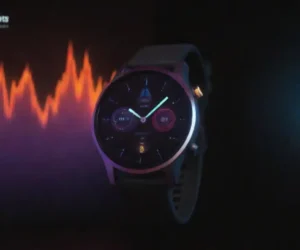
Mastering Machine Learning: Training and Deploying Models with Python
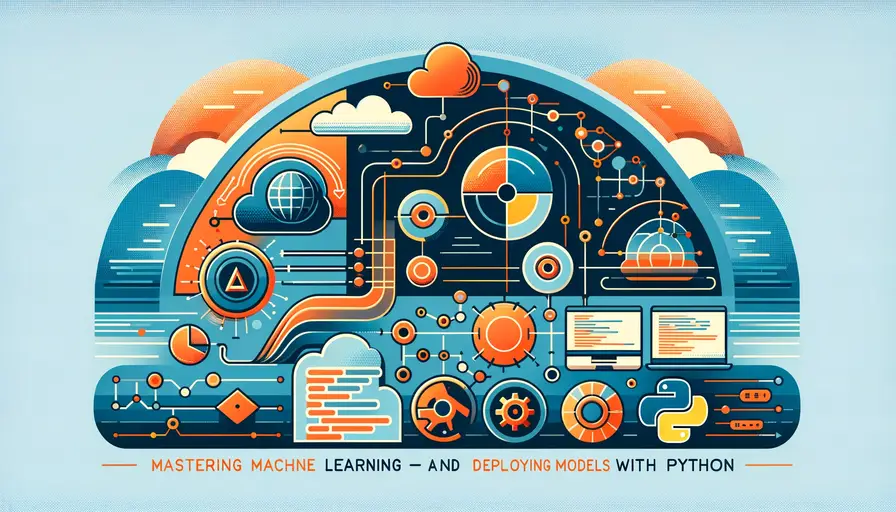
Machine learning has become a cornerstone in modern data-driven decision-making processes. Python, with its rich ecosystem of libraries and tools, is a popular language for building and deploying machine learning models. This article explores the complete workflow of training and deploying machine learning models using Python. From preparing the data and selecting algorithms to evaluating performance and deploying models in real-world applications, we will cover the essential steps and techniques required to master machine learning with Python.
Preparing Data for Machine Learning
Data Collection and Cleaning
The quality of data significantly impacts the performance of machine learning models. Data collection involves gathering relevant information from various sources, such as databases, APIs, or web scraping. Once collected, data often needs cleaning to handle missing values, remove duplicates, and correct inconsistencies.
Cleaning data ensures that the dataset is suitable for analysis and modeling. Techniques such as imputation for missing values, outlier detection, and normalization are commonly used. Python libraries like pandas and NumPy provide powerful functions for data manipulation and cleaning.
Example of data cleaning using pandas
:
import pandas as pd
# Load the dataset
data = pd.read_csv('data.csv')
# Display the first few rows of the dataset
print("Original Data:")
print(data.head())
# Handle missing values by filling them with the mean
data.fillna(data.mean(), inplace=True)
# Remove duplicate rows
data.drop_duplicates(inplace=True)
# Display the cleaned dataset
print("\nCleaned Data:")
print(data.head())
Feature Engineering
Feature engineering involves creating new features or modifying existing ones to improve model performance. This process includes techniques like encoding categorical variables, scaling numerical features, and creating interaction terms. Effective feature engineering can enhance the predictive power of machine learning models.
Tools like scikit-learn offer various preprocessing functions to facilitate feature engineering. For example, the LabelEncoder
and OneHotEncoder
classes can transform categorical variables, while StandardScaler
and MinMaxScaler
can normalize numerical features.
Example of feature engineering using scikit-learn
:
from sklearn.preprocessing import LabelEncoder, StandardScaler
# Encode categorical variables
label_encoder = LabelEncoder()
data['category'] = label_encoder.fit_transform(data['category'])
# Scale numerical features
scaler = StandardScaler()
data[['numerical_feature1', 'numerical_feature2']] = scaler.fit_transform(data[['numerical_feature1', 'numerical_feature2']])
print("\nTransformed Data:")
print(data.head())
Splitting Data
Splitting the data into training and testing sets is a crucial step in model training. The training set is used to train the model, while the testing set evaluates its performance. A common split ratio is 80/20, where 80% of the data is used for training and 20% for testing. This approach ensures that the model generalizes well to new, unseen data.
Rising Demand for AI and Machine Learning Specialists in TechThe train_test_split function from scikit-learn
simplifies this process by randomly splitting the dataset while maintaining the distribution of target variables.
Example of splitting data using scikit-learn
:
from sklearn.model_selection import train_test_split
# Define features and target variable
X = data.drop('target', axis=1)
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
print(f'Training set size: {X_train.shape[0]}')
print(f'Testing set size: {X_test.shape[0]}')
Training Machine Learning Models
Selecting Algorithms
Choosing the right algorithm is critical for building an effective machine learning model. Different algorithms are suited for different types of data and tasks. For instance, linear regression is ideal for continuous target variables, while decision trees and random forests are suitable for classification tasks.
Commonly used algorithms include:
Machine Learning vs Neural Networks: The Battle for Supremacy- Linear Regression: For predicting continuous outcomes.
- Logistic Regression: For binary classification.
- Decision Trees: For both classification and regression tasks.
- Random Forests: An ensemble method that improves prediction accuracy.
- Support Vector Machines (SVM): For both classification and regression with high-dimensional data.
- K-Nearest Neighbors (KNN): A simple algorithm for classification and regression based on proximity.
Example of training a decision tree classifier using scikit-learn
:
from sklearn.tree import DecisionTreeClassifier
# Initialize the decision tree classifier
model = DecisionTreeClassifier(random_state=42)
# Train the model
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
print("Predictions:")
print(y_pred)
Hyperparameter Tuning
Hyperparameter tuning involves optimizing the parameters of a model to improve its performance. Techniques such as grid search and random search systematically explore different combinations of hyperparameters to find the best settings.
Grid search evaluates all possible combinations of specified hyperparameters, while random search randomly samples a subset of combinations. Libraries like scikit-learn provide tools like GridSearchCV
and RandomizedSearchCV
for hyperparameter tuning.
Example of hyperparameter tuning using GridSearchCV
in scikit-learn
:
from sklearn.model_selection import GridSearchCV
# Define the hyperparameter grid
param_grid = {
'max_depth': [None, 10, 20, 30],
'min_samples_split': [2, 5, 10],
'min_samples_leaf': [1, 2, 4]
}
# Initialize the grid search
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5, scoring='accuracy')
# Perform the grid search
grid_search.fit(X_train, y_train)
# Display the best hyperparameters
print(f'Best Hyperparameters: {grid_search.best_params_}')
print(f'Best Cross-Validation Accuracy: {grid_search.best_score_}')
Model Evaluation
Evaluating the performance of machine learning models is crucial to ensure their reliability. Common evaluation metrics for classification tasks include accuracy, precision, recall, and F1 score. For regression tasks, metrics such as mean squared error (MSE), mean absolute error (MAE), and R-squared are used.
Confusion matrices, ROC curves, and precision-recall curves provide visual insights into model performance. Evaluating models on multiple metrics ensures a comprehensive assessment of their strengths and weaknesses.
Example of evaluating a classifier using scikit-learn
:
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
# Calculate evaluation metrics
accuracy = accuracy_score(y_test, y_pred)
precision = precision_score(y_test, y_pred, average='weighted')
recall = recall_score(y_test, y_pred, average='weighted')
f1 = f1_score(y_test, y_pred, average='weighted')
print(f'Accuracy: {accuracy}')
print(f'Precision: {precision}')
print(f'Recall: {recall}')
print(f'F1 Score: {f1}')
Deploying Machine Learning Models
Model Serialization
Serialization involves saving a trained model to a file so it can be loaded and used later without retraining. This process is essential for deploying models in production environments. Python libraries like joblib and pickle are commonly used for model serialization.
The Formula for Calculating the F-Score in Machine LearningExample of serializing and deserializing a model using joblib
:
import joblib
# Save the trained model to a file
joblib.dump(model, 'decision_tree_model.joblib')
# Load the model from the file
loaded_model = joblib.load('decision_tree_model.joblib')
# Make predictions using the loaded model
loaded_predictions = loaded_model.predict(X_test)
print("Loaded Model Predictions:")
print(loaded_predictions)
Building REST APIs with Flask
Deploying machine learning models often involves creating REST APIs to serve predictions. Flask is a lightweight web framework in Python that can be used to build APIs for machine learning models. Flask allows you to create endpoints that accept input data, make predictions using the trained model, and return the results.
Example of a Flask API for a machine learning model:
from flask import Flask, request, jsonify
import joblib
import numpy as np
# Load the trained model
model = joblib.load('decision_tree_model.joblib')
# Initialize Flask app
app = Flask(__name__)
# Define the prediction endpoint
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
Continuous Integration and Deployment (CI/CD)
Implementing CI/CD pipelines ensures that machine learning models are continuously tested, integrated, and deployed. Tools like Jenkins, GitHub Actions, and GitLab CI automate the deployment process, ensuring that models are updated and deployed efficiently.
Supervised Machine Learning Types: Exploring the Different ApproachesCI/CD pipelines typically include stages for data validation, model training, evaluation, and deployment. Automating these stages ensures that models are always up-to-date and that any issues are detected early.
Example of a simple CI/CD pipeline configuration using GitHub Actions:
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.8'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
pytest
- name: Deploy to production
if: github.ref == 'refs/heads/main'
run: |
# Add deployment script here
Practical Applications and Case Studies
Healthcare
Machine learning models are transforming healthcare by enabling predictive analytics, personalized treatment, and efficient diagnostics. For example, models can predict disease outbreaks, analyze medical images for diagnosis, and recommend personalized treatment plans based on patient data.
Deploying these models in real-world healthcare applications requires rigorous evaluation, robust deployment practices, and continuous monitoring to ensure accuracy and reliability. Ensuring patient data privacy and compliance with regulations is also critical.
Finance
In finance, machine learning models are used for credit scoring, fraud detection, and algorithmic trading. Accurate models can assess creditworthiness, detect fraudulent transactions, and optimize trading strategies, leading to better financial decisions and increased profitability.
Deploying machine learning models in the finance sector involves integrating with existing financial systems, ensuring data security, and complying with financial regulations. Continuous monitoring and retraining of models are essential to adapt to changing market conditions and maintain accuracy.
Marketing
Machine learning models enhance marketing strategies by enabling customer segmentation, predictive analytics, and personalized recommendations. Models can analyze customer data to identify target segments, predict customer behavior, and recommend products or services.
Deploying models in marketing involves integrating with customer relationship management (CRM) systems, ensuring data privacy, and continuously updating models based on new customer data. Effective deployment strategies lead to improved customer engagement and increased sales.
Example of a marketing use case with a machine learning model:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load customer data
data = pd.read_csv('customer_data.csv')
# Define features and target variable
X = data.drop('purchase', axis=1)
y = data['purchase']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
# Serialize the model
import joblib
joblib.dump(model, 'marketing_model.joblib')
Future Directions and Research Opportunities
Explainable AI
Explainable AI (XAI) focuses on making machine learning models interpretable and transparent. As models become more complex, understanding how they make decisions is crucial for gaining trust and ensuring fairness. Future research in XAI will develop techniques to explain model predictions, making them more accessible to stakeholders.
AutoML
Automated Machine Learning (AutoML) aims to automate the process of model selection, hyperparameter tuning, and feature engineering. AutoML tools like Auto-sklearn and TPOT simplify the machine learning workflow, making it accessible to non-experts and accelerating model development.
Federated Learning
Federated learning is a distributed approach to machine learning where models are trained across multiple devices or servers without sharing raw data. This technique enhances privacy and security, making it suitable for applications involving sensitive data. Future research will focus on improving federated learning algorithms and addressing challenges related to data heterogeneity and communication efficiency.
Mastering machine learning with Python involves a comprehensive workflow of data preparation, model training, evaluation, and deployment. By leveraging powerful libraries and tools, data scientists can build robust and scalable machine learning models for various applications. Continuous advancements in explainable AI, AutoML, and federated learning will further enhance the capabilities and accessibility of machine learning, driving innovation across different domains.
If you want to read more articles similar to Mastering Machine Learning: Training and Deploying Models with Python, you can visit the Artificial Intelligence category.
You Must Read