
Logistic Regression for Categorical Variables in Machine Learning
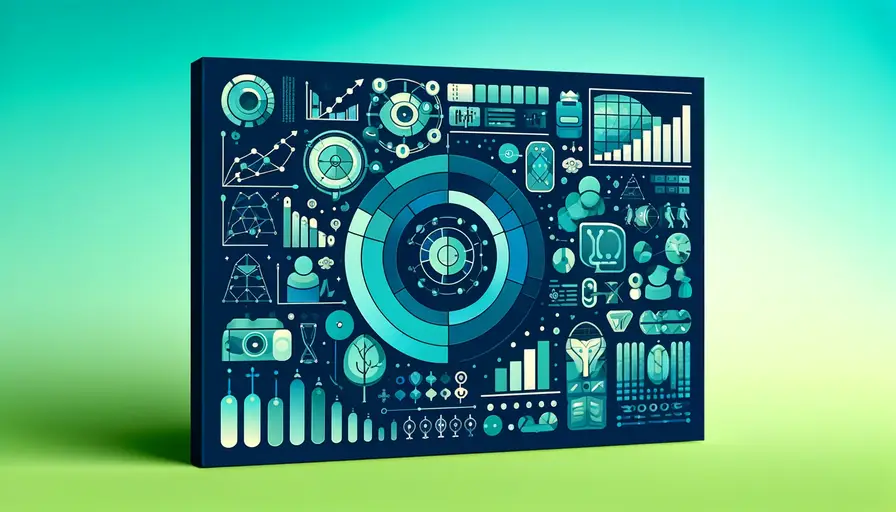
Content
- Use One-Hot Encoding to Convert Categorical Variables
- Apply Feature Scaling to Ensure Variables Are on the Same Scale
- Regularize the Logistic Regression Model
- Perform Cross-Validation to Choose Hyperparameters
- Use Regularization Techniques to Handle Multicollinearity
- Handle Missing Values in Categorical Variables
- Use Appropriate Evaluation Metrics
- Consider Using Ensemble Methods
Use One-Hot Encoding to Convert Categorical Variables
One-hot encoding is a crucial preprocessing step when dealing with categorical variables in logistic regression. This technique converts categorical data into a format that can be provided to machine learning algorithms to do a better job in prediction. Each category value is converted into a new categorical column and assigned a binary value of 1 or 0.
For example, consider a dataset with a categorical feature "Color" that includes values "Red", "Blue", and "Green". Using one-hot encoding, this feature would be transformed into three new binary features: "Color_Red", "Color_Blue", and "Color_Green".
import pandas as pd
from sklearn.preprocessing import OneHotEncoder
# Sample data
data = {'Color': ['Red', 'Blue', 'Green', 'Red']}
df = pd.DataFrame(data)
# One-hot encoding
encoder = OneHotEncoder()
encoded_data = encoder.fit_transform(df[['Color']]).toarray()
encoded_df = pd.DataFrame(encoded_data, columns=encoder.get_feature_names_out(['Color']))
print(encoded_df)
Using one-hot encoding ensures that the logistic regression model can process the categorical data correctly, as these binary variables can now be interpreted numerically, facilitating the calculation of weights and biases during model training.
Apply Feature Scaling to Ensure Variables Are on the Same Scale
Feature scaling is essential to ensure that all variables contribute equally to the analysis and to improve the efficiency and performance of logistic regression models. Scaling transforms the data to fit within a particular range or distribution, which can be especially important for algorithms that calculate distances or gradients.
Unveiling Decision Tree-based Ensemble MethodsTypes of Feature Scaling
The two most common types of feature scaling are min-max scaling and standardization. Min-max scaling (normalization) rescales the data to a fixed range, usually [0, 1], while standardization transforms the data to have a mean of 0 and a standard deviation of 1.
from sklearn.preprocessing import StandardScaler, MinMaxScaler
# Sample data
data = {'Age': [25, 35, 45, 50], 'Income': [50000, 60000, 70000, 80000]}
df = pd.DataFrame(data)
# Standardization
scaler = StandardScaler()
standardized_data = scaler.fit_transform(df[['Age', 'Income']])
standardized_df = pd.DataFrame(standardized_data, columns=['Age', 'Income'])
print(standardized_df)
# Min-max scaling
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(df[['Age', 'Income']])
normalized_df = pd.DataFrame(normalized_data, columns=['Age', 'Income'])
print(normalized_df)
By applying feature scaling, the logistic regression model can converge more quickly and perform better, as all features are considered equally, and the effects of scale differences are minimized.
Regularize the Logistic Regression Model
Regularization is a technique used to prevent overfitting by adding a penalty term to the logistic regression cost function. This penalty term discourages the model from fitting the training data too closely, promoting better generalization to unseen data.
Types of Regularization
The two main types of regularization used in logistic regression are L1 regularization (Lasso Regression) and L2 regularization (Ridge Regression). L1 regularization adds the absolute value of the coefficients as a penalty term, which can lead to sparse models where some feature coefficients are exactly zero. L2 regularization adds the squared value of the coefficients, which tends to keep all features but with reduced impact.
Fine-Tuning for Model Optimization in Machine LearningBenefits of Regularization
Regularization helps to improve model generalization by penalizing large coefficients, thus reducing the model's complexity. It is particularly useful in handling multicollinearity, where predictor variables are highly correlated. By adding regularization, the model's sensitivity to these correlated features is reduced, leading to more stable and reliable predictions.
from sklearn.linear_model import LogisticRegression
# Sample data
X = [[1, 2], [2, 3], [3, 4], [4, 5]]
y = [0, 0, 1, 1]
# L1 Regularization
model_l1 = LogisticRegression(penalty='l1', solver='liblinear')
model_l1.fit(X, y)
print("L1 Coefficients:", model_l1.coef_)
# L2 Regularization
model_l2 = LogisticRegression(penalty='l2')
model_l2.fit(X, y)
print("L2 Coefficients:", model_l2.coef_)
Using regularization techniques ensures that the logistic regression model maintains a balance between bias and variance, leading to more accurate and generalizable results.
Perform Cross-Validation to Choose Hyperparameters
Cross-validation is a technique used to evaluate the performance of a machine learning model and to select the best hyperparameters. By splitting the data into multiple folds and training the model on different subsets, cross-validation provides a more reliable estimate of the model's performance.
Implementing Cross-Validation
Implementing cross-validation involves dividing the dataset into k folds, training the model on k-1 folds, and validating it on the remaining fold. This process is repeated k times, with each fold used exactly once as the validation set.
Optimizing Machine Learning: Determining the Ideal Number of Epochsfrom sklearn.model_selection import cross_val_score
# Sample data
X = [[1, 2], [2, 3], [3, 4], [4, 5]]
y = [0, 0, 1, 1]
# Logistic Regression with Cross-Validation
model = LogisticRegression()
scores = cross_val_score(model, X, y, cv=4)
print("Cross-Validation Scores:", scores)
Benefits of Cross-Validation
Cross-validation helps in choosing the best hyperparameters by providing a more accurate measure of model performance. It reduces the risk of overfitting and ensures that the model is evaluated on multiple subsets of data, leading to more robust and reliable results.
Using cross-validation in logistic regression helps in fine-tuning the model and selecting the optimal hyperparameters, ensuring that the final model performs well on unseen data.
Use Regularization Techniques to Handle Multicollinearity
Multicollinearity occurs when predictor variables are highly correlated, leading to unreliable estimates of regression coefficients. Regularization techniques such as L1 and L2 regularization can help handle multicollinearity by adding penalty terms to the cost function.
L1 Regularization (Lasso Regression)
L1 regularization adds the absolute value of the coefficients as a penalty term, which can lead to sparse models where some feature coefficients are exactly zero. This property makes L1 regularization particularly useful for feature selection in the presence of multicollinearity.
Comparing Machine Learning TechniquesL2 Regularization (Ridge Regression)
L2 regularization adds the squared value of the coefficients as a penalty term, which tends to keep all features but with reduced impact. L2 regularization is effective in reducing the influence of correlated features, leading to more stable and reliable predictions.
from sklearn.linear_model import LogisticRegression
# Sample data
X = [[1, 2], [2, 3], [3, 4], [4, 5]]
y = [0, 0, 1, 1]
# L1 Regularization
model_l1 = LogisticRegression(penalty='l1', solver='liblinear')
model_l1.fit(X, y)
print("L1 Coefficients:", model_l1.coef_)
# L2 Regularization
model_l2 = LogisticRegression(penalty='l2')
model_l2.fit(X, y)
print("L2 Coefficients:", model_l2.coef_)
Using regularization techniques helps in handling multicollinearity by penalizing large coefficients, leading to more stable and reliable logistic regression models.
Handle Missing Values in Categorical Variables
Handling missing values in categorical variables is crucial for the accuracy and reliability of logistic regression models. Missing values can be addressed by either imputing them or creating a separate category for missing values.
Imputing Missing Values
Imputing missing values involves replacing missing data with estimated values. Common imputation methods include using the mode, median, or mean of the available data. For categorical variables, the mode is often used as it represents the most frequent category.
Linear Regression in Machine Learning with R: Step-by-Step GuideCreating a Separate Category for Missing Values
Another approach to handling missing values is to create a separate category for missing values. This method involves treating missing values as a distinct category, allowing the model to learn patterns associated with the absence of data.
import pandas as pd
from sklearn.impute import SimpleImputer
# Sample data
data = {'Color': ['Red', None, 'Green', 'Red']}
df = pd.DataFrame(data)
# Imputing missing values
imputer = SimpleImputer(strategy='most_frequent')
df['Color'] = imputer.fit_transform(df[['Color']])
print(df)
# Creating a separate category for missing values
df['Color'] = df['Color'].fillna('Missing')
print(df)
Effectively handling missing values ensures that the logistic regression model can process the data accurately, leading to more reliable and meaningful predictions.
Use Appropriate Evaluation Metrics
Evaluation metrics are essential for assessing the performance of logistic regression models on categorical variables. Common metrics include accuracy, precision, recall, and F1-score, each providing different insights into the model's performance.
Accuracy
Accuracy measures the proportion of correctly predicted instances out of the total instances. It is a useful metric when the classes are balanced, but it may not provide a complete picture when dealing with imbalanced datasets.
Machine Learning Algorithms for Simultaneously Handling Two DatasetsPrecision
Precision measures the proportion of true positive predictions out of the total predicted positives. It is particularly useful in scenarios where the cost of false positives is high, such as fraud detection or medical diagnosis.
Recall
Recall measures the proportion of true positive predictions out of the total actual positives. It is useful in scenarios where the cost of false negatives is high, such as in disease screening or safety-critical systems.
F1-Score
F1-score is the harmonic mean of precision and recall, providing a balanced measure of both metrics. It is particularly useful when dealing with imbalanced datasets, as it considers both false positives and false negatives.
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
# Sample data
y_true = [0, 1, 1, 0, 1, 1, 0]
y_pred = [0, 1, 0, 0, 1, 1, 1]
# Calculate evaluation metrics
accuracy = accuracy_score(y_true, y_pred)
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
print(f"Accuracy: {accuracy}")
print(f"Precision: {precision}")
print(f"Recall: {recall}")
print(f"F1-Score: {f1}")
Using appropriate evaluation metrics ensures that the logistic regression model's performance is accurately assessed, providing meaningful insights into its effectiveness.
Consider Using Ensemble Methods
Ensemble methods combine multiple models to improve the predictive power of logistic regression on categorical variables. Techniques such as Random Forest and Gradient Boosting are commonly used to enhance model performance.
Random Forest
Random Forest is an ensemble method that combines multiple decision trees to improve predictive accuracy and control overfitting. Each tree is trained on a random subset of the data, and the final prediction is made by averaging the predictions of all trees.
Gradient Boosting
Gradient Boosting is an ensemble method that sequentially builds models to correct the errors of previous models. Each new model focuses on the residuals of the previous models, improving the overall performance of the ensemble.
from sklearn.ensemble import RandomForestClassifier, GradientBoostingClassifier
# Sample data
X = [[1, 2], [2, 3], [3, 4], [4, 5]]
y = [0, 0, 1, 1]
# Random Forest
rf_model = RandomForestClassifier()
rf_model.fit(X, y)
rf_pred = rf_model.predict(X)
# Gradient Boosting
gb_model = GradientBoostingClassifier()
gb_model.fit(X, y)
gb_pred = gb_model.predict(X)
print(f"Random Forest Predictions: {rf_pred}")
print(f"Gradient Boosting Predictions: {gb_pred}")
Using ensemble methods can significantly enhance the predictive power of logistic regression models, providing more accurate and robust predictions.
By following these structured steps and techniques, you can effectively implement logistic regression for categorical variables in machine learning. This guide provides a comprehensive overview of the processes involved, from preprocessing and feature scaling to regularization and model evaluation.
If you want to read more articles similar to Logistic Regression for Categorical Variables in Machine Learning, you can visit the Algorithms category.
You Must Read