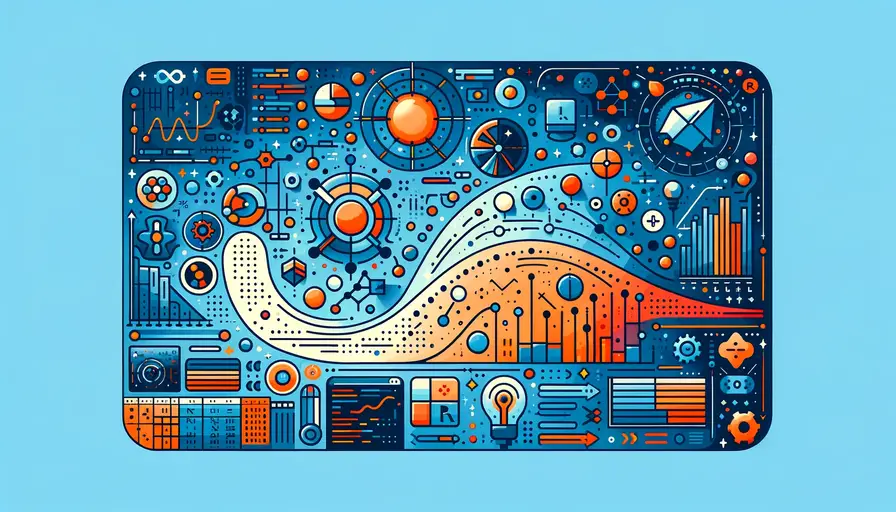
Enhancing Sports Betting with Machine Learning in Python
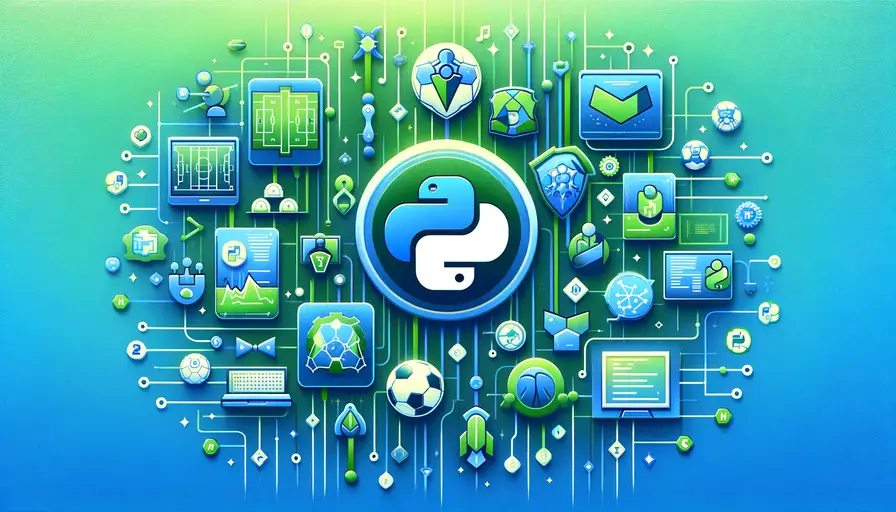
Sports betting is an industry driven by data and probabilities. Leveraging machine learning (ML) to analyze sports data can enhance betting strategies by providing insights and predictions that are more accurate than traditional methods. This article explores how machine learning can be used to enhance sports betting, focusing on various techniques, tools, and best practices. We will delve into data preparation, model building, and deployment using Python.
Data Preparation for Sports Betting
Gathering Sports Data
The first step in using machine learning for sports betting is to gather relevant data. Sports data can be obtained from various sources such as Kaggle, official sports websites, and APIs. This data includes historical match results, player statistics, team performance metrics, and more.
For instance, you can use the Kaggle API to download sports datasets:
import kaggle
# Download a dataset from Kaggle
kaggle.api.dataset_download_files('favorito/football-data', path='data/', unzip=True)
This code snippet shows how to download a football dataset from Kaggle, which can then be used for analysis and model training.

Cleaning and Transforming Data
Once you have gathered the data, the next step is to clean and transform it. This involves handling missing values, removing duplicates, and transforming data into a format suitable for machine learning algorithms. Pandas is a powerful library in Python that can be used for these tasks.
Here is an example of cleaning and transforming sports data using pandas:
import pandas as pd
# Load dataset
data = pd.read_csv('data/football.csv')
# Remove missing values
data = data.dropna()
# Convert date column to datetime
data['date'] = pd.to_datetime(data['date'])
# Encode categorical variables
data = pd.get_dummies(data, columns=['team', 'opponent'])
# Save the cleaned dataset
data.to_csv('data/cleaned_football.csv', index=False)
This code demonstrates how to clean a football dataset by removing missing values, converting date columns, and encoding categorical variables.
Feature Engineering for Sports Betting
Feature engineering is the process of creating new features from raw data to improve the performance of machine learning models. In sports betting, relevant features might include recent team performance, player injuries, weather conditions, and head-to-head statistics.

Here is an example of feature engineering in sports betting:
import pandas as pd
# Load cleaned dataset
data = pd.read_csv('data/cleaned_football.csv')
# Create new features
data['goal_difference'] = data['home_goals'] - data['away_goals']
data['total_goals'] = data['home_goals'] + data['away_goals']
# Calculate rolling averages for recent performance
data['home_goals_avg'] = data['home_goals'].rolling(window=5).mean()
data['away_goals_avg'] = data['away_goals'].rolling(window=5).mean()
# Save the dataset with new features
data.to_csv('data/featured_football.csv', index=False)
This script demonstrates how to create new features such as goal difference, total goals, and rolling averages for recent performance, which can enhance the predictive power of machine learning models.
Building Machine Learning Models
Choosing the Right Algorithm
Choosing the right machine learning algorithm is crucial for building accurate predictive models. Common algorithms for sports betting include logistic regression, decision trees, random forests, and gradient boosting machines. Each algorithm has its strengths and weaknesses, so it's important to experiment and find the best fit for your data.
Here is an example of building a logistic regression model for sports betting:

import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load dataset with features
data = pd.read_csv('data/featured_football.csv')
# Define features and target
X = data.drop('result', axis=1)
y = data['result']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions and evaluate model
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy}")
This code demonstrates how to train a logistic regression model and evaluate its accuracy for predicting sports betting outcomes.
Evaluating Model Performance
Evaluating the performance of machine learning models is essential to ensure their reliability and accuracy. Common evaluation metrics for classification models include accuracy, precision, recall, and F1-score.
Here is an example of evaluating a model using these metrics:
from sklearn.metrics import precision_score, recall_score, f1_score
# Calculate precision, recall, and F1-score
precision = precision_score(y_test, y_pred)
recall = recall_score(y_test, y_pred)
f1 = f1_score(y_test, y_pred)
print(f"Precision: {precision}")
print(f"Recall: {recall}")
print(f"F1-score: {f1}")
This code demonstrates how to calculate precision, recall, and F1-score to evaluate the performance of a machine learning model in sports betting.

Hyperparameter Tuning
Hyperparameter tuning involves optimizing the parameters of a machine learning algorithm to improve its performance. GridSearchCV and RandomizedSearchCV from scikit-learn are commonly used for hyperparameter tuning.
Here is an example of hyperparameter tuning using GridSearchCV:
from sklearn.model_selection import GridSearchCV
# Define parameter grid
param_grid = {
'C': [0.1, 1, 10, 100],
'penalty': ['l1', 'l2']
}
# Perform grid search
grid_search = GridSearchCV(estimator=LogisticRegression(), param_grid=param_grid, cv=5)
grid_search.fit(X_train, y_train)
# Print best parameters
print(f"Best Parameters: {grid_search.best_params_}")
This code demonstrates how to perform hyperparameter tuning using GridSearchCV to find the optimal parameters for a logistic regression model.
Deploying Machine Learning Models
Setting Up Flask for API Development
Flask is a lightweight web framework for Python that is ideal for deploying machine learning models as REST APIs. Flask allows you to create endpoints that can receive data and return predictions.

Here is an example of setting up a basic Flask API for sports betting predictions:
from flask import Flask, request, jsonify
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('model.pkl')
@app.route('/')
def home():
return "Welcome to the Sports Betting Prediction API!"
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
This script sets up a Flask API with a prediction endpoint, allowing users to send data and receive predictions from the model.
Creating Endpoints for Predictions
Creating endpoints for predictions involves defining routes and handling HTTP requests. Flask makes it easy to create these endpoints and process incoming data.
Here is an example of creating a prediction endpoint in Flask:

from flask import Flask, request, jsonify
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('model.pkl')
@app.route('/predict', methods=['POST'])
def predict():
try:
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
except Exception as e:
return jsonify({'error': str(e)})
if __name__ == '__main__':
app.run(debug=True)
This script enhances the prediction endpoint with error handling, ensuring that any issues with the request are properly communicated back to the user.
Deploying on Heroku
Heroku is a popular platform for deploying web applications. Deploying your Flask API on Heroku involves creating a Procfile
, setting up a Git repository, and pushing the code to Heroku.
Here is an example of deploying a Flask API on Heroku:
- Create a
Procfile
with the following content:web: gunicorn app:app
- Initialize a Git repository:
git init git add . git commit -m "Initial commit"
- Create a new Heroku app:
heroku create your-app-name
- Deploy the app to Heroku:
bash git push heroku master
This series of commands deploys your Flask API to Heroku, making it accessible online.
Best Practices for Machine Learning in Sports Betting
Data Security and Privacy
Ensuring data security and privacy is crucial when handling sports data and user information. Implementing secure communication protocols, encrypting sensitive data, and adhering to data privacy regulations are essential practices.
For example, you can use HTTPS for secure communication and encrypt sensitive data using libraries like cryptography:
from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Encrypt data
encrypted_data = cipher_suite.encrypt(b
"Sensitive Data")
print(f"Encrypted Data: {encrypted_data}")
# Decrypt data
decrypted_data = cipher_suite.decrypt(encrypted_data)
print(f"Decrypted Data: {decrypted_data.decode()}")
This script demonstrates how to encrypt and decrypt data using the cryptography library, ensuring data security.
Regular Model Updates
Sports betting models need to be regularly updated with new data to maintain their accuracy and relevance. Implementing a pipeline for continuous model training and deployment ensures that your models stay up-to-date with the latest sports data.
Here is an example of setting up a pipeline using Airflow:
from airflow import DAG
from airflow.operators.python_operator import PythonOperator
from datetime import datetime
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
import joblib
def fetch_data():
# Fetch new sports data
data = pd.read_csv('https://example.com/new_sports_data.csv')
data.to_csv('/tmp/new_data.csv', index=False)
def train_model():
# Load new data
data = pd.read_csv('/tmp/new_data.csv')
X = data.drop('result', axis=1)
y = data['result']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Save the trained model
joblib.dump(model, 'model.pkl')
default_args = {
'owner': 'airflow',
'start_date': datetime(2023, 1, 1),
'retries': 1,
}
dag = DAG('sports_betting_pipeline', default_args=default_args, schedule_interval='@daily')
fetch_data_task = PythonOperator(task_id='fetch_data', python_callable=fetch_data, dag=dag)
train_model_task = PythonOperator(task_id='train_model', python_callable=train_model, dag=dag)
fetch_data_task >> train_model_task
This script sets up an Airflow DAG to fetch new sports data and retrain the model daily, ensuring that the model is regularly updated.
Enhancing Predictive Accuracy
Enhancing the predictive accuracy of your sports betting models involves continuous experimentation and improvement. Techniques such as feature selection, ensemble methods, and model stacking can significantly boost model performance.
Here is an example of using ensemble methods to improve predictive accuracy:
from sklearn.ensemble import RandomForestClassifier, GradientBoostingClassifier
from sklearn.ensemble import VotingClassifier
# Train individual models
rf_model = RandomForestClassifier(n_estimators=100, random_state=42)
gb_model = GradientBoostingClassifier(n_estimators=100, random_state=42)
# Create an ensemble model using VotingClassifier
ensemble_model = VotingClassifier(estimators=[
('rf', rf_model),
('gb', gb_model)
], voting='soft')
# Train the ensemble model
ensemble_model.fit(X_train, y_train)
# Make predictions and evaluate the ensemble model
y_pred_ensemble = ensemble_model.predict(X_test)
ensemble_accuracy = accuracy_score(y_test, y_pred_ensemble)
print(f"Ensemble Model Accuracy: {ensemble_accuracy}")
This code demonstrates how to use ensemble methods to combine the predictions of multiple models, improving overall predictive accuracy.
By following these best practices and leveraging the power of machine learning, you can significantly enhance sports betting strategies. From data preparation to model deployment, each step is crucial in building a robust and accurate system that can provide valuable insights and predictions for sports betting.
If you want to read more articles similar to Enhancing Sports Betting with Machine Learning in Python, you can visit the Applications category.
You Must Read