
Is Python the Primary Programming Language for Machine Learning?
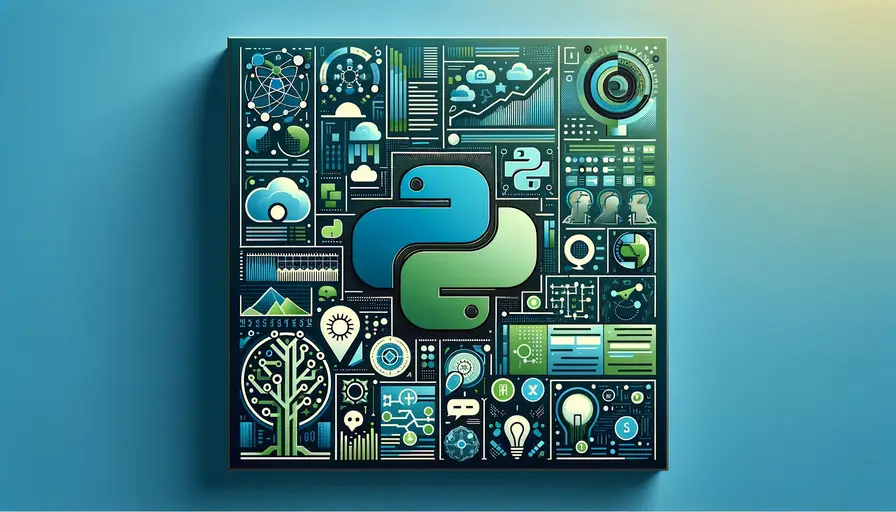
Python as the Primary Language
Why Python is the Primary Language
Python is widely considered the primary programming language for machine learning due to its simplicity, readability, and vast ecosystem of libraries and frameworks. These features make it an ideal choice for both beginners and experienced developers. Python's syntax is clear and concise, allowing users to write and understand code quickly and efficiently. This ease of use is crucial for complex fields like machine learning, where the focus should be on solving problems rather than struggling with language intricacies.
Additionally, Python's flexibility allows it to integrate with other languages and tools, making it a versatile option for various machine learning tasks. It can be used for data preprocessing, model training, and deployment, providing a comprehensive environment for machine learning projects.
Integration with Other Tools
Integration capabilities of Python further solidify its position as the primary language for machine learning. Python can easily interface with other programming languages such as C++, Java, and R, allowing developers to utilize the best tools available for their specific tasks. This interoperability ensures that Python can be a part of any machine learning pipeline, enhancing its utility and adaptability.
Moreover, Python's compatibility with popular development tools and platforms, such as Jupyter Notebooks, Anaconda, and Google Colab, makes it even more appealing. These tools provide interactive environments that facilitate experimentation, visualization, and collaboration, essential components of the machine learning workflow.
Predicting Categorical Variables with Linear RegressionPython's Ecosystem
The extensive ecosystem of libraries and frameworks available in Python makes it a powerhouse for machine learning. Libraries such as NumPy, Pandas, and SciPy provide robust tools for data manipulation and analysis, forming the foundation for machine learning tasks. This rich ecosystem allows developers to find and utilize the right tools for every stage of their machine learning projects, from data preprocessing to model deployment.
Here's an example of a simple machine learning model using Python's Scikit-learn library:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train the model
model = RandomForestClassifier(n_estimators=100)
model.fit(X_train, y_train)
# Predict
predictions = model.predict(X_test)
print("Accuracy:", accuracy_score(y_test, predictions))
This code demonstrates how to implement a Random Forest classifier in Python.
Extensive Libraries and Frameworks
TensorFlow and PyTorch
TensorFlow and PyTorch are two of the most popular deep learning frameworks available in Python. TensorFlow, developed by Google, provides a comprehensive ecosystem for developing machine learning models, including tools for deployment, optimization, and scalability. Its flexible architecture allows users to deploy models on various platforms, from servers to edge devices.
Major Players in Machine Learning Group Data ProvidersPyTorch, developed by Facebook, is known for its dynamic computational graph and ease of use, making it a favorite among researchers and developers for rapid prototyping and experimentation. PyTorch's integration with Python's native libraries ensures a smooth development experience, facilitating the creation of complex models with minimal code.
Other Essential Libraries
Other essential libraries include Scikit-learn, Keras, and XGBoost, each offering unique features and capabilities. Scikit-learn provides simple and efficient tools for data mining and analysis, covering various machine learning algorithms and utilities. Keras, now part of TensorFlow, offers a high-level API for building and training neural networks, simplifying the development process.
XGBoost, a powerful gradient boosting library, is widely used for its performance and efficiency in handling large datasets. These libraries, combined with Python's versatility, make it an indispensable tool for machine learning practitioners.
Here's an example of a simple neural network model using TensorFlow:
The Top Machine Learning Resources on Fresco Play for Learning Rimport tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Build the model
model = Sequential()
model.add(Dense(10, input_shape=(4,), activation='relu'))
model.add(Dense(3, activation='softmax'))
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=50, batch_size=5, verbose=1)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print("Accuracy:", accuracy)
This code demonstrates how to build and train a simple neural network using TensorFlow.
Simplicity and Readability
Ease of Learning
Python's simplicity and readability make it an excellent choice for beginners in machine learning. The language's straightforward syntax reduces the learning curve, allowing new developers to quickly grasp core concepts and start building models. This accessibility is crucial in a field that can be as complex and demanding as machine learning.
Python's readability also promotes better collaboration and maintenance of code. Clear and concise code is easier to review, debug, and extend, making Python a preferred language for team projects and long-term development.
Here's an example of a simple linear regression model using Python:
Is Learning Machine Learning Worth It for Beginners?import numpy as np
from sklearn.linear_model import LinearRegression
import matplotlib.pyplot as plt
# Generate some data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.5, 3.0, 4.5, 6.0, 7.5])
# Create and train the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict(X)
# Plot the results
plt.scatter(X, y, color='blue')
plt.plot(X, predictions, color='red')
plt.xlabel('X')
plt.ylabel('y')
plt.title('Simple Linear Regression')
plt.show()
This code demonstrates how to implement and visualize a simple linear regression model in Python.
Readability and Maintainability
Readability and maintainability are critical factors in software development. Python's clear syntax and extensive use of indentation ensure that code is easy to read and understand. This readability reduces the likelihood of errors and makes it easier for developers to collaborate and share code.
Moreover, Python's comprehensive documentation and strong community support provide ample resources for learning and troubleshooting, further enhancing its appeal as the primary language for machine learning.
Strong Community Support
Community Benefits
Python's strong community support is a significant advantage for machine learning practitioners. The Python community is active and extensive, offering a wealth of resources such as tutorials, forums, and libraries. This support network makes it easier for beginners to find help and for experienced developers to share knowledge and collaborate on projects.
Strategies to Safeguard Machine Learning Models from TheftThe community-driven nature of Python ensures that the language and its libraries are continuously updated and improved, keeping pace with the latest advancements in machine learning. This collective effort contributes to the robustness and reliability of Python as a programming language.
Finding Help
For instance, if you're encountering an issue with your machine learning code, you can easily find solutions on platforms like Stack Overflow, GitHub, and dedicated machine learning forums.
Here's a simple example of seeking help on Stack Overflow:
# Question posted on Stack Overflow
"""
I'm trying to fit a linear regression model using Scikit-learn, but I'm getting an error. Here is my code:
import numpy as np
from sklearn.linear_model import LinearRegression
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.5, 3.0, 4.5, 6.0, 7.5])
model = LinearRegression()
model.fit(X, y)
predictions = model.predict(X)
print(predictions)
"""
# A helpful response might include checking the shapes of X and y
"""
Make sure that X and y are properly shaped. Your code seems fine, but you might want to ensure that y is a 1D array.
"""
This example shows how you can get community support for common issues.
Effective Data Cleaning Techniques for Machine Learning on edXLearning Resources
Learning resources are abundant within the Python community. Numerous free and paid courses, tutorials, and documentation are available to help developers at all levels. Websites like Coursera, edX, and Udacity offer comprehensive machine learning courses in Python, while platforms like GitHub provide repositories of code examples and projects.
This extensive array of resources ensures that anyone can start learning machine learning with Python, regardless of their prior experience.
Python's Popularity and Ecosystem
Popularity Factors
Python's popularity in the machine learning community is driven by its ease of use, extensive libraries, and strong community support. These factors make Python a natural choice for many developers, enabling rapid development and prototyping.
The language's popularity also means that there is a wealth of job opportunities and career paths available for those skilled in Python. This demand further reinforces its position as the primary language for machine learning.
Career Opportunities
For example, job postings for data scientists, machine learning engineers, and AI specialists often list Python as a required or preferred skill. The demand for Python expertise ensures that professionals have numerous opportunities to apply their skills in various industries.
Ecosystem Advantages
The Python ecosystem offers a comprehensive set of tools and libraries that support the entire machine learning workflow. From data collection and preprocessing to model training and deployment, Python provides the necessary resources to streamline each stage of development.
Libraries like Pandas and NumPy facilitate data manipulation and analysis, while frameworks like TensorFlow and PyTorch enable complex model building and training. This integrated ecosystem makes Python a one-stop solution for machine learning projects.
Python's Simplicity for Beginners
Learning Curve
Python's simplicity and readability make it an excellent choice for beginners in machine learning. The language's straightforward syntax reduces the learning curve, allowing new developers to quickly grasp core concepts and start building models. This accessibility is crucial in a field that can be as complex and demanding as machine learning.
Python's readability also promotes better collaboration and maintenance of code. Clear and concise code is easier to review, debug, and extend, making Python a preferred language for team projects and long-term development.
Here's an example of a simple linear regression model using Python:
import numpy as np
from sklearn.linear_model import LinearRegression
import matplotlib.pyplot as plt
# Generate some data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.5, 3.0, 4.5, 6.0, 7.5])
# Create and train the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict(X)
# Plot the results
plt.scatter(X, y, color='blue')
plt.plot(X, predictions, color='red')
plt.xlabel('X')
plt.ylabel('y')
plt.title('Simple Linear Regression')
plt.show()
This code demonstrates how to implement and visualize a simple linear regression model in Python.
Readability and Maintainability
Readability and maintainability are critical factors in software development. Python's clear syntax and extensive use of indentation ensure that code is easy to read and understand. This readability reduces the likelihood of errors and makes it easier for developers to collaborate and share code.
Moreover, Python's comprehensive documentation and strong community support provide ample resources for learning and troubleshooting, further enhancing its appeal as the primary language for machine learning.
Python is widely considered the primary programming language for machine learning due to its simplicity, extensive libraries, and strong community support. Its ability to handle complex and large datasets, coupled with its flexibility and integration capabilities, makes it an ideal choice for both beginners and experienced developers. Python's popularity and comprehensive ecosystem ensure that it remains a top choice for machine learning projects, providing the necessary tools and resources for success in this rapidly evolving field.
If you want to read more articles similar to Is Python the Primary Programming Language for Machine Learning?, you can visit the Education category.
You Must Read