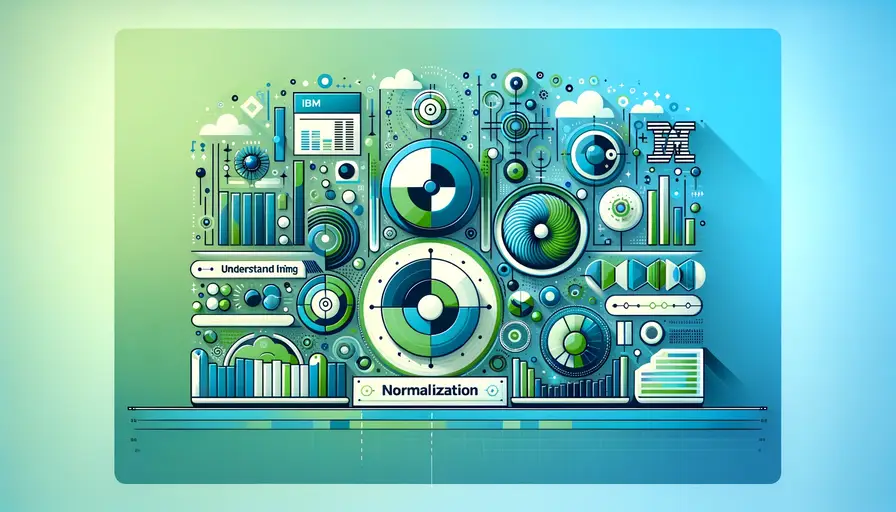
Decoding Decision Trees: A Crucial Machine Learning Algorithm

- Understand the Basic Concepts of Decision Trees
- Learn How Decision Trees Are Constructed
- Use Decision Trees for Classification
- Use Decision Trees for Regression
- Implement Decision Trees in ML Libraries
- Handle Missing Data in Decision Trees
- Prune Decision Trees to Avoid Overfitting
- Interpret the Results of Decision Trees
- Combine Decision Trees with Other Algorithms
- Use Decision Trees for Feature Selection
Understand the Basic Concepts of Decision Trees
What is a Decision Tree?
A decision tree is a type of supervised learning algorithm used for both classification and regression tasks. It operates by splitting the data into subsets based on the values of input features, creating a tree-like model of decisions and their possible consequences. Each internal node represents a "test" or "decision" on an attribute, each branch represents the outcome of the test, and each leaf node represents a class label or a continuous value in the case of regression.
Decision trees are intuitive and easy to visualize, making them a popular choice for data scientists and analysts. They mimic human decision-making processes and can be used to explain complex decision logic in a straightforward manner. This transparency is one of the main reasons why decision trees are favored in applications where interpretability is crucial.
How Does a Decision Tree Work?
Decision trees work by recursively splitting the data into subsets that maximize the separation of classes or the homogeneity of the resulting groups. The algorithm begins at the root node and evaluates different splits based on a chosen criterion, such as Gini impurity or information gain for classification tasks, and mean squared error for regression tasks.
At each step, the algorithm selects the feature and threshold that result in the best split. This process continues until the stopping criteria are met, such as a maximum tree depth or a minimum number of samples per leaf. Once the tree is built, it can be used to make predictions by traversing from the root to a leaf node, following the decisions at each node based on the input features.

Here’s a simple example using scikit-learn to build a decision tree classifier:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn import tree
import matplotlib.pyplot as plt
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Visualize the decision tree
plt.figure(figsize=(10, 8))
tree.plot_tree(clf, filled=True, feature_names=iris.feature_names, class_names=iris.target_names)
plt.show()
This code demonstrates how to create and visualize a decision tree classifier using scikit-learn.
Advantages of Decision Trees
Decision trees offer several advantages, making them a popular choice for various machine learning tasks. Firstly, they are easy to understand and interpret, as the tree structure visually represents the decision-making process. This transparency allows stakeholders to understand how decisions are made, which is particularly valuable in regulated industries.
Secondly, decision trees can handle both numerical and categorical data, making them versatile and applicable to a wide range of problems. They do not require feature scaling or normalization, simplifying the preprocessing steps. Additionally, decision trees can capture nonlinear relationships between features, which many linear models fail to do.

Thirdly, decision trees are robust to outliers and can handle missing values, providing flexibility in dealing with real-world data. They can also be used as building blocks for more complex ensemble methods, such as random forests and gradient boosting, which further enhance their predictive power and robustness.
Disadvantages of Decision Trees
Despite their advantages, decision trees also have several limitations. One of the main drawbacks is their tendency to overfit the training data, especially when the tree is deep and complex. Overfitting occurs when the model captures noise in the data rather than the underlying pattern, resulting in poor generalization to new data.
To mitigate overfitting, techniques such as pruning, setting a maximum tree depth, or requiring a minimum number of samples per leaf can be employed. However, these techniques may sometimes lead to underfitting, where the model is too simple to capture the underlying relationships in the data.
Another limitation is the instability of decision trees. Small changes in the data can result in significantly different tree structures, making them sensitive to variations in the dataset. This instability can be addressed by using ensemble methods, which combine multiple decision trees to produce a more stable and accurate model.

Learn How Decision Trees Are Constructed
Key Steps in Constructing Decision Trees
Constructing a decision tree involves several key steps. The process begins with selecting the best attribute to split the data at the root node. This selection is based on a splitting criterion, such as information gain, Gini impurity, or variance reduction. The goal is to find the attribute that maximizes the separation of the data into distinct classes or reduces the variance in the case of regression.
Once the best attribute is selected, the data is split into subsets based on the values of that attribute. This process is repeated recursively for each subset, creating branches and nodes until a stopping criterion is met. Common stopping criteria include reaching a maximum tree depth, having a minimum number of samples per leaf, or achieving a minimum impurity decrease.
The final step is to assign a class label or a continuous value to each leaf node based on the majority class or the average value of the samples in that node. The resulting tree structure can then be used to make predictions by traversing from the root to a leaf node, following the decisions at each internal node.
Advantages and Disadvantages
Advantages of constructing decision trees include their interpretability and simplicity. Decision trees can be visualized, making it easy to understand the decision-making process and identify important features. They are also versatile, capable of handling both classification and regression tasks, and can work with both numerical and categorical data.

However, decision trees also have disadvantages. They are prone to overfitting, especially when the tree becomes too complex. This can result in poor generalization to new data. To address this issue, techniques such as pruning, setting a maximum depth, and requiring a minimum number of samples per leaf are used.
Additionally, decision trees can be sensitive to small changes in the data. Slight variations in the training set can lead to significantly different tree structures. This instability can be mitigated by using ensemble methods like random forests and boosting, which combine multiple decision trees to improve accuracy and stability.
Use Decision Trees for Classification
Decision Trees for Classification
Decision trees are widely used for classification tasks due to their simplicity and interpretability. In classification, the goal is to assign a class label to an input sample based on its features. Decision trees achieve this by splitting the data at each node based on the values of the features, creating branches that lead to different class labels at the leaf nodes.
The tree structure allows for clear visualization of the decision-making process, making it easy to understand how the model arrives at its predictions. This transparency is particularly valuable in applications where interpretability is crucial, such as healthcare, finance, and regulatory environments.

Here’s an example of using a decision tree for classification with scikit-learn:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn import tree
import matplotlib.pyplot as plt
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Visualize the decision tree
plt.figure(figsize=(10, 8))
tree.plot_tree(clf, filled=True, feature_names=iris.feature_names, class_names=iris.target_names)
plt.show()
This code demonstrates how to create and visualize a decision tree classifier using scikit-learn.
How Decision Trees Work for Classification
Decision trees work for classification by recursively partitioning the data based on feature values. At each internal node, the algorithm selects the feature and threshold that best separates the classes. This selection is based on criteria such as Gini impurity, information gain, or entropy.
Once the best split is identified, the data is divided into subsets, and the process is repeated for each subset. This recursive splitting continues until a stopping criterion is met, such as reaching a maximum tree depth or having a minimum number of samples per leaf. The leaf nodes are then assigned class labels based on the majority class of the samples within them.

To make a prediction, the model starts at the root node and traverses the tree based on the input features, following the decisions at each node until it reaches a leaf node. The class label of the leaf node is then assigned as the prediction.
Advantages of Decision Trees for Classification
Decision trees offer several advantages for classification tasks. They are easy to understand and interpret, as the tree structure provides a clear visual representation of the decision-making process. This interpretability makes decision trees valuable in applications where transparency is important.
Additionally, decision trees can handle both numerical and categorical data, making them versatile for various classification problems. They do not require feature scaling or normalization, simplifying the preprocessing steps. Decision trees can also capture nonlinear relationships between features, which many linear models fail to do.
Furthermore, decision trees are robust to outliers and can handle missing values, providing flexibility in dealing with real-world data. They can also be used as building blocks for more complex ensemble methods, such as random forests and gradient boosting, which further enhance their predictive power and robustness.
Limitations of Decision Trees
Despite their advantages, decision trees have some limitations. One major drawback is their tendency to overfit the training data, especially when the tree is deep and complex. Overfitting occurs when the model captures noise in the data rather than the underlying patterns, resulting in poor generalization to new data.
To mitigate overfitting, techniques such as pruning, setting a maximum tree depth, or requiring a minimum number of samples per leaf can be employed. However, these techniques may sometimes lead to underfitting, where the model is too simple to capture the underlying relationships in the data.
Another limitation is the instability of decision trees. Small changes in the data can result in significantly different tree structures, making them sensitive to variations in the dataset. This instability can be addressed by using ensemble methods,
which combine multiple decision trees to produce a more stable and accurate model.
Use Decision Trees for Regression
Decision Trees for Regression
Decision trees can also be used for regression tasks, where the goal is to predict a continuous value rather than a class label. In regression, the decision tree algorithm splits the data at each node based on feature values, creating branches that lead to different numerical outcomes at the leaf nodes.
The tree structure for regression is similar to that of classification, with the main difference being the criteria used for splitting the data. Instead of maximizing class separation, the algorithm aims to minimize the variance or the mean squared error within the subsets created by each split. This approach ensures that the resulting groups are as homogeneous as possible in terms of the target variable.
Here’s an example of using a decision tree for regression with scikit-learn:
from sklearn.datasets import load_boston
from sklearn.tree import DecisionTreeRegressor
from sklearn import tree
import matplotlib.pyplot as plt
# Load dataset
boston = load_boston()
X, y = boston.data, boston.target
# Create and train the decision tree regressor
regressor = DecisionTreeRegressor()
regressor.fit(X, y)
# Visualize the decision tree
plt.figure(figsize=(12, 8))
tree.plot_tree(regressor, filled=True, feature_names=boston.feature_names)
plt.show()
This code demonstrates how to create and visualize a decision tree regressor using scikit-learn.
Splitting Criterion for Regression
Splitting criterion in decision trees for regression is typically based on minimizing the variance or mean squared error within the subsets created by each split. The goal is to create homogeneous groups in terms of the target variable, ensuring that the predicted values at the leaf nodes are as accurate as possible.
At each step, the algorithm evaluates different splits and selects the one that results in the lowest variance or mean squared error. This process is repeated recursively for each subset until a stopping criterion is met, such as reaching a maximum tree depth or having a minimum number of samples per leaf.
The resulting tree structure can then be used to make predictions by traversing from the root to a leaf node, following the decisions at each internal node based on the input features. The predicted value is the mean of the target values in the leaf node.
Predictions at Leaf Nodes
Predictions at leaf nodes in decision trees for regression are typically the mean or median of the target values within that node. This approach ensures that the predicted value is representative of the target variable for the subset of data falling into that leaf node.
To make a prediction for a new input sample, the model starts at the root node and traverses the tree based on the feature values, following the decisions at each node until it reaches a leaf node. The value of the leaf node is then assigned as the prediction.
This method provides an intuitive and straightforward way to predict continuous values, making decision trees a valuable tool for regression tasks. However, like with classification, it is important to manage the tree’s complexity to avoid overfitting and ensure good generalization to new data.
Implement Decision Trees in ML Libraries
scikit-learn
scikit-learn is a popular machine learning library in Python that provides a simple and efficient implementation of decision trees for both classification and regression tasks. It offers various parameters to control the tree’s complexity, such as max_depth
, min_samples_split
, and min_samples_leaf
, allowing users to fine-tune the model to their specific needs.
Using scikit-learn, developers can easily create, train, and visualize decision trees with just a few lines of code. The library also supports ensemble methods like random forests and gradient boosting, which combine multiple decision trees to improve accuracy and robustness.
Here’s an example of creating a decision tree classifier with scikit-learn:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn import tree
import matplotlib.pyplot as plt
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Visualize the decision tree
plt.figure(figsize=(10, 8))
tree.plot_tree(clf, filled=True, feature_names=iris.feature_names, class_names=iris.target_names)
plt.show()
This code demonstrates how to create and visualize a decision tree classifier using scikit-learn.
TensorFlow
TensorFlow, primarily known for deep learning, also provides support for decision trees through its tf.estimator
API. This API offers pre-built estimators for various machine learning tasks, including classification and regression using decision trees.
TensorFlow’s implementation of decision trees integrates seamlessly with the rest of the TensorFlow ecosystem, allowing users to leverage the same tools and workflows for building, training, and deploying their models. This makes it a convenient option for developers already familiar with TensorFlow.
Here’s an example of creating a decision tree regressor with TensorFlow:
import tensorflow as tf
import pandas as pd
# Load dataset
data = pd.read_csv('https://storage.googleapis.com/tf-datasets/titanic/train.csv')
data = data[['age', 'fare', 'survived']].dropna()
# Feature columns
feature_columns = [tf.feature_column.numeric_column('age'), tf.feature_column.numeric_column('fare')]
# Create and train the decision tree regressor
regressor = tf.estimator.BoostedTreesRegressor(feature_columns, n_batches_per_layer=1)
regressor.train(lambda: tf.data.Dataset.from_tensor_slices((data[['age', 'fare']], data['survived'])).batch(32))
# Predict
predictions = regressor.predict(lambda: tf.data.Dataset.from_tensor_slices(data[['age', 'fare']]).batch(32))
print(list(predictions))
This code demonstrates how to create and train a decision tree regressor using TensorFlow’s tf.estimator
API.
Handle Missing Data in Decision Trees
Ignore Missing Data
Ignoring missing data is one approach to handling missing values in decision trees. This method involves removing any samples with missing values from the dataset before training the model. While simple, this approach can lead to significant data loss if many samples have missing values, potentially reducing the model’s accuracy and robustness.
In some cases, ignoring missing data might be acceptable if the proportion of missing values is small. However, for datasets with substantial missing values, more sophisticated techniques are recommended to preserve as much data as possible and maintain model performance.
Replace Missing Values with Common Values
Replacing missing values with the most common value (mode) or the mean/median is another common approach. For categorical features, the mode is often used, while for numerical features, the mean or median is more appropriate. This method is straightforward and preserves the dataset's size, but it can introduce bias if the imputed values do not accurately represent the missing data.
Here’s an example of imputing missing values using scikit-learn:
import numpy as np
from sklearn.impute import SimpleImputer
# Sample data with missing values
data = np.array([[1, 2], [3, np.nan], [7, 6], [np.nan, 5]])
# Impute missing values with the mean
imputer = SimpleImputer(strategy='mean')
imputed_data = imputer.fit_transform(data)
print(imputed_data)
This code demonstrates how to replace missing values with the mean using scikit-learn’s SimpleImputer
.
Advanced Imputation Techniques
Advanced imputation techniques involve using models to predict the missing values based on other features in the dataset. Methods such as k-nearest neighbors (KNN) imputation and multiple imputation can provide more accurate estimates of missing values compared to simple imputation methods.
KNN imputation, for instance, uses the k-nearest neighbors of a sample with missing values to estimate the missing values based on the mean or median of the neighbors. Multiple imputation generates multiple datasets with different imputed values and combines the results to account for the uncertainty of the imputations.
Here’s an example of using KNN imputation with scikit-learn:
from sklearn.impute import KNNImputer
# Sample data with missing values
data = np.array([[1, 2], [3, np.nan], [7, 6], [np.nan, 5]])
# Impute missing values using KNN
imputer = KNNImputer(n_neighbors=2)
imputed_data = imputer.fit_transform(data)
print(imputed_data)
This code demonstrates how to use KNN imputation to handle missing values.
Prune Decision Trees to Avoid Overfitting
Pre-Pruning
Pre-pruning involves stopping the growth of the decision tree early to prevent it from becoming too complex and overfitting the training data. Techniques for pre-pruning include setting a maximum tree depth, requiring a minimum number of samples per leaf, or requiring a minimum number of samples for a split.
These constraints prevent the tree from growing too deep and capturing noise in the data. While pre-pruning can help reduce overfitting, it can also lead to underfitting if the constraints are too restrictive, resulting in a model that is too simple to capture the underlying patterns in the data.
Post-Pruning
Post-pruning involves first growing a full decision tree and then removing branches that do not provide significant predictive power. This is done by evaluating the performance of the tree on a validation set and removing branches that lead to overfitting. Post-pruning techniques include cost complexity pruning and reduced error pruning.
Cost complexity pruning, for instance, removes branches to minimize a cost function that balances the tree's complexity and its accuracy on the validation set. Reduced error pruning involves evaluating the error rate of the tree on a validation set and removing branches that increase the error rate.
Here’s an example of post-pruning using scikit-learn:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.tree import plot_tree
import matplotlib.pyplot as plt
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Perform post-pruning
path = clf.cost_complexity_pruning_path(X, y)
ccp_alphas, impurities = path.ccp_alphas, path.impurities
# Train trees with different alpha values
clfs = [DecisionTreeClassifier(ccp_alpha=alpha).fit(X, y) for alpha in ccp_alphas]
# Plot the resulting trees
fig, ax = plt.subplots(len(clfs), 1, figsize=(10, 40))
for i, clf in enumerate(clfs):
plot_tree(clf, ax=ax[i], filled=True)
ax[i].set_title(f'Tree with ccp_alpha={ccp_alphas[i]}')
plt.show()
This code demonstrates how to perform post-pruning using cost complexity pruning with scikit-learn.
Interpret the Results of Decision Trees
Feature Importance
Feature importance is a measure of how much each feature contributes to the decision-making process in a decision tree. It indicates the importance of each feature in predicting the target variable, providing insights into the most influential factors in the dataset.
In scikit-learn, feature importance can be accessed through the feature_importances_
attribute of the trained decision tree model. This information can be used to understand which features are driving the model's predictions and to make informed decisions about feature selection and engineering.
Here’s an example of extracting feature importance using scikit-learn:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
import matplotlib.pyplot as plt
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Extract and plot feature importance
importance = clf.feature_importances_
plt.bar(iris.feature_names, importance)
plt.xlabel('Feature')
plt.ylabel('Importance')
plt.title('Feature Importance')
plt.show()
This code demonstrates how to extract and visualize feature importance using scikit-learn.
Path Traversal
Path traversal involves tracing the path from the root to a leaf node for a given input sample. This path shows the decisions made at each node, providing a clear explanation of how the model arrived at its prediction. Path traversal helps interpret the model's decisions and understand the influence of different features.
By examining the path, users can gain insights into the decision-making process and identify potential issues or biases in the model. This interpretability is particularly valuable in applications where understanding the rationale behind predictions is essential.
Rule Extraction
Rule extraction refers to converting the decisions made by the decision tree into a set of if-then rules. Each path from the root to a leaf node represents a rule, where the conditions at each node form the if-part and the prediction at the leaf node forms the then-part.
These rules provide a transparent and interpretable representation of the model's logic, making it easy to understand and communicate the decision-making process. Rule extraction helps identify the most critical conditions that lead to specific predictions, providing valuable insights into the model's behavior.
Visualization
Visualization is a powerful tool for interpreting decision trees. Visualizing the tree structure provides a clear and intuitive representation of the decision-making process, making it easy to understand how the model arrives at its predictions.
Tools like scikit-learn’s plot_tree
function or specialized libraries like Graphviz can be used to visualize decision trees. These visualizations help identify important features, understand the tree's complexity, and communicate the model's logic to stakeholders.
Here’s an example of visualizing a decision tree using Graphviz:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier, export_graphviz
import graphviz
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Export the tree to Graphviz format
dot_data = export_graphviz(clf, out_file=None,
feature_names=iris.feature_names,
class_names=iris.target_names,
filled=True, rounded=True,
special_characters=True)
graph = graphviz.Source(dot_data)
graph.render("iris")
This code demonstrates how to export and visualize a decision tree using Graphviz.
Combine Decision Trees with Other Algorithms
Combining decision trees with other machine learning algorithms can enhance their performance and address their limitations. Ensemble methods like random forests and gradient boosting combine multiple decision trees to improve accuracy, robustness, and stability.
Random forests create multiple decision trees using different subsets of the data and features, and then aggregate their predictions. This approach reduces overfitting and increases generalization. Gradient boosting sequentially builds decision trees, where each tree corrects the errors of the previous ones, leading to a strong predictive model.
Combining decision trees with other algorithms can also involve integrating them into more complex models, such as stacking or using them as base learners in meta-learning frameworks. These approaches leverage the strengths of decision trees while mitigating their weaknesses, resulting in more accurate and robust models.
Use Decision Trees for Feature Selection
Feature Selection
Using decision trees for feature selection involves leveraging the feature importance scores to identify the most influential features in the dataset. By focusing on these important features, developers can reduce the dimensionality of the data, improve model performance, and enhance interpretability.
Feature selection helps eliminate irrelevant or redundant features that do not contribute significantly to the model's predictions. This simplification can lead to faster training times, reduced computational costs, and improved generalization by preventing overfitting.
Here’s an example of using feature importance from a decision tree for feature selection:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
import numpy as np
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X, y)
# Extract feature importance
importance = clf.feature_importances_
# Select features with importance above a threshold
threshold = 0.1
selected_features = np.where(importance > threshold)[0]
# Create a new dataset with selected features
X_selected = X[:, selected_features]
print("Selected features:", iris.feature_names[selected_features])
This code demonstrates how to use feature importance for feature selection.
Advantages of Feature Selection
Advantages of using decision trees for feature selection include their ability to handle both numerical and categorical data, their interpretability, and their capacity to capture nonlinear relationships. By identifying the most important features, decision trees can help simplify the model, improve performance, and provide insights into the data.
Feature selection can also enhance the robustness of the model by reducing the risk of overfitting. By focusing on the most relevant features, the model can generalize better to new data, leading to more accurate and reliable predictions.
Decision trees are a powerful and versatile machine learning algorithm with numerous applications in both classification and regression tasks. By understanding their basic concepts, construction, and implementation, developers can leverage their strengths to build accurate and interpretable models. Despite their limitations, decision trees remain a crucial tool in the machine learning toolkit, especially when combined with other algorithms and used for feature selection.
If you want to read more articles similar to Decoding Decision Trees: A Crucial Machine Learning Algorithm, you can visit the Artificial Intelligence category.
You Must Read