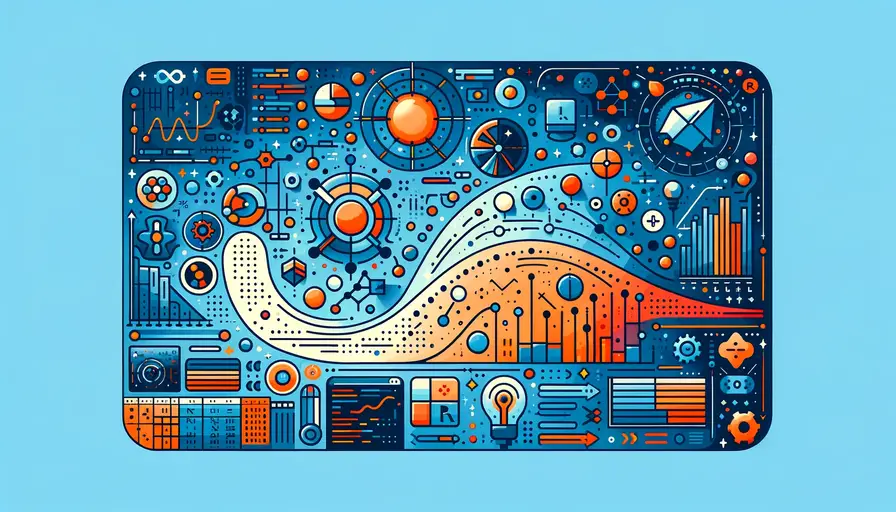
Machine Learning Models with Memory Integration

Integrating memory into machine learning models enhances their ability to learn and retain information over time, allowing them to perform better on sequential data tasks. This concept is central to various advanced neural network architectures like Recurrent Neural Networks (RNNs), Long Short-Term Memory (LSTM) units, Gated Recurrent Units (GRUs), and attention mechanisms. These architectures are designed to process and remember information, making them highly effective for tasks involving sequences, such as time-series forecasting, natural language processing, and speech recognition.
Recurrent Neural Networks (RNNs) to Incorporate Memory Into Machine Learning Models
Recurrent Neural Networks (RNNs) are a type of neural network specifically designed to handle sequential data. Unlike traditional feedforward neural networks, RNNs have loops that allow information to be passed from one step of the sequence to the next. This architecture enables RNNs to maintain a memory of previous inputs, making them well-suited for tasks where the order of data points is important.
One significant challenge with standard RNNs is their inability to retain information over long sequences due to issues like vanishing and exploding gradients. To address this, more advanced variants such as Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) networks have been developed. These variants include mechanisms that help the network retain information over longer periods, improving their performance on tasks with long-term dependencies.
LSTMs and GRUs introduce gating mechanisms that regulate the flow of information, allowing the network to learn which information to keep and which to discard. These gates help solve the vanishing gradient problem and enable the network to maintain a more stable learning process. As a result, LSTMs and GRUs have become the standard for many sequential data tasks, outperforming standard RNNs.

The benefits of integrating memory into machine learning models are substantial. By maintaining a memory of previous inputs, these models can make more informed predictions and better handle data with temporal dependencies. This capability is crucial for applications like language modeling, where the meaning of a word often depends on the words that come before it.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Build an LSTM model
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=(100, 1)))
model.add(Dense(1))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Model summary
model.summary()
Long Short-term Memory (LSTM) Units to Enable Models to Retain Information Over Longer Sequences
Long Short-Term Memory (LSTM) units are a type of RNN architecture explicitly designed to handle long-term dependencies. Unlike standard RNNs, LSTM units have a more complex structure that includes a cell state and three gating mechanisms: the input gate, the forget gate, and the output gate. These gates control the flow of information into, out of, and within the LSTM cell, allowing the network to retain and update information over long sequences.
The input gate determines how much of the new information should be added to the cell state. The forget gate decides how much of the existing information should be retained or discarded. Finally, the output gate controls how much of the information in the cell state should be passed to the next hidden state. This structure enables LSTM units to maintain a stable gradient, mitigating the vanishing gradient problem that plagues standard RNNs.
LSTM units are particularly beneficial for tasks involving long-term dependencies, such as language modeling, machine translation, and time-series forecasting. By effectively retaining relevant information over extended sequences, LSTMs can make more accurate predictions and better understand context, leading to improved performance in these applications.

The benefits of using LSTM units extend beyond just handling long sequences. They also improve the model's ability to learn complex patterns and relationships within the data. This capability is crucial for applications that require understanding nuanced temporal dynamics, such as speech recognition and video analysis. As a result, LSTMs have become a foundational component of many state-of-the-art neural network architectures.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Build an LSTM model
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=(100, 1)))
model.add(Dense(1))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Model summary
model.summary()
Employ Attention Mechanisms to Focus on Relevant Information and Improve Model Performance
Attention mechanisms are a crucial development in neural network architectures, particularly for tasks involving sequential data. They allow the model to focus on specific parts of the input sequence when making predictions, improving the model's ability to capture relevant information. This mechanism is especially useful for tasks like machine translation, where the meaning of a word can depend heavily on its context within the sentence.
Attention mechanisms work by assigning weights to different parts of the input sequence, highlighting the most relevant information for the task at hand. This process enables the model to concentrate on specific inputs that are more important for generating accurate outputs. As a result, attention mechanisms have become a standard component in many advanced neural network architectures, such as Transformers.
Integrating attention mechanisms into neural networks significantly enhances their performance on complex tasks. For instance, in machine translation, attention allows the model to align the source and target languages more effectively, resulting in more accurate translations. Similarly, in image captioning, attention mechanisms help the model focus on specific parts of the image to generate more descriptive and relevant captions.

The benefits of integrating attention mechanisms extend to various other applications, including speech recognition, text summarization, and sentiment analysis. By improving the model's ability to focus on relevant information, attention mechanisms enhance the overall performance and accuracy of neural networks, making them more effective for a wide range of tasks.
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, LSTM, Dense, Attention
# Define the inputs
input_seq = Input(shape=(None, 128))
output_seq = Input(shape=(None, 128))
# Define the LSTM layers
encoder_lstm = LSTM(128, return_sequences=True)(input_seq)
decoder_lstm = LSTM(128, return_sequences=True)(output_seq)
# Define the attention mechanism
attention = Attention()([encoder_lstm, decoder_lstm])
# Define the output layer
output = Dense(128, activation='softmax')(attention)
# Create the model
model = Model([input_seq, output_seq], output)
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy')
# Model summary
model.summary()
External Memory Systems
External memory systems represent a significant advancement in integrating memory into machine learning models. These systems provide a mechanism for models to read from and write to an external memory matrix, allowing them to store and retrieve information dynamically. This capability enhances the model's ability to handle tasks that require long-term memory and complex reasoning, such as language modeling, problem-solving, and game playing.
Neural Turing Machines (NTMs) are a prime example of models incorporating external memory systems. NTMs extend neural networks with a differentiable memory bank, enabling the network to learn read and write operations through gradient descent. This architecture allows NTMs to perform tasks traditionally challenging for neural networks, such as copying sequences, sorting, and associative recall.
The integration of external memory systems into neural networks offers several benefits. Firstly, it enhances the model's ability to retain and retrieve large amounts of information, improving performance on tasks with long-term dependencies. Secondly, it provides a flexible mechanism for dynamic memory management, allowing the model to adapt to different tasks and requirements. This flexibility is crucial for applications that require complex reasoning and memory-intensive operations.

Applications of memory integration in neural networks are vast and varied. In natural language processing, models with external memory can maintain context over long conversations, leading to more coherent and contextually relevant responses. In reinforcement learning, external memory systems enable agents to remember past experiences and use them to inform future decisions, enhancing learning efficiency and performance.
import torch
import torch.nn as nn
class NeuralTuringMachine(nn.Module):
def __init__(self, input_size, output_size, memory_size, memory_dim):
super(NeuralTuringMachine, self).__init__()
self.memory = torch.zeros(memory_size, memory_dim)
self.controller = nn.LSTM(input_size, output_size)
self.read_head = nn.Linear(output_size, memory_dim)
self.write_head = nn.Linear(output_size, memory_dim)
def forward(self, x):
controller_output, _ = self.controller(x)
read_vector = self.read_head(controller_output)
write_vector = self.write_head(controller_output)
self.memory += torch.mm(write_vector.t(), read_vector)
return read_vector
# Instantiate the model
ntm = NeuralTuringMachine(input_size=10, output_size=20, memory_size=128, memory_dim=40)
# Example input
x = torch.randn(5, 10)
# Forward pass
output = ntm(x)
print(output)
Integrating memory into machine learning models through RNNs, LSTMs, GRUs, attention mechanisms, and external memory systems significantly enhances their performance on sequential data tasks. These advancements enable models to retain and utilize relevant information more effectively, leading to improved accuracy and robustness in various applications. By leveraging these techniques, practitioners can build more powerful and versatile machine learning models that excel in complex, memory-intensive tasks.
If you want to read more articles similar to Machine Learning Models with Memory Integration, you can visit the Applications category.
You Must Read