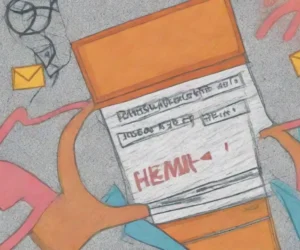
Comparing Clustering vs Classification: When to Use Each
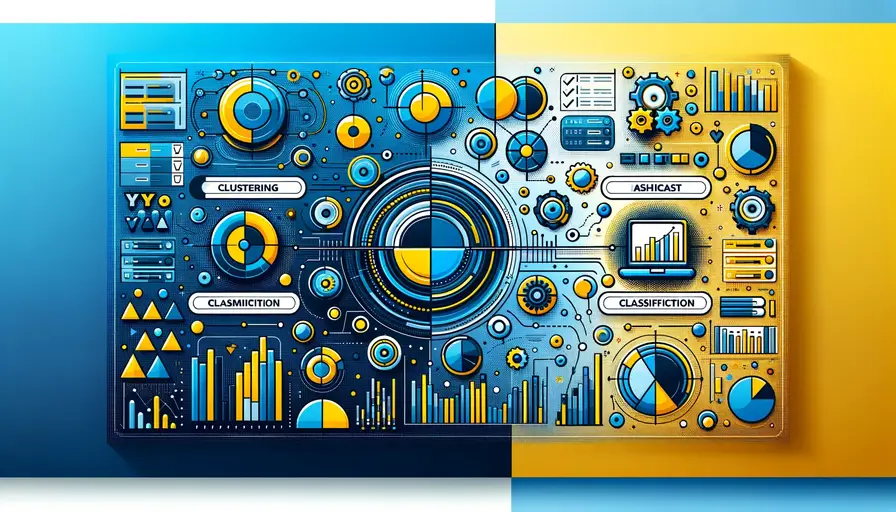
In the realm of machine learning, clustering and classification are two fundamental techniques used for analyzing and interpreting data. While they share similarities, they serve different purposes and are applied in distinct scenarios. This article delves into the differences between clustering and classification, highlighting their unique features, applications, and the contexts in which each should be used.
Understanding Clustering
Defining Clustering
Clustering is an unsupervised learning technique used to group similar data points into clusters. Unlike classification, which requires labeled data, clustering algorithms do not rely on predefined labels. Instead, they identify patterns and structures within the data, grouping data points that are more similar to each other than to those in other clusters.
Clustering is valuable for exploratory data analysis, helping to uncover hidden patterns and relationships within the data. It is often used in scenarios where the goal is to understand the inherent structure of the data, rather than to make specific predictions.
Common clustering algorithms include k-means, hierarchical clustering, and DBSCAN. Each algorithm has its strengths and is suited to different types of data and clustering tasks.
Intuition Behind K-means Algorithm in Machine LearningCalculating Clusters with K-Means
K-means is one of the most popular clustering algorithms. It partitions the data into ( k ) clusters, where each data point belongs to the cluster with the nearest mean. The algorithm iteratively updates the cluster centroids and reassigns data points to the nearest cluster until convergence.
Here is an example of using k-means clustering with scikit-learn:
from sklearn.cluster import KMeans
import numpy as np
import matplotlib.pyplot as plt
# Sample data
X = np.array([[1, 2], [1, 4], [1, 0], [4, 2], [4, 4], [4, 0]])
# Apply k-means clustering
kmeans = KMeans(n_clusters=2, random_state=0).fit(X)
labels = kmeans.labels_
centroids = kmeans.cluster_centers_
# Plot the clusters
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='viridis')
plt.scatter(centroids[:, 0], centroids[:, 1], c='red', marker='x')
plt.title('K-Means Clustering')
plt.show()
This code demonstrates how to perform k-means clustering on sample data, visualize the resulting clusters, and plot the cluster centroids.
Applications of Clustering
Clustering has a wide range of applications across various fields. In marketing, it is used for customer segmentation, allowing businesses to group customers based on similar behaviors and preferences. By understanding these segments, companies can tailor their marketing strategies to better meet the needs of each group.
Time Series Forecasting With RIn biology, clustering helps in analyzing gene expression data, identifying groups of genes with similar expression patterns. This can provide insights into gene functions and the underlying mechanisms of diseases, aiding in the development of new treatments.
Image processing also benefits from clustering, where it is used for image segmentation. By grouping similar pixels together, clustering algorithms can identify and isolate objects within an image, facilitating tasks such as object recognition and scene understanding.
Understanding Classification
Defining Classification
Classification is a supervised learning technique used to assign data points to predefined classes or categories. Unlike clustering, classification algorithms require labeled training data, where each data point is associated with a known class. The goal is to learn a mapping from input features to class labels, enabling the model to make predictions on new, unseen data.
Classification is widely used in applications where the objective is to predict the category or class of a given data point. It is particularly useful when there is a clear distinction between the classes and labeled data is available for training.
Maximizing Decision Tree Performance with Machine LearningCommon classification algorithms include logistic regression, decision trees, support vector machines (SVMs), and neural networks. Each algorithm has its advantages and is suitable for different types of classification tasks.
Predicting Classes with Logistic Regression
Logistic regression is a popular classification algorithm used for binary classification tasks. It models the probability that a given input belongs to a particular class using the logistic function. The model outputs a probability value, which can be thresholded to assign a class label.
Here is an example of using logistic regression with scikit-learn:
from sklearn.linear_model import LogisticRegression
import numpy as np
# Sample data
X = np.array([[1, 2], [2, 3], [3, 4], [4, 5], [5, 6]])
y = np.array([0, 0, 1, 1, 1])
# Create and train the model
model = LogisticRegression()
model.fit(X, y)
# Predict class labels for new data
X_new = np.array([[3, 3], [5, 5]])
y_pred = model.predict(X_new)
print(f'Predicted class labels: {y_pred}')
This code demonstrates how to train a logistic regression model on sample data and use it to predict class labels for new data points.
Strategies for Zero-Inflated Data in Machine Learning AlgorithmsApplications of Classification
Classification has numerous applications across different domains. In healthcare, it is used for disease diagnosis, where models predict the presence or absence of a disease based on patient data. This helps healthcare providers make informed decisions about treatment and management.
In finance, classification algorithms are employed for credit scoring, assessing the risk of loan applicants. By predicting the likelihood of default, financial institutions can make better lending decisions and manage risk more effectively.
Natural language processing (NLP) also benefits from classification, where it is used for tasks such as sentiment analysis, spam detection, and language translation. By categorizing text data into predefined classes, classification algorithms enable more effective communication and automation in various applications.
Comparing Clustering and Classification
Data Requirements
One of the key differences between clustering and classification lies in their data requirements. Clustering is an unsupervised learning technique that does not require labeled data. It is used to explore the inherent structure of the data and group similar data points together. This makes clustering suitable for scenarios where labeled data is scarce or unavailable.
Exploring Gradient Descent in Linear RegressionIn contrast, classification is a supervised learning technique that requires labeled data for training. The model learns from the labeled data to make predictions on new, unseen data. This makes classification suitable for scenarios where there is a clear distinction between classes and labeled data is available for training.
The choice between clustering and classification depends on the availability of labeled data and the specific goals of the analysis. If the goal is to explore and understand the structure of the data, clustering is the appropriate choice. If the goal is to predict class labels for new data points, classification is the appropriate choice.
Model Complexity and Interpretability
Another significant difference between clustering and classification is their model complexity and interpretability. Clustering algorithms are generally simpler and easier to interpret. For example, k-means clustering provides clear cluster centroids, and hierarchical clustering produces a dendrogram that visualizes the relationships between data points.
Classification algorithms, on the other hand, can range from simple models like logistic regression to complex models like neural networks. While simple classification models are easy to interpret, complex models can be more difficult to understand. However, complex models often provide higher accuracy and better performance, making them suitable for tasks where interpretability is less critical.
Feature Selection Methods in scikit-learn: A Comprehensive OverviewThe choice between clustering and classification also depends on the importance of model interpretability. If interpretability is crucial, clustering or simpler classification models may be preferred. If accuracy and performance are more important, complex classification models may be the better choice.
Handling Different Types of Data
Clustering and classification also differ in their ability to handle different types of data. Clustering algorithms are versatile and can be applied to various types of data, including numerical, categorical, and mixed data types. They can also handle high-dimensional data and identify patterns in complex datasets.
Classification algorithms are also versatile but may require more preprocessing and feature engineering to handle different types of data. For example, categorical data may need to be encoded, and numerical data may need to be scaled before training a classification model.
The choice between clustering and classification depends on the type of data and the specific requirements of the analysis. Clustering may be preferred for exploratory data analysis and pattern recognition in complex datasets. Classification may be preferred for predictive modeling and tasks that require precise class labels.
Choosing the Right Technique
When to Use Clustering
Clustering is the appropriate choice when the goal is to explore and understand the structure of the data without predefined labels. It is particularly useful for:
- Customer segmentation: Grouping customers based on similar behaviors and preferences to tailor marketing strategies.
- Anomaly detection: Identifying unusual patterns or outliers in the data, such as fraudulent transactions or network intrusions.
- Image segmentation: Dividing an image into meaningful segments to identify and isolate objects within the image.
- Gene expression analysis: Grouping genes with similar expression patterns to gain insights into gene functions and disease mechanisms.
Clustering is also useful for reducing the dimensionality of data and identifying patterns in high-dimensional datasets. By grouping similar data points together, clustering can simplify complex datasets and reveal hidden relationships.
When to Use Classification
Classification is the appropriate choice when the goal is to predict class labels for new data points based on labeled training data. It is particularly useful for:
- Disease diagnosis: Predicting the presence or absence of a disease based on patient data.
- Credit scoring: Assessing the risk of loan applicants by predicting the likelihood of default.
- Spam detection: Classifying emails as spam or not spam based on their content.
- Sentiment analysis: Categorizing text data into predefined classes, such as positive, negative, or neutral sentiment.
Classification is also useful for tasks that require precise and accurate predictions. By learning from labeled data, classification
algorithms can make informed predictions on new, unseen data, enabling more effective decision-making and automation.
Combining Clustering and Classification
In some cases, it may be beneficial to combine clustering and classification techniques to achieve better results. For example:
- Preprocessing for classification: Clustering can be used as a preprocessing step to create new features for a classification model. By grouping similar data points together, clustering can simplify the data and improve the performance of the classification model.
- Semi-supervised learning: Clustering can be used to generate labels for unlabeled data, which can then be used to train a classification model. This approach is useful when labeled data is scarce, and there is a need to leverage both labeled and unlabeled data.
- Hybrid models: Combining clustering and classification models can provide more robust and accurate predictions. For example, a hybrid model can use clustering to identify patterns in the data and classification to make precise predictions based on these patterns.
By combining the strengths of clustering and classification, it is possible to achieve better results and gain deeper insights into the data.
Practical Examples and Case Studies
Customer Segmentation with Clustering
Customer segmentation is a common application of clustering in marketing. By grouping customers based on similar behaviors and preferences, businesses can tailor their marketing strategies to better meet the needs of each segment. This leads to increased customer satisfaction, loyalty, and sales.
Here is an example of using k-means clustering for customer segmentation with scikit-learn:
from sklearn.cluster import KMeans
import pandas as pd
import matplotlib.pyplot as plt
# Sample data
data = {'Age': [25, 45, 35, 50, 23, 40, 38, 28],
'Income': [50000, 80000, 60000, 120000, 45000, 70000, 65000, 52000]}
df = pd.DataFrame(data)
# Apply k-means clustering
kmeans = KMeans(n_clusters=3, random_state=0).fit(df)
df['Cluster'] = kmeans.labels_
centroids = kmeans.cluster_centers_
# Plot the clusters
plt.scatter(df['Age'], df['Income'], c=df['Cluster'], cmap='viridis')
plt.scatter(centroids[:, 0], centroids[:, 1], c='red', marker='x')
plt.xlabel('Age')
plt.ylabel('Income')
plt.title('Customer Segmentation with K-Means Clustering')
plt.show()
This code demonstrates how to perform customer segmentation using k-means clustering and visualize the resulting clusters.
Disease Diagnosis with Classification
Disease diagnosis is a critical application of classification in healthcare. By predicting the presence or absence of a disease based on patient data, classification models help healthcare providers make informed decisions about diagnosis and treatment.
Here is an example of using logistic regression for disease diagnosis with scikit-learn:
from sklearn.linear_model import LogisticRegression
import numpy as np
# Sample data
X = np.array([[1, 50], [2, 60], [3, 70], [4, 80], [5, 90]])
y = np.array([0, 0, 1, 1, 1])
# Create and train the model
model = LogisticRegression()
model.fit(X, y)
# Predict class labels for new data
X_new = np.array([[2, 55], [4, 85]])
y_pred = model.predict(X_new)
print(f'Predicted class labels: {y_pred}')
This code demonstrates how to train a logistic regression model for disease diagnosis and use it to predict class labels for new patient data.
Image Segmentation with Clustering
Image segmentation is an important application of clustering in computer vision. By grouping similar pixels together, clustering algorithms can identify and isolate objects within an image, facilitating tasks such as object recognition and scene understanding.
Here is an example of using k-means clustering for image segmentation with scikit-learn and OpenCV:
import cv2
import numpy as np
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Load and reshape the image
image = cv2.imread('example_image.jpg')
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
pixels = image.reshape(-1, 3)
# Apply k-means clustering
kmeans = KMeans(n_clusters=3, random_state=0).fit(pixels)
segmented_pixels = kmeans.cluster_centers_[kmeans.labels_].reshape(image.shape).astype(np.uint8)
# Plot the segmented image
plt.imshow(segmented_pixels)
plt.title('Image Segmentation with K-Means Clustering')
plt.axis('off')
plt.show()
This code demonstrates how to perform image segmentation using k-means clustering and visualize the resulting segmented image.
Advanced Topics in Clustering and Classification
Dimensionality Reduction for Clustering
Dimensionality reduction is a technique used to reduce the number of features in a dataset while preserving important information. It is often used as a preprocessing step for clustering to simplify the data and improve the performance of clustering algorithms.
Principal Component Analysis (PCA) is a popular dimensionality reduction technique that transforms the data into a new set of orthogonal features (principal components) that capture the maximum variance in the data. By reducing the dimensionality of the data, PCA can help clustering algorithms identify patterns and structures more effectively.
Here is an example of using PCA for dimensionality reduction before clustering with scikit-learn:
from sklearn.decomposition import PCA
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Sample data
X = np.random.rand(100, 5)
# Apply PCA for dimensionality reduction
pca = PCA(n_components=2)
X_pca = pca.fit_transform(X)
# Apply k-means clustering
kmeans = KMeans(n_clusters=3, random_state=0).fit(X_pca)
labels = kmeans.labels_
# Plot the clusters
plt.scatter(X_pca[:, 0], X_pca[:, 1], c=labels, cmap='viridis')
plt.xlabel('Principal Component 1')
plt.ylabel('Principal Component 2')
plt.title('PCA and K-Means Clustering')
plt.show()
This code demonstrates how to use PCA for dimensionality reduction and apply k-means clustering to the transformed data.
Ensemble Methods for Classification
Ensemble methods combine multiple machine learning models to improve prediction accuracy and robustness. By leveraging the strengths of individual models, ensemble methods reduce the risk of overfitting and improve generalization to new data. Common ensemble methods include bagging, boosting, and stacking.
Random Forest is a popular bagging ensemble method that builds multiple decision trees on different subsets of the training data and averages their predictions. Gradient Boosting is a boosting method that sequentially trains models, each focusing on correcting the errors of the previous ones. Stacking combines the predictions of several models using a meta-model that learns how to best combine the base models' predictions.
Here is an example of using a Random Forest classifier with scikit-learn:
from sklearn.ensemble import RandomForestClassifier
import numpy as np
# Sample data
X = np.array([[1, 50], [2, 60], [3, 70], [4, 80], [5, 90]])
y = np.array([0, 0, 1, 1, 1])
# Create and train the model
model = RandomForestClassifier(n_estimators=100, random_state=0)
model.fit(X, y)
# Predict class labels for new data
X_new = np.array([[2, 55], [4, 85]])
y_pred = model.predict(X_new)
print(f'Predicted class labels: {y_pred}')
This code demonstrates how to train a Random Forest classifier and use it to predict class labels for new data points.
Handling Imbalanced Data in Classification
Imbalanced data is a common challenge in classification tasks, where one class significantly outnumbers the other. This can lead to biased models that favor the majority class and perform poorly on the minority class. To address this issue, various techniques can be used to handle imbalanced data.
Resampling techniques such as oversampling the minority class or undersampling the majority class can help balance the class distribution. Synthetic Minority Over-sampling Technique (SMOTE) is a popular method that generates synthetic samples for the minority class to balance the data.
Algorithmic techniques such as adjusting class weights or using cost-sensitive learning can also help address class imbalance. By giving more weight to the minority class or penalizing misclassifications of the minority class, these techniques can improve the model's performance on imbalanced data.
Here is an example of using SMOTE for handling imbalanced data with scikit-learn and imbalanced-learn:
from imblearn.over_sampling import SMOTE
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import classification_report
import numpy as np
# Sample data
X = np.array([[1, 50], [2, 60], [3, 70], [4, 80], [5, 90], [6, 100], [7, 110], [8, 120], [9, 130], [10, 140]])
y = np.array([0, 0, 0, 0, 0, 1, 1, 1, 1, 1])
# Apply SMOTE to balance the data
smote = SMOTE(random_state=0)
X_res, y_res = smote.fit_resample(X, y)
# Create and train the model
model = LogisticRegression()
model.fit(X_res, y_res)
# Predict class labels for new data
X_new = np.array([[2, 55], [4, 85]])
y_pred = model.predict(X_new)
print(f'Predicted class labels: {y_pred}')
This code demonstrates how to use SMOTE to balance imbalanced data and train a logistic regression model on the resampled data.
Clustering and classification are two fundamental techniques in machine learning, each with its unique strengths and applications. Clustering is an unsupervised learning technique used for exploratory data analysis and pattern recognition, while classification is a supervised learning technique used for predictive modeling and decision-making. By understanding the differences between clustering and classification and knowing when to use each, data scientists and practitioners can effectively analyze and interpret data, making informed decisions and driving better outcomes across various domains.
If you want to read more articles similar to Comparing Clustering vs Classification: When to Use Each, you can visit the Algorithms category.
You Must Read