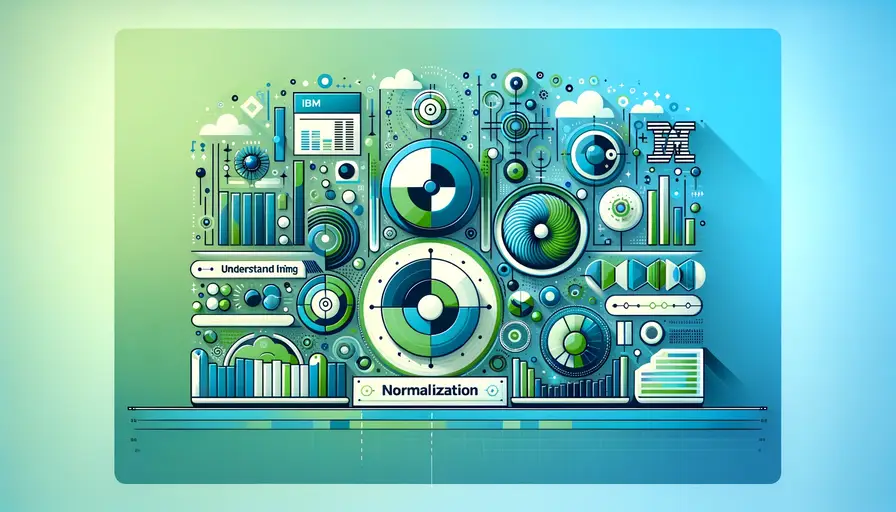
Exploring the Latest Breakthroughs in Modern Machine Learning Models

Revolutionary Advances in Deep Learning Architectures
Transformers: Transforming NLP and Beyond
The introduction of transformers has revolutionized natural language processing (NLP) and expanded into various other domains. Transformers, introduced in the paper "Attention is All You Need" by Vaswani et al., have become the backbone of many state-of-the-art models. Unlike traditional recurrent neural networks (RNNs), transformers utilize self-attention mechanisms to process entire sequences in parallel, significantly improving training efficiency and performance.
One of the most prominent transformer-based models is BERT (Bidirectional Encoder Representations from Transformers). BERT's ability to understand the context of words in both directions has led to significant improvements in various NLP tasks such as sentiment analysis, question answering, and named entity recognition. The model pre-trains on a vast corpus of text and fine-tunes for specific tasks, showcasing its versatility and power.
Beyond NLP, transformers have also impacted fields such as computer vision and speech processing. Vision transformers (ViTs) apply the transformer architecture to image patches, achieving competitive performance with traditional convolutional neural networks (CNNs). In speech processing, transformers have enhanced automatic speech recognition (ASR) and text-to-speech (TTS) systems by capturing long-range dependencies more effectively.
Example: Sentiment Analysis Using BERT in Python
import numpy as np
import pandas as pd
from transformers import BertTokenizer, BertForSequenceClassification
from transformers import Trainer, TrainingArguments
import torch
# Load dataset
data = pd.read_csv('sentiment_data.csv')
train_data = data.sample(frac=0.8, random_state=42)
test_data = data.drop(train_data.index)
# Tokenize data
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
train_encodings = tokenizer(train_data['text'].tolist(), truncation=True, padding=True)
test_encodings = tokenizer(test_data['text'].tolist(), truncation=True, padding=True)
# Create dataset class
class SentimentDataset(torch.utils.data.Dataset):
def __init__(self, encodings, labels):
self.encodings = encodings
self.labels = labels
def __getitem__(self, idx):
item = {key: torch.tensor(val[idx]) for key, val in self.encodings.items()}
item['labels'] = torch.tensor(self.labels[idx])
return item
def __len__(self):
return len(self.labels)
train_dataset = SentimentDataset(train_encodings, train_data['label'].tolist())
test_dataset = SentimentDataset(test_encodings, test_data['label'].tolist())
# Initialize model
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=16,
per_device_eval_batch_size=16,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
logging_steps=10,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=test_dataset
)
# Train model
trainer.train()
In this example, a BERT model is used for sentiment analysis, highlighting how transformer architectures can be applied to various NLP tasks to achieve superior performance.

Graph Neural Networks: Expanding the Frontier of Structured Data
Graph Neural Networks (GNNs) represent another groundbreaking advancement in modern machine learning, particularly for structured data. Unlike traditional neural networks that process data in Euclidean space, GNNs operate on graph-structured data, making them ideal for tasks involving social networks, molecular structures, and recommendation systems.
GNNs work by propagating information between nodes in a graph, allowing them to learn representations that capture the relationships and interactions between different entities. This capability is crucial for applications such as predicting molecular properties, where the arrangement of atoms significantly impacts the molecule's behavior. In social networks, GNNs can identify influential nodes and detect communities based on the connections between users.
One popular variant of GNNs is the Graph Convolutional Network (GCN), which generalizes the concept of convolution to graph data. GCNs aggregate information from a node's neighbors to update its representation, enabling the model to learn hierarchical features. This approach has been highly effective in various domains, demonstrating the versatility and power of GNNs in handling complex structured data.
Improving Image Recognition with Convolutional Neural Networks
Convolutional Neural Networks (CNNs) have long been the cornerstone of advancements in image recognition and computer vision. CNNs utilize convolutional layers to automatically detect patterns and features within images, making them highly effective for tasks such as object detection, image classification, and segmentation.

One significant advancement in CNNs is the introduction of architectures like ResNet (Residual Networks). ResNet addresses the problem of vanishing gradients by introducing skip connections, which allow gradients to flow more effectively through deep networks. This innovation has enabled the development of much deeper networks that achieve state-of-the-art performance on challenging image recognition benchmarks.
Moreover, CNNs have been instrumental in the development of real-time image processing applications. Models like YOLO (You Only Look Once) have made it possible to detect objects in images and videos with high speed and accuracy. These models have found applications in autonomous driving, surveillance, and augmented reality, showcasing the transformative impact of CNNs on various industries.
Example: Image Classification Using ResNet in Python
import torch
import torchvision
import torchvision.transforms as transforms
from torch.utils.data import DataLoader
from torchvision.models import resnet50
# Data preprocessing
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]),
])
# Load dataset
train_dataset = torchvision.datasets.CIFAR10(root='./data', train=True, download=True, transform=transform)
test_dataset = torchvision.datasets.CIFAR10(root='./data', train=False, download=True, transform=transform)
train_loader = DataLoader(train_dataset, batch_size=32, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=32, shuffle=False)
# Initialize model
model = resnet50(pretrained=True)
model.fc = torch.nn.Linear(model.fc.in_features, 10) # Adjust the final layer for CIFAR-10
# Training setup
criterion = torch.nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# Training loop
for epoch in range(10):
model.train()
running_loss = 0.0
for inputs, labels in train_loader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}, Loss: {running_loss / len(train_loader)}")
# Evaluation
model.eval()
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in test_loader:
outputs = model(inputs)
_, predicted = torch.max(outputs, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f"Accuracy: {100 * correct / total}%")
In this example, a ResNet model is used for image classification on the CIFAR-10 dataset, illustrating how CNN architectures can be applied to achieve high performance in image recognition tasks.
Innovations in Reinforcement Learning
Advancing Autonomous Agents with Deep Reinforcement Learning
Reinforcement learning (RL) has seen remarkable progress with the integration of deep learning, leading to the development of deep reinforcement learning (DRL) algorithms. DRL combines the decision-making capabilities of RL with the representational power of deep neural networks, enabling the creation of autonomous agents that can learn complex tasks through trial and error.

One of the most notable DRL algorithms is Deep Q-Learning (DQN), which uses a neural network to approximate the Q-value function. DQN has been successfully applied to a variety of tasks, including playing video games at a superhuman level. The algorithm's ability to learn optimal policies directly from raw pixel data demonstrates the potential of DRL in developing intelligent agents.
Another significant advancement in DRL is Proximal Policy Optimization (PPO), an algorithm that balances exploration and exploitation more effectively than traditional methods. PPO has been used to train agents for continuous control tasks, such as robotic manipulation and autonomous driving, showcasing its versatility and robustness in real-world applications.
Example: Training an Agent Using DQN in Python
import gym
import torch
import torch.nn as nn
import torch.optim as optim
import random
import numpy as np
from collections import deque
# Neural network for DQN
class DQN(nn.Module):
def __init__(self, state_dim, action_dim):
super(DQN, self).__init__()
self.fc1 = nn.Linear(state_dim, 128)
self.fc2 = nn.Linear(128, 128)
self.fc3 = nn.Linear(128, action_dim)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
return self.fc3(x)
# Experience replay buffer
class ReplayBuffer:
def __init__(self, capacity):
self.buffer = deque(maxlen=capacity)
def push(self, state, action, reward, next_state, done):
self.buffer.append((state, action, reward, next_state, done))
def sample(self, batch_size
):
state, action, reward, next_state, done = zip(*random.sample(self.buffer, batch_size))
return np.array(state), np.array(action), np.array(reward), np.array(next_state), np.array(done)
def __len__(self):
return len(self.buffer)
# Training the DQN agent
def train_dqn(env, num_episodes=500, batch_size=64, gamma=0.99, epsilon_start=1.0, epsilon_end=0.01, epsilon_decay=0.995):
state_dim = env.observation_space.shape[0]
action_dim = env.action_space.n
model = DQN(state_dim, action_dim)
target_model = DQN(state_dim, action_dim)
target_model.load_state_dict(model.state_dict())
optimizer = optim.Adam(model.parameters(), lr=0.001)
replay_buffer = ReplayBuffer(10000)
epsilon = epsilon_start
for episode in range(num_episodes):
state = env.reset()
total_reward = 0
done = False
while not done:
if random.random() < epsilon:
action = env.action_space.sample()
else:
action = model(torch.FloatTensor(state)).argmax().item()
next_state, reward, done, _ = env.step(action)
replay_buffer.push(state, action, reward, next_state, done)
state = next_state
total_reward += reward
if len(replay_buffer) >= batch_size:
states, actions, rewards, next_states, dones = replay_buffer.sample(batch_size)
states = torch.FloatTensor(states)
actions = torch.LongTensor(actions)
rewards = torch.FloatTensor(rewards)
next_states = torch.FloatTensor(next_states)
dones = torch.FloatTensor(dones)
q_values = model(states).gather(1, actions.unsqueeze(1)).squeeze(1)
next_q_values = target_model(next_states).max(1)[0]
target_q_values = rewards + gamma * next_q_values * (1 - dones)
loss = nn.MSELoss()(q_values, target_q_values.detach())
optimizer.zero_grad()
loss.backward()
optimizer.step()
if epsilon > epsilon_end:
epsilon *= epsilon_decay
if episode % 10 == 0:
target_model.load_state_dict(model.state_dict())
print(f"Episode {episode}, Total Reward: {total_reward}, Epsilon: {epsilon}")
return model
# Example usage
env = gym.make('CartPole-v1')
trained_model = train_dqn(env)
In this example, a DQN agent is trained to play the CartPole-v1 game using deep reinforcement learning, showcasing the potential of DRL algorithms in developing intelligent agents.
Optimizing Hyperparameters with Bayesian Optimization
Hyperparameter optimization is a critical aspect of machine learning model development, as the choice of hyperparameters can significantly impact model performance. Traditional methods such as grid search and random search can be inefficient and time-consuming, especially for complex models with many hyperparameters. Bayesian optimization offers a more efficient alternative by using a probabilistic model to guide the search for optimal hyperparameters.

Bayesian optimization constructs a surrogate model, typically a Gaussian process, to model the objective function's distribution. It then uses this model to select hyperparameters that are likely to improve performance. This approach allows Bayesian optimization to explore the hyperparameter space more intelligently, focusing on promising regions and converging to the optimal set of hyperparameters more quickly.
Tools like Optuna and Hyperopt provide implementations of Bayesian optimization that are easy to integrate with various machine learning frameworks. These tools have been widely adopted in both academia and industry, demonstrating their effectiveness in optimizing hyperparameters for a wide range of models and applications.
Example: Hyperparameter Optimization Using Optuna in Python
import optuna
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader, TensorDataset
# Define a simple neural network
class SimpleNN(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(input_dim, hidden_dim)
self.fc2 = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Objective function for Optuna
def objective(trial):
# Hyperparameter space
hidden_dim = trial.suggest_int('hidden_dim', 16, 128)
lr = trial.suggest_float('lr', 1e-5, 1e-1, log=True)
# Load dataset
X = torch.randn(1000, 10)
y = torch.randint(0, 2, (1000,))
dataset = TensorDataset(X, y)
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
# Initialize model, criterion, and optimizer
model = SimpleNN(input_dim=10, hidden_dim=hidden_dim, output_dim=2)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=lr)
# Training loop
for epoch in range(10):
for inputs, labels in dataloader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# Evaluate model
with torch.no_grad():
X_test = torch.randn(200, 10)
y_test = torch.randint(0, 2, (200,))
outputs = model(X_test)
preds = torch.argmax(outputs, dim=1)
accuracy = (preds == y_test).float().mean().item()
return accuracy
# Optimize hyperparameters
study = optuna.create_study(direction='maximize')
study.optimize(objective, n_trials=50)
print(f"Best trial: {study.best_trial.value}")
print(f"Best hyperparameters: {study.best_trial.params}")
In this example, Optuna is used to optimize the hyperparameters of a simple neural network, illustrating how Bayesian optimization can efficiently search for the best hyperparameters to enhance model performance.
Real-World Applications of Modern Machine Learning Models
Enhancing Healthcare with Predictive Analytics
Modern machine learning models have significantly advanced the field of healthcare, enabling more accurate diagnostics, personalized treatment plans, and improved patient outcomes. Predictive analytics, powered by machine learning, allows healthcare providers to identify at-risk patients, predict disease progression, and optimize treatment strategies.

For instance, machine learning models can analyze electronic health records (EHRs) to predict the likelihood of readmission, allowing healthcare providers to take preventive measures. Models like random forests and gradient boosting machines are commonly used for these tasks due to their ability to handle large datasets and capture complex interactions between variables.
Moreover, deep learning models have been employed in medical imaging to detect diseases such as cancer and pneumonia with high accuracy. Convolutional neural networks (CNNs) can analyze X-rays, MRIs, and CT scans to identify anomalies that may indicate the presence of a disease. These models assist radiologists in making faster and more accurate diagnoses, ultimately improving patient care.
Example: Disease Prediction Using Random Forest in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
data = pd.read_csv('healthcare_data.csv')
X = data.drop('disease', axis=1)
y = data['disease']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
In this example, a random forest classifier is trained to predict diseases based on healthcare data, demonstrating the application of machine learning in improving healthcare outcomes.
Revolutionizing Finance with Algorithmic Trading
The finance industry has been transformed by the adoption of machine learning models, particularly in the realm of algorithmic trading. These models can analyze vast amounts of financial data to identify patterns and make trading decisions in real-time, often outperforming human traders.

Reinforcement learning has been particularly impactful in algorithmic trading, where agents learn to make optimal trading decisions through interactions with the market environment. Techniques such as Q-learning and Deep Q-Networks (DQNs) have been employed to develop trading algorithms that maximize returns while managing risk.
Additionally, machine learning models are used for credit scoring and fraud detection in finance. By analyzing transaction data, these models can identify fraudulent activities and assess the creditworthiness of individuals and businesses. This capability enhances the security and reliability of financial systems, benefiting both institutions and customers.
Enhancing Marketing with Personalization
Machine learning has also revolutionized marketing by enabling personalized customer experiences. By analyzing customer data, machine learning models can predict individual preferences and tailor marketing efforts accordingly. This personalization improves customer engagement and increases conversion rates.
Collaborative filtering and content-based filtering are commonly used techniques for recommendation systems. Collaborative filtering leverages user behavior data to recommend products or services that similar users have liked. Content-based filtering, on the other hand, uses information about the items themselves to make recommendations based on user preferences.
Moreover, natural language processing (NLP) is used to analyze customer reviews and social media posts, providing insights into customer sentiment and preferences. These insights help businesses understand their customers better and refine their marketing strategies to meet customer needs more effectively.
Example: Building a Recommendation System Using Collaborative Filtering in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.metrics.pairwise import cosine_similarity
from scipy.sparse import csr_matrix
# Load dataset
data = pd.read_csv('ratings.csv')
user_item_matrix = data.pivot(index='user_id', columns='item_id', values='rating').fillna(0)
user_item_matrix_sparse = csr_matrix(user_item_matrix.values)
# Compute cosine similarity between users
user_similarity = cosine_similarity(user_item_matrix_sparse)
# Function to recommend items for a given user
def recommend_items(user_id, user_item_matrix, user_similarity, top_n=5):
user_index = user_item_matrix.index.get_loc(user_id)
similarity_scores = user_similarity[user_index]
user_ratings = user_item_matrix.iloc[user_index]
unrated_items = user_ratings[user_ratings == 0].index
scores = user_item_matrix[unrated_items].dot(similarity_scores)
recommended_items = scores.nlargest(top_n).index
return recommended_items
# Example recommendation
recommended_items = recommend_items(1, user_item_matrix, user_similarity)
print(f'Recommended items for user 1: {recommended_items}')
In this example, a recommendation system using collaborative filtering is built to suggest items for users based on their similarity to other users, showcasing the application of machine learning in personalized marketing.
Modern machine learning models have achieved remarkable breakthroughs, transforming industries such as healthcare, finance, and marketing. From advanced deep learning architectures like transformers and GNNs to innovative reinforcement learning algorithms and hyperparameter optimization techniques, these models continue to push the boundaries of what is possible. By exploring and leveraging these cutting-edge advancements, businesses and researchers can harness the full potential of machine learning to drive progress and innovation across various domains.
If you want to read more articles similar to Exploring the Latest Breakthroughs in Modern Machine Learning Models, you can visit the Artificial Intelligence category.
You Must Read