
Comparing X and Y: Evaluating the Superiority for Machine Learning
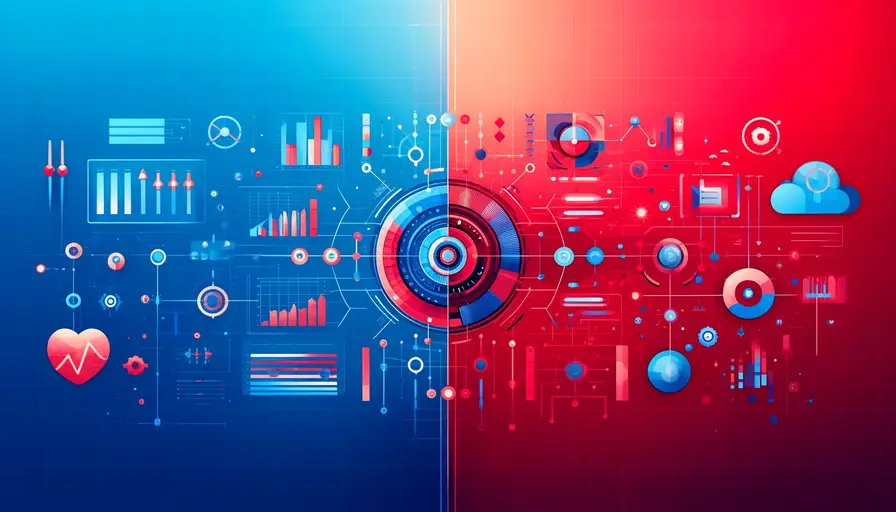
In the ever-evolving field of machine learning, comparing different algorithms and techniques is crucial to determine which ones are best suited for specific tasks. This article will delve into the comparison of two prominent approaches, referred to as "X" and "Y", examining their strengths, weaknesses, and practical applications. By exploring various facets of these techniques, we aim to provide a comprehensive guide to help practitioners make informed decisions in their machine learning endeavors.
Evaluating Algorithm Performance
Accuracy and Precision
When it comes to machine learning, accuracy and precision are vital metrics for evaluating the performance of an algorithm. Accuracy measures the proportion of correct predictions among the total number of cases examined, while precision focuses on the correctness of positive predictions.
Algorithm X, often celebrated for its high accuracy, is particularly effective in scenarios with balanced datasets. However, in cases where the data is imbalanced, its precision might not be as high, leading to a trade-off between these two metrics. Algorithm Y, on the other hand, tends to maintain better precision across varied datasets, making it suitable for applications where false positives are costly.
Example of calculating accuracy and precision using scikit-learn
:
from sklearn.metrics import accuracy_score, precision_score
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
import pandas as pd
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize and train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Calculate accuracy and precision
accuracy = accuracy_score(y_test, y_pred)
precision = precision_score(y_test, y_pred)
print("Accuracy:", accuracy)
print("Precision:", precision)
Speed and Scalability
Another critical aspect of algorithm evaluation is speed and scalability. Speed refers to how quickly an algorithm can process data and generate predictions, while scalability indicates the algorithm’s ability to handle increasing amounts of data without a significant drop in performance.
Algorithm X is known for its rapid processing capabilities, making it ideal for real-time applications. It can handle large datasets efficiently, scaling well with the growing size of data. Algorithm Y, while slightly slower, offers robust performance with moderate-sized datasets. However, its scalability can be a concern when dealing with extensive data, requiring additional resources to maintain performance levels.
Example of timing an algorithm using time
module in Python:
import time
from sklearn.linear_model import LogisticRegression
# Initialize the model
model = LogisticRegression()
# Measure the time taken to train the model
start_time = time.time()
model.fit(X_train, y_train)
end_time = time.time()
training_time = end_time - start_time
print("Training Time:", training_time)
Flexibility and Adaptability
Flexibility and adaptability are essential for machine learning algorithms to perform well across diverse datasets and changing conditions. Flexibility refers to an algorithm’s ability to be applied to various problem types, while adaptability indicates how well it can adjust to new data or modifications in the data environment.
Machine Learning in IT SystemsAlgorithm X exhibits high flexibility, suitable for a wide range of applications from classification to regression tasks. Its adaptability is enhanced by its ability to incorporate new data without extensive retraining. Algorithm Y, while also flexible, excels in scenarios requiring customization and fine-tuning. Its adaptable nature makes it a strong candidate for complex problems where specific adjustments can lead to significant performance improvements.
Example of using an algorithm in both classification and regression tasks:
from sklearn.tree import DecisionTreeClassifier, DecisionTreeRegressor
# Classification task
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
y_pred_class = clf.predict(X_test)
# Regression task
reg = DecisionTreeRegressor()
reg.fit(X_train, y_train)
y_pred_reg = reg.predict(X_test)
print("Classification Predictions:", y_pred_class)
print("Regression Predictions:", y_pred_reg)
Practical Applications
Healthcare Diagnostics
In healthcare, the choice of machine learning algorithms can significantly impact the accuracy of diagnostics and patient outcomes. Algorithm X is often utilized in diagnostic applications due to its high accuracy and speed. For example, it can quickly analyze medical images to detect anomalies, such as tumors or fractures, providing doctors with timely and accurate information.
Algorithm Y, with its adaptability, is valuable in personalized medicine. By analyzing patient data, it can tailor treatment plans to individual needs, predicting responses to various treatments and medications. This personalized approach can improve patient outcomes and reduce the likelihood of adverse reactions.
Machine Learning and PredictionExample of using machine learning for healthcare diagnostics:
from sklearn.datasets import load_breast_cancer
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
# Load dataset
data = load_breast_cancer()
X = data.data
y = data.target
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize and train the model
model = SVC(kernel='linear', probability=True)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
print("Diagnostic Predictions:", y_pred)
Financial Forecasting
Machine learning plays a crucial role in financial forecasting, where predicting market trends and identifying investment opportunities are vital. Algorithm X, with its fast processing and scalability, is ideal for real-time stock market analysis. It can process large volumes of market data, detecting patterns and trends that inform trading strategies.
Algorithm Y is suited for risk assessment and credit scoring. Its precision and adaptability allow it to evaluate the risk associated with loans and investments accurately. By analyzing historical data and current market conditions, it provides financial institutions with insights to make informed decisions and mitigate risks.
Example of using machine learning for financial forecasting:
Is Machine Learning the Same as Artificial Intelligence?from sklearn.ensemble import RandomForestRegressor
# Load dataset
data = pd.read_csv('financial_data.csv')
X = data.drop('stock_price', axis=1)
y = data['stock_price']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize and train the model
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
print("Stock Price Predictions:", y_pred)
E-commerce Personalization
In the realm of e-commerce, machine learning enhances the customer experience through personalization. Algorithm X is often employed in recommendation systems, where its speed and accuracy are crucial for suggesting products to customers in real-time. By analyzing user behavior and preferences, it can recommend products that match individual tastes, increasing the likelihood of purchases.
Algorithm Y, with its flexibility, is used to optimize marketing strategies. By segmenting customers based on their purchasing behavior and preferences, it helps businesses tailor their marketing efforts to different customer groups. This targeted approach enhances engagement and boosts sales.
Example of using machine learning for e-commerce personalization:
from surprise import Dataset, Reader, KNNBasic
# Load dataset
data = Dataset.load_builtin('ml-100k')
trainset, testset = train_test_split(data, test_size=0.2)
# Initialize and train the KNNBasic model
algo = KNNBasic()
algo.fit(trainset)
# Make predictions
predictions = algo.test(testset)
print("E-commerce Recommendations:", predictions)
Future Prospects and Innovations
Integration with IoT
The integration of machine learning with the Internet of Things (IoT) is poised to revolutionize various industries. Algorithm X can process data from IoT devices in real-time, enabling immediate responses and decision-making. For instance, in smart homes, it can analyze data from sensors to optimize energy usage and enhance security.
Optimal Machine Learning Algorithms for Training AI in GamesAlgorithm Y can leverage IoT data for predictive maintenance in industrial settings. By monitoring machinery and equipment, it can predict failures and schedule maintenance proactively, reducing downtime and operational costs.
Example of integrating machine learning with IoT using Python:
import time
import Adafruit_DHT
from sklearn.ensemble import GradientBoostingClassifier
# Sensor type and GPIO pin
sensor = Adafruit_DHT.DHT22
pin = 4
# Load dataset
data = pd.read_csv('iot_data.csv')
X = data.drop('failure', axis=1)
y = data['failure']
# Initialize and train the model
model = GradientBoostingClassifier(n_estimators=100, learning_rate=0.1, random_state=42)
model.fit(X, y)
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if humidity is not None and temperature is not None:
features = [[temperature, humidity]]
prediction = model.predict(features)
print(f'Temperature: {temperature:.1f}°C, Humidity: {humidity:.1f}%, Failure Prediction: {prediction[0]}')
else:
print('Failed to get reading. Try again!')
time.sleep(2)
Advances in Explainability
As machine learning models become more complex, explainability is becoming increasingly important. Algorithm X is benefiting from advances in explainable AI (XAI) techniques, such as SHAP (Shapley Additive Explanations) and LIME (Local Interpretable Model-agnostic Explanations), which provide insights into model predictions and build trust with users.
Algorithm Y is also seeing improvements in explainability, particularly in high-stakes applications like healthcare and finance. By understanding how models make decisions, practitioners can ensure that the models are fair, transparent, and accountable.
Is CNN a Machine Learning Algorithm? A Comprehensive AnalysisExample of using SHAP for model interpretability:
import shap
from sklearn.ensemble import RandomForestClassifier
# Train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Create a SHAP explainer
explainer = shap.TreeExplainer(model)
# Calculate SHAP values
shap_values = explainer.shap_values(X_test)
# Plot SHAP values
shap.summary_plot(shap_values, X_test)
Ethical Considerations
Ethical considerations are paramount as machine learning becomes more integrated into our daily lives. Algorithm X must be designed to avoid biases and ensure fairness, especially in applications like hiring, lending, and law enforcement. Implementing robust frameworks and conducting regular audits can help mitigate ethical concerns.
Algorithm Y needs to prioritize data privacy and security, particularly when dealing with sensitive information. Techniques such as differential privacy and secure multi-party computation can enhance data protection and ensure compliance with regulations.
Example of implementing differential privacy using diffprivlib
:
from diffprivlib.models import LogisticRegression
from sklearn.model_selection import train_test_split
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize the differentially private logistic regression model
model = LogisticRegression(epsilon=1.0)
# Train the model
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
print("Predictions with Differential Privacy:", y_pred)
The comparison of Algorithm X and Algorithm Y reveals their distinct strengths and weaknesses, making each suitable for different applications. By understanding these differences, practitioners can make informed decisions about which algorithm to use based on their specific needs and goals. As machine learning continues to evolve, ongoing research and innovation will further enhance the capabilities and applications of these algorithms, driving progress across various fields.
If you want to read more articles similar to Comparing X and Y: Evaluating the Superiority for Machine Learning, you can visit the Artificial Intelligence category.
You Must Read