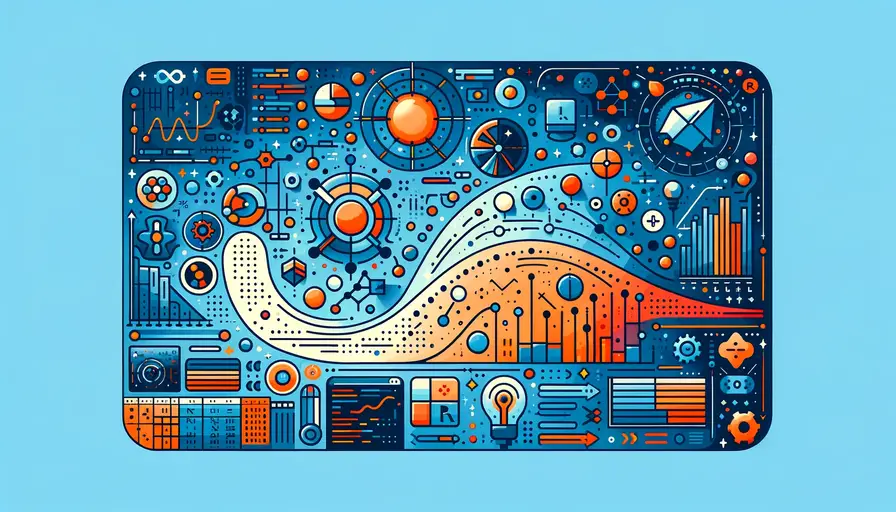
Deploying a Machine Learning Model as an API

Importance of Model Deployment in Machine Learning
Bridging the Gap Between Development and Production
Deploying a machine learning model as an API is a crucial step in bringing the power of data science from the development environment to real-world applications. While building and training models is a significant part of the machine learning pipeline, deployment ensures that the models can be accessed and utilized in production systems. This transition from development to production allows organizations to harness the predictive capabilities of machine learning models to make data-driven decisions and automate processes.
Creating an API for your machine learning model allows different applications and services to communicate with it over the web. This means that the model can be used by various clients, regardless of the programming language they are written in, facilitating seamless integration into existing systems. Moreover, deploying models as APIs simplifies the process of updating and maintaining models, ensuring that the most accurate and up-to-date predictions are available to users.
Using tools and platforms such as Google Cloud, AWS, and Azure can significantly streamline the deployment process. These platforms offer managed services that handle the infrastructure and scalability aspects, allowing data scientists to focus on model development and optimization. By leveraging these cloud services, organizations can deploy machine learning models quickly and efficiently, ensuring high availability and performance.
Enhancing Accessibility and Scalability
One of the primary advantages of deploying a machine learning model as an API is the enhanced accessibility it provides. APIs allow multiple users and applications to access the model's functionality over the web, making it easy to integrate machine learning capabilities into various workflows. This accessibility is particularly beneficial for large organizations with multiple teams that need to use the model for different purposes, such as marketing, finance, and operations.

Scalability is another critical aspect of model deployment. As the demand for predictions grows, the deployment infrastructure must be able to handle increasing loads without compromising performance. Cloud platforms provide auto-scaling capabilities that dynamically adjust the number of resources allocated to the API based on demand. This ensures that the model remains responsive and performant, even during peak usage times.
By deploying models as APIs, organizations can also take advantage of load balancing and fault tolerance features offered by cloud providers. These features distribute incoming requests across multiple instances of the API, ensuring that no single instance becomes a bottleneck. Additionally, automatic failover mechanisms help maintain service continuity in case of hardware failures or other issues, enhancing the reliability of the deployed model.
Example: Deploying a Simple Flask API for a Machine Learning Model
from flask import Flask, request, jsonify
import joblib
# Load the trained model
model = joblib.load('model.pkl')
# Initialize Flask app
app = Flask(__name__)
# Define a prediction route
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
prediction = model.predict([data['features']])
return jsonify({'prediction': prediction.tolist()})
# Run the app
if __name__ == '__main__':
app.run(debug=True)
In this example, a Flask application is created to serve a machine learning model. The model is loaded using joblib, and a /predict
route is defined to handle prediction requests. The Flask app listens for incoming requests and returns predictions in JSON format, making it easy to integrate into other applications.
Preparing the Machine Learning Model for Deployment
Model Training and Serialization
Before deploying a machine learning model as an API, it is essential to ensure that the model is properly trained and serialized. Training involves using historical data to build a model that can make accurate predictions on new data. This process typically includes data preprocessing, feature engineering, model selection, and hyperparameter tuning.

Once the model is trained, it must be serialized (or saved) so that it can be loaded and used by the deployment infrastructure. Common serialization formats include joblib and pickle in Python, which allow models to be saved to disk and loaded later without retraining. Serialization ensures that the model's state, including its learned parameters and hyperparameters, is preserved.
It is also important to validate the model's performance on a separate test dataset to ensure that it generalizes well to new data. Validation metrics such as accuracy, precision, recall, and F1-score provide insights into the model's effectiveness. If the model's performance is satisfactory, it can be serialized and prepared for deployment.
Example: Training and Serializing a Model in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
import joblib
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Evaluate model performance
accuracy = model.score(X_test, y_test)
print(f'Accuracy: {accuracy}')
# Serialize the model
joblib.dump(model, 'model.pkl')
In this example, a Random Forest classifier is trained on a dataset and its performance is evaluated. The trained model is then serialized using joblib, allowing it to be loaded and used in the deployment phase.
Environment Setup and Dependencies
Setting up the deployment environment involves installing the necessary software and dependencies required to run the machine learning model and the API. This typically includes the programming language runtime (e.g., Python), web framework (e.g., Flask, Django), and any libraries used by the model (e.g., scikit-learn, TensorFlow).

Using virtual environments can help manage dependencies and ensure that the deployment environment matches the development environment. Tools like virtualenv and conda allow you to create isolated environments with specific versions of libraries and dependencies. This isolation helps prevent conflicts between different projects and ensures that the model runs smoothly in production.
Additionally, creating a requirements file (e.g., requirements.txt
in Python) lists all the dependencies required by the project. This file can be used to install the necessary packages in the deployment environment, ensuring consistency and reproducibility.
Example: Setting Up a Virtual Environment and Installing Dependencies
# Create a virtual environment
python -m venv venv
# Activate the virtual environment
source venv/bin/activate # On Windows, use `venv\Scripts\activate`
# Install dependencies
pip install flask joblib scikit-learn pandas
# Freeze dependencies to a requirements file
pip freeze > requirements.txt
In this example, a virtual environment is created and activated, and the necessary dependencies are installed. The dependencies are then listed in a requirements file, which can be used to set up the deployment environment.
Creating and Testing the API
Designing the API Endpoints
Designing API endpoints involves defining the routes and methods that the API will expose to users. Each endpoint corresponds to a specific functionality of the API, such as making predictions, updating models, or retrieving model information. Properly designing these endpoints ensures that the API is intuitive and easy to use.

Common HTTP methods used in APIs include GET, POST, PUT, and DELETE. For a machine learning API, the POST method is typically used for prediction endpoints, as it allows users to send data in the request body. GET methods can be used to retrieve information about the model, such as its version or performance metrics.
It is also important to consider input validation and error handling when designing API endpoints. Input validation ensures that the data sent to the API is in the correct format and meets the required criteria. Error handling provides informative responses to users when something goes wrong, such as invalid input or internal server errors.
Example: Designing API Endpoints in Flask
from flask import Flask, request, jsonify
import joblib
# Load the trained model
model = joblib.load('model.pkl')
# Initialize Flask app
app = Flask(__name__)
# Define a prediction route
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
if 'features' not in data:
return jsonify({'error': 'Invalid input'}), 400
prediction = model.predict([data['features']])
return jsonify({'prediction': prediction.tolist()})
# Define a model info route
@app.route('/model-info', methods=['GET'])
def model_info():
return jsonify({'model': 'Random Forest', 'version': '1.0'})
# Run the app
if __name__ == '__main__':
app.run(debug=True)
In this example, a Flask application is created with two endpoints: /predict
for making predictions and /model-info
for retrieving information about the model. Input validation is implemented to ensure that the prediction endpoint receives the correct data format.
Testing the API Locally
Testing the API locally is a crucial step to ensure that it functions as expected before deploying it to a production environment. Local testing involves sending requests to the API endpoints and verifying the responses. Tools like Postman and cURL can be used to send HTTP requests and inspect the results.

Unit tests can also be written to automate the testing process and ensure that the API handles different scenarios correctly. Testing frameworks like pytest in Python allow you to define test cases and check the API's behavior under various conditions, such as valid input, invalid input, and error handling.
It is important to test the API for performance and scalability as well. Simulating high traffic scenarios and measuring response times can help identify potential bottlenecks and ensure that the API can handle the expected load.
Example: Testing the API with Postman
- Open Postman and create a new request.
- Set the request method to POST and the URL to
http://localhost:5000/predict
. - In the Body tab, select raw and set the content type to JSON.
- Enter the following JSON data:
{
"features": [0.1, 0.2, 0.3, 0.4, 0.5]
}
- Send the request and verify the response.
In this example, Postman is used to send a prediction request to the Flask API and verify the response. This process helps ensure that the API functions correctly before deployment.
Deploying the API to a Production Environment
Choosing a Hosting Platform
Choosing the right hosting platform is a critical decision when deploying a machine learning API. Several cloud providers offer managed services for deploying and scaling APIs, including Google Cloud, AWS, and Azure. These platforms provide various options for hosting APIs, such as serverless functions, containerized applications, and virtual machines.

Serverless functions, such as AWS Lambda and Google Cloud Functions, allow you to deploy APIs without managing the underlying infrastructure. These services automatically scale based on demand and charge only for the actual usage, making them a cost-effective option for many applications.
Containerized applications, using technologies like Docker and Kubernetes, offer greater control over the deployment environment. Containers package the application and its dependencies into a portable unit that can run consistently across different environments. Kubernetes provides orchestration and management of containerized applications, enabling seamless scaling and deployment.
Example: Deploying a Flask API on Heroku
# Install the Heroku CLI
curl https://cli-assets.heroku.com/install.sh | sh
# Log in to Heroku
heroku login
# Create a new Heroku app
heroku create my-flask-api
# Deploy the app
git init
heroku git:remote -a my-flask-api
git add .
git commit -m "Initial commit"
git push heroku master
# Scale the app
heroku ps:scale web=1
# Open the app in the browser
heroku open
In this example, a Flask API is deployed on Heroku, a platform-as-a-service (PaaS) provider. The Heroku CLI is used to create a new app, deploy the code, and scale the application.
Monitoring and Maintaining the API
Once the API is deployed, continuous monitoring and maintenance are essential to ensure its reliability and performance. Monitoring involves tracking various metrics, such as response times, error rates, and resource usage. Cloud providers offer monitoring services, such as AWS CloudWatch and Google Cloud Monitoring, that provide insights into the health and performance of the deployed API.
Maintenance includes updating the model with new data, retraining the model, and redeploying the updated model. Automated pipelines can streamline this process, ensuring that the API always uses the most accurate and up-to-date model. Continuous integration and continuous deployment (CI/CD) tools, such as Jenkins and GitHub Actions, can automate the deployment process and ensure that changes are tested and deployed seamlessly.
Additionally, implementing security measures, such as authentication and authorization, protects the API from unauthorized access and potential threats. Using tools like OAuth and JWT (JSON Web Tokens) can help secure the API and ensure that only authorized users can access its functionality.
Example: Setting Up Monitoring with AWS CloudWatch
- Log in to the AWS Management Console.
- Navigate to CloudWatch and create a new dashboard.
- Add widgets to monitor metrics such as CPU utilization, memory usage, and API request counts.
- Set up alarms to notify you of any anomalies or performance issues.
In this example, AWS CloudWatch is used to monitor the performance and health of the deployed API. Setting up alarms ensures that any issues are detected and addressed promptly.
Deploying a machine learning model as an API involves several critical steps, including model training and serialization, environment setup, API creation and testing, and deployment to a production environment. By following these steps and leveraging tools and platforms such as Flask, Postman, Heroku, and AWS, you can ensure a smooth and efficient deployment process. Continuous monitoring and maintenance are essential to keep the API running smoothly and provide accurate and reliable predictions to users. By deploying machine learning models as APIs, organizations can unlock the full potential of their data science efforts and integrate predictive capabilities into their applications and workflows.
If you want to read more articles similar to Deploying a Machine Learning Model as an API, you can visit the Applications category.
You Must Read