax())
-- Move the model to GPU
model:cuda()
-- Define the loss function
local criterion = nn.ClassNLLCriterion():cuda()
-- Train the model
for epoch=1, 5 do
for i=1, trainset.size do
local input = inputs[i]:cuda()
local target = targets[i]:cuda()
local output = model:forward(input)
local loss = criterion:forward(output, target)
model:zeroGradParameters()
local gradOutput = criterion:backward(output, target)
model:backward(input, gradOutput)
model:updateParameters(0.01)
end
end
This code demonstrates how to build and train a simple neural network using Torch.
Keras
Features of Keras
Keras is a high-level deep learning framework that provides a user-friendly interface for building and training neural networks. It is designed to be easy to use, allowing developers to quickly prototype models and experiment with different architectures. Keras supports multiple backend engines, including TensorFlow, Theano, and CNTK, providing flexibility in how models are built and deployed.
Keras simplifies the process of developing deep learning models with its intuitive API, enabling developers to focus on the creative aspects of model design. Its extensive documentation and strong community support make it an excellent choice for both beginners and experienced practitioners.
Keras on NVIDIA GPUs
Keras leverages NVIDIA GPUs through its backend engines, such as TensorFlow, to accelerate deep learning computations. This integration ensures that models built with Keras can take full advantage of the computational power of NVIDIA GPUs, resulting in faster training times and improved performance.
Here’s an example of a simple neural network using Keras:
from keras.models import Sequential
from keras.layers import Dense
from keras.datasets import mnist
from keras.utils import to_categorical
# Load sample data
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train, X_test = X_train.reshape(60000, 784) / 255.0, X_test.reshape(10000, 784) / 255.0
y_train, y_test = to_categorical(y_train), to_categorical(y_test)
# Build the model
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(784,)))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=5, batch_size=64, validation_data=(X_test, y_test))
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
This code demonstrates how to build and train a simple neural network using Keras.
Choosing the right deep learning software for NVIDIA GPUs depends on the specific requirements and preferences of the developer. TensorFlow, PyTorch, Caffe, CNTK, MXNet, Torch, and Keras each offer unique features and advantages that cater to different aspects of deep learning. By leveraging these powerful tools, developers can efficiently build, train, and deploy deep learning models to tackle a wide range of applications.
If you want to read more articles similar to Best Deep Learning Software for NVIDIA GPUs: A Complete Guide, you can visit the Tools category.
You Must Read