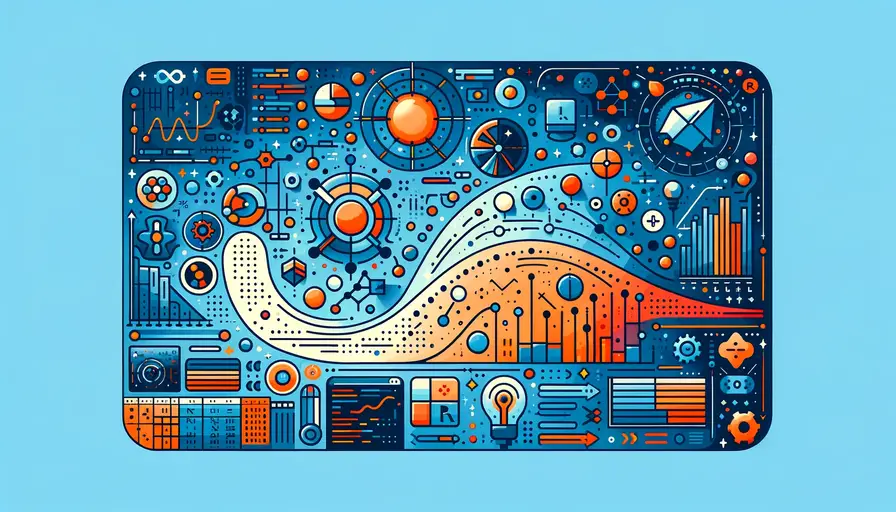
Machine Learning Models for REST APIs: A Comprehensive Guide

Deploying machine learning models as REST APIs is an effective way to integrate machine learning capabilities into various applications. This approach allows different systems to communicate and utilize machine learning models via HTTP requests, making it easy to scale and manage. This guide delves into the process of deploying machine learning models as REST APIs, providing a detailed look at the necessary steps, best practices, and examples using popular tools and frameworks.
Setting Up the Environment
Installing Required Libraries
To deploy machine learning models as REST APIs, you need to set up your environment with the necessary libraries and tools. Key libraries include Flask, FastAPI, pandas, numpy, and scikit-learn. These libraries provide the foundation for building, training, and serving machine learning models through a web API.
First, install the required libraries using pip
:
pip install Flask fastapi pandas numpy scikit-learn uvicorn
These installations prepare your environment for developing and deploying machine learning models as REST APIs.

Creating a Project Structure
Organizing your project structure is crucial for maintaining a clean and scalable codebase. A well-structured project should include directories for models, API code, configuration files, and utilities.
Here is an example of a project structure:
project/
│
├── app/
│ ├── __init__.py
│ ├── main.py
│ ├── models/
│ │ ├── __init__.py
│ │ ├── model.pkl
│ └── utils/
│ ├── __init__.py
│ └── preprocess.py
├── data/
│ └── data.csv
├── requirements.txt
└── README.md
This structure helps organize your code and resources, making it easier to manage and scale your project.
Preparing the Dataset
Before deploying a model, you need to prepare the dataset. This involves loading the data, cleaning it, and transforming it into a format suitable for model training. Pandas and numpy are essential for these tasks.

Here is an example of preparing a dataset using pandas:
import pandas as pd
# Load dataset
data = pd.read_csv('data/data.csv')
# Clean and preprocess data
data = data.dropna() # Remove missing values
data['feature'] = data['feature'].astype(float) # Convert feature to float
# Save the cleaned dataset
data.to_csv('data/cleaned_data.csv', index=False)
This script demonstrates how to load, clean, and preprocess data using pandas, preparing it for model training.
Training Machine Learning Models
Building and Training the Model
Once the data is prepared, the next step is to build and train the machine learning model. scikit-learn provides a wide range of algorithms for classification, regression, and clustering tasks.
Here is an example of building and training a simple linear regression model:

import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
import joblib
# Load cleaned dataset
data = pd.read_csv('data/cleaned_data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Save the trained model
joblib.dump(model, 'app/models/model.pkl')
This script demonstrates how to build, train, and save a linear regression model using scikit-learn.
Evaluating Model Performance
Evaluating the model's performance is essential to ensure it meets the desired accuracy and reliability. Metrics such as mean squared error (MSE), R-squared, and mean absolute error (MAE) are commonly used for evaluation.
Here is an example of evaluating a trained model:
from sklearn.metrics import mean_squared_error, r2_score
import joblib
# Load the trained model
model = joblib.load('app/models/model.pkl')
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
print(f"R-squared: {r2}")
This script demonstrates how to evaluate a model's performance using common metrics.

Saving and Loading Models
Saving and loading models is a crucial part of deploying machine learning models as REST APIs. joblib and pickle are commonly used for this purpose.
Here is an example of saving and loading a model using joblib:
import joblib
# Save the model
joblib.dump(model, 'app/models/model.pkl')
# Load the model
loaded_model = joblib.load('app/models/model.pkl')
# Make predictions with the loaded model
y_pred_loaded = loaded_model.predict(X_test)
This script demonstrates how to save and load a model using joblib, ensuring that the model can be easily deployed and reused.
Developing the REST API
Setting Up Flask for API Development
Flask is a lightweight web framework for Python that is ideal for developing REST APIs. It provides the necessary tools to create endpoints, handle requests, and serve responses.

Here is an example of setting up a basic Flask API:
from flask import Flask, request, jsonify
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('app/models/model.pkl')
@app.route('/')
def home():
return "Welcome to the Machine Learning API!"
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
This script sets up a Flask API with a prediction endpoint, allowing users to send data and receive predictions from the model.
Creating Endpoints for Model Predictions
Creating endpoints for model predictions involves defining routes and handling HTTP requests. Flask makes it easy to create these endpoints and process incoming data.
Here is an example of creating a prediction endpoint in Flask:

from flask import Flask, request, jsonify
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('app/models/model.pkl')
@app.route('/predict', methods=['POST'])
def predict():
try:
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
except Exception as e:
return jsonify({'error': str(e)})
if __name__ == '__main__':
app.run(debug=True)
This script enhances the prediction endpoint with error handling, ensuring that any issues with the request are properly communicated back to the user.
Using FastAPI for High-Performance APIs
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python. It is based on standard Python type hints and offers automatic interactive API documentation.
Here is an example of setting up a FastAPI application:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
import joblib
import numpy as np
app = FastAPI()
# Load the trained model
model = joblib.load('app/models/model.pkl')
class Features(BaseModel):
features: list
@app.get("/")
def read_root():
return {"message": "Welcome to the Machine Learning API with FastAPI!"}
@app.post("/predict")
def predict(features: Features):
try:
data = np.array(features.features).reshape(1, -1)
prediction = model.predict(data)
return {"prediction": prediction.tolist()}
except Exception as e:
raise HTTPException(status_code=400, detail=str(e))
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
This script sets up a FastAPI application with a prediction endpoint, providing an alternative to Flask for creating high-performance APIs.
Testing and Deploying the API
Writing Unit Tests
Writing unit tests ensures that your API functions correctly and reliably. Tools like pytest can be used to write and run tests for your API endpoints.
Here is an example of writing unit tests for a Flask API using pytest:
import pytest
from app.main import app
@pytest.fixture
def client():
with app.test_client() as client:
yield client
def test_home(client):
response = client.get('/')
assert response.status_code == 200
assert response.json == {"message": "Welcome to the Machine Learning API with FastAPI!"}
def test_predict(client):
response = client.post('/predict', json={"features": [1, 2, 3, 4, 5]})
assert response.status_code == 200
assert "prediction" in response.json
This script demonstrates how to write unit tests for a Flask API, ensuring that the endpoints return the expected responses.
Deploying the API on Heroku
Heroku is a popular platform for deploying web applications. Deploying your Flask or FastAPI application on Heroku involves creating a Procfile
, setting up a Git repository, and pushing the code to Heroku.
Here is an example of deploying a Flask API on Heroku:
- Create a
Procfile
with the following content:web: gunicorn app.main:app
- Initialize a Git repository:
git init git add . git commit -m "Initial commit"
- Create a new Heroku app:
heroku create your-app-name
- Deploy the app to Heroku:
bash git push heroku master
This series of commands deploys your Flask API to Heroku, making it accessible online.
Scaling and Monitoring the API
Scaling and monitoring your API ensures that it can handle increased traffic and maintains optimal performance. Tools like Prometheus and Grafana can be used to monitor metrics, while Kubernetes can help with scaling.
Here is an example of setting up monitoring for a Flask API:
- Install Prometheus and Grafana:
pip install prometheus_client
- Add Prometheus metrics to your Flask app:
from prometheus_client import start_http_server, Summary REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request') @app.before_first_request def start_metrics_server(): start_http_server(8001) @REQUEST_TIME.time() def process_request(t): pass
- Configure Grafana to visualize the metrics collected by Prometheus.
This setup allows you to monitor your Flask API's performance and ensure it scales effectively with demand.
Best Practices for API Development
Securing the API
Securing your API is crucial to protect it from unauthorized access and potential attacks. Implementing authentication, using HTTPS, and validating input are essential security measures.
Here is an example of adding basic authentication to a Flask API:
from flask import Flask, request, jsonify
from functools import wraps
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('app/models/model.pkl')
def check_auth(username, password):
return username == 'admin' and password == 'secret'
def authenticate():
return jsonify({"message": "Authentication Required"}), 401
def requires_auth(f):
@wraps(f)
def decorated(*args, **kwargs):
auth = request.authorization
if not auth or not check_auth(auth.username, auth.password):
return authenticate()
return f(*args, **kwargs)
return decorated
@app.route('/predict', methods=['POST'])
@requires_auth
def predict():
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
This script adds basic authentication to the prediction endpoint, ensuring that only authorized users can access the API.
Logging and Monitoring
Implementing logging and monitoring helps track the API's performance and troubleshoot issues. Flask and FastAPI provide built-in support for logging.
Here is an example of adding logging to a Flask API:
import logging
from flask import Flask, request, jsonify
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('app/models/model.pkl')
# Set up logging
logging.basicConfig(level=logging.INFO)
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
app.logger.info(f"Prediction made for input: {data['features']}")
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
This script demonstrates how to add logging to a Flask API, helping monitor its usage and performance.
Documentation and Testing
Providing comprehensive documentation and thorough testing ensures that your API is easy to use and reliable. Tools like Swagger and Postman can help document and test your API.
Here is an example of generating API documentation with FastAPI:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
import joblib
import numpy as np
app = FastAPI()
# Load the trained model
model = joblib.load('app/models/model.pkl')
class Features(BaseModel):
features: list
@app.get("/")
def read_root():
return {"message": "Welcome to the Machine Learning API with FastAPI!"}
@app.post("/predict")
def predict(features: Features):
try:
data = np.array(features.features).reshape(1, -1)
prediction = model.predict(data)
return {"prediction": prediction.tolist()}
except Exception as e:
raise HTTPException(status_code=400, detail=str(e))
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
FastAPI automatically generates interactive API documentation, making it easy for users to understand and test your API endpoints.
By following these best practices and leveraging the power of Flask, FastAPI, and other tools, you can effectively deploy machine learning models as REST APIs. This approach not only enhances the accessibility of your models but also ensures scalability, security, and maintainability, driving more value from your machine learning projects.
If you want to read more articles similar to Machine Learning Models for REST APIs: A Comprehensive Guide, you can visit the Applications category.
You Must Read