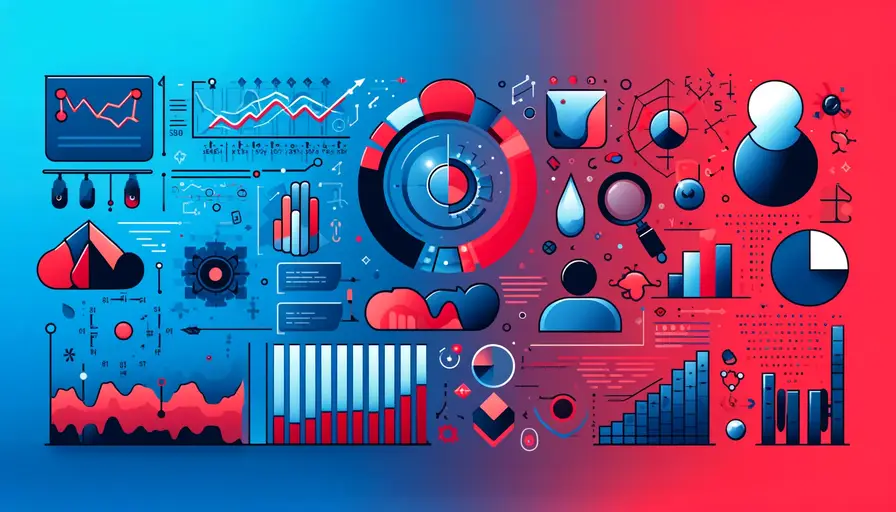
Best Machine Learning Models Algorithm for Classification

- Evaluating Accuracy of ML Models
- Feature Selection for Improved Performance
- Cross-Validation for Model Robustness
- Hyperparameter Tuning for Optimization
- Comparing Precision and Recall
- Analyzing Computational Complexity
- Considering Model Interpretability
- Assessing Scalability
- Matching Algorithms to Specific Requirements
Evaluating Accuracy of ML Models
Evaluating the accuracy of various machine learning (ML) models is essential to determine the best algorithm for classification tasks. Different models have their strengths and weaknesses, and understanding their performance can help in making an informed decision.
Logistic Regression
Logistic Regression is a popular method for binary classification problems. It models the probability of a binary outcome based on one or more predictor variables. Logistic regression is easy to implement and interpret, making it a go-to choice for many practical applications.
Its simplicity, however, can be a limitation. Logistic regression assumes a linear relationship between the input variables and the log-odds of the outcome, which might not always be the case. Despite this, it performs well on linearly separable data and provides probabilistic interpretations of the classification.
Here’s an example of implementing logistic regression using Python:

from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Assuming X and y are predefined features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LogisticRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
print(f'Accuracy: {accuracy}')
Decision Trees
Decision Trees are another commonly used classification method. They work by splitting the data into subsets based on the value of input features, creating a tree-like model of decisions. Decision trees are intuitive and easy to visualize, which aids in understanding the model's decision-making process.
However, decision trees are prone to overfitting, especially when the tree becomes very deep. This overfitting can lead to poor performance on new, unseen data. Pruning techniques and limiting the depth of the tree can help mitigate this issue.
Random Forest
Random Forest is an ensemble learning method that combines multiple decision trees to improve classification accuracy. By averaging the results of many trees, random forests reduce the risk of overfitting and increase robustness.
Random forests are particularly effective when dealing with large datasets with numerous features. They handle missing values well and provide feature importance metrics, helping in understanding which features are most influential in the prediction.

Feature Selection for Improved Performance
Feature selection is a critical step in improving the performance of classification models. It involves selecting the most relevant features for the model, reducing dimensionality, and improving accuracy.
Importance of Feature Selection
Feature selection is important because it helps in eliminating irrelevant or redundant features, which can negatively impact the model's performance. By focusing on the most significant features, models can achieve better accuracy, reduced overfitting, and faster training times.
Methods for Feature Selection
Several methods are used for feature selection, including filter methods (like correlation coefficient scores), wrapper methods (such as recursive feature elimination), and embedded methods (like Lasso regression). These methods help in identifying the best subset of features for the classification task.
Here’s an example of using Recursive Feature Elimination (RFE) for feature selection:

from sklearn.feature_selection import RFE
from sklearn.linear_model import LogisticRegression
model = LogisticRegression()
rfe = RFE(model, 10) # Select top 10 features
fit = rfe.fit(X, y)
print(f'Selected Features: {fit.support_}')
Cross-Validation for Model Robustness
Cross-validation techniques are crucial for assessing the robustness of ML models. They help in evaluating how the model will perform on an independent dataset, providing a more reliable estimate of its accuracy.
Advantages of Cross-Validation
The main advantages of cross-validation include its ability to use the entire dataset for both training and validation, reducing bias and variance. It also provides insights into the model's performance across different subsets of the data, ensuring that the model generalizes well to unseen data.
Steps in Cross-Validation
Steps in performing cross-validation typically involve splitting the data into k subsets (folds), training the model on k-1 folds, and validating it on the remaining fold. This process is repeated k times, with each fold serving as the validation set once. The results are then averaged to obtain a final performance estimate.
Here’s an example of k-fold cross-validation using Python:

from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
model = RandomForestClassifier()
scores = cross_val_score(model, X, y, cv=5) # 5-fold cross-validation
print(f'Cross-Validation Scores: {scores}')
print(f'Average Score: {scores.mean()}')
Hyperparameter Tuning for Optimization
Hyperparameter tuning is essential for optimizing the performance of classification algorithms. Proper tuning can significantly enhance model accuracy and robustness.
Why Tune Hyperparameters
Tuning hyperparameters is crucial because default settings might not yield the best performance for a specific dataset. Hyperparameters control various aspects of the learning process, such as learning rate, tree depth, and the number of estimators, and adjusting them can lead to better model performance.
Methods for Tuning
Common methods for tuning hyperparameters include grid search, random search, and more advanced techniques like Bayesian optimization. These methods systematically explore the hyperparameter space to find the best combination that maximizes model performance.
Here’s an example of Grid Search for hyperparameter tuning using Python:

from sklearn.model_selection import GridSearchCV
from sklearn.svm import SVC
model = SVC()
param_grid = {'C': [0.1, 1, 10], 'gamma': [1, 0.1, 0.01], 'kernel': ['rbf', 'linear']}
grid = GridSearchCV(model, param_grid, refit=True, verbose=2)
grid.fit(X_train, y_train)
print(f'Best Parameters: {grid.best_params_}')
Comparing Precision and Recall
Comparing the precision and recall of different ML models helps identify the most suitable algorithm for classification, especially in imbalanced datasets where accuracy alone might be misleading.
Importance of Precision and Recall
Precision and recall are critical metrics for evaluating classification models. Precision measures the proportion of true positive predictions among all positive predictions, while recall measures the proportion of true positive predictions among all actual positives. Both metrics provide insights into the model's ability to identify positive instances correctly.
Comparing Models
Comparing precision and recall across different models involves calculating these metrics for each model and analyzing their trade-offs. A model with high precision but low recall might be suitable for tasks where false positives are costly, while a model with high recall but lower precision might be better for tasks where missing positives is more detrimental.
Here’s an example of calculating precision and recall using Python:

from sklearn.metrics import precision_score, recall_score
# Assuming y_test and predictions are predefined
precision = precision_score(y_test, predictions)
recall = recall_score(y_test, predictions)
print(f'Precision: {precision}')
print(f'Recall: {recall}')
Analyzing Computational Complexity
Analyzing the computational complexity of each algorithm is crucial for selecting the most efficient model, especially when dealing with large datasets.
Logistic Regression
Logistic Regression has a linear computational complexity with respect to the number of features and training examples. It is generally fast to train and predict, making it suitable for large datasets with numerous features.
Decision Trees
Decision Trees have a computational complexity that depends on the depth of the tree and the number of features. Training can be relatively fast, but very deep trees can become computationally expensive and prone to overfitting.
Random Forest
Random Forest is more complex than individual decision trees due to the ensemble nature of the method. Training involves building multiple trees, which increases computational time but generally results in more accurate and robust models.
Considering Model Interpretability
Considering the interpretability of ML models is important for choosing the best algorithm for classification, as it affects how easily the model's predictions can be understood and trusted.
Decision Trees
Decision Trees are highly interpretable. The tree structure allows for a clear visualization of decision paths, making it easy to understand how predictions are made. This transparency is beneficial for applications where understanding the model's decision process is critical.
Logistic Regression
Logistic Regression is also interpretable, as it provides coefficients that represent the relationship between each feature and the outcome. These coefficients can be easily understood and communicated, making logistic regression a good choice for explainability.
Naive Bayes
Naive Bayes models are simple and interpretable. They provide probabilistic interpretations of predictions, which can be valuable for understanding how different features contribute to the classification.
Assessing Scalability
Assessing the scalability of each algorithm determines its suitability for handling large datasets, which is essential for applications with vast amounts of data.
Random Forest
Random Forest can handle large datasets effectively due to its ensemble nature, which allows parallel processing. This scalability makes it a good choice for applications with extensive data.
Support Vector Machines
Support Vector Machines (SVM) can become computationally intensive as the size of the dataset grows. However, they perform well with smaller, high-dimensional datasets and can be efficient with proper kernel selection and optimization techniques.
Gradient Boosting
Gradient Boosting methods, such as XGBoost, are scalable and can handle large datasets efficiently. They use boosting techniques to improve performance iteratively, making them suitable for high-dimensional data and large-scale applications.
Matching Algorithms to Specific Requirements
Matching algorithms to the specific requirements of the classification problem is essential for identifying the most appropriate ML algorithm.
Logistic Regression
Logistic Regression is suitable for binary classification problems with linearly separable data. Its simplicity, interpretability, and fast computation make it a good choice for many practical applications.
Decision Trees
Decision Trees are ideal for problems where interpretability is crucial. They handle both categorical and numerical data and can model complex decision boundaries, making them versatile for various applications.
Random Forest
Random Forest is a robust choice for handling high-dimensional data and large datasets. Its ensemble nature provides high accuracy and resilience to overfitting, making it suitable for a wide range of classification problems.
Support Vector Machines
Support Vector Machines (SVM) are powerful for high-dimensional datasets where the decision boundary is complex. With proper kernel selection, SVMs can achieve high accuracy and are suitable for tasks requiring precise classification.
By evaluating accuracy, performing feature selection, using cross-validation, tuning hyperparameters, comparing precision and recall, analyzing computational complexity, considering interpretability, assessing scalability, and matching algorithms to specific requirements, you can effectively identify the best ML algorithm for classification tasks.
If you want to read more articles similar to Best Machine Learning Models Algorithm for Classification, you can visit the Performance category.
You Must Read