
Comparing Machine Learning Techniques: Understanding Differences
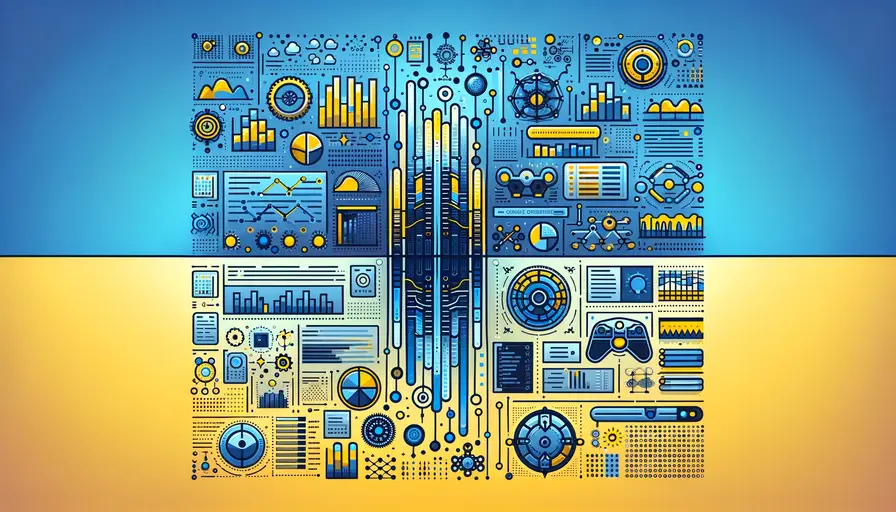
Machine learning has revolutionized various industries by enabling systems to learn from data and make predictions or decisions. However, with numerous machine learning techniques available, it can be challenging to determine which method is best suited for a particular problem.
Supervised Learning Techniques
Linear Regression
Linear regression is one of the most fundamental and widely used techniques in machine learning. It models the relationship between a dependent variable and one or more independent variables by fitting a linear equation to observed data. Linear regression is particularly effective for predicting continuous outcomes.
This technique assumes a linear relationship between the input variables and the output, which may not always hold true in real-world scenarios. Despite this limitation, linear regression is valued for its simplicity, interpretability, and efficiency. It is commonly used in fields such as economics, biology, and social sciences to predict trends and analyze relationships.
Example of linear regression using scikit-learn:
Analysis of Popular Off-the-Shelf Machine Learning Modelsimport numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
# Generate synthetic data
np.random.seed(0)
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
# Fit linear regression model
model = LinearRegression()
model.fit(X, y)
# Predict values
X_new = np.array([[0], [2]])
y_pred = model.predict(X_new)
# Plot the results
plt.scatter(X, y, color='blue')
plt.plot(X_new, y_pred, color='red', linewidth=2)
plt.xlabel("X")
plt.ylabel("y")
plt.title("Linear Regression Example")
plt.show()
Decision Trees
Decision trees are versatile models used for both classification and regression tasks. They work by splitting the data into subsets based on the value of input features, creating a tree-like structure. Each internal node represents a test on an attribute, each branch represents the outcome of the test, and each leaf node represents a class label or continuous value.
Decision trees are easy to interpret and visualize. However, they are prone to overfitting, especially when the tree is deep. Pruning, which involves removing branches that provide little value, can help mitigate overfitting. Decision trees are widely used in various domains, including finance, healthcare, and marketing.
Example of a decision tree classifier using scikit-learn:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn import tree
import matplotlib.pyplot as plt
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train Decision Tree classifier
model = DecisionTreeClassifier(max_depth=3)
model.fit(X_train, y_train)
# Plot the tree
plt.figure(figsize=(12,8))
tree.plot_tree(model, filled=True, feature_names=iris.feature_names, class_names=iris.target_names)
plt.show()
Support Vector Machines
Support Vector Machines (SVMs) are powerful supervised learning models used for classification and regression tasks. They work by finding the optimal hyperplane that maximizes the margin between different classes in the feature space. SVMs are effective in high-dimensional spaces and are particularly useful when the number of dimensions exceeds the number of samples.
Building Machine Learning AISVMs can handle linear and non-linear classification tasks by using kernel functions such as linear, polynomial, and radial basis function (RBF) kernels. They are known for their robustness to overfitting, especially in high-dimensional spaces. However, SVMs can be computationally intensive and require careful tuning of hyperparameters.
Example of an SVM classifier using scikit-learn:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Load dataset
iris = datasets.load_iris()
X, y = iris.data, iris.target
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train SVM classifier
model = SVC(kernel='linear')
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Unsupervised Learning Techniques
K-Means Clustering
K-means clustering is a popular unsupervised learning technique used to partition data into k distinct clusters based on feature similarity. It works by initializing k centroids and iteratively refining them until convergence, ensuring that the sum of the squared distances between data points and their nearest centroid is minimized.
K-means is efficient and easy to implement, making it a go-to method for clustering tasks. However, it requires specifying the number of clusters in advance and can struggle with clusters of varying shapes and densities. It is widely used in market segmentation, image compression, and anomaly detection.
The Role of Abstract Algebra in Data Analysis for Machine LearningExample of K-means clustering using scikit-learn:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.cluster import KMeans
# Generate synthetic data
np.random.seed(0)
X = np.random.rand(100, 2)
# Apply K-means clustering
kmeans = KMeans(n_clusters=3, random_state=0).fit(X)
# Plot the results
plt.scatter(X[:, 0], X[:, 1], c=kmeans.labels_, cmap='viridis')
plt.scatter(kmeans.cluster_centers_[:, 0], kmeans.cluster_centers_[:, 1], s=200, c='red', marker='X')
plt.xlabel("Feature 1")
plt.ylabel("Feature 2")
plt.title("K-Means Clustering Example")
plt.show()
Principal Component Analysis
Principal Component Analysis (PCA) is a dimensionality reduction technique that transforms high-dimensional data into a lower-dimensional space while preserving as much variance as possible. It does so by identifying the principal components, which are orthogonal vectors that capture the maximum variance in the data.
PCA is valuable for visualizing high-dimensional data, noise reduction, and feature extraction. It is commonly used in image processing, genomics, and finance. However, PCA assumes linearity and may not capture complex relationships in the data.
Example of PCA using scikit-learn:
KNN Machine Learning in R: A Syntax Guideimport numpy as np
import matplotlib.pyplot as plt
from sklearn.decomposition import PCA
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X = iris.data
# Apply PCA
pca = PCA(n_components=2)
principal_components = pca.fit_transform(X)
# Plot the results
plt.scatter(principal_components[:, 0], principal_components[:, 1], c=iris.target, cmap='viridis')
plt.xlabel("Principal Component 1")
plt.ylabel("Principal Component 2")
plt.title("PCA Example")
plt.show()
Hierarchical Clustering
Hierarchical clustering is another unsupervised learning technique that builds a hierarchy of clusters. There are two main types: agglomerative (bottom-up) and divisive (top-down). Agglomerative clustering starts with each data point as a separate cluster and merges them iteratively, while divisive clustering starts with one cluster and splits it iteratively.
Hierarchical clustering does not require specifying the number of clusters in advance and provides a dendrogram that shows the hierarchy of clusters. This makes it useful for exploratory data analysis. However, it can be computationally intensive and sensitive to noise.
Example of hierarchical clustering using scipy:
import numpy as np
import matplotlib.pyplot as plt
from scipy.cluster.hierarchy import dendrogram, linkage
# Generate synthetic data
np.random.seed(0)
X = np.random.rand(30, 2)
# Apply hierarchical clustering
linked = linkage(X, 'single')
# Plot the dendrogram
plt.figure(figsize=(10, 7))
dendrogram(linked, labels=np.arange(1, 31))
plt.title("Hierarchical Clustering Dendrogram")
plt.xlabel("Sample index")
plt.ylabel("Distance")
plt.show()
Reinforcement Learning Techniques
Q-Learning
Q-Learning is a model-free reinforcement learning algorithm that aims to learn the optimal policy for an agent interacting with an environment. It uses a Q-table to store the expected rewards for state-action pairs and updates it iteratively using the Bellman equation.
Bayesian Machine Learning for AB Testing with Python TechniquesQ-Learning is effective for environments with discrete state and action spaces. It is commonly used in robotics, game playing, and autonomous driving. However, it can struggle with large state-action spaces and requires sufficient exploration to find the optimal policy.
Example of Q-Learning using NumPy:
import numpy as np
# Define the environment
n_states = 5
n_actions = 2
Q = np.zeros((n_states, n_actions))
learning_rate = 0.1
discount_factor = 0.9
epsilon = 0.1
# Define a simple policy (epsilon-greedy)
def choose_action(state):
if np.random.uniform(0, 1) < epsilon:
return np.random.choice(n_actions)
else:
return np.argmax(Q[state, :])
# Simulate an episode
for episode in range(1000):
state = np.random.choice(n_states)
while state != n_states - 1:
action = choose_action(state)
next_state = (state + action) % n_states
reward = 1 if next_state == n_states - 1 else 0
Q[state, action] = Q[state, action] + learning_rate * (reward + discount_factor * np.max(Q[next_state, :]) - Q[state, action])
state = next_state
# Display the Q-table
print("Learned Q-table:")
print(Q)
Deep Q-Networks
Deep Q-Networks (DQNs) extend Q-Learning by using deep neural networks to approximate the Q-function. This allows DQNs to handle environments with high-dimensional state spaces, such as video games. DQNs use experience replay and target networks to stabilize training.
DQNs have achieved impressive results in various applications, including playing Atari games and robotic control. However, they require substantial computational resources and careful tuning of hyperparameters. Despite these challenges, DQNs represent a significant advancement in reinforcement learning.
Machine Learning vs AI: Understanding the DifferenceExample of a DQN using TensorFlow/Keras:
import numpy as np
import tensorflow as tf
from tensorflow.keras import layers, models
import random
# Define the environment (simple grid world)
n_states = 10
n_actions = 2
# Define the DQN model
model = models.Sequential([
layers.Dense(24, activation='relu', input_shape=(n_states,)),
layers.Dense(24, activation='relu'),
layers.Dense(n_actions, activation='linear')
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=0.001), loss='mse')
# Define the replay buffer
replay_buffer = []
# Training parameters
batch_size = 32
gamma = 0.99
# Training loop
for episode in range(1000):
state = np.zeros(n_states)
state[0] = 1 # Start state
done = False
while not done:
# Choose action (epsilon-greedy)
if np.random.rand() < 0.1:
action = np.random.choice(n_actions)
else:
q_values = model.predict(state.reshape(1, -1))
action = np.argmax(q_values[0])
# Take action
next_state = np.roll(state, action) # Simple state transition
reward = 1 if next_state[-1] == 1 else 0
done = next_state[-1] == 1
# Store experience in replay buffer
replay_buffer.append((state, action, reward, next_state, done))
if len(replay_buffer) > 1000:
replay_buffer.pop(0)
# Sample mini-batch from replay buffer
if len(replay_buffer) >= batch_size:
batch = random.sample(replay_buffer, batch_size)
states, actions, rewards, next_states, dones = zip(*batch)
# Convert to NumPy arrays
states = np.array(states)
actions = np.array(actions)
rewards = np.array(rewards)
next_states = np.array(next_states)
dones = np.array(dones)
# Compute Q-values and targets
q_values = model.predict(states)
next_q_values = model.predict(next_states)
targets = rewards + gamma * np.max(next_q_values, axis=1) * (1 - dones)
# Update Q-values
for i in range(batch_size):
q_values[i, actions[i]] = targets[i]
# Train the model
model.train_on_batch(states, q_values)
state = next_state
# Display the learned Q-values
print("Learned Q-values:")
for state in range(n_states):
print(f"State {state}: {model.predict(np.eye(n_states)[state].reshape(1, -1))[0]}")
Policy Gradient Methods
Policy gradient methods are a class of reinforcement learning algorithms that optimize the policy directly. Unlike value-based methods like Q-Learning and DQNs, policy gradient methods parameterize the policy and update it using gradient ascent on the expected reward.
Policy gradient methods, such as REINFORCE and Actor-Critic, are well-suited for continuous action spaces and complex environments. They have been successfully applied to robotic control, autonomous driving, and game playing. However, they can be sensitive to hyperparameters and require extensive training data.
Example of a policy gradient method using TensorFlow/Keras:
import numpy as np
import tensorflow as tf
from tensorflow.keras import layers, models
import gym
# Define the environment
env = gym.make('CartPole-v1')
# Define the policy model
model = models.Sequential([
layers.Dense(24, activation='relu', input_shape=(env.observation_space.shape[0],)),
layers.Dense(24, activation='relu'),
layers.Dense(env.action_space.n, activation='softmax')
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=0.001), loss='categorical_crossentropy')
# Training parameters
n_episodes = 1000
gamma = 0.99
# Training loop
for episode in range(n_episodes):
state = env.reset()
states, actions, rewards = [], [], []
done = False
while not done:
# Choose action
action_probs = model.predict(state.reshape(1, -1))
action = np.random.choice(env.action_space.n, p=action_probs[0])
# Take action
next_state, reward, done, _ = env.step(action)
# Store experience
states.append(state)
actions.append(action)
rewards.append(reward)
state = next_state
# Compute returns
returns = []
G = 0
for reward in reversed(rewards):
G = reward + gamma * G
returns.insert(0, G)
returns = np.array(returns)
# Normalize returns
returns = (returns - np.mean(returns)) / (np.std(returns) + 1e-6)
# Update policy
states = np.array(states)
actions = np.array(actions)
action_masks = tf.keras.utils.to_categorical(actions, num_classes=env.action_space.n)
model.train_on_batch(states, action_masks, sample_weight=returns)
# Test the learned policy
state = env.reset()
done = False
while not done:
env.render()
action_probs = model.predict(state.reshape(1, -1))
action = np.argmax(action_probs[0])
state, _, done, _ = env.step(action)
env.close()
Comparing Model Performance
Evaluation Metrics
Choosing the right evaluation metrics is crucial for comparing machine learning techniques. For classification tasks, common metrics include accuracy, precision, recall, F1-score, and ROC-AUC. For regression tasks, metrics such as mean squared error (MSE), mean absolute error (MAE), and R-squared are used.
These metrics provide insights into different aspects of model performance. For instance, accuracy measures the overall correctness, while precision and recall focus on the model's performance for specific classes. ROC-AUC evaluates the trade-off between true positive and false positive rates.
Example of evaluating a classification model using scikit-learn:
from sklearn.metrics import classification_report, confusion_matrix
# Generate classification report and confusion matrix
print(classification_report(y_test, y_pred))
print(confusion_matrix(y_test, y_pred))
Cross-Validation
Cross-validation is a robust technique for evaluating machine learning models. It involves splitting the dataset into k folds and training the model on k-1 folds while using the remaining fold for testing. This process is repeated k times, and the results are averaged to obtain a more reliable estimate of model performance.
Cross-validation helps in assessing the model's generalizability and reducing the risk of overfitting. It is particularly useful when the dataset is small, as it maximizes the use of available data.
Example of cross-validation using scikit-learn:
from sklearn.model_selection import cross_val_score
# Perform 5-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
print(f'Cross-validation scores: {scores}')
print(f'Mean cross-validation score: {np.mean(scores)}')
Hyperparameter Tuning
Hyperparameter tuning is the process of optimizing the hyperparameters of a machine learning model to improve its performance. Techniques such as grid search, random search, and Bayesian optimization are commonly used to find the best hyperparameter values.
Grid search exhaustively searches through a specified parameter grid, while random search samples random combinations of parameters. Bayesian optimization uses probabilistic models to guide the search for optimal hyperparameters. Effective hyperparameter tuning can significantly enhance model performance.
Example of hyperparameter tuning using scikit-learn:
from sklearn.model_selection import GridSearchCV
# Define parameter grid
param_grid = {
'max_depth': [3, 5, 7],
'min_samples_split': [2, 5, 10]
}
# Perform grid search
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X_train, y_train)
# Display best parameters
print(f'Best parameters: {grid_search.best_params_}')
print(f'Best cross-validation score: {grid_search.best_score_}')
Comparing machine learning techniques involves understanding their strengths, weaknesses, and appropriate use cases. By exploring different supervised, unsupervised, and reinforcement learning methods, and evaluating their performance using appropriate metrics, cross-validation, and hyperparameter tuning, data scientists can select the most suitable technique for their specific problem. The key is to continuously experiment, learn, and adapt to the evolving landscape of machine learning.
If you want to read more articles similar to Comparing Machine Learning Techniques: Understanding Differences, you can visit the Artificial Intelligence category.
You Must Read