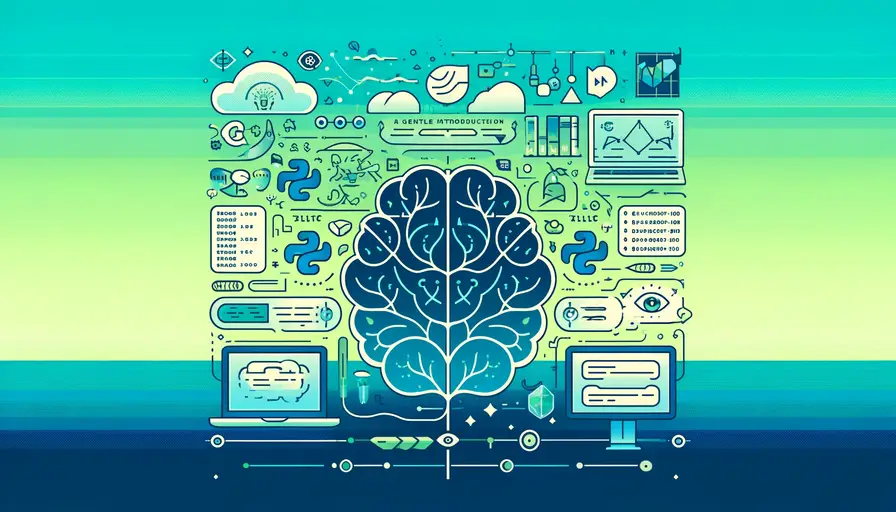
Bootstrapping: Training Deep Neural Networks on Noisy Labels

Training deep neural networks on noisy labels is a challenging task that requires careful consideration of techniques to mitigate the negative impact of noise on model performance. This guide explores various strategies, including data augmentation, label smoothing, semi-supervised learning, active learning, regularization techniques, ensemble methods, robust loss functions, model distillation, learning rate scheduling, and model-agnostic methods. By implementing these techniques, practitioners can enhance the robustness and accuracy of deep neural networks even when faced with noisy labels.
Data Augmentation Techniques
Image Augmentation
Data augmentation is a powerful technique that involves generating additional training samples by applying various transformations to the existing data. Image augmentation includes operations like rotation, flipping, scaling, cropping, and color adjustments. These transformations help the model generalize better by exposing it to a wider variety of scenarios, thereby reducing the impact of noisy labels.
# Example: Image Augmentation with Keras
from keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest'
)
# Apply augmentation to an image
image = load_img('path/to/image.jpg')
x = img_to_array(image)
x = x.reshape((1,) + x.shape)
i = 0
for batch in datagen.flow(x, batch_size=1):
plt.figure(i)
imgplot = plt.imshow(array_to_img(batch[0]))
i += 1
if i % 4 == 0:
break
plt.show()
Text Augmentation
For text data, augmentation techniques include synonym replacement, random insertion, random swap, and random deletion. These techniques help create variations of the text data, making the model more robust to variations and noise in the labels.
# Example: Text Augmentation with NLP Augment Library
from nlpaug.augmenter.word import SynonymAug
aug = SynonymAug(aug_src='wordnet')
text = 'The quick brown fox jumps over the lazy dog'
augmented_text = aug.augment(text)
print(augmented_text)
Audio Augmentation
In the case of audio data, augmentation can involve pitch shifting, time stretching, adding noise, and applying filters. These augmentations help the model to better handle variability and noise in audio signals, improving its robustness to noisy labels.

# Example: Audio Augmentation with pydub
from pydub import AudioSegment
from pydub.playback import play
audio = AudioSegment.from_file('path/to/audio.wav')
pitch_shifted_audio = audio.speedup(playback_speed=1.5)
pitch_shifted_audio.export('augmented_audio.wav', format='wav')
play(pitch_shifted_audio)
Label Smoothing to Make the Mode
Steps to Implement Label Smoothing
Label smoothing is a technique that helps prevent the model from becoming overconfident in its predictions. By slightly adjusting the true labels, the model becomes less sensitive to noise in the labels and can generalize better.
# Example: Implementing Label Smoothing in TensorFlow
import tensorflow as tf
def smooth_labels(labels, factor=0.1):
labels *= (1 - factor)
labels += (factor / labels.shape[1])
return labels
labels = tf.constant([[1, 0, 0], [0, 1, 0], [0, 0, 1]], dtype=tf.float32)
smoothed_labels = smooth_labels(labels)
print(smoothed_labels)
Label smoothing involves modifying the target labels by distributing a small portion of the target value across all classes. This adjustment reduces the model's confidence in any single class, making it more robust to incorrect labels.
Label smoothing is particularly effective in classification tasks where the presence of noisy labels can significantly degrade model performance. By preventing overfitting to noisy labels, label smoothing improves the model's ability to generalize to unseen data.
Semi-Supervised Learning Methods
The Benefits of Bootstrapping
Semi-supervised learning leverages both labeled and unlabeled data to improve model performance. Bootstrapping is a common semi-supervised learning technique where the model is trained iteratively using its own predictions as pseudo-labels for the unlabeled data.

Bootstrapping helps the model learn from the available unlabeled data, reducing the reliance on noisy labeled data. This approach can significantly improve the model's performance by providing additional training examples that enhance its generalization capabilities.
Semi-supervised learning is particularly useful when labeled data is scarce and noisy, but a large amount of unlabeled data is available. By effectively utilizing the unlabeled data, the model can achieve better performance and robustness.
# Example: Bootstrapping with Pseudo-Labels in PyTorch
import torch
import torch.nn as nn
import torch.optim as optim
# Define model, loss function, and optimizer
model = nn.Sequential(nn.Linear(10, 5), nn.ReLU(), nn.Linear(5, 2))
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Training loop with bootstrapping
for epoch in range(10):
for inputs, labels in train_loader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# Generate pseudo-labels for unlabeled data
with torch.no_grad():
pseudo_labels = torch.argmax(model(unlabeled_inputs), dim=1)
# Train on pseudo-labeled data
optimizer.zero_grad()
outputs = model(unlabeled_inputs)
loss = criterion(outputs, pseudo_labels)
loss.backward()
optimizer.step()
Active Learning Strategies
What is Active Learning?
Active learning is a technique where the model selectively queries the most informative samples for labeling. By focusing on the samples that are most uncertain or ambiguous, the model can improve its performance with fewer labeled examples, reducing the impact of noisy labels.
Active learning involves iteratively training the model and selecting new samples to label based on the model's uncertainty. This process helps the model learn more effectively from the available data, improving its robustness to noise.

Active learning can be particularly beneficial in scenarios where labeling is expensive or time-consuming. By carefully selecting the samples to label, the model can achieve better performance with fewer labeled examples.
Applying Active Learning to Reduce the Impact of Noisy Labels
Active learning strategies can help mitigate the impact of noisy labels by focusing on the most informative samples. Techniques such as uncertainty sampling, query-by-committee, and expected model change are commonly used in active learning.
By iteratively selecting and labeling the most informative samples, the model can learn more effectively from the available data. This approach helps reduce the impact of noisy labels and improves the model's generalization capabilities.
Active learning is particularly useful in scenarios where the labeling budget is limited. By carefully selecting the samples to label, the model can achieve better performance with fewer labeled examples, reducing the overall labeling cost.

# Example: Implementing Active Learning with Uncertainty Sampling in Scikit-Learn
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Generate synthetic data
X, y = make_classification(n_samples=1000, n_features=20, n_classes=2, random_state=42)
X_train, X_unlabeled, y_train, _ = train_test_split(X, y, test_size=0.5, random_state=42)
# Initial training
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Active learning loop
for _ in range(10):
# Predict probabilities on unlabeled data
probs = model.predict_proba(X_unlabeled)
# Select samples with highest uncertainty
uncertainty = 1 - np.max(probs, axis=1)
query_indices = np.argsort(uncertainty)[-10:]
# Label selected samples and add to training set
X_train = np.vstack((X_train, X_unlabeled[query_indices]))
y_train = np.hstack((y_train, y_unlabeled[query_indices]))
# Remove selected samples from unlabeled set
X_unlabeled = np.delete(X_unlabeled, query_indices, axis=0)
y_unlabeled = np.delete(y_unlabeled, query_indices, axis=0)
# Retrain model
model.fit(X_train, y_train)
# Evaluate model
accuracy = accuracy_score(y_test, model.predict(X_test))
print(f"Accuracy: {accuracy}")
Regularization Techniques Such as Dropout or Weight
Dropout
Dropout is a regularization technique that randomly drops units from the neural network during training. This prevents the network from becoming too reliant on any single unit and encourages it to learn more robust features.
By randomly dropping units, dropout forces the network to learn multiple redundant representations of the data. This helps prevent overfitting to noisy labels and improves the network's generalization capabilities.
Dropout is particularly effective in large neural networks, where the risk of overfitting is high. By regularizing the network, dropout helps the model generalize better to new data.
# Example: Implementing Dropout in TensorFlow
import tensorflow as tf
from tensorflow.keras.layers import Dense, Dropout
from tensorflow.keras.models import Sequential
# Define the model
model = Sequential([
Dense(128, activation='relu', input_shape=(784,)),
Dropout(0.5),
Dense(64, activation='relu'),
Dropout(0.5),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, validation_data=(X_val, y_val))
Weight Decay
Weight decay, also known as L2 regularization, adds a penalty term to the loss function based on the magnitude of the model's weights. This discourages the model from assigning too much importance to any single feature, preventing overfitting.

Weight decay helps the model learn more generalizable features by penalizing large weights. This regularization technique is particularly useful in scenarios where the training data contains noise or outliers.
By preventing the model from overfitting to noisy labels, weight decay improves the model's ability to generalize to new data. This leads to better performance and robustness in real-world applications.
# Example: Implementing Weight Decay in PyTorch
import torch
import torch.nn as nn
import torch.optim as optim
# Define the model
model = nn.Sequential(
nn.Linear(784, 128),
nn.ReLU(),
nn.Linear(128, 64),
nn.ReLU(),
nn.Linear(64, 10)
)
# Define the loss function and optimizer with weight decay
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001, weight_decay=0.01)
# Train the model
for epoch in range(10):
for inputs, labels in train_loader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Ensemble Methods to Combine Multiple Models
Benefits of Ensemble Methods
Ensemble methods combine the predictions of multiple models to improve overall performance. By leveraging the strengths of different models, ensemble methods can reduce the impact of noisy labels and enhance the robustness of the final prediction.
Ensemble methods are particularly effective in scenarios where individual models may be susceptible to noise in the data. By averaging the predictions of multiple models, the impact of noisy labels is minimized, leading to more accurate and reliable results.

Ensemble methods can be used with various types of models, including decision trees, neural networks, and support vector machines. This flexibility makes ensemble methods a powerful tool for improving model performance in the presence of noisy labels.
Implementing Ensemble Methods
There are several ensemble techniques, including bagging, boosting, and stacking. Bagging involves training multiple models on different subsets of the data and averaging their predictions. Boosting sequentially trains models, each focusing on the errors of the previous model. Stacking combines the predictions of multiple models using a meta-learner.
Ensemble methods can significantly improve the robustness and accuracy of machine learning models. By combining the strengths of different models, ensemble methods provide a powerful solution for handling noisy labels and improving overall performance.
# Example: Implementing Bagging with Random Forest in Scikit-Learn
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Define the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
# Train the model
model.fit(X_train, y_train)
# Evaluate the model
predictions = model.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
print(f"Accuracy: {accuracy}")
Using Robust Loss Functions
Robust loss functions, such as the bootstrapped cross-entropy loss, are less sensitive to noisy labels. These loss functions help the model focus on the correct labels, reducing the impact of noise and improving overall performance.
By using robust loss functions, the model becomes more resilient to noise in the labels. This resilience leads to better generalization and improved performance in real-world applications.
Implementing robust loss functions is a crucial step in training deep neural networks on noisy labels. By mitigating the impact of noisy labels, robust loss functions enhance the model's ability to learn meaningful patterns from the data.
# Example: Implementing Bootstrapped Cross-Entropy Loss in PyTorch
import torch
import torch.nn as nn
class BootstrappedCrossEntropyLoss(nn.Module):
def __init__(self, alpha=0.95):
super(BootstrappedCrossEntropyLoss, self).__init__()
self.alpha = alpha
self.ce_loss = nn.CrossEntropyLoss()
def forward(self, inputs, targets):
ce_loss = self.ce_loss(inputs, targets)
with torch.no_grad():
soft_targets = torch.softmax(inputs, dim=1)
bs_loss = self.alpha * ce_loss + (1 - self.alpha) * (-soft_targets * torch.log(soft_targets)).sum(dim=1).mean()
return bs_loss
# Define the model and loss function
model = nn.Sequential(nn.Linear(784, 128), nn.ReLU(), nn.Linear(128, 10))
criterion = BootstrappedCrossEntropyLoss(alpha=0.95)
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# Train the model
for epoch in range(10):
for inputs, labels in train_loader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Learning Rate Scheduling
Learning rate scheduling involves adaptively adjusting the learning rate during training. By decreasing the learning rate over time, the model can converge to a better solution despite the presence of noisy labels.
Learning rate scheduling helps the model avoid overshooting the optimal solution, leading to more stable and accurate training. This technique is particularly useful in scenarios where the training data contains noise or outliers.
Implementing learning rate scheduling can significantly improve the model's performance and robustness. By adaptively adjusting the learning rate, the model can better navigate the noisy training data and achieve higher accuracy.
# Example: Implementing Learning Rate Scheduling in TensorFlow
import tensorflow as tf
from tensorflow.keras.callbacks import LearningRateScheduler
def scheduler(epoch, lr):
if epoch < 10:
return lr
else:
return lr * tf.math.exp(-0.1)
callback = LearningRateScheduler(scheduler)
# Define the model
model = tf.keras.Sequential([
tf.keras.layers.Dense(128, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model with learning rate scheduling
model.fit(X_train, y_train, epochs=20, validation_data=(X_val, y_val), callbacks=[callback])
Model-Agnostic Methods
Co-Training
Co-training is a semi-supervised learning technique where two models are trained on different views of the data. Each model provides pseudo-labels for the unlabeled data, which are then used to train the other model. This iterative process helps improve the model's predictions and reduce the impact of noisy labels.
Co-training leverages the strengths of both models, allowing them to learn from each other's predictions. This approach helps mitigate the impact of noisy labels and enhances the model's generalization capabilities.
Co-training is particularly effective in scenarios where the available labeled data is noisy or limited. By leveraging the unlabeled data, co-training helps the model achieve better performance and robustness.
# Example: Implementing Co-Training with Two Classifiers in Scikit-Learn
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier, GradientBoostingClassifier
# Split the data into two views
X1, X2, y1, y2 = train_test_split(X, y, test_size=0.5, random_state=42)
# Define the models
model1 = RandomForestClassifier(n_estimators=100, random_state=42)
model2 = GradientBoostingClassifier(n_estimators=100, random_state=42)
# Initial training on labeled data
model1.fit(X1, y1)
model2.fit(X2, y2)
# Co-training loop
for _ in range(10):
# Generate pseudo-labels
pseudo_labels1 = model1.predict(X2)
pseudo_labels2 = model2.predict(X1)
# Train on pseudo-labeled data
model1.fit(X2, pseudo_labels2)
model2.fit(X1, pseudo_labels1)
# Evaluate the models
accuracy1 = model1.score(X_test, y_test)
accuracy2 = model2.score(X_test, y_test)
print(f"Model 1 Accuracy: {accuracy1}, Model 2 Accuracy: {accuracy2}")
Self-Training
Self-training is another semi-supervised learning technique where a single model is used to generate pseudo-labels for the unlabeled data. The model is then retrained using both the labeled and pseudo-labeled data. This iterative process helps improve the model's predictions and reduce the impact of noisy labels.
Self-training leverages the model's own predictions to enhance its performance. By iteratively refining the pseudo-labels, self-training helps the model achieve better generalization and robustness.
Self-training is particularly useful in scenarios where the labeled data is limited or noisy. By effectively utilizing the unlabeled data, self-training helps the model achieve better performance and robustness.
# Example: Implementing Self-Training in Scikit-Learn
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Split the data into labeled and unlabeled sets
X_labeled, X_unlabeled, y_labeled, _ = train_test_split(X, y, test_size=0.5, random_state=42)
# Define the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
# Initial training on labeled data
model.fit(X_labeled, y_labeled)
# Self-training loop
for _ in range(10):
# Generate pseudo-labels for unlabeled data
pseudo_labels = model.predict(X_unlabeled)
# Retrain the model using both labeled and pseudo-labeled data
model.fit(np.vstack((X_labeled, X_unlabeled)), np.hstack((y_labeled, pseudo_labels)))
# Evaluate the model
accuracy = accuracy_score(y_test, model.predict(X_test))
print(f"Accuracy: {accuracy}")
By implementing these techniques, practitioners can effectively train deep neural networks on noisy labels, improving their robustness and accuracy. Each method addresses different aspects of the challenge, providing a comprehensive approach to handling noisy labels in machine learning models.
If you want to read more articles similar to Bootstrapping: Training Deep Neural Networks on Noisy Labels, you can visit the Algorithms category.
You Must Read