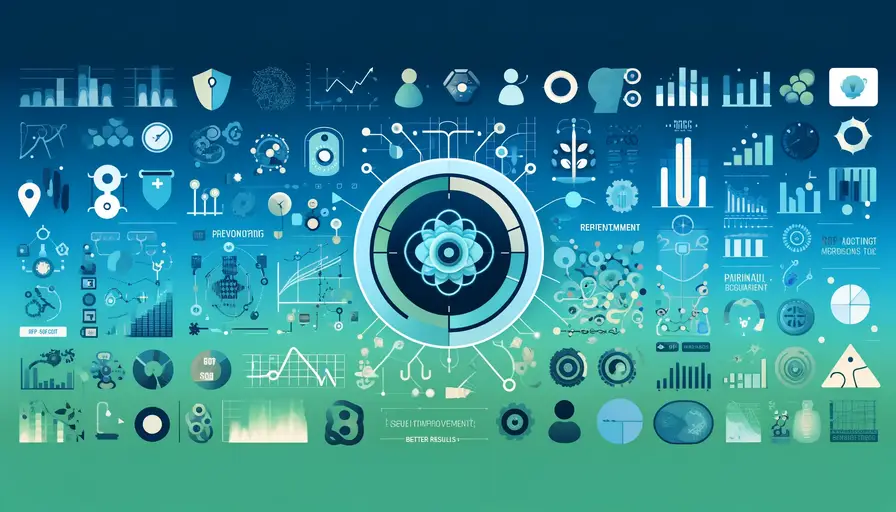
Low Bias in Machine Learning Models and Overfitting

Understanding Bias and Overfitting
Bias and overfitting are two critical concepts in machine learning that influence the performance of models. Understanding their relationship is essential for building robust and accurate models.
What is Bias?
Bias refers to the error introduced by approximating a real-world problem, which may be extremely complex, by a simplified model. High bias can cause a model to miss relevant relations between features and target outputs, leading to underfitting.
What is Overfitting?
Overfitting occurs when a machine learning model learns not only the underlying patterns in the training data but also the noise. This results in a model that performs well on training data but poorly on unseen data.
Example: High Bias and Overfitting
Here’s an example of a high bias model and an overfitted model using Python and Scikit-Learn:

import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
from sklearn.pipeline import make_pipeline
# Generate data
np.random.seed(0)
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel() + np.random.normal(0, 0.2, X.shape[0])
# Fit linear model (high bias)
linear_model = LinearRegression()
linear_model.fit(X, y)
y_pred_linear = linear_model.predict(X)
# Fit polynomial model (overfitting)
poly_model = make_pipeline(PolynomialFeatures(10), LinearRegression())
poly_model.fit(X, y)
y_pred_poly = poly_model.predict(X)
# Plot results
plt.scatter(X, y, color='black', label='Data')
plt.plot(X, y_pred_linear, color='blue', label='Linear Model (High Bias)')
plt.plot(X, y_pred_poly, color='red', label='Polynomial Model (Overfitting)')
plt.legend()
plt.show()
The Bias-Variance Tradeoff
The bias-variance tradeoff is a fundamental concept in machine learning that describes the balance between the error introduced by bias and the error introduced by variance. Understanding this tradeoff is crucial for model development.
Bias-Variance Tradeoff Explained
Bias is the error due to overly simplistic assumptions in the learning algorithm, while variance is the error due to sensitivity to small fluctuations in the training set. Ideally, a model should have low bias and low variance, but in practice, reducing one often increases the other.
Managing the Tradeoff
Balancing bias and variance involves choosing a model that performs well on both the training data and unseen data. Techniques like cross-validation, regularization, and ensemble methods can help manage this tradeoff.
Example: Cross-Validation to Balance Bias and Variance
Here’s an example of using cross-validation to balance bias and variance in Python:

from sklearn.model_selection import cross_val_score
from sklearn.tree import DecisionTreeRegressor
# Generate data
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel() + np.random.normal(0, 0.2, X.shape[0])
# Fit decision tree model
tree_model = DecisionTreeRegressor()
# Perform cross-validation
scores = cross_val_score(tree_model, X, y, cv=5, scoring='neg_mean_squared_error')
print(f"Cross-Validation Scores: {scores}")
print(f"Mean Cross-Validation Score: {np.mean(scores)}")
Causes of Overfitting
Overfitting can be caused by various factors, including an overly complex model, insufficient training data, and noise in the data. Identifying these causes is the first step in addressing overfitting.
Complex Models
Complex models with many parameters can capture noise in the training data, leading to overfitting. Simplifying the model or using regularization techniques can help mitigate this issue.
Insufficient Training Data
When the training data is limited, the model may not learn the underlying patterns adequately, resulting in overfitting. Increasing the size of the training dataset can improve the model's performance.
Example: Regularization to Prevent Overfitting
Here’s an example of using L2 regularization to prevent overfitting in Python:

from sklearn.linear_model import Ridge
# Generate data
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel() + np.random.normal(0, 0.2, X.shape[0])
# Fit ridge regression model
ridge_model = Ridge(alpha=1.0)
ridge_model.fit(X, y)
y_pred_ridge = ridge_model.predict(X)
# Plot results
plt.scatter(X, y, color='black', label='Data')
plt.plot(X, y_pred_ridge, color='green', label='Ridge Regression')
plt.legend()
plt.show()
Techniques to Mitigate Overfitting
Several techniques can be employed to mitigate overfitting, ensuring that the model generalizes well to unseen data. These techniques include regularization, data augmentation, and cross-validation.
Regularization
Regularization techniques like L1 (Lasso) and L2 (Ridge) add a penalty to the loss function, discouraging overly complex models. This helps in reducing overfitting and improving model generalization.
Data Augmentation
Data augmentation involves artificially increasing the size of the training dataset by adding modified copies of existing data. This technique is particularly useful in image and text data.
Example: Data Augmentation with Images
Here’s an example of applying data augmentation to images using TensorFlow:

import tensorflow as tf
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Load dataset
(X_train, y_train), (X_test, y_test) = tf.keras.datasets.cifar10.load_data()
# Create an image data generator
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
horizontal_flip=True
)
# Fit the generator on the training data
datagen.fit(X_train)
# Use the augmented data to train a model
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model using augmented data
model.fit(datagen.flow(X_train, y_train, batch_size=32), epochs=10, validation_data=(X_test, y_test))
Cross-Validation
Cross-validation involves splitting the dataset into multiple subsets and training the model on each subset while validating on the remaining data. This technique helps in assessing the model's performance and reducing overfitting.
The Role of Bias in Model Performance
Bias plays a crucial role in determining the performance of machine learning models. While low bias is generally desirable, it must be balanced with variance to achieve optimal performance.
Low Bias and Overfitting
A model with low bias fits the training data well but may capture noise, leading to overfitting. It’s essential to strike a balance between bias and variance to prevent overfitting and improve generalization.
High Bias and Underfitting
High bias indicates that the model is too simple to capture the underlying patterns in the data, leading to underfitting. This results in poor performance on both training and unseen data.

Example: High Bias and Low Bias Models
Here’s an example of comparing high bias and low bias models in Python:
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
from sklearn.pipeline import make_pipeline
# Generate data
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel() + np.random.normal(0, 0.2, X.shape[0])
# Fit high bias model (linear regression)
high_bias_model = LinearRegression()
high_bias_model.fit(X, y)
y_pred_high_bias = high_bias_model.predict(X)
# Fit low bias model (polynomial regression)
low_bias_model = make_pipeline(PolynomialFeatures(10), LinearRegression())
low_bias_model.fit(X, y)
y_pred_low_bias = low_bias_model.predict(X)
# Plot results
plt.scatter(X, y, color='black', label='Data')
plt.plot(X, y_pred_high_bias, color='blue', label='High Bias Model')
plt.plot(X, y_pred_low_bias, color='red', label='Low Bias Model')
plt.legend()
plt.show()
Strategies for Balancing Bias and Variance
Achieving a balance between bias and variance is crucial for developing effective machine learning models. Several strategies can help in finding the right balance.
Model Selection
Choosing the appropriate model complexity based on the dataset is essential. Simple models have high bias and low variance, while complex models have low bias and high variance. Selecting the right model involves evaluating the tradeoff between bias and variance.
Regularization
Regularization techniques add a penalty to the loss function, which discourages complex models and helps in balancing bias and variance. L1 and L2 regularization are commonly used methods.

Example: Applying L1 Regularization
Here’s an example of using L1 regularization (Lasso) in Python:
from sklearn.linear_model import Lasso
# Generate data
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel() + np.random.normal(0, 0.2, X.shape[0])
# Fit Lasso model
lasso_model = Lasso(alpha=0.1)
lasso_model.fit(X, y)
y_pred_lasso = lasso_model.predict(X)
# Plot results
plt.scatter(X, y, color='black', label='Data')
plt.plot(X, y_pred_lasso, color='green', label='Lasso Regression')
plt.legend()
plt.show()
Ensemble Methods
Ensemble methods combine multiple models to improve performance and reduce overfitting. Techniques like bagging, boosting, and stacking can help in balancing bias and variance.
The Impact of Data Quality
The quality of the training data significantly impacts the performance of machine learning models. High-quality data helps in reducing bias and variance, leading to better generalization.
Importance of Data Quality
Data quality is crucial for building accurate and reliable models. Poor quality data with errors, missing values, or noise can lead to high bias and variance, affecting the model's performance.
Data Cleaning
Data cleaning involves identifying and correcting errors, handling missing values, and removing noise. This process ensures that the data used for training is of high quality.
Example: Data Cleaning
Here’s an example of data cleaning using Pandas in Python:
import pandas as pd
# Load dataset
data = pd.read_csv('data.csv')
# Remove missing values
clean_data = data.dropna()
# Remove duplicates
clean_data = clean_data.drop_duplicates()
# Correct data types
clean_data['column_name'] = clean_data['column_name'].astype('int')
print(clean_data.head())
Feature Engineering
Feature engineering involves creating new features from existing data to improve model performance. High-quality features help in reducing bias and variance, leading to better generalization.
Evaluating Model Performance
Evaluating model performance is crucial for understanding the impact of bias and variance. Various metrics and techniques can help in assessing the model's performance.
Performance Metrics
Common performance metrics for regression models include Mean Absolute Error (MAE), Mean Squared Error (MSE), and R-squared. For classification models, metrics like accuracy, precision, recall, and F1 score are used.
Cross-Validation
Cross-validation involves splitting the dataset into multiple subsets and training the model on each subset while validating on the remaining data. This technique helps in assessing the model's performance and reducing overfitting.
Example: Cross-Validation for Model Evaluation
Here’s an example of performing cross-validation using Scikit-Learn:
from sklearn.model_selection import cross_val_score
from sklearn.tree import DecisionTreeClassifier
# Generate data
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel() + np.random.normal(0, 0.2, X.shape[0])
# Fit decision tree model
tree_model = DecisionTreeClassifier()
# Perform cross-validation
scores = cross_val_score(tree_model, X, y, cv=5, scoring='accuracy')
print(f"Cross-Validation Scores: {scores}")
print(f"Mean Cross-Validation Score: {np.mean(scores)}")
Understanding the relationship between low bias and overfitting is essential for developing robust and accurate machine learning models. By balancing bias and variance, employing techniques to mitigate overfitting, and ensuring high data quality, you can build models that generalize well to unseen data. Regular evaluation of model performance using appropriate metrics and cross-validation helps in maintaining the model's effectiveness. Leveraging these strategies will enhance your ability to tackle complex machine learning tasks and achieve better results.
If you want to read more articles similar to Low Bias in Machine Learning Models and Overfitting, you can visit the Bias and Overfitting category.
You Must Read