
Exploring the Depths of Machine Learning: Beyond Linear Regression
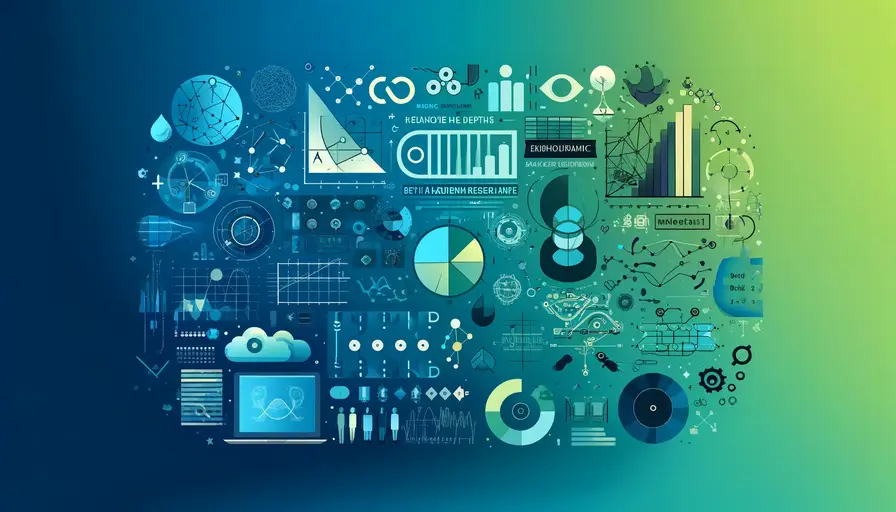
Machine learning encompasses a vast array of techniques and algorithms, each designed to handle different types of data and tasks. While linear regression is a foundational tool in the machine learning toolkit, there are numerous other methods that offer more flexibility and power, particularly when dealing with complex, non-linear relationships. This article explores advanced machine learning algorithms beyond linear regression, highlighting their capabilities, applications, and key concepts.
Content
Decision Trees and Ensemble Methods
Understanding Decision Trees
Decision trees are intuitive and powerful tools for both classification and regression tasks. They work by splitting the data into subsets based on the value of input features, creating a tree-like model of decisions. Each internal node represents a test on an attribute, each branch represents the outcome of the test, and each leaf node represents a class label or continuous value.
Decision trees have the advantage of being easy to interpret and visualize. However, they are prone to overfitting, especially when the tree is deep. Pruning methods can be applied to reduce overfitting by removing branches that have little importance.
Example of building a decision tree using scikit-learn:
Optimizing Text Classification with SIFT Method in MLimport pandas as pd
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load dataset
data = pd.read_csv('data/titanic.csv')
# Define features and target
features = data[['Pclass', 'Age', 'SibSp', 'Parch']]
target = data['Survived']
# Handle missing values
features['Age'].fillna(features['Age'].mean(), inplace=True)
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(features, target, test_size=0.2, random_state=42)
# Fit decision tree classifier
model = DecisionTreeClassifier(max_depth=3)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Random Forests and Bagging
Random forests are an extension of decision trees that address their tendency to overfit. A random forest is an ensemble of decision trees, usually trained with the bagging method. Bagging, short for Bootstrap Aggregating, involves training each tree on a different random subset of the training data and then averaging the results.
Random forests improve accuracy and robustness over individual decision trees. They also provide estimates of feature importance, helping identify the most influential variables in the dataset.
Example of building a random forest using scikit-learn:
from sklearn.ensemble import RandomForestClassifier
# Fit random forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Boosting Methods
Boosting is another ensemble technique that combines the outputs of several weak learners to create a strong learner. Unlike bagging, which trains models independently, boosting trains models sequentially. Each new model focuses on the errors made by the previous ones, gradually improving the performance.
Machine Learning and Web ServicesGradient boosting is a popular boosting method that builds models in a stage-wise fashion. It is highly effective for both regression and classification tasks but can be computationally intensive.
Example of building a gradient boosting model using scikit-learn:
from sklearn.ensemble import GradientBoostingClassifier
# Fit gradient boosting classifier
model = GradientBoostingClassifier(n_estimators=100, learning_rate=0.1, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Support Vector Machines
Concept of Support Vector Machines
Support Vector Machines (SVMs) are powerful supervised learning models used for classification and regression tasks. SVMs aim to find the optimal hyperplane that maximizes the margin between different classes. The data points closest to the hyperplane, known as support vectors, are critical in defining the decision boundary.
SVMs are effective in high-dimensional spaces and are versatile, using different kernel functions (linear, polynomial, radial basis function) to handle various types of data. They are particularly useful for binary classification problems.
Using Machine Learning to Predict Horse Racing OutcomesExample of building an SVM classifier using scikit-learn:
from sklearn.svm import SVC
# Fit SVM classifier
model = SVC(kernel='rbf', gamma='scale')
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
SVM for Regression
Support Vector Regression (SVR) extends SVM to regression problems. SVR uses the same principles as SVM for classification, aiming to find a function that deviates from the actual values by a margin no greater than a specified value, while still being as flat as possible.
SVR is robust to outliers and can model complex relationships through kernel functions. It is a powerful tool for time series forecasting, financial modeling, and other regression tasks.
Example of building an SVR model using scikit-learn:
Machine Learning Models for Trading Using Variables X and Yfrom sklearn.svm import SVR
# Fit SVR model
model = SVR(kernel='rbf', gamma='scale')
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
Kernel Trick in SVM
The kernel trick allows SVMs to operate in a high-dimensional space without explicitly computing the coordinates of the data in that space. Instead, it computes the inner products between the images of all pairs of data in the feature space. This enables SVMs to efficiently perform non-linear classification and regression.
Different kernels can be used depending on the data and the problem. The most common kernels are linear, polynomial, and radial basis function (RBF). The choice of kernel and its parameters can significantly impact the model's performance.
Example of using different kernels in SVM:
# Linear kernel
model_linear = SVC(kernel='linear')
model_linear.fit(X_train, y_train)
y_pred_linear = model_linear.predict(X_test)
accuracy_linear = accuracy_score(y_test, y_pred_linear)
print(f'Accuracy with Linear Kernel: {accuracy_linear}')
# Polynomial kernel
model_poly = SVC(kernel='poly', degree=3)
model_poly.fit(X_train, y_train)
y_pred_poly = model_poly.predict(X_test)
accuracy_poly = accuracy_score(y_test, y_pred_poly)
print(f'Accuracy with Polynomial Kernel: {accuracy_poly}')
# RBF kernel
model_rbf = SVC(kernel='rbf')
model_rbf.fit(X_train, y_train)
y_pred_rbf = model_rbf.predict(X_test)
accuracy_rbf = accuracy_score(y_test, y_pred_rbf)
print(f'Accuracy with RBF Kernel: {accuracy_rbf}')
Neural Networks and Deep Learning
Basics of Neural Networks
Neural networks are inspired by the human brain and consist of interconnected layers of neurons. Each neuron receives inputs, applies a linear transformation, and passes the result through a non-linear activation function. Neural networks can model complex patterns and relationships in data.
Enhancing JavaScript Chatbot with Machine LearningA neural network typically consists of an input layer, one or more hidden layers, and an output layer. The weights and biases of the connections between neurons are learned during training through backpropagation, which minimizes the error between the predicted and actual outputs.
Example of building a neural network using TensorFlow/Keras:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Define the model
model = Sequential([
Dense(64, activation='relu', input_shape=(X_train.shape[1],)),
Dense(64, activation='relu'),
Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_split=0.2)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
Deep Learning with Convolutional Neural Networks
Convolutional Neural Networks (CNNs) are a specialized type of neural network designed for processing structured grid data, such as images. CNNs use convolutional layers to automatically and adaptively learn spatial hierarchies of features from input images, making them highly effective for computer vision tasks.
CNNs consist of convolutional layers, pooling layers, and fully connected layers. The convolutional layers apply filters to the input image to detect features like edges, textures, and shapes. Pooling layers reduce the dimensionality of the feature maps, retaining essential information while reducing computational complexity.
Machine Learning Predicts Transcription and PhenotypesExample of building a CNN using TensorFlow/Keras:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Define the model
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_split=0.2)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Accuracy: {accuracy}')
Recurrent Neural Networks for Sequence Data
Recurrent Neural Networks (RNNs) are designed for processing sequential data, such as time series, speech, and text. RNNs have connections that form directed cycles, allowing them to maintain a memory of previous inputs. This makes them suitable for tasks where the context of previous data points is crucial.
However, standard RNNs suffer from the vanishing gradient problem, which makes training deep networks challenging. Long Short-Term Memory (LSTM) networks and Gated Recurrent Units (GRUs) are variants of RNNs that address this issue by introducing gating mechanisms to control the flow of information.
Example of building an LSTM using TensorFlow/Keras:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Define the model
model = Sequential([
LSTM(50, activation='relu', input_shape=(X_train.shape[1], X_train.shape[2])),
Dense(1)
])
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Train the model
model.fit(X_train, y_train, epochs=50, batch_size=32, validation_split=0.2)
# Evaluate the model
loss = model.evaluate(X_test, y_test)
print(f'Mean Squared Error: {loss}')
Clustering and Dimensionality Reduction
K-Means Clustering
K-means clustering is a popular unsupervised learning algorithm used for partitioning a dataset into K distinct clusters. The algorithm aims to minimize the within-cluster sum of squares by iteratively updating the cluster centroids and assigning data points to the nearest centroids.
K-means is simple and efficient for small to medium-sized datasets but may struggle with complex, non-spherical clusters. It requires the number of clusters (K) to be specified in advance.
Example of K-means clustering using scikit-learn:
import pandas as pd
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Load dataset
data = pd.read_csv('data/iris.csv')
# Define features
features = data[['SepalLength', 'SepalWidth', 'PetalLength', 'PetalWidth']]
# Fit K-means clustering model
model = KMeans(n_clusters=3, random_state=42)
model.fit(features)
# Predict cluster labels
labels = model.predict(features)
# Plot results
plt.scatter(features['SepalLength'], features['SepalWidth'], c=labels, cmap='viridis')
plt.xlabel('Sepal Length')
plt.ylabel('Sepal Width')
plt.title('K-Means Clustering')
plt.show()
Hierarchical Clustering
Hierarchical clustering builds a hierarchy of clusters using either an agglomerative (bottom-up) or divisive (top-down) approach. In agglomerative clustering, each data point starts as its own cluster, and clusters are recursively merged based on their similarity. Divisive clustering starts with one cluster and recursively splits it into smaller clusters.
Hierarchical clustering does not require specifying the number of clusters in advance and produces a dendrogram that illustrates the merging or splitting process. However, it can be computationally intensive for large datasets.
Example of hierarchical clustering using scikit-learn:
from scipy.cluster.hierarchy import dendrogram, linkage
import matplotlib.pyplot as plt
# Load dataset
data = pd.read_csv('data/iris.csv')
# Define features
features = data[['SepalLength', 'SepalWidth', 'PetalLength', 'PetalWidth']]
# Perform hierarchical clustering
linked = linkage(features, method='ward')
# Plot dendrogram
plt.figure(figsize=(10, 7))
dendrogram(linked, orientation='top', distance_threshold=0, no_labels=True)
plt.title('Hierarchical Clustering Dendrogram')
plt.xlabel('Data Points')
plt.ylabel('Distance')
plt.show()
Principal Component Analysis
Principal Component Analysis (PCA) is a dimensionality reduction technique that transforms high-dimensional data into a lower-dimensional space while retaining as much variance as possible. PCA identifies the principal components, which are orthogonal directions that capture the maximum variance in the data.
PCA is useful for visualizing high-dimensional data, removing noise, and improving the performance of machine learning algorithms by reducing overfitting and computational complexity.
Example of PCA using scikit-learn:
import pandas as pd
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
# Load dataset
data = pd.read_csv('data/iris.csv')
# Define features
features = data[['SepalLength', 'SepalWidth', 'PetalLength', 'PetalWidth']]
# Perform PCA
pca = PCA(n_components=2)
principal_components = pca.fit_transform(features)
# Plot results
plt.scatter(principal_components[:, 0], principal_components[:, 1], c=data['Species'], cmap='viridis')
plt.xlabel('Principal Component 1')
plt.ylabel('Principal Component 2')
plt.title('PCA of Iris Dataset')
plt.show()
Machine learning offers a rich and diverse set of algorithms beyond linear regression, each suited for different types of data and tasks. From decision trees and ensemble methods to support vector machines and neural networks, these advanced techniques provide the flexibility and power needed to tackle complex problems. By exploring and understanding these methods, practitioners can unlock the full potential of machine learning and drive innovation across various domains.
If you want to read more articles similar to Exploring the Depths of Machine Learning: Beyond Linear Regression, you can visit the Applications category.
You Must Read