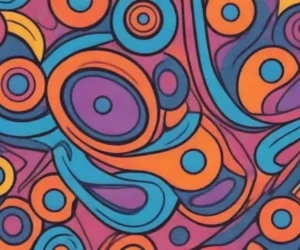
Maximizing Decision Tree Performance with Machine Learning
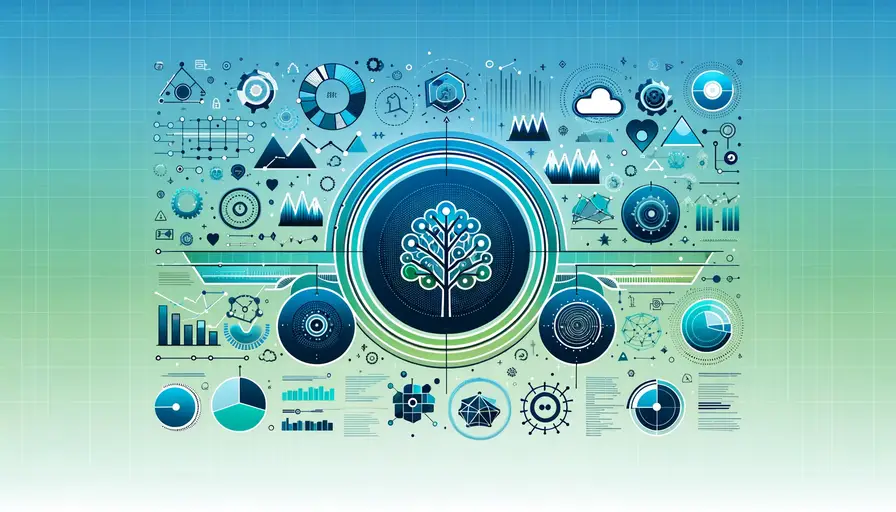
- Use Feature Selection Techniques
- Perform Data Preprocessing
- Implement Ensemble Methods
- Tune Hyperparameters
- Implement Cross-Validation
- Use Boosting Techniques
- Handle Class Imbalance
- Implement Pruning Techniques
- Use Different Splitting Criteria
- Incorporate Feature Engineering
- Gather More Data
- Implement Early Stopping
Use Feature Selection Techniques
Univariate Selection
Univariate selection involves evaluating each feature individually to determine its significance in predicting the target variable. This method uses statistical tests, such as chi-squared for categorical data and ANOVA for continuous data, to assess the importance of each feature. The most relevant features are selected based on their scores.
Here's an example of univariate selection using Scikit-learn:
from sklearn.datasets import load_iris
from sklearn.feature_selection import SelectKBest, chi2
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Apply univariate feature selection
selector = SelectKBest(chi2, k=2)
X_new = selector.fit_transform(X, y)
print(X_new)
This code demonstrates how to perform univariate selection to identify important features.
Recursive Feature Elimination
Recursive Feature Elimination (RFE) is a technique that recursively removes less important features and builds a model on the remaining features. It continues this process until the specified number of features is reached. RFE helps in identifying the features that contribute the most to the model's performance.
Strategies for Zero-Inflated Data in Machine Learning AlgorithmsHere's an example of RFE using Scikit-learn:
from sklearn.datasets import load_iris
from sklearn.feature_selection import RFE
from sklearn.tree import DecisionTreeClassifier
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Apply RFE with Decision Tree
estimator = DecisionTreeClassifier()
selector = RFE(estimator, n_features_to_select=2, step=1)
selector = selector.fit(X, y)
print(selector.support_)
print(selector.ranking_)
This code shows how to use RFE to select important features for a decision tree.
Perform Data Preprocessing
Clean the Dataset
Cleaning the dataset involves handling missing values, correcting data types, and removing duplicates. This step ensures that the data is consistent and reliable for model training. Missing values can be handled by imputation or removal, while incorrect data types need to be converted to appropriate formats.
Here's an example of cleaning a dataset using Pandas:
Exploring Gradient Descent in Linear Regressionimport pandas as pd
# Sample data
data = {'Feature1': [1, 2, None, 4], 'Feature2': ['A', 'B', 'B', None]}
df = pd.DataFrame(data)
# Handle missing values
df['Feature1'].fillna(df['Feature1'].mean(), inplace=True)
df['Feature2'].fillna('Unknown', inplace=True)
# Convert data types if necessary
# df['Feature1'] = df['Feature1'].astype(int)
print(df)
This code demonstrates basic data cleaning techniques.
Normalize the Dataset
Normalizing the dataset ensures that features are on a similar scale, which can improve the performance of many machine learning algorithms. Normalization scales the data to a range of [0, 1] or standardizes it to have a mean of zero and a standard deviation of one.
Here's an example of normalizing a dataset using Scikit-learn:
import pandas as pd
from sklearn.preprocessing import MinMaxScaler
# Sample data
data = {'Feature1': [10, 20, 30, 40], 'Feature2': [1, 2, 3, 4]}
df = pd.DataFrame(data)
# Normalize the data
scaler = MinMaxScaler()
df_scaled = scaler.fit_transform(df)
print(df_scaled)
This code normalizes the features in a dataset to a [0, 1] range.
Feature Selection Methods in scikit-learn: A Comprehensive OverviewHandle Categorical Variables
Handling categorical variables is essential for incorporating categorical data into machine learning models. Common techniques include one-hot encoding and label encoding. One-hot encoding creates binary columns for each category, while label encoding assigns a unique integer to each category.
Here's an example of one-hot encoding using Pandas:
import pandas as pd
# Sample data
data = {'Color': ['Red', 'Blue', 'Green', 'Blue']}
df = pd.DataFrame(data)
# Apply one-hot encoding
df_encoded = pd.get_dummies(df, columns=['Color'])
print(df_encoded)
This code demonstrates how to apply one-hot encoding to categorical variables.
Split the Dataset
Splitting the dataset into training and testing sets is crucial for evaluating the model's performance. The training set is used to build the model, while the testing set is used to assess its accuracy and generalization.
Exploring Machine Learning Techniques for Feature SelectionHere's an example of splitting a dataset using Scikit-learn:
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
print(X_train.shape, X_test.shape)
This code splits the dataset into training and testing sets.
Handle Imbalanced Classes
Handling imbalanced classes is important to ensure the model performs well across all classes. Techniques include oversampling the minority class, undersampling the majority class, and using synthetic data generation methods like SMOTE (Synthetic Minority Over-sampling Technique).
Here's an example of using SMOTE to handle imbalanced classes:
Optimizing Performance: AI Feedback and Reinforcement Learningfrom imblearn.over_sampling import SMOTE
from sklearn.datasets import make_classification
# Create imbalanced dataset
X, y = make_classification(n_samples=1000, n_features=2, n_informative=2, n_redundant=0, n_clusters_per_class=1, weights=[0.99], flip_y=0, random_state=1)
# Apply SMOTE
smote = SMOTE()
X_resampled, y_resampled = smote.fit_resample(X, y)
print(X_resampled.shape, y_resampled.shape)
This code demonstrates how to use SMOTE to balance an imbalanced dataset.
Implement Ensemble Methods
Implementing ensemble methods such as random forests can significantly improve a decision tree's performance. Random forests combine multiple decision trees to create a more robust and accurate model by averaging their predictions and reducing overfitting.
Random Forests
Random forests build multiple decision trees on different subsets of the dataset and aggregate their results. This approach reduces the variance and improves the model's generalization ability.
Here's an example of implementing a random forest using Scikit-learn:
PCA: An Unsupervised Dimensionality Reduction Techniquefrom sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Create and train the random forest classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X, y)
print(clf.feature_importances_)
This code demonstrates how to create and train a random forest classifier.
Tune Hyperparameters
Maximum Depth
Tuning the maximum depth (max_depth) of a decision tree helps control overfitting. A deeper tree can capture more complex patterns but may overfit the training data, while a shallower tree may underfit.
Here's an example of tuning max_depth using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import GridSearchCV
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the model
model = DecisionTreeClassifier()
# Define the grid of parameters
param_grid = {'max_depth': [3, 5, 7, 9]}
# Apply GridSearchCV
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
print(grid_search.best_params_)
This code tunes the maximum depth of a decision tree.
Minimum Samples Split
Tuning the minimum samples split (min_samples_split) parameter helps control the growth of the tree by setting the minimum number of samples required to split an internal node.
Here's an example of tuning min_samples_split using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import GridSearchCV
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the model
model = DecisionTreeClassifier()
# Define the grid of parameters
param_grid = {'min_samples_split': [2, 5, 10]}
# Apply GridSearchCV
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
print(grid_search.best_params_)
This code tunes the minimum samples split of a decision tree.
Minimum Samples Leaf
Tuning the minimum samples leaf (min_samples_leaf) parameter ensures that each leaf node has a minimum number of samples, preventing the model from learning noise in the data.
Here's an example of tuning min_samples_leaf using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import GridSearchCV
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the model
model = DecisionTreeClassifier()
# Define the grid of parameters
param_grid = {'min_samples_leaf': [1, 2, 4]}
# Apply GridSearchCV
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
print(grid_search.best_params_)
This code tunes the minimum samples leaf of a decision tree.
Maximum Features
Tuning the maximum features (max_features) parameter controls the number of features to consider when looking for the best split. This parameter can help balance the trade-off between bias and variance.
Here's
an example of tuning max_features using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import GridSearchCV
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the model
model = DecisionTreeClassifier()
# Define the grid of parameters
param_grid = {'max_features': [2, 3, 4]}
# Apply GridSearchCV
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
print(grid_search.best_params_)
This code tunes the maximum features of a decision tree.
Criterion
Tuning the criterion parameter involves selecting the function used to measure the quality of a split. Common criteria include Gini impurity and information gain (entropy).
Here's an example of tuning the criterion using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import GridSearchCV
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the model
model = DecisionTreeClassifier()
# Define the grid of parameters
param_grid = {'criterion': ['gini', 'entropy']}
# Apply GridSearchCV
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
print(grid_search.best_params_)
This code tunes the criterion of a decision tree.
Implement Cross-Validation
Cross-Validation Benefits
Cross-validation is a technique used to evaluate the performance of a model on different subsets of the data. It helps ensure that the model's performance is consistent and not dependent on a specific train-test split. Cross-validation can also help in detecting overfitting.
Here's an example of applying cross-validation using Scikit-learn:
from sklearn.model_selection import cross_val_score
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the model
model = DecisionTreeClassifier()
# Apply cross-validation
scores = cross_val_score(model, X, y, cv=5)
print(scores)
print("Average score:", scores.mean())
This code demonstrates how to apply cross-validation to evaluate a decision tree's performance.
Use Boosting Techniques
Boosting techniques like AdaBoost can enhance the accuracy of decision trees by combining the outputs of multiple weak learners to create a strong learner. Boosting iteratively adjusts the weights of the training instances, focusing more on the harder-to-predict examples.
AdaBoost
AdaBoost (Adaptive Boosting) is a popular boosting technique that combines multiple weak classifiers to form a strong classifier. Each classifier is trained on the weighted data, and weights are updated based on the classifier's accuracy.
Here's an example of implementing AdaBoost using Scikit-learn:
from sklearn.ensemble import AdaBoostClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Define the base model
base_model = DecisionTreeClassifier(max_depth=1)
# Apply AdaBoost
model = AdaBoostClassifier(base_model, n_estimators=50, random_state=42)
model.fit(X, y)
print(model.score(X, y))
This code demonstrates how to implement AdaBoost to enhance a decision tree's accuracy.
Handle Class Imbalance
Oversampling
Oversampling involves increasing the number of instances in the minority class to balance the dataset. Techniques like SMOTE generate synthetic samples to achieve this balance, improving the model's performance on the minority class.
Here's an example of oversampling using SMOTE:
from imblearn.over_sampling import SMOTE
from sklearn.datasets import make_classification
# Create imbalanced dataset
X, y = make_classification(n_samples=1000, n_features=2, n_informative=2, n_redundant=0, n_clusters_per_class=1, weights=[0.99], flip_y=0, random_state=1)
# Apply SMOTE
smote = SMOTE()
X_resampled, y_resampled = smote.fit_resample(X, y)
print(X_resampled.shape, y_resampled.shape)
This code demonstrates how to use SMOTE for oversampling.
Undersampling
Undersampling involves reducing the number of instances in the majority class to balance the dataset. This technique can help the model learn equally from both classes but may result in loss of information from the majority class.
Here's an example of undersampling using Scikit-learn:
from imblearn.under_sampling import RandomUnderSampler
from sklearn.datasets import make_classification
# Create imbalanced dataset
X, y = make_classification(n_samples=1000, n_features=2, n_informative=2, n_redundant=0, n_clusters_per_class=1, weights=[0.99], flip_y=0, random_state=1)
# Apply undersampling
rus = RandomUnderSampler()
X_resampled, y_resampled = rus.fit_resample(X, y)
print(X_resampled.shape, y_resampled.shape)
This code demonstrates how to apply undersampling to balance the dataset.
Implement Pruning Techniques
Types of Pruning
Pruning techniques such as pre-pruning and post-pruning help prevent overfitting in decision trees. Pre-pruning stops the tree from growing beyond a certain point during training, while post-pruning removes branches from a fully grown tree that do not provide additional predictive power.
Here's an example of post-pruning using cost complexity pruning in Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Train the decision tree
clf = DecisionTreeClassifier(random_state=42)
path = clf.cost_complexity_pruning_path(X, y)
ccp_alphas, impurities = path.ccp_alphas, path.impurities
# Create a list of pruned trees
clfs = [DecisionTreeClassifier(random_state=42, ccp_alpha=alpha).fit(X, y) for alpha in ccp_alphas]
print("Number of nodes in the last tree is: {} with ccp_alpha: {}".format(clfs[-1].tree_.node_count, ccp_alphas[-1]))
This code demonstrates how to apply post-pruning using cost complexity pruning.
Benefits of Pruning
Pruning benefits include reducing the complexity of the decision tree, which helps improve its generalization ability and performance on new data. By eliminating branches that add little value, pruning reduces the risk of overfitting and makes the model more interpretable.
Use Different Splitting Criteria
Different splitting criteria such as information gain and Gini index help determine the best splits in a decision tree. Information gain measures the reduction in entropy, while Gini index measures the impurity of a node.
Information Gain
Information gain is used to measure the effectiveness of a split. It calculates the reduction in entropy before and after the split, with higher values indicating better splits.
Here's an example of setting the splitting criterion to information gain using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Train the decision tree with information gain
clf = DecisionTreeClassifier(criterion='entropy', random_state=42)
clf.fit(X, y)
print(clf.tree_.node_count)
This code demonstrates how to use information gain as the splitting criterion.
Gini Index
Gini index measures the impurity of a node. A split that results in pure nodes (nodes with only one class) has a Gini index of 0, while nodes with a mix of classes have higher Gini values.
Here's an example of setting the splitting criterion to Gini index using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Train the decision tree with Gini index
clf = DecisionTreeClassifier(criterion='gini', random_state=42)
clf.fit(X, y)
print(clf.tree_.node_count)
This code demonstrates how to use Gini index as the splitting criterion.
Incorporate Feature Engineering
Feature Selection
Feature selection involves choosing the most relevant features to include in the model. Techniques like univariate selection, recursive feature elimination, and feature importance from tree-based models help identify the best features.
Feature Scaling
Feature scaling ensures that all features contribute equally to the model by transforming them to a common scale. Techniques like normalization and standardization are commonly used.
Here's an example of feature scaling using Scikit-learn:
from sklearn.preprocessing import StandardScaler
import pandas as pd
# Sample data
data = {'Feature1': [10, 20, 30, 40], 'Feature2': [100, 200, 300, 400]}
df = pd.DataFrame(data)
# Standardize the data
scaler = StandardScaler()
df_scaled = scaler.fit_transform(df)
print(df_scaled)
This code standardizes the features in a dataset.
Feature Encoding
Feature encoding converts categorical variables into numerical values. Techniques like one-hot encoding and label encoding are commonly used to handle categorical data.
Here's an example of one-hot encoding using Pandas:
import pandas as pd
# Sample
data
data = {'Color': ['Red', 'Blue', 'Green', 'Blue']}
df = pd.DataFrame(data)
# Apply one-hot encoding
df_encoded = pd.get_dummies(df, columns=['Color'])
print(df_encoded)
This code demonstrates how to apply one-hot encoding to categorical variables.
Gather More Data
Gathering more data can improve the decision tree's performance by providing additional examples for training. More data helps the model learn better and generalize well to new examples.
Increasing Training Set
Increasing the training set enhances the model's ability to learn patterns and relationships in the data. This approach can lead to better performance and more accurate predictions.
Feature Selection and Ensemble Methods
Utilizing feature selection techniques and applying ensemble methods can further enhance the decision tree's performance. By carefully selecting features and combining multiple models, you can build a robust and accurate predictive model.
Implement Early Stopping
Early stopping techniques prevent the decision tree from growing too deep and overfitting. By stopping the growth of the tree when further splits do not significantly improve performance, you can ensure better generalization.
Here's an example of implementing early stopping using Scikit-learn:
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Train the decision tree with early stopping
clf = DecisionTreeClassifier(random_state=42, max_depth=5)
clf.fit(X, y)
print(clf.tree_.node_count)
This code demonstrates how to apply early stopping by limiting the maximum depth of the tree.
Maximizing decision tree performance involves a combination of feature selection, data preprocessing, hyperparameter tuning, and using advanced techniques like ensemble methods and boosting. By implementing these strategies, you can build more robust, accurate, and efficient decision tree models for machine learning applications.
If you want to read more articles similar to Maximizing Decision Tree Performance with Machine Learning, you can visit the Algorithms category.
You Must Read