
Detecting Fake News on X (Twitter) with Machine Learning Models
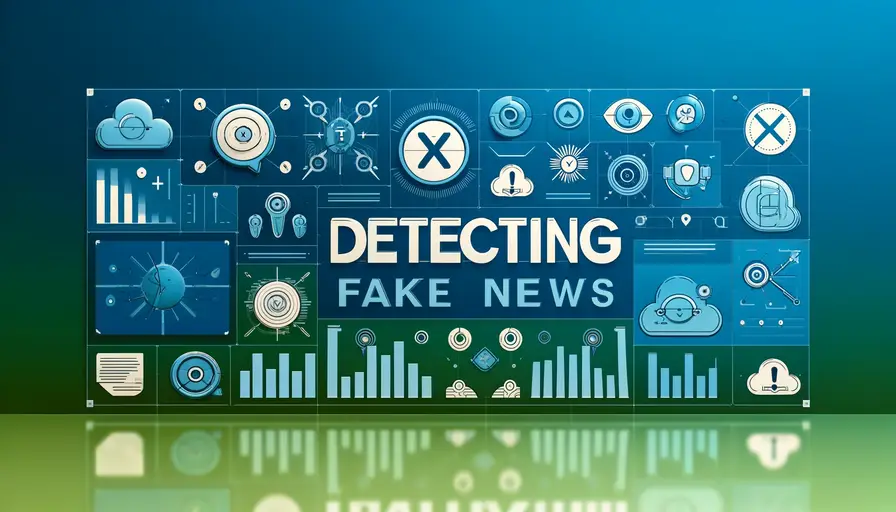
Content
Natural Language Processing Techniques
Tokenization
Tokenization is the process of breaking down text into smaller units called tokens, such as words or phrases. In the context of analyzing tweets, tokenization helps in understanding the structure and content of the text. By splitting tweets into tokens, machine learning models can analyze each component individually, identifying patterns and frequencies of words that may indicate fake news.
For example, a tokenized tweet might be analyzed for the presence of specific keywords or phrases commonly associated with misinformation. This process is crucial for preparing text data for further natural language processing (NLP) techniques and machine learning models.
Here’s an example of tokenizing a tweet using Python and the NLTK library:
import nltk
from nltk.tokenize import word_tokenize
tweet = "Breaking news! This is an example of a tweet."
tokens = word_tokenize(tweet)
print(tokens)
This code breaks down a tweet into individual words, making it easier to analyze.
Machine Learning for Accurate Home Electricity Load ForecastingStemming
Stemming is the process of reducing words to their base or root form. This technique helps in standardizing words that have similar meanings but different forms, such as "running" and "run." By reducing words to their stems, machine learning models can better understand the core content of tweets and identify patterns associated with fake news.
Stemming is particularly useful in handling variations in word forms, ensuring that the analysis focuses on the main concept rather than superficial differences. This technique can significantly improve the performance of NLP models by reducing the dimensionality of the text data.
Here’s an example of stemming using Python and the NLTK library:
from nltk.stem import PorterStemmer
ps = PorterStemmer()
words = ["running", "ran", "runner"]
stemmed_words = [ps.stem(word) for word in words]
print(stemmed_words)
This code reduces words to their stem forms, which helps in standardizing the text for analysis.
Synergistic IoT Projects: Enhancing Capabilities with Machine LearningSentiment Analysis
Sentiment analysis involves determining the emotional tone of a piece of text. By analyzing the sentiment of tweets, machine learning models can detect patterns that may indicate fake news. For example, fake news tweets might exhibit extreme sentiments or exaggerated language to provoke reactions from readers.
Sentiment analysis can be performed using various NLP techniques and pre-trained models. This process helps in understanding the underlying emotions in tweets, which can be a crucial factor in identifying misinformation.
Here’s an example of performing sentiment analysis using Python and the TextBlob library:
from textblob import TextBlob
tweet = "Breaking news! This is an example of a tweet."
analysis = TextBlob(tweet)
print(analysis.sentiment)
This code analyzes the sentiment of a tweet, providing insights into its emotional tone.
Machine Learning for Data Loss Prevention: Strategies and SolutionsDeveloping Machine Learning Models
Source Analysis
Source analysis involves examining the credibility and reputation of the sources of tweets. By analyzing the source, such as verified accounts or known news organizations, machine learning models can differentiate between reliable information and potential fake news. Features like the account's history, follower count, and previous tweet behavior are crucial in this analysis.
By incorporating source analysis, models can assign a credibility score to tweets, aiding in the classification of real versus fake news. This approach leverages metadata and social network analysis to enhance the accuracy of fake news detection.
Content Analysis
Content analysis focuses on the actual text of the tweets. This involves examining the language, keywords, and phrases used in the tweet. Machine learning models can identify patterns and anomalies in the content that are indicative of fake news, such as sensationalist language, clickbait phrases, or specific keywords that are frequently associated with misinformation.
By analyzing the content, machine learning models can detect subtle cues that may not be immediately apparent. This detailed examination of the tweet's text helps in building a robust classification system.
Detect and Prevent Phishing AttacksUser Behavior Analysis
User behavior analysis examines the actions and interactions of users on Twitter. Features such as retweet patterns, likes, and the types of accounts followed can provide insights into the likelihood of a tweet being fake. Users who frequently engage with dubious sources or exhibit unusual activity patterns may be more likely to spread fake news.
Analyzing user behavior can help in identifying suspicious accounts and tweets. By incorporating this information, machine learning models can better understand the context in which tweets are shared and classify them more accurately.
Model Training and Evaluation
Model training and evaluation are critical steps in developing effective machine learning models. Training involves feeding the model a large dataset of labeled tweets (real and fake) and allowing it to learn patterns and features associated with each class. Evaluation involves testing the model on a separate dataset to assess its accuracy and performance.
Here’s an example of training and evaluating a machine learning model using Scikit-learn:
Exploring Machine Learning Models for POC: A Comprehensive Guidefrom sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# Sample data
X = [...] # Feature set
y = [...] # Labels
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Predict and evaluate
y_pred = model.predict(X_test)
print(classification_report(y_test, y_pred))
This code demonstrates how to train and evaluate a machine learning model for classifying tweets.
Training Models with Large Datasets
Natural Language Processing Techniques
Applying natural language processing (NLP) techniques is essential for preparing text data for machine learning models. Techniques like tokenization, stemming, and sentiment analysis help in transforming raw tweets into structured data that can be used for model training.
By leveraging NLP, models can better understand the nuances of language used in tweets, enhancing their ability to detect patterns associated with fake news. These techniques are crucial for preprocessing and feature extraction in the model training process.
Evaluating Model Performance
Evaluating model performance involves assessing how well the machine learning models classify tweets as real or fake. Metrics such as accuracy, precision, recall, and F1-score provide insights into the model's effectiveness. Evaluating performance on a validation dataset helps in fine-tuning the model and improving its accuracy.
Guide to Named Entity Recognition in Machine LearningContinuous evaluation is necessary to ensure that the models remain effective as new types of fake news emerge. By regularly testing and updating the models, developers can maintain high levels of accuracy and reliability.
Implementing an Automated System
How It Works
Implementing an automated system for detecting fake news involves deploying trained machine learning models that can analyze tweets in real-time. The system uses features such as content, source, and user behavior to classify tweets and flag potentially fake ones.
This automated approach ensures that fake news can be identified and addressed quickly, reducing its spread. The system can be integrated with social media platforms to provide users with warnings about questionable content.
Benefits and Limitations
Benefits of an automated fake news detection system include increased efficiency in identifying misinformation, reduced human effort, and the ability to process large volumes of data quickly. However, there are also limitations, such as the potential for false positives, the need for continuous updates, and the challenge of keeping up with evolving tactics used by fake news creators.
Balancing these benefits and limitations is crucial for developing a reliable and effective system. Continuous improvement and user feedback can help in refining the system over time.
Continuous Model Updates
Need for Updates
Continuous updates are necessary to keep machine learning models effective against new types of fake news. As misinformation tactics evolve, models must be retrained with new data to recognize emerging patterns. Regular updates ensure that the models stay current and maintain high accuracy.
Improving Accuracy
Improving accuracy involves refining the models based on performance metrics and user feedback. By analyzing errors and adjusting model parameters, developers can enhance the system's ability to correctly classify tweets. Incorporating new data and refining feature extraction techniques are key strategies for improving accuracy.
Here’s an example of updating a machine learning model using new data:
# Assume we have new labeled data
new_X = [...] # New feature set
new_y = [...] # New labels
# Retrain the model with new data
model.fit(new_X, new_y)
# Evaluate the updated model
new_y_pred = model.predict(X_test)
print(classification_report(y_test, new_y_pred))
This code demonstrates how to update a model with new data to improve its performance.
Integrating with Platforms
Collaborating with social media platforms involves integrating machine learning models into their systems to provide real-time detection of fake news. By working together, platforms can leverage advanced algorithms to flag potentially fake content and warn users.
This collaboration ensures that fake news is addressed quickly and efficiently, reducing its impact on public opinion. Social media platforms play a crucial role in the dissemination of information, and their involvement is vital for effective fake news detection.
Empowering Users
Empowering users with information about potentially fake news helps them make informed decisions. By providing warnings and context about the credibility of tweets, users can critically evaluate the information they encounter. This approach fosters a more informed and discerning user base, reducing the spread of misinformation.
Educating users about the characteristics of fake news and how to spot it can further enhance their ability to navigate social media responsibly. This combined approach of technology and education is essential for combating fake news effectively.
Research and Experiments
Designing Experiments
Conducting research and experiments is crucial for evaluating the effectiveness of machine learning models in detecting fake news. Experiments involve testing different models, feature sets, and NLP techniques to determine the most effective approach. This process helps in identifying the strengths and weaknesses of various methods.
By systematically testing and refining models, researchers can develop more robust solutions for fake news detection. Experimental results provide valuable insights that guide the development of better models and algorithms.
Training and Testing
Training and testing models on different datasets help in evaluating their performance and generalizability. By using diverse datasets, researchers can ensure that the models are effective across various types of content and not overfitted to specific examples. This approach improves the reliability and accuracy of fake news detection.
Here’s an example of conducting experiments with different models:
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier, GradientBoostingClassifier
# Sample data
X = [...] # Feature set
y = [...] # Labels
# Define models
models = {
'Random Forest': RandomForestClassifier(n_estimators=100),
'Gradient Boosting': GradientBoostingClassifier(n_estimators=100)
}
# Evaluate models using cross-validation
for name, model in models.items():
scores = cross_val_score(model, X, y, cv=5)
print(f"{name}: {scores.mean()}")
This code demonstrates how to evaluate different models using cross-validation.
Educating Users
Characteristics of Fake News
Educating users about the characteristics of fake news helps them identify and avoid misinformation. Key characteristics include sensationalist language, lack of credible sources, and emotionally charged content. By understanding these traits, users can critically assess the credibility of the information they encounter on social media.
Critical Evaluation
Teaching users to critically evaluate information involves encouraging them to verify sources, check for supporting evidence, and consider the credibility of the author. Providing guidelines and tools for fact-checking can empower users to make informed decisions about the content they consume and share.
Educating users about these critical evaluation techniques is essential for fostering a more discerning and informed online community. By promoting media literacy, we can reduce the spread of fake news and its impact on society.
Detecting fake news on Twitter with machine learning involves leveraging NLP techniques, developing robust models, continuous updating, and collaboration with social media platforms. Educating users plays a crucial role in enhancing their ability to identify and avoid misinformation. By combining technology and education, we can create a more reliable and informed social media environment.
If you want to read more articles similar to Detecting Fake News on X (Twitter) with Machine Learning Models, you can visit the Applications category.
You Must Read