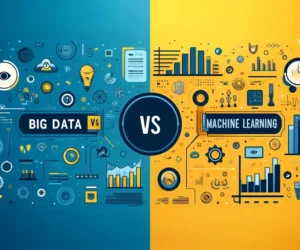
Exploring NLP: Machine Learning or Alternative Approaches?

Advancements in Natural Language Processing
Revolutionizing Text Analysis with Machine Learning
Machine learning has brought about a paradigm shift in the field of natural language processing (NLP), enabling more sophisticated and accurate text analysis. Traditional methods relied heavily on hand-crafted rules and manual feature extraction, which were often limited in their ability to handle the complexities and nuances of human language. Machine learning models, particularly those based on deep learning, have surpassed these limitations by learning directly from large datasets.
Deep learning models, such as transformers, have been particularly influential in advancing text analysis. Transformers like BERT (Bidirectional Encoder Representations from Transformers) and GPT-3 (Generative Pre-trained Transformer 3) have set new benchmarks in various NLP tasks, including sentiment analysis, text classification, and named entity recognition. These models can understand context by processing text bidirectionally, capturing the meaning of words based on their surrounding context.
Sentiment analysis, for instance, has been significantly improved with machine learning. By training on extensive corpora of labeled text, machine learning models can accurately gauge the sentiment of a given text, whether it be positive, negative, or neutral. This capability is essential for businesses to understand customer opinions and tailor their strategies accordingly.
Example: Sentiment Analysis Using BERT in Python
import numpy as np
import pandas as pd
from transformers import BertTokenizer, BertForSequenceClassification
from transformers import Trainer, TrainingArguments
import torch
# Load dataset
data = pd.read_csv('sentiment_data.csv')
train_data = data.sample(frac=0.8, random_state=42)
test_data = data.drop(train_data.index)
# Tokenize data
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
train_encodings = tokenizer(train_data['text'].tolist(), truncation=True, padding=True)
test_encodings = tokenizer(test_data['text'].tolist(), truncation=True, padding=True)
# Create dataset class
class SentimentDataset(torch.utils.data.Dataset):
def __init__(self, encodings, labels):
self.encodings = encodings
self.labels = labels
def __getitem__(self, idx):
item = {key: torch.tensor(val[idx]) for key, val in self.encodings.items()}
item['labels'] = torch.tensor(self.labels[idx])
return item
def __len__(self):
return len(self.labels)
train_dataset = SentimentDataset(train_encodings, train_data['label'].tolist())
test_dataset = SentimentDataset(test_encodings, test_data['label'].tolist())
# Initialize model
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=16,
per_device_eval_batch_size=16,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
logging_steps=10,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=test_dataset
)
# Train model
trainer.train()
In this example, a BERT model is used for sentiment analysis, showcasing how deep learning can be applied to understand and classify the sentiment of textual data accurately.
Unveiling the Top Attacks Targeting Machine Learning and AI SystemsEnhancing Machine Translation
Machine translation has been another area significantly enhanced by machine learning. Early translation systems relied on extensive rule-based systems and bilingual dictionaries, which often resulted in grammatically incorrect and awkward translations. The advent of neural machine translation (NMT) models, such as Google's GNMT and OpenAI's GPT-3, has greatly improved translation quality by considering entire sentences rather than translating word-by-word.
NMT models use encoder-decoder architectures to translate text from one language to another. The encoder converts the input text into a high-dimensional vector that captures its meaning, while the decoder generates the translated text from this vector. This method allows the model to maintain the context of the entire sentence, resulting in more fluent and coherent translations.
A notable advancement in this area is zero-shot translation, where models can translate between language pairs they have never seen during training. This capability is achieved by training on multilingual datasets, allowing the model to learn general language representations. This advancement has made it possible to translate less common languages and dialects, broadening access to information globally.
Improving Conversational AI
Conversational AI, including chatbots and virtual assistants, has seen remarkable improvements with machine learning. Traditional rule-based systems were limited by predefined scripts and could not handle unexpected user inputs effectively. Machine learning models, especially those based on transformer architectures like GPT-3, have enabled the development of more intelligent and responsive conversational agents.
Pattern Recognition and Machine Learning with Christopher BishopThese models are trained on vast amounts of conversational data, allowing them to generate contextually appropriate and coherent responses. For instance, chatbots powered by GPT-3 can understand complex queries and provide detailed answers, making them suitable for applications in customer service, technical support, and personal assistants.
Furthermore, reinforcement learning has been applied to train conversational agents to optimize their responses based on user feedback. This approach allows chatbots to improve over time by learning from interactions, leading to more effective and engaging conversations. Techniques like Deep Q-Learning and Policy Gradient Methods have been used to enhance the learning process of these agents.
Example: Building a Simple Chatbot with GPT-3 in Python
import openai
# Set up OpenAI API key
openai.api_key = 'your-api-key-here'
# Define a function to interact with GPT-3
def chat_with_gpt3(prompt):
response = openai.Completion.create(
engine="davinci",
prompt=prompt,
max_tokens=150
)
return response.choices[0].text.strip()
# Example conversation
print(chat_with_gpt3("Hello, how are you?"))
In this example, a simple chatbot is created using OpenAI's GPT-3. The chatbot can generate human-like responses based on the given prompt, demonstrating the capabilities of modern conversational AI.
Alternative Approaches to NLP
Rule-Based Systems and Their Applications
While machine learning has dominated recent advancements in NLP, rule-based systems still have their place in certain applications. Rule-based systems use predefined linguistic rules and patterns to process and analyze text. These systems are particularly useful when dealing with well-defined tasks and structured data, where the complexity of machine learning models may not be necessary.
K-Nearest Neighbors Algorithm in Machine LearningOne advantage of rule-based systems is their interpretability. Unlike machine learning models, which can be black boxes, rule-based systems provide clear and understandable outputs based on explicit rules. This transparency is beneficial in applications where explainability is crucial, such as legal document analysis and regulatory compliance.
However, rule-based systems have limitations in handling the variability and ambiguity of natural language. They often require extensive manual effort to create and maintain the rules, making them less scalable compared to machine learning models. Despite these limitations, they remain valuable in specific contexts where their simplicity and clarity are advantageous.
Example: Rule-Based Text Processing in Python
import re
# Define a rule-based function to extract dates from text
def extract_dates(text):
date_pattern = r'\b\d{1,2}/\d{1,2}/\d{2,4}\b'
dates = re.findall(date_pattern, text)
return dates
# Example usage
text = "The meeting is scheduled for 12/05/2021. Another event is on 01/15/2022."
print(extract_dates(text))
In this example, a simple rule-based function is used to extract dates from text using regular expressions. This demonstrates the application of rule-based systems in NLP tasks.
Statistical Methods in NLP
Before the rise of deep learning, statistical methods played a significant role in NLP. Techniques such as n-grams, hidden Markov models (HMMs), and latent semantic analysis (LSA) were widely used for tasks like text classification, speech recognition, and topic modeling. These methods rely on statistical properties of text, such as word frequencies and co-occurrence patterns, to derive meaning.
Is Machine Learning an Extension of Statistics?N-grams, for example, are sequences of n words used to predict the next word in a sequence. This approach is useful for tasks like text generation and speech recognition, where the likelihood of word sequences needs to be estimated. HMMs have been used for part-of-speech tagging and named entity recognition, leveraging the probabilistic nature of language.
While statistical methods have been largely superseded by machine learning, they still offer valuable insights and can be effective for specific tasks, particularly when computational resources are limited. Their simplicity and effectiveness in certain contexts make them a viable alternative to more complex machine learning models.
Hybrid Approaches Combining Machine Learning and Rule-Based Methods
Hybrid approaches that combine machine learning with rule-based methods have emerged as a powerful strategy in NLP. These approaches leverage the strengths of both paradigms, using rules to handle well-defined tasks and machine learning to manage more complex and ambiguous aspects of language processing.
For instance, a hybrid system might use rule-based methods for initial text preprocessing, such as tokenization and entity recognition, and then apply machine learning models for deeper semantic analysis and sentiment classification. This combination can enhance the overall performance of NLP systems, providing robustness and flexibility.
Unsupervised Learning: Unlocking Hidden PatternsHybrid approaches are particularly useful in applications where domain-specific knowledge is critical. By incorporating expert-defined rules, these systems can ensure that the output is relevant and accurate, while machine learning models can adapt and improve over time based on new data.
Example: Hybrid NLP System in Python
import re
import spacy
from transformers import pipeline
# Load spaCy model
nlp = spacy.load('en_core_web_sm')
# Load sentiment analysis pipeline
sentiment_pipeline = pipeline('sentiment-analysis')
# Define a function to process text using hybrid approach
def process_text(text):
# Rule-based entity extraction
doc = nlp(text)
entities = [(ent.text, ent.label_) for ent in doc.ents]
# Machine learning-based sentiment analysis
sentiment = sentiment_pipeline(text)
return entities, sentiment
# Example usage
text = "Apple is expected to release a new iPhone in September. The market reaction is positive."
entities, sentiment = process_text(text)
print(f"Entities: {entities}")
print(f"Sentiment: {sentiment}")
In this example, a hybrid NLP system combines rule-based entity extraction using spaCy with sentiment analysis using transformer models. This approach leverages the strengths of both methods to provide comprehensive text analysis.
Real-World Applications of NLP
Enhancing Customer Support
NLP has significantly improved customer support by enabling the development of intelligent chatbots and virtual assistants. These systems can handle a wide range of customer queries, providing instant responses and reducing the workload on human agents. By leveraging machine learning models, chatbots can understand and respond to complex questions, improving customer satisfaction.
For example, companies like Zendesk and LivePerson use NLP-powered chatbots to enhance their customer support services. These chatbots can resolve common issues, provide product information, and escalate complex queries to human agents when necessary. The ability to handle multiple queries simultaneously ensures that customers receive prompt and efficient support.
Beginner's Guide to Machine Learning: Dive into AIAdditionally, NLP can be used to analyze customer feedback and sentiment, providing valuable insights into customer satisfaction and areas for improvement. By processing large volumes of customer reviews and social media posts, businesses can identify common pain points and tailor their services to meet customer needs more effectively.
Streamlining Document Processing
Document processing is another area where NLP has made a significant impact. Traditional methods of document analysis and data extraction were time-consuming and prone to errors. NLP models can automate these tasks, extracting relevant information and categorizing documents with high accuracy.
Applications such as contract analysis, invoice processing, and compliance monitoring benefit from NLP's ability to understand and process unstructured text. For instance, tools like DocuSign and Adobe Sign leverage NLP to automate the extraction of key terms and clauses from contracts, streamlining the review process and ensuring compliance with legal standards.
Moreover, NLP can be used to summarize long documents, making it easier for professionals to review and understand large volumes of text quickly. Summarization models can identify the most important information and present it concisely, saving time and improving productivity.
Example: Document Summarization Using NLP in Python
from transformers import pipeline
# Load summarization pipeline
summarizer = pipeline('summarization')
# Example text
text = """
Machine learning is a branch of artificial intelligence (AI) that focuses on building systems that can learn from and make decisions based on data. Unlike traditional programming, where developers write explicit instructions for a computer to follow, machine learning algorithms use statistical techniques to allow computers to learn patterns in data and make predictions. The applications of machine learning are vast and include areas such as healthcare, finance, marketing, and autonomous vehicles.
"""
# Generate summary
summary = summarizer(text, max_length=50, min_length=25, do_sample=False)
print(summary[0]['summary_text'])
In this example, an NLP model is used to summarize a long piece of text, demonstrating how document summarization can improve efficiency and comprehension.
Facilitating Language Translation
NLP has revolutionized language translation, making it possible to break down language barriers and facilitate global communication. Machine translation models can translate text from one language to another with high accuracy and fluency, enabling businesses to reach a wider audience and individuals to access information in their native language.
Services like Google Translate and Microsoft Translator use advanced NMT models to provide real-time translations. These models are trained on large multilingual datasets, allowing them to capture the nuances and context of different languages. The ability to translate between numerous language pairs, including less common languages, ensures that information is accessible to a global audience.
Moreover, machine translation is used in various applications, such as translating legal documents, technical manuals, and marketing content. By automating the translation process, businesses can save time and resources while ensuring that their content is accurately translated and culturally relevant.
Exploring NLP through machine learning and alternative approaches reveals the vast potential of this field. While machine learning models, especially deep learning and transformers, have significantly advanced NLP capabilities, alternative methods such as rule-based systems and statistical techniques still play a valuable role. Real-world applications in customer support, document processing, and language translation demonstrate the transformative impact of NLP, enabling more efficient, accurate, and accessible communication across various domains. By continuing to innovate and integrate different approaches, NLP will continue to evolve, driving further advancements and applications.
If you want to read more articles similar to Exploring NLP: Machine Learning or Alternative Approaches?, you can visit the Artificial Intelligence category.
You Must Read