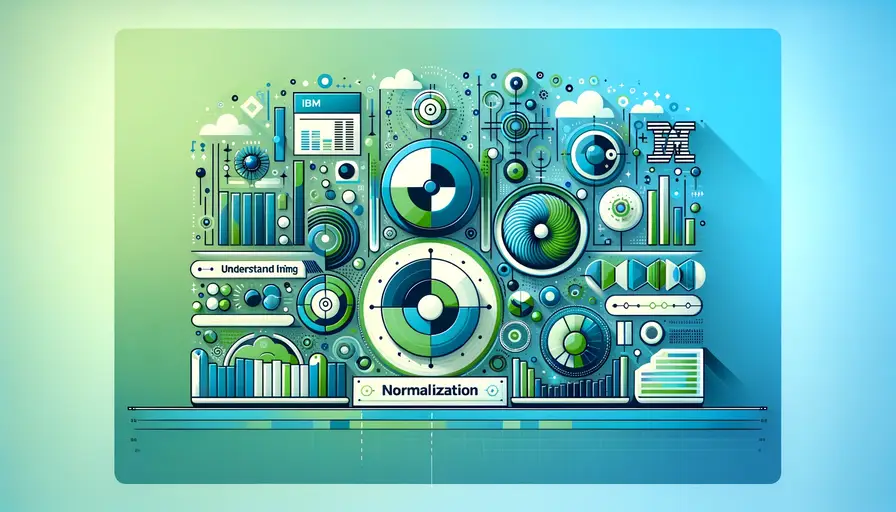
Machine Learning and Prediction
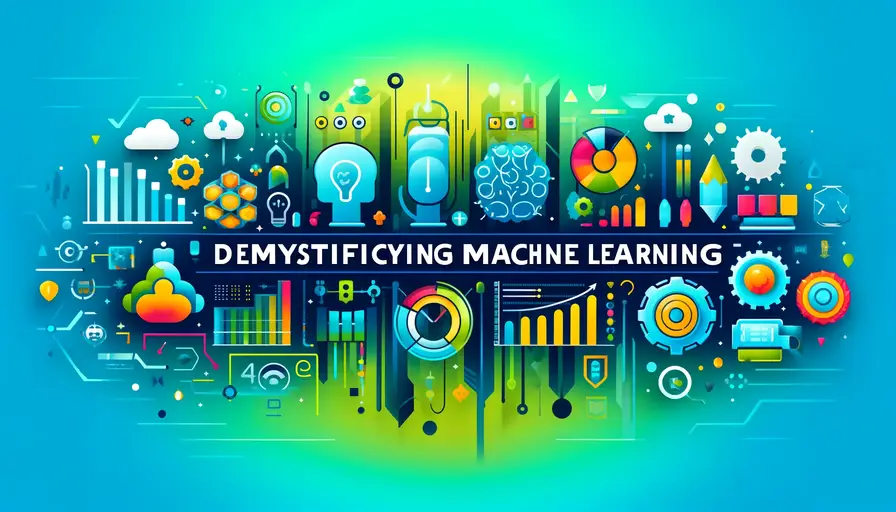
Machine Learning
Machine learning (ML) has become a pivotal technology in modern data analysis and artificial intelligence. This guide aims to demystify machine learning by explaining the concepts of learning and prediction, which are fundamental to understanding how ML works.
What is Machine Learning?
Machine learning is a subset of artificial intelligence that enables systems to learn from data and make predictions or decisions without being explicitly programmed. It involves training algorithms on data to identify patterns and make informed predictions.
Importance of Machine Learning
Machine learning is crucial for automating decision-making processes, improving accuracy in data analysis, and driving innovation across various industries. From personalized recommendations to predictive maintenance, ML applications are vast and impactful.
Example: Simple ML Workflow in Python
Here’s an example of a basic machine learning workflow using Python and Scikit-Learn:

import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop(columns=['target'])
y = data['target']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Types of Machine Learning
There are several types of machine learning, each suited for different tasks and data types. The main categories include supervised learning, unsupervised learning, and reinforcement learning.
Supervised Learning
Supervised learning involves training a model on labeled data, where the correct output is known. The model learns to map inputs to outputs by minimizing the error between predicted and actual values.
Unsupervised Learning
Unsupervised learning deals with unlabeled data, where the model tries to identify patterns and relationships within the data without predefined outputs. Clustering and dimensionality reduction are common techniques in unsupervised learning.
Example: K-Means Clustering in Python
Here’s an example of performing K-Means clustering using Scikit-Learn:

import pandas as pd
from sklearn.cluster import KMeans
# Load dataset
data = pd.read_csv('data.csv')
features = data.drop(columns=['id'])
# Perform K-Means clustering
kmeans = KMeans(n_clusters=3, random_state=42)
clusters = kmeans.fit_predict(features)
# Add cluster labels to the dataset
data['cluster'] = clusters
print(data.head())
Reinforcement Learning
Reinforcement learning involves training a model to make a sequence of decisions by rewarding desirable actions and punishing undesirable ones. It is widely used in robotics, gaming, and autonomous systems.
How Reinforcement Learning Works
In reinforcement learning, an agent interacts with an environment by taking actions and receiving feedback in the form of rewards or penalties. The goal is to learn a policy that maximizes cumulative rewards over time.
Applications of Reinforcement Learning
Reinforcement learning is used in various applications, including autonomous driving, game playing (such as AlphaGo), and optimizing industrial processes. Its ability to handle complex decision-making scenarios makes it highly valuable.
Example: Simple Reinforcement Learning with Q-Learning
Here’s an example of implementing a basic Q-learning algorithm using Python:

import numpy as np
# Define the environment
states = ['A', 'B', 'C', 'D']
actions = ['left', 'right']
rewards = {'A': {'left': 0, 'right': 1}, 'B': {'left': 1, 'right': 0}, 'C': {'left': 1, 'right': 0}, 'D': {'left': 0, 'right': 1}}
q_table = {state: {action: 0 for action in actions} for state in states}
# Define parameters
alpha = 0.1
gamma = 0.9
epsilon = 0.1
episodes = 100
# Q-learning algorithm
for _ in range(episodes):
state = np.random.choice(states)
while state != 'D':
if np.random.uniform(0, 1) < epsilon:
action = np.random.choice(actions)
else:
action = max(q_table[state], key=q_table[state].get)
reward = rewards[state][action]
next_state = 'D' if state == 'C' and action == 'right' else state
q_table[state][action] = q_table[state][action] + alpha * (reward + gamma * max(q_table[next_state].values()) - q_table[state][action])
state = next_state
print(q_table)
Evaluating Machine Learning Models
Evaluating the performance of machine learning models is essential to ensure their accuracy and reliability. Various metrics and techniques are used to assess how well a model performs.
Performance Metrics
Performance metrics vary depending on the type of problem (classification, regression, clustering). Common metrics for classification include accuracy, precision, recall, and F1 score. For regression, metrics like mean squared error (MSE) and R-squared are used.
Cross-Validation
Cross-validation is a technique to assess the generalizability of a model. It involves splitting the data into multiple subsets and training the model on different combinations of these subsets to ensure it performs well on unseen data.
Example: Cross-Validation in Python
Here’s an example of performing cross-validation using Scikit-Learn:

import pandas as pd
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop(columns=['target'])
y = data['target']
# Perform cross-validation
model = RandomForestClassifier(random_state=42)
scores = cross_val_score(model, X, y, cv=5, scoring='accuracy')
print(f"Cross-Validation Scores: {scores}")
print(f"Mean Cross-Validation Score: {np.mean(scores)}")
Feature Engineering
Feature engineering involves creating new features from existing data to improve model performance. It plays a crucial role in enhancing the predictive power of machine learning models.
Importance of Feature Engineering
Good features can significantly impact the performance of a model. Feature engineering involves techniques such as scaling, normalization, and creating interaction features to provide better inputs for the model.
Techniques for Feature Engineering
Common techniques include encoding categorical variables, scaling numerical features, and creating polynomial features. Each technique aims to make the data more suitable for the chosen algorithm.
Example: Feature Engineering with Scikit-Learn
Here’s an example of feature engineering using Scikit-Learn:

import pandas as pd
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.compose import ColumnTransformer
from sklearn.pipeline import Pipeline
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop(columns=['target'])
y = data['target']
# Define preprocessing steps
numeric_features = ['age', 'income']
numeric_transformer = StandardScaler()
categorical_features = ['gender', 'occupation']
categorical_transformer = OneHotEncoder()
preprocessor = ColumnTransformer(
transformers=[
('num', numeric_transformer, numeric_features),
('cat', categorical_transformer, categorical_features)
])
# Create preprocessing pipeline
pipeline = Pipeline(steps=[('preprocessor', preprocessor)])
# Apply preprocessing
X_preprocessed = pipeline.fit_transform(X)
print(X_preprocessed)
Model Selection
Choosing the right model is crucial for achieving optimal performance. Different models have different strengths and are suitable for various types of data and problems.
Criteria for Model Selection
Criteria for selecting a model include the nature of the problem (classification, regression), the size and complexity of the dataset, and the interpretability requirements.
Commonly Used Models
Commonly used models include linear regression, decision trees, support vector machines (SVM), and neural networks. Each model has its advantages and is suitable for specific types of problems.
Example: Model Selection with Grid Search
Here’s an example of selecting a model using grid search in Scikit-Learn:

import pandas as pd
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop(columns=['target'])
y = data['target']
# Define parameter grid
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 10, 20],
'min_samples_split': [2, 5, 10]
}
# Perform grid search
model = RandomForestClassifier(random_state=42)
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5, scoring='accuracy')
grid_search.fit(X, y)
# Print best parameters
print(f"Best Parameters: {grid_search.best_params_}")
Hyperparameter Tuning
Hyperparameters are parameters that are set before the learning process begins and control the model’s behavior. Tuning these hyperparameters is essential to optimize the model's performance.
Importance of Hyperparameter Tuning
Properly tuned hyperparameters can significantly improve the performance of a model. Tuning involves searching for the best combination of hyperparameters to maximize the model’s accuracy.
Techniques for Hyperparameter Tuning
Common techniques for hyperparameter tuning include grid search, random search, and Bayesian optimization. Each technique aims to find the best hyperparameters efficiently.
Example: Hyperparameter Tuning with Random Search
Here’s an example of hyperparameter tuning using random search in Scikit-Learn:
import pandas as pd
from sklearn.model_selection import RandomizedSearchCV
from sklearn.ensemble import RandomForestClassifier
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop(columns=['target'])
y = data['target']
# Define parameter grid
param_distributions = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 10, 20],
'min_samples_split': [2, 5, 10]
}
# Perform random search
model = RandomForestClassifier(random_state=42)
random_search = RandomizedSearchCV(estimator=model, param_distributions=param_distributions, n_iter=10, cv=5, scoring='accuracy', random_state=42)
random_search.fit(X, y)
# Print best parameters
print(f"Best Parameters: {random_search.best_params_}")
Model Evaluation
Evaluating a model’s performance is crucial to understand its effectiveness. This involves using various metrics and techniques to assess how well the model performs on unseen data.
Importance of Model Evaluation
Model evaluation helps in identifying potential issues, comparing different models, and ensuring that the model generalizes well to new data. It is a critical step in the machine learning workflow.
Techniques for Model Evaluation
Common techniques for model evaluation include cross-validation, holdout validation, and using metrics such as accuracy, precision, recall, and F1 score for classification problems.
Example: Evaluating a Classification Model
Here’s an example of evaluating a classification model using Scikit-Learn:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# Load dataset
data = pd.read_csv('data.csv')
X = data.drop(columns=['target'])
y = data['target']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = RandomForestClassifier(random_state=42)
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate model
report = classification_report(y_test, predictions)
print(report)
Mastering the art of machine learning involves understanding the fundamental concepts of learning and prediction. From data preprocessing and feature engineering to model selection and evaluation, each step plays a crucial role in building robust and reliable models. By leveraging various techniques and tools, and continuously refining your approach, you can harness the power of machine learning to drive innovation and make informed decisions. With a solid foundation in these concepts, you are well-equipped to tackle complex problems and contribute to the advancement of AI and machine learning.
If you want to read more articles similar to Machine Learning and Prediction, you can visit the Artificial Intelligence category.
You Must Read