
Exploring the Potential of Machine Learning in R: Can It Be Done?
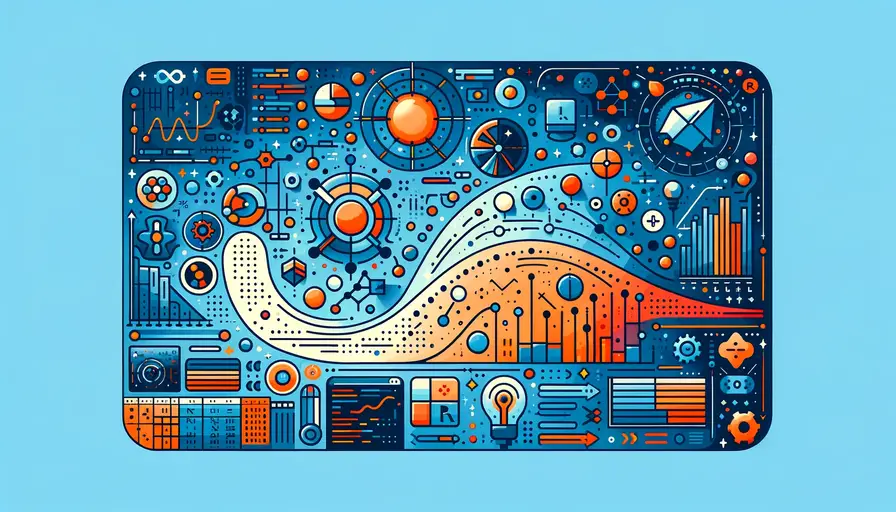
Machine learning has become a cornerstone of modern data analysis, providing powerful tools for prediction, classification, and pattern recognition. While Python often dominates the conversation around machine learning, R, a language traditionally associated with statistical computing, also offers robust capabilities for machine learning.
Content
The Power of R in Machine Learning
Why Choose R for Machine Learning?
R is a language and environment designed for statistical computing and graphics, making it inherently suitable for data analysis tasks. One of the key advantages of using R for machine learning is its extensive collection of packages and libraries specifically developed for statistical modeling and machine learning. These packages provide a rich ecosystem for data preprocessing, model training, and evaluation.
Additionally, R's strong data visualization capabilities allow for in-depth exploratory data analysis (EDA), which is crucial for understanding data patterns and relationships before applying machine learning models. R's integration with other tools and languages, such as SQL and Python, further enhances its versatility in data science workflows.
R also has a vibrant community and extensive documentation, which can be invaluable for learning and troubleshooting. The Comprehensive R Archive Network (CRAN) hosts numerous packages that extend R's functionality, making it a powerful tool for machine learning.
Exploring the Role of Machine Learning in Facial Expression EvaluationKey Machine Learning Packages in R
Several packages in R are specifically designed for machine learning, offering a wide range of algorithms and utilities. Some of the most popular packages include:
- caret: The Classification and Regression Training package is a comprehensive package that streamlines the process of model training and tuning. It provides a unified interface for numerous machine learning algorithms and includes tools for data preprocessing, feature selection, and model evaluation.
- randomForest: This package implements the random forest algorithm, a powerful ensemble method for classification and regression tasks. It is known for its robustness and ability to handle large datasets.
- e1071: This package includes functions for Support Vector Machines (SVMs), among other algorithms. SVMs are widely used for classification and regression tasks.
- xgboost: An implementation of gradient boosting, this package is known for its high performance and efficiency in handling large-scale datasets.
- nnet: This package provides functions for training neural networks, allowing for the implementation of deep learning models within R.
Example of installing key machine learning packages in R:
# Install key machine learning packages
install.packages(c("caret", "randomForest", "e1071", "xgboost", "nnet"))
Data Visualization and Preprocessing in R
R's data visualization capabilities are unmatched, thanks to packages like ggplot2 and plotly. These tools allow for the creation of detailed and interactive visualizations that can uncover insights and guide model development. Preprocessing is also a critical step in any machine learning workflow, and R provides a suite of tools for cleaning, transforming, and preparing data.
Data visualization example using ggplot2:
Applications of Machine Learning for Predicting X and Y# Load necessary libraries
library(ggplot2)
library(datasets)
# Load the iris dataset
data(iris)
# Create a scatter plot
ggplot(iris, aes(x = Sepal.Length, y = Petal.Length, color = Species)) +
geom_point() +
labs(title = "Sepal Length vs Petal Length by Species",
x = "Sepal Length",
y = "Petal Length")
Data preprocessing example using dplyr:
# Load necessary libraries
library(dplyr)
# Load the iris dataset
data(iris)
# Preprocess the data: filter, mutate, and select
iris_processed <- iris %>%
filter(Sepal.Length > 5) %>%
mutate(Petal.Area = Petal.Length * Petal.Width) %>%
select(Sepal.Length, Petal.Area, Species)
# View the processed data
head(iris_processed)
Implementing Machine Learning Models in R
Supervised Learning with caret
The caret package is a cornerstone for machine learning in R, providing a streamlined workflow for model training, tuning, and evaluation. It supports a wide range of algorithms and offers tools for cross-validation, feature selection, and performance assessment.
Example of implementing a decision tree classifier with caret:
# Load necessary libraries
library(caret)
library(datasets)
# Load the iris dataset
data(iris)
# Split the data into training and testing sets
set.seed(123)
trainIndex <- createDataPartition(iris$Species, p = .8,
list = FALSE,
times = 1)
irisTrain <- iris[trainIndex,]
irisTest <- iris[-trainIndex,]
# Train a decision tree model
model <- train(Species ~ ., data = irisTrain, method = "rpart")
# Make predictions on the test set
predictions <- predict(model, newdata = irisTest)
# Evaluate the model
confusionMatrix(predictions, irisTest$Species)
Ensemble Methods with randomForest
randomForest is a powerful package for implementing random forest models, an ensemble learning method that is effective for both classification and regression tasks. Random forests are known for their robustness and ability to handle large datasets with high-dimensional features.
Enhancing Data Mining Techniques with Machine Learning and AIExample of implementing a random forest classifier with randomForest:
# Load necessary libraries
library(randomForest)
library(datasets)
# Load the iris dataset
data(iris)
# Train a random forest model
set.seed(123)
model <- randomForest(Species ~ ., data = iris, ntree = 100)
# Make predictions on the training set
predictions <- predict(model, iris)
# Evaluate the model
confusionMatrix(predictions, iris$Species)
Support Vector Machines with e1071
The e1071 package provides an implementation of Support Vector Machines (SVMs), a popular and effective method for classification and regression tasks. SVMs are particularly useful for problems with high-dimensional feature spaces.
Example of implementing an SVM classifier with e1071:
# Load necessary libraries
library(e1071)
library(datasets)
# Load the iris dataset
data(iris)
# Split the data into training and testing sets
set.seed(123)
trainIndex <- createDataPartition(iris$Species, p = .8,
list = FALSE,
times = 1)
irisTrain <- iris[trainIndex,]
irisTest <- iris[-trainIndex,]
# Train an SVM model
model <- svm(Species ~ ., data = irisTrain, kernel = "linear")
# Make predictions on the test set
predictions <- predict(model, newdata = irisTest)
# Evaluate the model
confusionMatrix(predictions, irisTest$Species)
Advanced Machine Learning with R
Gradient Boosting with xgboost
xgboost is an implementation of gradient boosting that is known for its speed and performance. It is widely used in competitive machine learning due to its efficiency and accuracy in handling large-scale datasets.
Exploring Road-Related Machine Learning DatasetsExample of implementing a gradient boosting model with xgboost:
# Load necessary libraries
library(xgboost)
library(datasets)
# Load the iris dataset
data(iris)
# Prepare the data for xgboost
set.seed(123)
trainIndex <- createDataPartition(iris$Species, p = .8,
list = FALSE,
times = 1)
irisTrain <- iris[trainIndex,]
irisTest <- iris[-trainIndex,]
# Convert the data to matrix format
train_matrix <- xgb.DMatrix(data.matrix(irisTrain[, -5]), label = as.numeric(irisTrain$Species) - 1)
test_matrix <- xgb.DMatrix(data.matrix(irisTest[, -5]), label = as.numeric(irisTest$Species) - 1)
# Define parameters for xgboost
params <- list(
objective = "multi:softprob",
num_class = 3,
eval_metric = "mlogloss"
)
# Train the model
model <- xgboost(data = train_matrix, params = params, nrounds = 100, verbose = FALSE)
# Make predictions
preds <- predict(model, test_matrix)
pred_labels <- max.col(matrix(preds, ncol = 3)) - 1
# Evaluate the model
confusionMatrix(as.factor(pred_labels), irisTest$Species)
Neural Networks with nnet
nnet is a package for training neural networks in R. Although not as advanced as some deep learning frameworks, it provides a straightforward way to implement basic neural networks for classification and regression tasks.
Example of implementing a neural network with nnet:
# Load necessary libraries
library(nnet)
library(datasets)
# Load the iris dataset
data(iris)
# Train a neural network model
set.seed(123)
model <- nnet(Species ~ ., data = iris, size = 5, maxit = 200)
# Make predictions on the training set
predictions <- predict(model, iris, type = "class")
# Evaluate the model
confusionMatrix(predictions, iris$Species)
Deep Learning with keras
For more advanced deep learning tasks, R provides the keras package, which is an interface to the Keras deep learning library. This package allows R users to build and train deep learning models with the flexibility of Keras and TensorFlow.
Enhancing Empirical Asset Pricing with Machine LearningExample of implementing a deep learning model with keras:
# Load necessary libraries
library(keras)
library(datasets)
# Load the iris dataset
data(iris)
# Prepare the data for keras
iris$Species <- as.numeric(iris$Species) - 1
X <- as.matrix(iris[, -5])
y <- to_categorical(iris$Species)
# Split the data into training and testing sets
set.seed(123)
trainIndex <- createDataPartition(iris$Species, p = .8, list = FALSE)
X_train <- X[trainIndex,]
y_train <- y[trainIndex,]
X_test <- X[-trainIndex,]
y_test <- y[-trainIndex,]
# Define the model
model <- keras_model_sequential() %>%
layer_dense(units = 128, activation = 'relu', input_shape = ncol(X_train)) %>%
layer_dropout(rate = 0.2) %>%
layer_dense(units = 3, activation = 'softmax')
# Compile the model
model %>% compile(
loss = 'categorical_crossentropy',
optimizer = 'adam',
metrics = 'accuracy'
)
# Train the model
model %>% fit(X_train, y_train, epochs = 50, batch_size = 32, validation_split = 0.2)
# Evaluate the model
scores <- model %>% evaluate(X_test, y_test)
print(scores)
The exploration of machine learning in R reveals that the language offers robust and comprehensive tools for implementing various machine learning algorithms. From supervised learning to advanced techniques like gradient boosting and neural networks, R provides a versatile environment for data scientists and statisticians. With its strong data visualization capabilities, extensive package ecosystem, and integration with other tools, R proves to be a powerful tool for machine learning, showcasing that it can indeed be done effectively.
If you want to read more articles similar to Exploring the Potential of Machine Learning in R: Can It Be Done?, you can visit the Applications category.
You Must Read