
Maximize Your Data: Discovering the Optimal Value for Feature Scaling
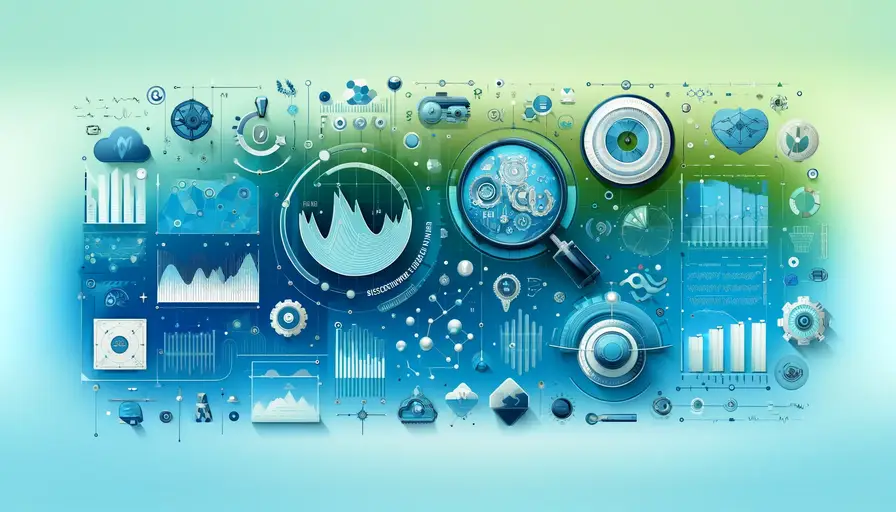
Content
Feature Scaling Fundamentals
Feature scaling is a crucial preprocessing step in data analysis and machine learning. It involves transforming the features of your dataset so that they lie within a specific range or follow a specific distribution. This transformation ensures that each feature contributes equally to the analysis, preventing any single feature from dominating the model due to its scale.
In many machine learning algorithms, the distance between data points is used to determine the relationships within the data. When features are on vastly different scales, this distance can be skewed, leading to biased or inaccurate models. Feature scaling helps mitigate this issue by standardizing the scales of all features.
There are several methods for feature scaling, each with its own advantages and appropriate use cases. Understanding these methods and their applications is key to effectively scaling your data and improving your model's performance.
Why is Feature Scaling Important?
Feature scaling is important because it ensures that all features contribute equally to the analysis, improving the performance and reliability of machine learning models. Algorithms such as k-nearest neighbors (KNN), support vector machines (SVM), and principal component analysis (PCA) are particularly sensitive to the scale of features. Without proper scaling, these algorithms may produce biased results, as features with larger scales can disproportionately influence the outcome.
ROC and Precision-Recall Curves in PythonIn addition to improving model accuracy, feature scaling can also accelerate the convergence of gradient-based optimization algorithms. When features are on similar scales, the optimization process can proceed more smoothly and quickly, leading to faster training times. This is especially beneficial for deep learning models, where training can be computationally intensive.
Finally, feature scaling helps in the interpretability of the model. By standardizing the scales of features, it becomes easier to compare the relative importance of different features and understand their impact on the model. This can provide valuable insights into the underlying structure of the data and guide further analysis.
Different Types of Feature Scaling Methods
Standardization
Standardization is a widely used feature scaling method that transforms features to have a zero mean and unit variance. This method is particularly useful when the data follows a Gaussian distribution. Standardization ensures that each feature contributes equally to the model, preventing features with larger scales from dominating.
The formula for standardization is:
$$z = \frac{(x - \mu)}{\sigma}$$
where \(x\) is the original feature value, \(\mu\) is the mean of the feature, and \(\sigma\) is the standard deviation.
Here’s an example of standardization in Python using scikit-learn
:
from sklearn.preprocessing import StandardScaler
# Sample data
data = [[1, 2], [3, 4], [5, 6], [7, 8], [9, 10]]
# Initialize the standard scaler
scaler = StandardScaler()
# Fit and transform the data
scaled_data = scaler.fit_transform(data)
print(scaled_data)
This code demonstrates how to standardize features to have zero mean and unit variance.
Normalization
Normalization scales features to a specific range, usually between 0 and 1. This method is useful when the data does not follow a Gaussian distribution and when you want to maintain the relative distances between features. Normalization is particularly effective for algorithms that use distance measures, such as KNN and SVM.
The formula for normalization is:
$$x' = \frac{(x - x_{min})}{(x_{max} - x_{min})}$$
where \(x\) is the original feature value, \(x_{min}\) is the minimum value of the feature, and \(x_{max}\) is the maximum value of the feature.
Here’s an example of normalization in Python using scikit-learn
:
from sklearn.preprocessing import MinMaxScaler
# Sample data
data = [[1, 2], [3, 4], [5, 6], [7, 8], [9, 10]]
# Initialize the min-max scaler
scaler = MinMaxScaler()
# Fit and transform the data
normalized_data = scaler.fit_transform(data)
print(normalized_data)
This code demonstrates how to normalize features to a specific range.
Min-Max Scaling
Min-max scaling is a specific type of normalization that scales features to lie between a specified minimum and maximum value. This method is particularly useful when the range of feature values is known and consistent. Min-max scaling ensures that all features are on a similar scale, improving the performance of machine learning algorithms that are sensitive to feature scales.
The formula for min-max scaling is:
$$x' = \frac{(x - x_{min})}{(x_{max} - x_{min})} \times (new_{max} - new_{min}) + new_{min}$$
where \(x\) is the original feature value, \(x_{min}\) and \(x_{max}\) are the minimum and maximum values of the feature, and \(new_{min}\) and \(new_{max}\) are the desired minimum and maximum values after scaling.
Here’s an example of min-max scaling in Python using scikit-learn
:
from sklearn.preprocessing import MinMaxScaler
# Sample data
data = [[1, 2], [3, 4], [5, 6], [7, 8], [9, 10]]
# Initialize the min-max scaler with specific range
scaler = MinMaxScaler(feature_range=(0, 1))
# Fit and transform the data
scaled_data = scaler.fit_transform(data)
print(scaled_data)
This code demonstrates how to scale features between specified minimum and maximum values.
Median and IQR Scaling
Median and interquartile range (IQR) scaling is a robust scaling method that is less sensitive to outliers. This method scales features based on the median and IQR, providing a more stable transformation when the data contains extreme values. Median and IQR scaling is particularly useful when the dataset has a skewed distribution.
The formula for median and IQR scaling is:
$$z = \frac{(x - \text{median})}{\text{IQR}}$$
where \(x\) is the original feature value, \(\text{median}\) is the median of the feature, and \(\text{IQR}\) is the interquartile range (the difference between the 75th and 25th percentiles).
Here’s an example of median and IQR scaling in Python:
import numpy as np
# Sample data
data = np.array([[1, 2], [3, 4], [5, 6], [7, 8], [9, 10]])
# Calculate median and IQR
median = np.median(data, axis=0)
iqr = np.percentile(data, 75, axis=0) - np.percentile(data, 25, axis=0)
# Perform scaling
scaled_data = (data - median) / iqr
print(scaled_data)
This code demonstrates how to scale features using median and IQR.
Choosing the Right Feature Scaling Method for Your Data
Choosing the right feature scaling method depends on the nature of your data and the requirements of your machine learning algorithm. Each scaling method has its own advantages and is suited for different types of data and applications. Understanding the characteristics of your data and the needs of your model is essential for selecting the most appropriate scaling technique.
For instance, if your data follows a Gaussian distribution, standardization might be the best choice. Standardization ensures that features have zero mean and unit variance, which can improve the performance of algorithms that assume normally distributed data, such as linear regression and logistic regression.
Can Machine Learning Classification Be Validated for Accuracy?On the other hand, if your data does not follow a Gaussian distribution or contains outliers, normalization or median and IQR scaling might be more appropriate. Normalization scales features to a specific range, preserving the relative distances between them, while median and IQR scaling provides a robust transformation that is less sensitive to extreme values.
Experimenting with different scaling methods and evaluating their impact on model performance is a good practice. Cross-validation can be used to compare the performance of different scaling techniques and select the one that provides the best results for your specific application.
Challenges and Considerations in Feature Scaling
Common Challenges
Common challenges in feature scaling include handling outliers, dealing with categorical variables, and selecting the appropriate scaling method. Outliers can significantly impact the scaling process, especially when using methods like min-max scaling or standardization, which are sensitive to extreme values. Identifying and addressing outliers before scaling is crucial to ensure accurate transformations.
Another challenge is scaling categorical variables, which require different techniques than continuous variables. One-hot encoding is commonly used for nominal variables, while label encoding is suitable for ordinal variables. Ensuring that these encoded variables are appropriately scaled is important for maintaining the integrity of the data.
Choosing the right scaling method can also be challenging, as it depends on the characteristics of the data and the requirements of the machine learning algorithm. Experimenting with different methods and evaluating their impact on model performance can help identify the most suitable scaling technique.
Best Practices
Best practices for feature scaling involve understanding the data, selecting the appropriate scaling method, and evaluating the impact on model performance. Begin by exploring the data to identify its distribution, presence of outliers, and the types of variables. This initial exploration helps in selecting the most appropriate scaling technique.
When scaling features, it's important to apply the same transformation to both the training and test data to ensure consistency. Failing to do so can lead to discrepancies in model performance and biased results. Using pipelines in scikit-learn
can help automate this process and ensure that the same scaling is applied consistently.
Regularly evaluate the impact of feature scaling on model performance using cross-validation. This approach provides a robust assessment of how different scaling methods affect the model's accuracy and generalizability. Continuously refining the scaling process based on these evaluations can lead to more reliable and effective models.
Importance of Feature Scaling in Data Analysis
Feature scaling plays a vital role in data analysis, ensuring that all features contribute equally to the analysis and improving the performance of machine learning models. Without proper scaling, features with larger scales can dominate the analysis, leading to biased or inaccurate results. Scaling helps create a level playing field for all features, enhancing the reliability and accuracy of the analysis.
In addition to improving model performance, feature scaling can enhance the interpretability of the results. When features are on similar scales, it's easier to compare their relative importance and understand their impact on the model. This understanding can provide valuable insights into the underlying structure of the data and guide further analysis.
Overall, feature scaling is an essential step in the data preprocessing pipeline, ensuring that machine learning models perform optimally and produce reliable, interpretable results. By understanding the fundamentals of feature scaling and selecting the appropriate methods for your data, you can maximize the potential of your data and improve the performance of your machine learning models.
If you want to read more articles similar to Maximize Your Data: Discovering the Optimal Value for Feature Scaling, you can visit the Performance category.
You Must Read