
Machine Learning for RSS Feed Analysis in R
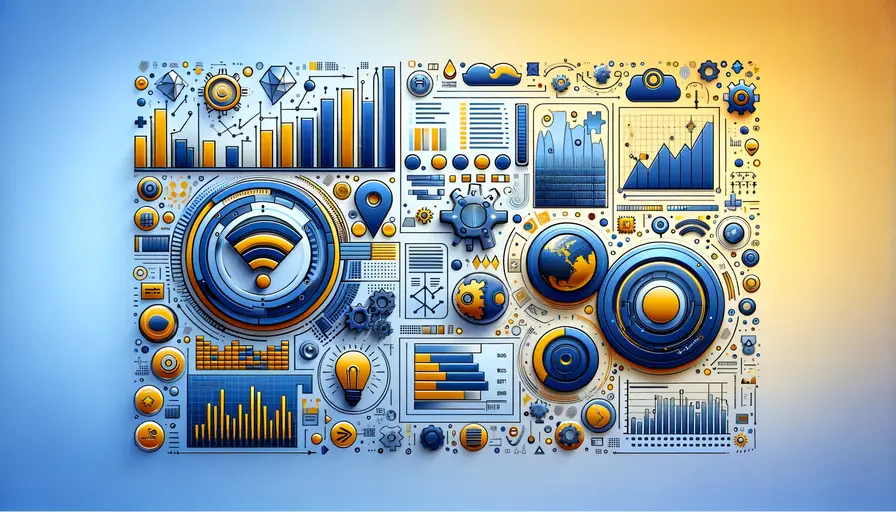
Machine learning offers powerful tools for analyzing and extracting insights from RSS feeds. By leveraging machine learning techniques, you can identify patterns, predict trends, automate analysis, and build recommendation systems tailored to users' interests. Here's a comprehensive guide to using machine learning for RSS feed analysis in R.
Content
- Analyze RSS Feed Data in R
- Code Example for RSS Feed in R
- Identify Patterns and Trends in RSS Feed
- Predictive Models to Forecast Future RSS Feed
- Automate the Process of RSS Feed Analysis
- Natural Language Processing Techniques
- Use Clustering Algorithms to Group Similar RSS Feed
- Recommendation System for Personalized RSS Feed Content
- Improve the Accuracy of RSS Feed Classification
- Sentiment Analysis to Determine the Sentiment of RSS
Analyze RSS Feed Data in R
Analyzing RSS feed data in R involves collecting, preprocessing, and applying machine learning algorithms to gain meaningful insights from the data. R provides various packages and functions that simplify these tasks, making it an excellent choice for RSS feed analysis.
Why Use Machine Learning for RSS Feed Analysis?
Why use machine learning for RSS feed analysis? Machine learning enables you to handle large volumes of data efficiently, uncover hidden patterns, and make predictions based on historical data. It enhances the analysis by automating repetitive tasks, improving accuracy, and providing scalable solutions for real-time data processing.
Getting Started With Machine Learning for RSS Feed Analysis in R
Getting started with machine learning for RSS feed analysis in R requires a basic understanding of R programming and machine learning concepts. You will need to install and load relevant libraries such as tidyverse
for data manipulation, tm
for text mining, and caret
for machine learning. Collecting RSS feed data can be done using packages like xml2
and rvest
.
Code Example for RSS Feed in R
This example will focus on extracting RSS feed data, processing it, and performing a simple text classification using the tm
and caret
packages.
Install and Load Required Packages
First, you'll need to install and load the necessary packages. If you don't have these packages installed, you can install them using install.packages()
.
# Install packages if not already installed
install.packages("tidyverse")
install.packages("xml2")
install.packages("tm")
install.packages("caret")
install.packages("text2vec")
# Load libraries
library(tidyverse)
library(xml2)
library(tm)
library(caret)
library(text2vec)
Extract and Parse RSS Feed Data
Next, we'll extract and parse the RSS feed data. For this example, let's use a sample RSS feed URL.
# Sample RSS feed URL
rss_url <- "https://rss.cnn.com/rss/cnn_topstories.rss"
# Parse RSS feed
rss_content <- xml2::read_xml(rss_url)
# Extract item nodes
items <- xml2::xml_find_all(rss_content, "//item")
# Extract titles and descriptions
titles <- xml2::xml_text(xml2::xml_find_all(items, "title"))
descriptions <- xml2::xml_text(xml2::xml_find_all(items, "description"))
# Combine titles and descriptions into a data frame
rss_data <- data.frame(title = titles, description = descriptions, stringsAsFactors = FALSE)
rss_data$text <- paste(rss_data$title, rss_data$description)
Preprocess Text Data
We need to preprocess the text data by cleaning and tokenizing it.
Comparing Affordable Machine Learning Models# Create a text corpus
corpus <- VCorpus(VectorSource(rss_data$text))
# Text preprocessing
corpus <- tm_map(corpus, content_transformer(tolower))
corpus <- tm_map(corpus, removePunctuation)
corpus <- tm_map(corpus, removeNumbers)
corpus <- tm_map(corpus, removeWords, stopwords("en"))
corpus <- tm_map(corpus, stripWhitespace)
# Create a Document-Term Matrix
dtm <- DocumentTermMatrix(corpus)
Create a Machine Learning Model
We'll create a simple text classification model. For simplicity, let's assume we are classifying articles into two categories: "news" and "other."
# Create a binary response variable
# For the sake of this example, let's randomly assign labels
set.seed(123)
rss_data$category <- sample(c("news", "other"), nrow(rss_data), replace = TRUE)
# Convert DTM to a matrix and create a data frame
dtm_matrix <- as.matrix(dtm)
data <- data.frame(category = rss_data$category, dtm_matrix)
# Split data into training and testing sets
set.seed(123)
trainIndex <- createDataPartition(data$category, p = 0.8, list = FALSE)
train_data <- data[trainIndex, ]
test_data <- data[-trainIndex, ]
# Train a simple Naive Bayes model
model <- train(category ~ ., data = train_data, method = "nb", trControl = trainControl(method = "cv", number = 10))
# Predict on test data
predictions <- predict(model, newdata = test_data)
# Evaluate the model
confusionMatrix(predictions, test_data$category)
You can enhance this code by improving the preprocessing steps, trying different models, and tuning hyperparameters for better performance.
Identify Patterns and Trends in RSS Feed
Identifying patterns and trends in RSS feeds is crucial for understanding user behavior, preferences, and emerging topics. Machine learning models can analyze the temporal and textual aspects of RSS feed data to reveal these insights.
Why Use Machine Learning for RSS Feed Analysis?
Why use machine learning for RSS feed analysis? Machine learning models can automatically detect trends and patterns that might be missed through manual analysis. They can process large datasets quickly and provide insights that drive decision-making, such as identifying popular topics or predicting future trends.
Is ChatGPT: A Deep Learning Model for Conversational AI?Types of Machine Learning Algorithms for RSS Feed Analysis
Types of machine learning algorithms for RSS feed analysis include supervised learning algorithms like linear regression, decision trees, and support vector machines, as well as unsupervised learning algorithms like k-means clustering and hierarchical clustering. These algorithms can classify, cluster, and predict data, making them suitable for various analysis tasks.
Steps to Perform Machine Learning for RSS Feed Analysis in R
Steps to perform machine learning for RSS feed analysis in R:
- Collect RSS Feed Data: Use web scraping tools like
rvest
to gather RSS feed data. - Preprocess Data: Clean and format the data, handle missing values, and normalize text data.
- Exploratory Data Analysis (EDA): Visualize data patterns and relationships using plots and summary statistics.
- Feature Engineering: Create relevant features from the data to improve model performance.
- Model Building: Train machine learning models using the
caret
package. - Model Evaluation: Assess model performance using metrics like accuracy, precision, recall, and F1-score.
- Deployment: Implement the model for real-time RSS feed analysis.
Predictive Models to Forecast Future RSS Feed
Predictive models can forecast future trends and topics in RSS feeds, helping businesses and content creators stay ahead of the curve.
Exploratory Data Analysis
Exploratory Data Analysis (EDA) involves summarizing the main characteristics of the data, often using visual methods. EDA helps in understanding the distribution, trends, and anomalies in the data, providing a foundation for further analysis.
Top Python-Based Machine Learning Projects to ExploreData Preprocessing
Data preprocessing is essential for preparing raw data for machine learning models. It includes steps like cleaning the data, handling missing values, normalizing text, and transforming features. Effective preprocessing ensures that the data is in a suitable format for training models.
Building Predictive Models
Building predictive models involves selecting appropriate algorithms, training the models on historical data, and tuning hyperparameters to improve performance. Models such as linear regression, ARIMA, or more advanced methods like recurrent neural networks (RNNs) can be used for time series forecasting.
Automate the Process of RSS Feed Analysis
Automating the process of RSS feed analysis streamlines workflows, saves time, and ensures consistency in data processing and analysis.
Why Automate RSS Feed Analysis?
Why automate RSS feed analysis? Automation reduces manual effort, minimizes errors, and allows for real-time processing of incoming RSS feed data. This leads to faster insights and more timely decision-making.
Popular Machine Learning Models for Analyzing Malware FeaturesGetting Started with RSS Feed Analysis in R
Getting started with RSS feed analysis in R involves setting up automated scripts to collect, preprocess, and analyze data. Tools like cronR
can schedule R scripts to run at regular intervals, ensuring continuous data collection and analysis.
Preprocessing the RSS Feed Data
Preprocessing the RSS feed data includes cleaning text data, tokenization, removing stop words, and stemming or lemmatization. These steps prepare the data for further analysis and improve the accuracy of machine learning models.
Applying Machine Learning Algorithms to Analyze RSS Feeds
Applying machine learning algorithms to analyze RSS feeds involves using models to classify content, detect trends, and make predictions. Models can be trained to categorize articles, identify sentiment, and recommend personalized content.
Natural Language Processing Techniques
Natural Language Processing (NLP) techniques are essential for analyzing the textual content of RSS feeds. NLP helps in extracting meaningful information from text data, making it a vital tool for RSS feed analysis.
Applying Machine Learning for Regression Analysis on YouTube DataWhy Analyze RSS Feed Text Data?
Why analyze RSS feed text data? Analyzing text data helps in understanding the content, sentiment, and relevance of RSS feeds. It enables content categorization, trend detection, and sentiment analysis, providing valuable insights for decision-making.
Getting Started With Machine Learning in R
Getting started with machine learning in R for text analysis requires installing and using packages like tm
, text2vec
, and tidytext
. These packages offer tools for text mining, vectorization, and text analysis, making it easier to preprocess and analyze text data.
Preprocessing RSS Feed Text Data
Preprocessing RSS feed text data involves steps like tokenization, stop words removal, and stemming. These steps convert raw text into a structured format suitable for machine learning models.
Exploratory Data Analysis
Exploratory Data Analysis (EDA) for text data includes visualizing word frequencies, n-grams, and sentiment distributions. EDA helps in understanding the key themes and patterns in the text data.
Applying Machine Learning Algorithms
Applying machine learning algorithms to text data involves using models like Naive Bayes, support vector machines, or neural networks to classify, cluster, or predict text content. These models can help in content categorization, sentiment analysis, and trend detection.
Use Clustering Algorithms to Group Similar RSS Feed
Clustering algorithms group similar RSS feed articles together, helping in content categorization and trend identification.
Why Use Clustering Algorithms for RSS Feed Analysis?
Why use clustering algorithms for RSS feed analysis? Clustering helps in identifying groups of similar articles, enabling efficient content categorization and trend detection. It provides insights into the structure and distribution of the content, facilitating better understanding and management.
Popular Clustering Algorithms for RSS Feed Analysis
Popular clustering algorithms for RSS feed analysis include k-means, hierarchical clustering, and DBSCAN. These algorithms group articles based on their content similarity, making it easier to identify themes and trends.
Recommendation System for Personalized RSS Feed Content
Recommendation systems provide personalized content to users based on their preferences and behavior. Machine learning models analyze user interactions and suggest relevant articles.
Understanding RSS Feeds
Understanding RSS feeds involves analyzing the structure, content, and metadata of feed articles. This understanding is crucial for developing effective recommendation systems.
The Importance of Personalization
The importance of personalization lies in its ability to enhance user engagement and satisfaction. Personalized recommendations ensure that users receive content that aligns with their interests, improving their overall experience.
Machine Learning for RSS Feed Analysis
Machine learning for RSS feed analysis enables the development of sophisticated recommendation systems. Models can analyze user behavior, content similarity, and contextual factors to provide tailored recommendations.
Building a Recommendation System in R
Building a recommendation system in R involves using collaborative filtering, content-based filtering, or hybrid approaches. R packages like recommenderlab
provide tools for developing and evaluating recommendation systems.
Steps to Develop a Recommendation System
Steps to develop a recommendation system include:
- Data Collection: Gather user interaction data and content information.
- Preprocessing: Clean and transform the data.
- Model Training: Train recommendation models using collaborative or content-based filtering.
- Evaluation: Assess the model's performance using metrics like precision, recall, and F1-score.
- Deployment: Implement the model for real-time recommendations.
Improve the Accuracy of RSS Feed Classification
Improving the accuracy of RSS feed classification involves several steps, from data collection to model evaluation and optimization.
Data Collection
Data collection is the first step, involving the gathering of a comprehensive and diverse dataset of RSS feed articles. A robust dataset is crucial for training accurate machine learning models.
Data Preprocessing
Data preprocessing includes cleaning the data, handling missing values, and transforming text data into numerical features. Effective preprocessing enhances the quality of the data, leading to better model performance.
Feature Extraction
Feature extraction involves creating meaningful features from the raw data. Techniques like TF-IDF, word embeddings, and n-grams can be used to represent text data in a format suitable for machine learning models.
Model Selection and Training
Model selection and training involve choosing the appropriate algorithms and tuning their hyperparameters to achieve the best performance. Models like logistic regression, support vector machines,
and neural networks can be used for classification tasks.
Model Evaluation and Optimization
Model evaluation and optimization include assessing the model's performance using metrics like accuracy, precision, recall, and F1-score. Techniques like cross-validation and grid search help in optimizing the model for better results.
Deployment and Integration
Deployment and integration involve implementing the trained model into a production environment. The model should be integrated with the RSS feed system to classify new articles in real-time, providing continuous insights.
Sentiment Analysis to Determine the Sentiment of RSS
Sentiment analysis determines the sentiment of RSS feed articles, providing insights into the tone and emotional content of the text.
Tokenization
Tokenization is the process of breaking down text into individual words or tokens. This step is essential for analyzing the text and extracting meaningful features.
Stop Words Removal
Stop words removal involves eliminating common words like "the," "is," and "and" that do not contribute to the sentiment analysis. Removing stop words reduces noise and improves the accuracy of the analysis.
Sentiment Scores Calculation
Sentiment scores calculation involves assigning a sentiment score to each token or sentence. Techniques like lexicon-based approaches or machine learning models can be used to determine the overall sentiment of the text.
Machine learning for RSS feed analysis in R offers powerful tools and techniques for extracting insights, predicting trends, automating processes, and personalizing content. By leveraging machine learning and natural language processing, you can transform raw RSS feed data into valuable information that drives decision-making and enhances user experiences.
If you want to read more articles similar to Machine Learning for RSS Feed Analysis in R, you can visit the Applications category.
You Must Read