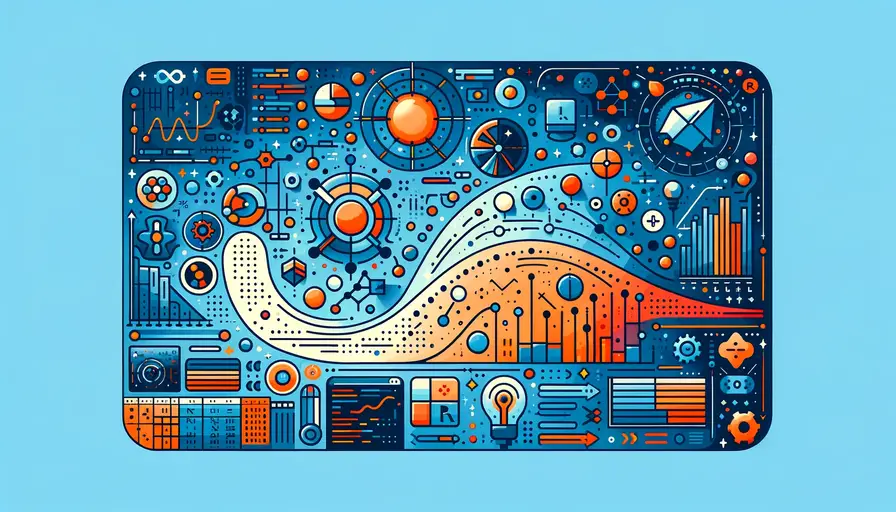
Expanding Machine Learning Beyond Regression

- Use Classification Algorithms for Non-Regression Problems
- Apply Natural Language Processing for Text Classification
- Utilize Clustering Algorithms for Unsupervised Learning
- Employ Anomaly Detection for Identifying Outliers
- Use Reinforcement Learning for Decision-Making
- Apply Deep Learning for Image and Speech Recognition
- Utilize Time Series Forecasting for Predictions
- Use Recommendation Systems for Personalized Suggestions
- Employ Dimensionality Reduction for High-Dimensional Data
- Utilize Transfer Learning for New Tasks
Use Classification Algorithms for Non-Regression Problems
Classification Algorithms
Classification algorithms are crucial for non-regression problems, where the goal is to categorize data into predefined classes. Unlike regression, which predicts continuous values, classification predicts discrete labels. Algorithms such as Logistic Regression, Decision Trees, Random Forests, and Support Vector Machines (SVMs) are commonly used for classification tasks.
Logistic Regression is a simple yet effective algorithm for binary classification problems. It models the probability that a given input belongs to a particular class. Decision Trees and Random Forests are powerful tools that can handle both binary and multiclass classification problems. They work by creating a series of decision rules based on the input features, leading to accurate predictions.
Support Vector Machines (SVMs) are particularly effective for high-dimensional spaces. They find the optimal hyperplane that separates the classes in the feature space, maximizing the margin between them. SVMs are versatile and can be used for both linear and non-linear classification tasks through the use of kernel functions.
# Example: Implementing a classification algorithm with scikit-learn
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
X, y = load_data() # Replace with actual data loading code
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Initialize the classifier
classifier = RandomForestClassifier(n_estimators=100)
# Train the classifier
classifier.fit(X_train, y_train)
# Make predictions
y_pred = classifier.predict(X_test)
# Evaluate the accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Apply Natural Language Processing for Text Classification
Text Classification Techniques
Natural Language Processing (NLP) techniques are essential for text classification tasks. NLP involves analyzing and manipulating human language data, enabling machines to understand and process text. Techniques like tokenization, stemming, and lemmatization prepare text data for machine learning models.

Tokenization is the process of splitting text into individual words or tokens, which can then be analyzed. Stemming reduces words to their base or root form, while lemmatization transforms words to their canonical form. These preprocessing steps help in standardizing the text data, making it more suitable for analysis.
Vectorization techniques, such as TF-IDF (Term Frequency-Inverse Document Frequency) and word embeddings (Word2Vec, GloVe), convert text into numerical representations. These vectors can then be fed into classification algorithms like Naive Bayes, Support Vector Machines, and Recurrent Neural Networks (RNNs) for text classification tasks.
# Example: Implementing text classification with scikit-learn and NLTK
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.pipeline import make_pipeline
from sklearn.metrics import classification_report
import nltk
# Load and preprocess dataset
text_data, labels = load_text_data() # Replace with actual data loading code
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(text_data, labels, test_size=0.3, random_state=42)
# Create a pipeline for TF-IDF vectorization and Naive Bayes classification
model = make_pipeline(TfidfVectorizer(), MultinomialNB())
# Train the model
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
print(classification_report(y_test, y_pred))
Utilize Clustering Algorithms for Unsupervised Learning
Clustering Techniques
Clustering algorithms are used in unsupervised learning to group similar data points together based on their features. These algorithms do not require labeled data, making them useful for exploring data structure and identifying patterns. Common clustering algorithms include K-Means, Hierarchical Clustering, and DBSCAN.
K-Means is one of the simplest and most popular clustering algorithms. It partitions the data into K clusters, with each data point assigned to the cluster with the nearest mean. Hierarchical Clustering builds a tree of clusters by either a bottom-up approach (agglomerative) or a top-down approach (divisive). DBSCAN (Density-Based Spatial Clustering of Applications with Noise) identifies clusters based on the density of data points, making it effective for discovering clusters of arbitrary shapes.

Clustering is widely used in customer segmentation, image compression, and anomaly detection. By grouping similar data points, it helps in understanding the underlying structure of the data and in making data-driven decisions.
# Example: Implementing K-Means clustering with scikit-learn
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Load dataset
X = load_data() # Replace with actual data loading code
# Initialize the K-Means algorithm
kmeans = KMeans(n_clusters=3, random_state=42)
# Fit the algorithm to the data
kmeans.fit(X)
# Get the cluster labels
labels = kmeans.labels_
# Plot the clusters
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='viridis')
plt.show()
Employ Anomaly Detection for Identifying Outliers
What is Anomaly Detection?
Anomaly detection involves identifying data points that deviate significantly from the majority of the data. These outliers can indicate critical insights, such as fraudulent transactions, network intrusions, or defects in manufacturing processes. Anomaly detection algorithms are essential for maintaining the integrity and security of systems.
Isolation Forest and Local Outlier Factor (LOF) are popular anomaly detection algorithms. Isolation Forest works by isolating observations by randomly selecting a feature and then randomly selecting a split value between the maximum and minimum values of the selected feature. The algorithm isolates anomalies closer to the root of the tree, making it efficient for large datasets. LOF calculates the local density deviation of a given data point with respect to its neighbors, identifying anomalies based on their relative density.
Why is Anomaly Detection Important?
Anomaly detection is crucial in various domains. In finance, it helps in identifying fraudulent transactions and unusual trading activities. In cybersecurity, it detects network intrusions and suspicious activities. In manufacturing, it identifies defects and ensures product quality. By identifying anomalies, organizations can take proactive measures to mitigate risks and ensure smooth operations.

Types of Anomaly Detection Algorithms
There are several types of anomaly detection algorithms, including statistical methods, clustering-based methods, and machine learning-based methods. Statistical methods involve defining a normal behavior model and identifying deviations from this model. Clustering-based methods group similar data points together and identify points that do not belong to any cluster. Machine learning-based methods, such as autoencoders and neural networks, learn patterns from the data and identify deviations from these patterns.
# Example: Implementing Isolation Forest for anomaly detection with scikit-learn
from sklearn.ensemble import IsolationForest
# Load dataset
X = load_data() # Replace with actual data loading code
# Initialize the Isolation Forest algorithm
iso_forest = IsolationForest(contamination=0.1, random_state=42)
# Fit the algorithm to the data
iso_forest.fit(X)
# Predict anomalies
anomalies = iso_forest.predict(X)
# -1 indicates an anomaly, 1 indicates normal data
print(anomalies)
Use Reinforcement Learning for Decision-Making
How Reinforcement Learning Works
Reinforcement learning (RL) is a type of machine learning where an agent learns to make decisions by interacting with an environment. The agent takes actions based on a policy, receives rewards or penalties, and updates its policy to maximize cumulative rewards. RL is inspired by behavioral psychology and is used for decision-making tasks where an optimal sequence of actions is desired.
Q-Learning and Deep Q-Networks (DQNs) are popular RL algorithms. Q-Learning is a model-free algorithm that learns the value of actions based on their rewards. DQNs combine Q-learning with deep learning, using neural networks to approximate the Q-value function, enabling RL to handle high-dimensional state spaces.
Applications of Reinforcement Learning
Reinforcement learning is widely used in robotics, gaming, finance, and autonomous systems. In robotics, RL enables robots to learn complex tasks through trial and error. In gaming, it helps in developing intelligent agents that can play games at superhuman levels. In finance, RL is used for portfolio optimization and algorithmic trading. Autonomous systems, such as self-driving cars, use RL to make real-time decisions and navigate complex environments.

# Example: Implementing Q-Learning for a simple environment
import numpy as np
# Initialize the Q-table
Q = np.zeros((state_space_size, action_space_size))
# Define learning parameters
alpha = 0.1 # Learning rate
gamma = 0.6 # Discount factor
epsilon = 0.1 # Exploration rate
# Q-Learning algorithm
for episode in range(total_episodes):
state = reset_environment() # Reset the environment and get initial state
done = False
while not done:
# Choose an action (epsilon-greedy strategy)
if np.random.uniform(0, 1) < epsilon:
action = np.random.choice(action_space_size)
else:
action = np.argmax(Q[state, :])
# Take action and observe the next state and reward
next_state, reward, done =
take_action(state, action)
# Update Q-value
Q[state, action] = Q[state, action] + alpha * (reward + gamma * np.max(Q[next_state, :]) - Q[state, action])
# Move to the next state
state = next_state
print('Trained Q-Table:', Q)
Apply Deep Learning for Image and Speech Recognition
Image Recognition
Deep learning models, particularly Convolutional Neural Networks (CNNs), have revolutionized image recognition tasks. CNNs are designed to automatically and adaptively learn spatial hierarchies of features from input images. They consist of layers that perform convolutions, pooling, and activation functions, enabling the model to capture intricate patterns and details in images.
CNNs are widely used for tasks such as object detection, facial recognition, and medical image analysis. They have achieved state-of-the-art performance in these domains by learning from large datasets and leveraging their ability to generalize well to new images. The architecture of CNNs, with its hierarchical structure, allows for the detection of low-level features like edges and textures as well as high-level features like shapes and objects.
# Example: Implementing a CNN for image recognition with Keras
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Initialize the model
model = Sequential()
# Add convolutional and pooling layers
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(32, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
# Add flattening and fully connected layers
model.add(Flatten())
model.add(Dense(units=128, activation='relu'))
model.add(Dense(units=1, activation='sigmoid'))
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(training_data, training_labels, epochs=25, batch_size=32)
Speech Recognition
Recurrent Neural Networks (RNNs), especially Long Short-Term Memory (LSTM) networks, are well-suited for speech recognition tasks. These models excel at processing sequential data and capturing temporal dependencies. LSTMs address the limitations of traditional RNNs by using memory cells and gating mechanisms to retain important information over long sequences.
Speech recognition involves converting spoken language into text. This task requires models to understand the temporal dynamics of speech and handle variations in pronunciation, accent, and noise. LSTMs and Convolutional Neural Networks (CNNs) are often combined in end-to-end speech recognition systems to achieve high accuracy and robustness.

# Example: Implementing an LSTM for speech recognition with Keras
from keras.models import Sequential
from keras.layers import LSTM, Dense
# Initialize the model
model = Sequential()
# Add LSTM layers
model.add(LSTM(128, input_shape=(100, 13), return_sequences=True))
model.add(LSTM(128))
# Add fully connected layer
model.add(Dense(units=10, activation='softmax'))
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(training_data, training_labels, epochs=25, batch_size=32)
Applications Beyond Regression
Deep learning models are used in various applications beyond image and speech recognition. In healthcare, they are employed for disease diagnosis and treatment planning. In autonomous driving, they enable vehicles to perceive and navigate their environment. In finance, deep learning models are used for fraud detection, algorithmic trading, and credit scoring. Their ability to learn from vast amounts of data and generalize to new scenarios makes them invaluable across industries.
Utilize Time Series Forecasting for Predictions
ARIMA (AutoRegressive Integrated Moving Average)
ARIMA is a popular time series forecasting algorithm that combines autoregression (AR), differencing (I), and moving average (MA) components. It is used to model and forecast time series data by capturing the linear relationships between past and future values. ARIMA is effective for non-stationary time series data that exhibit trends and seasonality.
# Example: Implementing ARIMA for time series forecasting with statsmodels
from statsmodels.tsa.arima_model import ARIMA
# Load time series data
data = load_time_series_data() # Replace with actual data loading code
# Fit the ARIMA model
model = ARIMA(data, order=(5, 1, 0))
model_fit = model.fit(disp=0)
# Make predictions
predictions = model_fit.forecast(steps=10)[0]
print(predictions)
LSTM (Long Short-Term Memory)
LSTM networks are a type of recurrent neural network (RNN) that are well-suited for time series forecasting. They can capture long-term dependencies in sequential data, making them ideal for modeling temporal patterns. LSTMs use memory cells and gating mechanisms to retain and update information over time, addressing the vanishing gradient problem in traditional RNNs.
# Example: Implementing LSTM for time series forecasting with Keras
from keras.models import Sequential
from keras.layers import LSTM, Dense
# Initialize the model
model = Sequential()
# Add LSTM layer
model.add(LSTM(50, activation='relu', input_shape=(n_steps, n_features)))
# Add fully connected layer
model.add(Dense(1))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Train the model
model.fit(X_train, y_train, epochs=300, verbose=0)
# Make predictions
y_pred = model.predict(X_test)
print(y_pred)
Time Series Applications
Time series forecasting is used in various applications, such as stock market prediction, weather forecasting, and demand planning. Accurate time series forecasts enable businesses to make informed decisions, optimize inventory levels, and improve resource allocation. By capturing temporal patterns and trends, time series models provide valuable insights for future planning.

Use Recommendation Systems for Personalized Suggestions
Benefits of Recommendation Systems
Recommendation systems provide personalized content suggestions based on user preferences and behavior. They enhance user experience by offering relevant items, such as products, movies, or articles. Recommendation systems use various techniques, including collaborative filtering, content-based filtering, and hybrid approaches, to deliver accurate recommendations.
# Example: Implementing a simple collaborative filtering recommendation system
from surprise import Dataset, Reader, SVD
from surprise.model_selection import train_test_split
# Load dataset
data = Dataset.load_builtin('ml-100k')
# Split the data into training and testing sets
trainset, testset = train_test_split(data, test_size=0.25)
# Initialize the algorithm
algo = SVD()
# Train the algorithm on the training set
algo.fit(trainset)
# Make predictions on the test set
predictions = algo.test(testset)
# Evaluate the algorithm
accuracy.rmse(predictions)
Enhancing User Experience
Recommendation systems play a crucial role in enhancing user engagement and satisfaction. By understanding user preferences and providing personalized suggestions, they increase the likelihood of user interactions and retention. This personalization fosters a deeper connection between the user and the platform, driving loyalty and increasing revenue.
Applications in Various Industries
Recommendation systems are used in various industries, including e-commerce, entertainment, and content streaming. In e-commerce, they suggest products based on user browsing and purchase history. In entertainment, they recommend movies, TV shows, and music based on user preferences. In content streaming, they personalize news articles, blog posts, and videos, keeping users engaged and informed.
Employ Dimensionality Reduction for High-Dimensional Data
Benefits of Dimensionality Reduction
Dimensionality reduction techniques simplify high-dimensional data by reducing the number of features while preserving important information. This process improves model performance, reduces computational complexity, and mitigates the curse of dimensionality. Techniques like Principal Component Analysis (PCA) and t-Distributed Stochastic Neighbor Embedding (t-SNE) are commonly used.
# Example: Implementing PCA for dimensionality reduction with scikit-learn
from sklearn.decomposition import PCA
# Load dataset
X = load_data() # Replace with actual data loading code
# Initialize PCA
pca = PCA(n_components=2)
# Fit and transform the data
X_reduced = pca.fit_transform(X)
# Plot the reduced data
plt.scatter(X_reduced[:, 0], X_reduced[:, 1])
plt.show()
Principal Component Analysis (PCA)
PCA is a linear dimensionality reduction technique that transforms the data into a new coordinate system, where the greatest variance lies on the first principal component. Subsequent components capture decreasing amounts of variance. PCA is useful for visualization, noise reduction, and feature extraction.
t-SNE for Visualization
t-SNE is a non-linear dimensionality reduction technique used for visualizing high-dimensional data in a low-dimensional space. It emphasizes preserving local structure, making it suitable for clustering and visualizing complex datasets. t-SNE is widely used in exploratory data analysis and identifying patterns in high-dimensional data.
# Example: Implementing t-SNE for visualization with scikit-learn
from sklearn.manifold import TSNE
# Load dataset
X = load_data() # Replace with actual data loading code
# Initialize t-SNE
tsne = TSNE(n_components=2)
# Fit and transform the data
X_embedded = tsne.fit_transform(X)
# Plot the embedded data
plt.scatter(X_embedded[:, 0],
X_embedded[:, 1])
plt.show()
Utilize Transfer Learning for New Tasks
Benefits of Transfer Learning
Transfer learning leverages pre-trained models to solve new tasks, reducing the need for extensive training data and computational resources. By transferring knowledge from one domain to another, transfer learning accelerates the development of machine learning models and improves performance.
# Example: Implementing transfer learning with a pre-trained model in Keras
from keras.applications import VGG16
from keras.models import Model
from keras.layers import Dense, Flatten
# Load the pre-trained VGG16 model
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Add custom layers on top of the pre-trained model
x = base_model.output
x = Flatten()(x)
x = Dense(1024, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# Define the new model
model = Model(inputs=base_model.input, outputs=predictions)
# Freeze the layers of the pre-trained model
for layer in base_model.layers:
layer.trainable = False
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(training_data, training_labels, epochs=10, batch_size=32)
Accelerating Model Development
Transfer learning significantly reduces the training time and computational cost of developing new models. By starting with a pre-trained model, developers can fine-tune it on their specific task, leveraging the existing knowledge encoded in the model. This approach is particularly useful in scenarios with limited training data.
Applications Across Domains
Transfer learning is widely used in various domains, including computer vision, natural language processing, and speech recognition. In computer vision, models pre-trained on large datasets like ImageNet are fine-tuned for specific tasks such as medical image analysis or object detection. In NLP, pre-trained language models like BERT and GPT-3 are adapted for tasks like sentiment analysis and text generation. Transfer learning enables rapid development and deployment of high-performing models across diverse applications.
If you want to read more articles similar to Expanding Machine Learning Beyond Regression, you can visit the Applications category.
You Must Read