
Deploying Machine Learning Models as Web Services: Best Practices
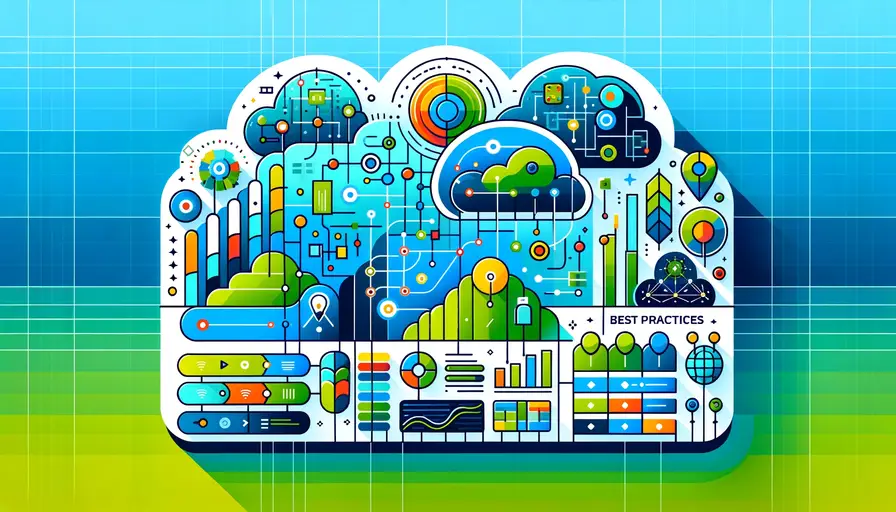
Understanding the Importance of Model Deployment
Enhancing Accessibility and Usability
Deploying machine learning models as web services enhances accessibility and usability by making the models available through APIs. This approach allows developers to integrate the models into various applications, such as mobile apps, web applications, and other software systems, without requiring in-depth knowledge of the underlying machine learning algorithms. By exposing the models as web services, they become reusable components that can be accessed by multiple applications simultaneously.
Web services provide a standardized way to interact with machine learning models, enabling seamless communication between different systems. This interoperability is crucial for businesses that rely on diverse technologies and platforms. By using web services, companies can leverage their existing infrastructure while incorporating advanced machine learning capabilities, leading to more efficient and scalable solutions.
Moreover, deploying models as web services allows for real-time predictions and decision-making. This capability is essential for applications that require immediate responses, such as fraud detection, recommendation systems, and predictive maintenance. By providing instant access to model predictions, businesses can enhance their operations and deliver better user experiences.
Ensuring Scalability and Performance
Scalability is a critical aspect of deploying machine learning models as web services. As the demand for predictions increases, the deployment infrastructure must handle the growing load without compromising performance. Using cloud-based solutions, such as AWS, Google Cloud, and Azure, provides the necessary scalability to accommodate varying workloads.
The Top Machine Learning Applications Transforming Smart CitiesThese cloud platforms offer managed services that automatically scale resources based on demand, ensuring that the deployed models remain responsive under high traffic conditions. Additionally, cloud providers offer load balancing and auto-scaling features that distribute the incoming requests across multiple instances, further enhancing the system's resilience and performance.
Performance optimization is also crucial for deploying machine learning models as web services. Techniques such as model quantization, pruning, and using specialized hardware like GPUs and TPUs can significantly reduce latency and improve throughput. By optimizing both the deployment infrastructure and the models themselves, businesses can achieve high-performance, scalable solutions that meet their operational requirements.
Example: Deploying a Model with Flask
from flask import Flask, request, jsonify
import numpy as np
import tensorflow as tf
# Load the pre-trained model
model = tf.keras.models.load_model('model.h5')
app = Flask(__name__)
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json(force=True)
input_data = np.array(data['input']).reshape((1, -1))
prediction = model.predict(input_data)
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
In this example, a Flask API is created to deploy a pre-trained TensorFlow model. The API receives input data via POST requests, processes the data, and returns predictions. This setup demonstrates how to expose a machine learning model as a web service, making it accessible for real-time predictions.
Best Practices for Model Deployment
Using Containerization Technologies
Containerization technologies like Docker provide a consistent environment for deploying machine learning models. Containers encapsulate the model, its dependencies, and the runtime environment, ensuring that the model runs consistently across different platforms. This consistency eliminates issues related to dependency conflicts and environment mismatches, making the deployment process more reliable.
Seeking Fresh Machine Learning Project Concepts for ExplorationDocker allows developers to create lightweight, portable containers that can be easily deployed on any system that supports Docker. By using Docker, teams can streamline the development, testing, and deployment workflows, ensuring that the model performs as expected in various environments. Additionally, Docker images can be versioned and stored in container registries, facilitating collaboration and version control.
Combining Docker with orchestration tools like Kubernetes enhances the scalability and manageability of machine learning deployments. Kubernetes automates the deployment, scaling, and management of containerized applications, ensuring that the system can handle varying workloads efficiently. This combination provides a robust and scalable solution for deploying machine learning models as web services.
Implementing Continuous Integration and Continuous Deployment (CI/CD)
Continuous Integration and Continuous Deployment (CI/CD) practices are essential for maintaining the quality and reliability of machine learning models in production. CI/CD pipelines automate the process of building, testing, and deploying models, ensuring that any changes are thoroughly validated before being released. This automation reduces the risk of errors and accelerates the deployment process.
Tools like Jenkins, GitHub Actions, and GitLab CI/CD provide robust frameworks for implementing CI/CD pipelines. These tools integrate with version control systems, enabling automatic triggers for build and deployment processes whenever changes are committed to the repository. By incorporating testing and validation steps, CI/CD pipelines ensure that only high-quality models are deployed to production.
Top Websites for Machine Learning Project IdeasIn addition to automating the deployment process, CI/CD practices enable continuous monitoring and feedback. By tracking key performance metrics and monitoring system logs, teams can quickly identify and address any issues that arise in production. This proactive approach helps maintain the reliability and performance of the deployed models, ensuring a seamless user experience.
Example: Dockerizing a Flask Application
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container
COPY . /app
# Install the dependencies
RUN pip install --no-cache-dir -r requirements.txt
# Make port 5000 available to the world outside this container
EXPOSE 5000
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
In this example, a Dockerfile is created to containerize a Flask application. The Dockerfile specifies the base image, sets the working directory, copies the application code, installs dependencies, exposes the necessary port, and defines the command to run the application. This setup ensures that the Flask application can be consistently deployed across different environments.
Ensuring Security and Compliance
Security is a critical aspect of deploying machine learning models as web services. Implementing robust authentication and authorization mechanisms ensures that only authorized users can access the model's predictions. This protection is essential for safeguarding sensitive data and preventing unauthorized access to the system.
Authentication verifies the identity of users, typically through mechanisms like API keys, OAuth tokens, or JWT tokens. Authorization determines the level of access granted to authenticated users, ensuring that they can only perform actions they are permitted to. By combining these mechanisms, businesses can enforce strict access controls and protect their deployed models.
Machine Learning Projects with Recommendation EnginesFrameworks like OAuth 2.0 and tools like Auth0 provide comprehensive solutions for implementing authentication and authorization in web services. These tools offer features like user management, role-based access control, and integration with various identity providers, simplifying the process of securing machine learning deployments.
Ensuring Data Privacy and Compliance
Deploying machine learning models often involves handling sensitive data, making data privacy and compliance critical considerations. Compliance with regulations like GDPR, CCPA, and HIPAA ensures that data is handled responsibly and legally. These regulations impose strict requirements on data collection, storage, processing, and sharing, necessitating robust data protection measures.
Data anonymization and encryption are essential techniques for ensuring data privacy. Anonymization removes personally identifiable information (PII) from the data, making it difficult to trace back to individual users. Encryption protects data in transit and at rest, preventing unauthorized access. By implementing these techniques, businesses can safeguard sensitive data and comply with regulatory requirements.
Regular audits and assessments are necessary to ensure ongoing compliance with data privacy regulations. These audits evaluate the effectiveness of data protection measures and identify any areas for improvement. By maintaining a strong focus on data privacy and compliance, businesses can build trust with their users and avoid legal repercussions.
ChatGPT: A Cutting-Edge Machine Learning Model for Chatbot DevelopmentExample: Implementing JWT Authentication in Flask
from flask import Flask, request, jsonify
import jwt
import datetime
from functools import wraps
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key'
def token_required(f):
@wraps(f)
def decorated(*args, **kwargs):
token = request.headers.get('x-access-tokens')
if not token:
return jsonify({'message': 'Token is missing!'}), 403
try:
data = jwt.decode(token, app.config['SECRET_KEY'], algorithms=["HS256"])
except:
return jsonify({'message': 'Token is invalid!'}), 403
return f(*args, **kwargs)
return decorated
@app.route('/login', methods=['POST'])
def login():
auth_data = request.get_json()
if auth_data and auth_data['username'] == 'user' and auth_data['password'] == 'pass':
token = jwt.encode({'user': auth_data['username'], 'exp': datetime.datetime.utcnow() + datetime.timedelta(minutes=30)}, app.config['SECRET_KEY'], algorithm="HS256")
return jsonify({'token': token})
return jsonify({'message': 'Invalid credentials'}), 401
@app.route('/predict', methods=['POST'])
@token_required
def predict():
data = request.get_json()
# Your prediction logic here
return jsonify({'prediction': 'result'})
if __name__ == '__main__':
app.run(debug=True)
In this example, JWT authentication is implemented in a Flask application to secure the /predict
endpoint. The login
endpoint generates a JWT token for authenticated users, and the token_required
decorator enforces token validation for protected routes. This setup ensures that only authorized users can access the model's predictions.
Monitoring and Logging
Effective monitoring and logging are essential for maintaining the performance and reliability of deployed machine learning models. Monitoring involves tracking key performance metrics, such as response times, error rates, and resource usage, to ensure that the system operates within acceptable parameters. Logging captures detailed information about system events, providing valuable insights for debugging and performance optimization.
Tools like Prometheus and Grafana offer comprehensive solutions for monitoring machine learning deployments. Prometheus collects and stores metrics, while Grafana provides powerful visualization capabilities, enabling teams to create real-time dashboards and set up alerts for critical issues. By leveraging these tools, businesses can proactively monitor their systems and address any performance bottlenecks.
Logging frameworks like Logstash and Fluentd facilitate the collection, aggregation, and analysis of log data. These frameworks can integrate with various data sources and provide centralized logging solutions, making it easier to manage and analyze logs. By implementing robust monitoring and logging practices, businesses can ensure the reliability and performance of their machine learning deployments.
Optimize Mobile Apps with Machine Learning RecommendationsExample: Setting Up Monitoring with Prometheus and Grafana
# Prometheus configuration (prometheus.yml)
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'flask_app'
static_configs:
- targets: ['localhost:5000']
# Docker Compose file for Prometheus and Grafana (docker-compose.yml)
version: '3'
services:
prometheus:
image: prom/prometheus
volumes:
- ./prometheus.yml:/etc/prometheus/prometheus.yml
ports:
- "9090:9090"
grafana:
image: grafana/grafana
ports:
- "3000:3000"
environment:
- GF_SECURITY_ADMIN_PASSWORD=admin
In this example, Prometheus and Grafana are set up using Docker Compose to monitor a Flask application. Prometheus is configured to scrape metrics from the Flask app, and Grafana provides visualization and dashboard capabilities. This setup ensures that key performance metrics are tracked and visualized in real-time, facilitating proactive monitoring and performance management.
Future Trends in Model Deployment
Serverless Architectures
Serverless architectures are gaining popularity for deploying machine learning models due to their scalability, cost-efficiency, and ease of management. In a serverless setup, the cloud provider automatically manages the infrastructure, scaling resources up or down based on demand. This approach eliminates the need for manual provisioning and maintenance, allowing teams to focus on developing and deploying models.
Platforms like AWS Lambda, Google Cloud Functions, and Azure Functions provide serverless computing capabilities. These platforms can execute code in response to events, such as HTTP requests or changes in data, making them ideal for real-time machine learning predictions. By leveraging serverless architectures, businesses can achieve high scalability and cost-efficiency while simplifying the deployment process.
Edge Computing
Edge computing involves deploying machine learning models closer to the data source, such as on IoT devices or edge servers. This approach reduces latency and bandwidth usage, enabling real-time predictions and decision-making in environments with limited connectivity. Edge computing is particularly valuable for applications in healthcare, manufacturing, and autonomous systems, where immediate responses are critical.
Frameworks like TensorFlow Lite and ONNX Runtime support deploying machine learning models on edge devices. These frameworks provide optimized runtimes that can execute models efficiently on resource-constrained hardware. By leveraging edge computing, businesses can deploy models that deliver fast, reliable predictions in real-world environments.
Example: Deploying a Model with AWS Lambda
import json
import numpy as np
import boto3
import tensorflow as tf
# Load the pre-trained model
model = tf.keras.models.load_model('model.h5')
def lambda_handler(event, context):
input_data = np.array(json.loads(event['body'])['input']).reshape((1, -1))
prediction = model.predict(input_data)
return {
'statusCode': 200,
'body': json.dumps({'prediction': prediction.tolist()})
}
In this example, a TensorFlow model is deployed using AWS Lambda. The lambda_handler
function processes input data, makes predictions using the pre-trained model, and returns the results. This serverless setup demonstrates how to deploy a machine learning model that scales automatically based on demand.
MLOps and Automation
MLOps (Machine Learning Operations) is an emerging discipline that focuses on streamlining the deployment, monitoring, and management of machine learning models in production. MLOps combines principles from DevOps, data engineering, and machine learning to create automated, scalable, and reproducible workflows. By adopting MLOps practices, businesses can accelerate the deployment of machine learning models and ensure their reliability and performance.
Key components of MLOps include automated data pipelines, continuous integration/continuous deployment (CI/CD), model versioning, and monitoring. Tools like Kubeflow, MLflow, and TensorFlow Extended (TFX) provide comprehensive frameworks for implementing MLOps practices. These tools enable teams to build end-to-end machine learning workflows that are automated, scalable, and reproducible.
By adopting MLOps, businesses can improve collaboration between data scientists, engineers, and operations teams, leading to more efficient and reliable machine learning deployments. This approach ensures that models are continuously monitored, maintained, and updated, delivering consistent performance and value over time.
Deploying machine learning models as web services involves several best practices to ensure accessibility, scalability, performance, security, and compliance. By leveraging containerization technologies, implementing CI/CD pipelines, and adopting robust security measures, businesses can create reliable and scalable deployments. Future trends such as serverless architectures, edge computing, and MLOps promise to further enhance the deployment and management of machine learning models, enabling businesses to unlock their full potential.
If you want to read more articles similar to Deploying Machine Learning Models as Web Services: Best Practices, you can visit the Applications category.
You Must Read