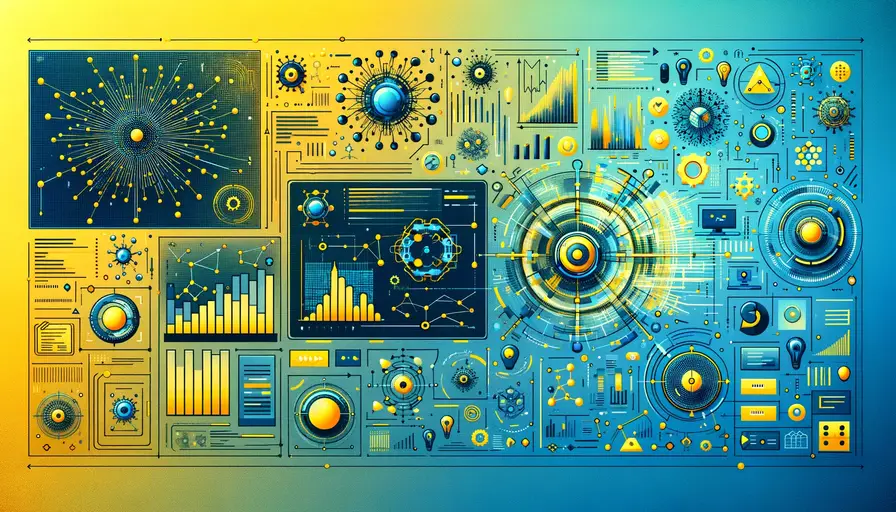
Key Skills for Machine Learning Specialists: A Complete Guide

- Machine Learning Skills
- Programming Skills
- Mathematics and Statistics
- Data Manipulation and Preprocessing
- Understanding Machine Learning Algorithms
- Model Evaluation and Validation
- Big Data Technologies
- Soft Skills for Machine Learning Specialists
- Continuous Learning and Adaptation
- Ethical Considerations in Machine Learning
Machine Learning Skills
The field of machine learning (ML) is rapidly evolving, and the demand for skilled professionals is higher than ever. To excel as a machine learning specialist, one must possess a diverse set of skills ranging from programming and mathematics to data analysis and model evaluation. This guide provides a comprehensive overview of the essential skills required to thrive in this dynamic field.
The Importance of Machine Learning Skills
Machine learning skills are crucial because they enable professionals to develop algorithms that can learn from and make predictions based on data. These skills are applicable in various industries, including finance, healthcare, and technology, where data-driven decisions can significantly impact outcomes.
Core Skills Overview
Core skills for machine learning specialists include programming, mathematics, data manipulation, and understanding of machine learning algorithms. Additionally, soft skills such as problem-solving and communication are equally important to convey complex concepts to non-technical stakeholders.
Example: Core Skills in Action
Here’s an example of how these core skills come together in a real-world ML project using Python:

import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load dataset
data = pd.read_csv('data.csv')
# Data preprocessing
data.fillna(0, inplace=True)
# Split data into training and testing sets
X = data.drop('target', axis=1)
y = data['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Evaluate the model
predictions = model.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
print(f'Accuracy: {accuracy}')
Programming Skills
Programming is a fundamental skill for any machine learning specialist. Proficiency in programming languages like Python, R, and Java allows you to implement ML algorithms, manipulate data, and develop scalable solutions.
Python for Machine Learning
Python is the most widely used language in machine learning due to its simplicity and the extensive range of libraries available, such as NumPy, pandas, and scikit-learn. Python’s versatility makes it an essential tool for both beginners and experts.
R for Statistical Analysis
R is another popular language, particularly in academic and research settings. It excels in statistical analysis and visualization, making it a valuable asset for machine learning tasks that require detailed data exploration and statistical modeling.
Example: Python vs. R for Data Analysis
Here’s an example of performing data analysis in both Python and R:

# Python
import pandas as pd
# Load dataset
data = pd.read_csv('data.csv')
# Basic statistics
print(data.describe())
# R
data <- read.csv('data.csv')
# Basic statistics
summary(data)
Mathematics and Statistics
A solid understanding of mathematics and statistics is crucial for machine learning. Concepts such as linear algebra, calculus, probability, and statistics form the foundation upon which ML algorithms are built.
Linear Algebra and Calculus
Linear algebra and calculus are essential for understanding the inner workings of machine learning algorithms. They are used in optimization problems, where the goal is to minimize or maximize a function.
Probability and Statistics
Probability and statistics are vital for making inferences about data, assessing model performance, and understanding the likelihood of events. These concepts help in developing robust models that generalize well to new data.
Example: Calculating Descriptive Statistics
Here’s an example of calculating descriptive statistics using Python:

import pandas as pd
# Load dataset
data = pd.read_csv('data.csv')
# Calculate mean, median, and standard deviation
mean_value = data['feature'].mean()
median_value = data['feature'].median()
std_dev_value = data['feature'].std()
print(f'Mean: {mean_value}, Median: {median_value}, Standard Deviation: {std_dev_value}')
Data Manipulation and Preprocessing
Effective data manipulation and preprocessing are critical for building high-quality machine learning models. This process involves cleaning data, handling missing values, and transforming features to ensure that the data is suitable for modeling.
Data Cleaning
Data cleaning involves removing or correcting inaccurate records from a dataset. This step is crucial for improving the quality of data and the accuracy of the resulting model.
Handling Missing Values
Handling missing values can be done by imputing data, removing records, or using algorithms that can handle missing data inherently. Proper handling ensures that the model is not biased or skewed.
Example: Data Cleaning and Imputation
Here’s an example of data cleaning and imputation using Python:

import pandas as pd
from sklearn.impute import SimpleImputer
# Load dataset
data = pd.read_csv('data.csv')
# Impute missing values with the mean
imputer = SimpleImputer(strategy='mean')
data_imputed = imputer.fit_transform(data)
# Convert back to DataFrame
data_clean = pd.DataFrame(data_imputed, columns=data.columns)
print(data_clean.head())
Understanding Machine Learning Algorithms
Knowledge of various machine learning algorithms and their applications is essential for selecting the right model for a given problem. Familiarity with supervised, unsupervised, and reinforcement learning techniques allows for a comprehensive approach to different types of data and tasks.
Supervised Learning
Supervised learning involves training a model on labeled data. Common algorithms include linear regression, decision trees, and support vector machines. These algorithms are used for tasks like classification and regression.
Unsupervised Learning
Unsupervised learning deals with unlabeled data. Algorithms such as k-means clustering and principal component analysis (PCA) are used to find hidden patterns and groupings within the data.
Example: Supervised vs. Unsupervised Learning
Here’s an example of implementing both supervised and unsupervised learning in Python:

# Supervised Learning: Logistic Regression
from sklearn.linear_model import LogisticRegression
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Train model
model = LogisticRegression()
model.fit(X, y)
# Unsupervised Learning: K-means Clustering
from sklearn.cluster import KMeans
# Load dataset
data = pd.read_csv('data.csv')
# Apply K-means
kmeans = KMeans(n_clusters=3)
kmeans.fit(data)
# Get cluster labels
labels = kmeans.labels_
print(labels)
Model Evaluation and Validation
Evaluating and validating machine learning models is critical for ensuring their performance and generalizability. Techniques such as cross-validation, confusion matrices, and ROC curves help in assessing the accuracy and robustness of models.
Cross-Validation
Cross-validation is used to assess how well a model generalizes to an independent dataset. It involves partitioning the data into training and testing sets multiple times to ensure that the model performs well across different subsets of data.
Confusion Matrix and ROC Curves
A confusion matrix provides a summary of prediction results on a classification problem, while ROC curves (Receiver Operating Characteristic curves) illustrate the diagnostic ability of a binary classifier. These tools are essential for evaluating the performance of classification models.
Example: Cross-Validation and Model Evaluation
Here’s an example of using cross-validation and evaluating a model in Python:

from sklearn.model_selection import cross_val_score
from sklearn.metrics import confusion_matrix, roc_curve, auc
import matplotlib.pyplot as plt
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Train model with cross-validation
model = LogisticRegression()
cv_scores = cross_val_score(model, X, y, cv=5)
print(f'Cross-validation scores: {cv_scores}')
# Evaluate model with confusion matrix and ROC curve
model.fit(X, y)
predictions = model.predict(X)
conf_matrix = confusion_matrix(y, predictions)
fpr, tpr, thresholds = roc_curve(y, model.predict_proba(X)[:,1])
roc_auc = auc(fpr, tpr)
# Plot ROC curve
plt.figure()
plt.plot(fpr, tpr, color='blue', label=f'ROC curve (area = {roc_auc:0.2f})')
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver Operating Characteristic')
plt.legend(loc="lower right")
plt.show()
Big Data Technologies
Proficiency in big data technologies is increasingly important as datasets grow in size and complexity. Tools such as Hadoop, Spark, and databases like SQL are essential for managing and processing large volumes of data efficiently.
Hadoop and Spark
Hadoop and Spark are frameworks for distributed storage and processing of large datasets. Hadoop provides a scalable storage system, while Spark offers fast, in-memory processing capabilities, making it ideal for iterative machine learning tasks.
SQL for Data Management
SQL (Structured Query Language) is essential for querying and managing relational databases. It enables efficient data retrieval and manipulation, which is crucial for preparing data for machine learning models.
Example: Using Spark for Data Processing
Here’s an example of using PySpark to process data:
from pyspark.sql import SparkSession
# Initialize Spark session
spark = SparkSession.builder.appName("MLExample").getOrCreate()
# Load dataset
data = spark.read.csv('data.csv', header=True, inferSchema=True)
# Show dataset
data.show()
# Perform a simple transformation
data_filtered = data.filter(data['feature'] > 10)
data_filtered.show()
Soft Skills for Machine Learning Specialists
In addition to technical skills, soft skills such as communication, problem-solving, and collaboration are vital for machine learning specialists. These skills help in effectively conveying complex ideas, working within teams, and addressing challenges creatively.
Communication Skills
Communication skills are crucial for explaining machine learning concepts and results to non-technical stakeholders. Clear and concise communication ensures that the value of ML projects is understood and appreciated across the organization.
Problem-Solving Abilities
Problem-solving abilities enable machine learning specialists to tackle complex challenges and find innovative solutions. This skill involves critical thinking and the ability to approach problems methodically.
Example: Communicating ML Results
Here’s an example of summarizing machine learning results for a non-technical audience:
# Summary of results
accuracy = 0.85
precision = 0.80
recall = 0.75
# Communicate results
summary = f"""
The machine learning model achieved an accuracy of {accuracy*100:.2f}%,
with a precision of {precision*100:.2f}% and a recall of {recall*100:.2f}%.
These metrics indicate that the model performs well in distinguishing between
positive and negative classes, making it suitable for our use case.
"""
print(summary)
Continuous Learning and Adaptation
The field of machine learning is constantly evolving, and continuous learning is essential for staying current with the latest advancements. Engaging with academic research, attending conferences, and participating in online courses are all effective ways to stay updated.
Engaging with the ML Community
Engaging with the ML community through forums, conferences, and social media platforms like Kaggle, Reddit, and GitHub can provide valuable insights and opportunities for collaboration.
Keeping Up with Research
Keeping up with research involves reading academic papers, attending webinars, and participating in workshops. Websites like arXiv and Google Scholar are excellent resources for accessing the latest research in machine learning.
Example: Exploring Kaggle Competitions
Here’s an example of how to get started with a Kaggle competition:
import kaggle
from kaggle.api.kaggle_api_extended import KaggleApi
# Initialize Kaggle API
api = KaggleApi()
api.authenticate()
# Download dataset from a competition
api.competition_download_files('titanic')
# Load dataset
import pandas as pd
data = pd.read_csv('titanic/train.csv')
print(data.head())
Ethical Considerations in Machine Learning
Ethical considerations are increasingly important in the field of machine learning. Issues such as bias, fairness, and transparency must be addressed to ensure that ML models are used responsibly and ethically.
Addressing Bias and Fairness
Addressing bias and fairness involves ensuring that ML models do not inadvertently perpetuate or amplify existing biases. This requires careful selection of training data, evaluation of model performance across different demographic groups, and implementing fairness constraints.
Transparency and Accountability
Transparency and accountability are crucial for building trust in machine learning models. Making models interpretable and explaining their decisions helps stakeholders understand how predictions are made, ensuring that the models are used appropriately.
Example: Evaluating Model Fairness
Here’s an example of evaluating model fairness using Python:
from sklearn.metrics import classification_report
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Train model
model = LogisticRegression()
model.fit(X, y)
# Evaluate model performance
predictions = model.predict(X)
report = classification_report(y, predictions, target_names=['Class 0', 'Class 1'], output_dict=True)
# Assess fairness across different groups
group_0_performance = report['Class 0']
group_1_performance = report['Class 1']
print(f'Performance for Class 0: {group_0_performance}')
print(f'Performance for Class 1: {group_1_performance}')
Becoming a proficient machine learning specialist requires mastering a diverse set of skills. From programming and mathematics to data manipulation and ethical considerations, each skill plays a vital role in developing robust and reliable ML models. Continuous learning, effective communication, and engagement with the ML community are also essential for staying current in this rapidly evolving field. By understanding and honing these skills, aspiring machine learning specialists can build successful careers and contribute meaningfully to the advancement of technology.
If you want to read more articles similar to Key Skills for Machine Learning Specialists: A Complete Guide, you can visit the Education category.
You Must Read