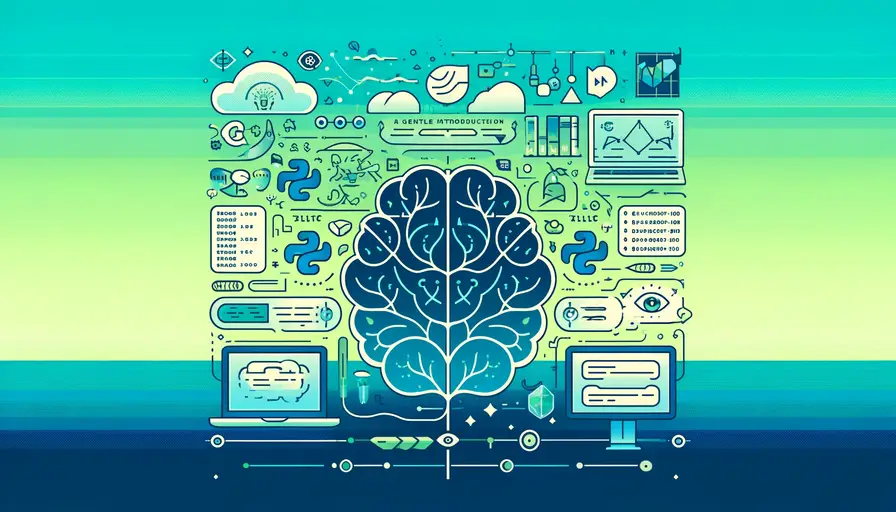
Decision Tree vs Random Forest
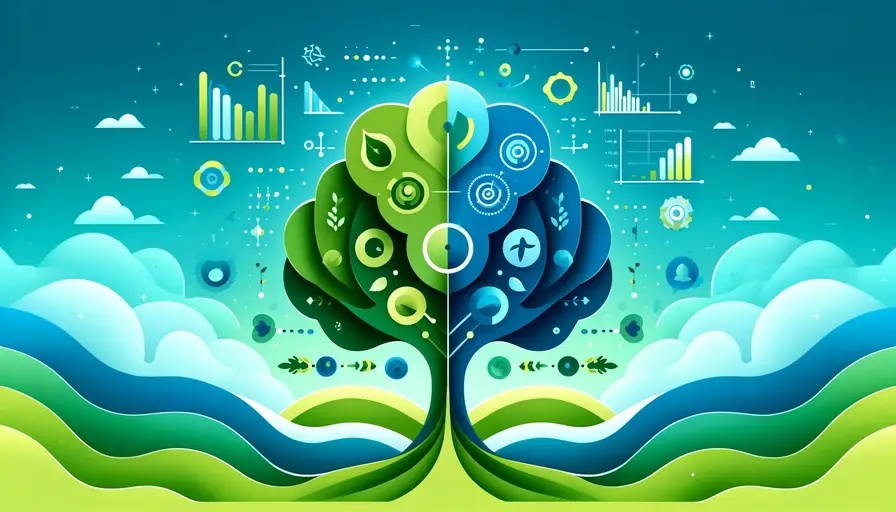
Decision Trees: Powerful and Interpretable
Decision trees are a powerful and interpretable classification algorithm widely used in various applications. They work by splitting the data into subsets based on the value of input features, creating a tree-like model of decisions.
Structure and Functionality
A decision tree is built by recursively partitioning the data into subsets based on the feature that provides the highest information gain. Each node represents a decision based on a feature value, and each branch represents the outcome of the decision, leading to further splits or final predictions.
Advantages of Decision Trees
The primary advantage of decision trees is their interpretability. They provide a clear visual representation of the decision-making process, making it easy to understand how the model arrives at its predictions. This transparency is particularly valuable in fields where interpretability is crucial, such as healthcare and finance.
Example of Decision Tree in Python
Here's an example of creating and visualizing a decision tree using Python and scikit-learn:

from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn import tree
import matplotlib.pyplot as plt
# Load data
iris = load_iris()
X, y = iris.data, iris.target
# Train decision tree
model = DecisionTreeClassifier()
model.fit(X, y)
# Plot the decision tree
plt.figure(figsize=(12, 8))
tree.plot_tree(model, filled=True)
plt.show()
Random Forests: An Ensemble of Decision Trees
Random forests are an ensemble of decision trees that combine the predictions of multiple trees to improve accuracy and robustness. This approach mitigates the limitations of individual decision trees by averaging their results.
How Random Forests Work
Random forests create multiple decision trees using different subsets of the data and features. Each tree is trained independently, and the final prediction is made by averaging the predictions of all trees. This process reduces variance and improves generalization.
Advantages of Random Forests
The main advantages of random forests include higher accuracy and robustness compared to individual decision trees. They are less prone to overfitting because they average the results of many trees, each trained on different data subsets. This makes random forests suitable for complex datasets with high variability.
Example of Random Forest in Python
Here's an example of creating and using a random forest for classification using Python and scikit-learn:

from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
# Load data
iris = load_iris()
X, y = iris.data, iris.target
# Train random forest
model = RandomForestClassifier(n_estimators=100)
model.fit(X, y)
# Predict and evaluate
predictions = model.predict(X)
print(f'Accuracy: {model.score(X, y)}')
Overfitting: Decision Trees vs Random Forests
Decision trees are prone to overfitting, especially when they are deep and complex. Overfitting occurs when the model captures noise in the training data, leading to poor generalization on new data.
Prone to Overfitting
Decision trees can create very specific rules to fit the training data perfectly, which may not generalize well to unseen data. This overfitting results in high variance and lower accuracy on test data.
Mitigating Overfitting with Random Forests
Random forests help mitigate the overfitting issue by averaging the predictions of multiple trees. Each tree in the forest is trained on a different subset of the data, reducing the model's sensitivity to noise and improving its generalization ability.
Example of Overfitting Mitigation
Here's an example of how random forests reduce overfitting compared to a single decision tree using Python:

from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train decision tree
tree_model = DecisionTreeClassifier()
tree_model.fit(X_train, y_train)
tree_predictions = tree_model.predict(X_test)
print(f'Decision Tree Accuracy: {accuracy_score(y_test, tree_predictions)}')
# Train random forest
forest_model = RandomForestClassifier(n_estimators=100)
forest_model.fit(X_train, y_train)
forest_predictions = forest_model.predict(X_test)
print(f'Random Forest Accuracy: {accuracy_score(y_test, forest_predictions)}')
Handling Input Features: Decision Trees vs Random Forests
Random forests can handle a larger number of input features compared to decision trees. This capability makes them more suitable for high-dimensional datasets.
Feature Handling in Decision Trees
Decision trees split the data based on the most informative features, but they may struggle with high-dimensional data where the number of features is very large. This can lead to overfitting and decreased performance.
Random Forests and High-Dimensional Data
Random forests handle high-dimensional data better because each tree is trained on a random subset of features. This randomness reduces the risk of overfitting and improves the model's ability to generalize.
Example of Feature Handling
Here's an example demonstrating how random forests can handle high-dimensional data using Python:

from sklearn.datasets import make_classification
# Generate high-dimensional data
X, y = make_classification(n_samples=1000, n_features=100, n_informative=10, random_state=42)
# Train random forest
forest_model = RandomForestClassifier(n_estimators=100)
forest_model.fit(X, y)
print(f'Random Forest Accuracy: {forest_model.score(X, y)}')
Visualization and Interpretability
Decision trees are easier to visualize and understand compared to random forests. Their simple, hierarchical structure allows for straightforward interpretation of the decision-making process.
Visualizing Decision Trees
Decision trees provide a clear visual representation of how decisions are made based on feature values. This interpretability is valuable for explaining the model to stakeholders and understanding the factors influencing predictions.
Random Forests Complexity
Random forests, being an ensemble of many trees, do not offer the same level of interpretability. The combined predictions of multiple trees can be difficult to visualize and understand, making it challenging to explain the model's decision-making process.
Example of Decision Tree Visualization
Here's an example of visualizing a decision tree using Python and scikit-learn:

from sklearn.tree import plot_tree
# Train decision tree
tree_model = DecisionTreeClassifier()
tree_model.fit(X, y)
# Plot the decision tree
plt.figure(figsize=(20, 10))
plot_tree(tree_model, filled=True)
plt.show()
Computational Expense
Random forests are computationally more expensive than decision trees due to the ensemble nature of the algorithm. Training and predicting with multiple trees require more resources and time.
Computational Efficiency of Decision Trees
Decision trees are relatively fast to train and predict, making them suitable for applications where computational resources are limited or real-time predictions are required.
Random Forests Resource Requirements
Random forests require more computational power and memory as they involve training and maintaining multiple decision trees. This can be a limitation in scenarios with large datasets or constrained computational resources.
Example of Computational Expense
Here's an example demonstrating the difference in training time between a decision tree and a random forest using Python:

import time
# Train decision tree
start_time = time.time()
tree_model = DecisionTreeClassifier()
tree_model.fit(X, y)
print(f'Decision Tree Training Time: {time.time() - start_time} seconds')
# Train random forest
start_time = time.time()
forest_model = RandomForestClassifier(n_estimators=100)
forest_model.fit(X, y)
print(f'Random Forest Training Time: {time.time() - start_time} seconds')
Choosing the Right Algorithm
The choice between decision tree and random forest depends on the specific dataset and problem at hand. Both algorithms have their strengths and weaknesses, and the best choice varies based on the context.
Dataset Characteristics
Consider the characteristics of your dataset when choosing the algorithm. If interpretability is crucial and the dataset is relatively simple, a decision tree may be the better choice. For more complex datasets with high variability, a random forest is likely to perform better.
Problem Requirements
Evaluate the specific requirements of your problem. If computational resources are limited, a decision tree may be preferable due to its efficiency. However, if accuracy and robustness are paramount, the additional computational expense of a random forest may be justified.
Example of Algorithm Selection
Here's an example demonstrating how to choose between a decision tree and a random forest using Python:
# Define a function to compare models
def compare_models(X, y):
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train and evaluate decision tree
tree_model = DecisionTreeClassifier()
tree_model.fit(X_train, y_train)
tree_accuracy = accuracy_score(y_test, tree_model.predict(X_test))
# Train and evaluate random forest
forest_model = RandomForestClassifier(n_estimators=100)
forest_model.fit(X_train, y_train)
forest_accuracy = accuracy_score(y_test, forest_model.predict(X_test))
return tree_accuracy, forest_accuracy
# Load data and compare models
iris = load_iris()
X, y = iris.data, iris.target
tree_acc, forest_acc = compare_models(X, y)
print(f'Decision Tree Accuracy: {tree_acc}')
print(f'Random Forest Accuracy: {forest_acc}')
Both decision trees and random forests have their unique advantages and limitations.
Decision trees offer simplicity and interpretability, making them suitable for straightforward problems. Random forests, on the other hand, provide higher accuracy and robustness, particularly for complex datasets. The choice between these algorithms should be based on the specific requirements of the problem, the nature of the data, and the available computational resources. By carefully considering these factors, you can select the most appropriate algorithm for your classification tasks.
If you want to read more articles similar to Decision Tree vs Random Forest, you can visit the Algorithms category.
You Must Read