
Optimal Frequency for Regression Testing: How Often is Ideal?
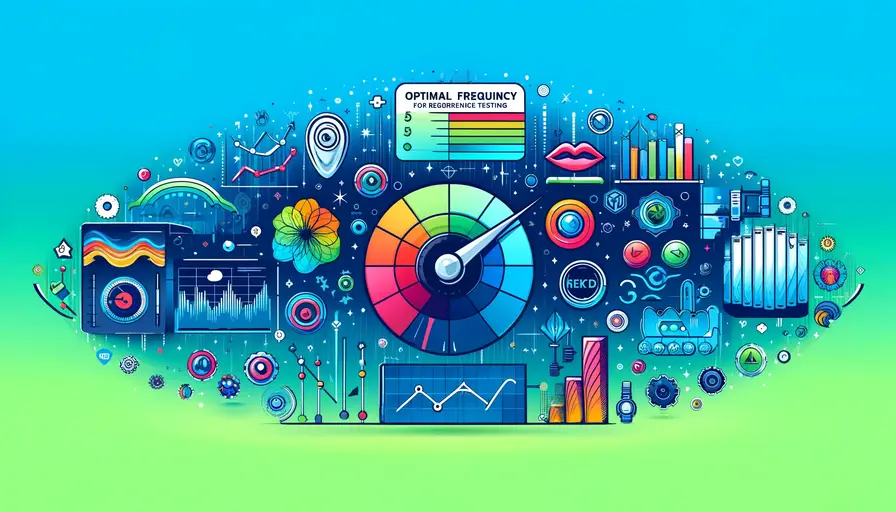
Content
Importance of Regression Testing
Ensuring Software Quality
Regression testing is a critical aspect of software development, aimed at ensuring that recent code changes do not adversely affect the existing functionality of the application. This type of testing is essential for maintaining software quality over time. By continuously verifying that new updates or bug fixes do not introduce new errors, regression testing helps in preserving the integrity of the software.
Regular regression testing can catch bugs early in the development cycle, reducing the cost and time associated with fixing issues later. It acts as a safeguard against unintended consequences of code modifications, ensuring that the software remains stable and reliable. This process is particularly crucial in complex systems where changes in one part of the code can have cascading effects on other parts of the system.
In addition to catching bugs, regression testing also helps in maintaining user confidence. Users expect consistent performance and reliability from software products. Frequent regression testing ensures that updates do not degrade the user experience, thereby maintaining trust and satisfaction. For businesses, this translates into reduced downtime and fewer customer support issues, ultimately protecting the company's reputation.
Managing Risk in Development
Regression testing is a key strategy for managing risk in software development. Every change in the codebase, whether it's a new feature, an improvement, or a bug fix, carries the risk of introducing new issues. Regression testing helps mitigate these risks by validating that the existing functionality continues to work as intended after each change.
Securing and Ensuring Reliability of Your Machine Learning PipelineBy incorporating regression testing into the development process, teams can identify potential issues before they reach production. This proactive approach to quality assurance minimizes the risk of costly post-release defects, which can lead to significant financial and reputational damage. Moreover, it allows development teams to move quickly and confidently, knowing that they have a safety net to catch regressions.
Effective regression testing also involves prioritizing test cases based on the areas of the application most likely to be affected by changes. This targeted approach ensures that testing efforts are focused where they are most needed, maximizing the efficiency and effectiveness of the testing process. By managing risk through strategic regression testing, organizations can achieve a balance between rapid development and high-quality software delivery.
Example: Implementing Regression Tests with Selenium in Python
from selenium import webdriver
import unittest
class RegressionTest(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
self.driver.get("https://www.example.com")
def test_example(self):
driver = self.driver
element = driver.find_element_by_id("exampleElement")
self.assertEqual(element.text, "Expected Text")
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
In this example, Selenium is used to perform regression testing in Python. The script sets up a web driver, navigates to a webpage, and verifies that a specific element contains the expected text. This simple test ensures that recent changes have not affected the specified functionality, demonstrating the importance of regular regression testing in maintaining software quality.
Determining the Optimal Frequency for Regression Testing
Factors Influencing Testing Frequency
Several factors influence the optimal frequency for regression testing, including the size and complexity of the codebase, the frequency of code changes, and the criticality of the application. For instance, a complex application with frequent updates may require more frequent regression testing compared to a simpler application with less frequent changes. The goal is to balance the need for thorough testing with the resources available.
Top SVM Models for Machine Learning: An ExplorationThe development methodology also plays a significant role in determining the testing frequency. Agile methodologies, which involve frequent iterations and continuous integration, often necessitate more regular regression testing to ensure that each iteration does not introduce new defects. In contrast, traditional waterfall methodologies might have less frequent but more comprehensive regression testing phases.
Resource availability, including the availability of testing tools and the capacity of the testing team, is another crucial factor. Automated regression testing tools can significantly reduce the time and effort required for testing, allowing for more frequent testing cycles. However, the initial setup and maintenance of automated tests require investment in time and resources. Balancing these factors helps in determining the optimal frequency for regression testing.
Agile Development and Continuous Integration
In Agile development environments, frequent code changes and continuous integration (CI) are standard practices. This iterative approach to development demands regular regression testing to ensure that each new code commit does not break existing functionality. The CI pipeline typically includes automated regression tests that run with each build, providing immediate feedback to developers.
The integration of regression testing into the CI pipeline helps in identifying issues early, reducing the time and cost associated with fixing defects. Automated tests run consistently across different environments, ensuring that code changes are thoroughly validated before being merged into the main codebase. This approach aligns with the Agile principles of rapid and continuous delivery of high-quality software.
Pros and Cons of Random Forest AlgorithmBy adopting continuous integration and automated regression testing, development teams can maintain a high level of code quality while accelerating the delivery process. This practice also fosters a culture of collaboration and accountability, as developers receive immediate feedback on their changes and can address issues promptly. In Agile environments, the combination of CI and regression testing is essential for achieving efficient and reliable software development.
Example: Setting Up Continuous Integration with Jenkins
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'make build'
}
}
stage('Test') {
steps {
sh 'make test'
}
}
stage('Regression Test') {
steps {
sh 'pytest --regression'
}
}
stage('Deploy') {
steps {
sh 'make deploy'
}
}
}
post {
always {
junit 'reports/**/*.xml'
archiveArtifacts artifacts: 'target/*.jar', fingerprint: true
}
}
}
In this example, a Jenkins pipeline is set up to include regression testing as part of the CI process. The pipeline defines stages for building, testing, regression testing, and deploying the application. The pytest command with the --regression
flag runs the regression tests, ensuring that any new changes do not introduce defects. This setup highlights the integration of regression testing into the CI pipeline, a best practice in Agile development.
Balancing Frequency and Resources
Determining the optimal frequency for regression testing involves balancing the need for thorough testing with the available resources. While more frequent testing can catch defects earlier and improve software quality, it also requires more time and resources. Therefore, it is essential to strike a balance that maximizes the benefits of regression testing while minimizing the impact on development productivity.
One approach to balancing frequency and resources is to prioritize regression tests based on the criticality of the features being tested. High-priority tests, which cover the most critical and frequently used features, can be run more frequently, while lower-priority tests can be run less often. This prioritization ensures that the most important aspects of the application are always thoroughly tested.
Time-Based Machine Learning MethodsAnother approach is to leverage automated testing tools to reduce the time and effort required for regression testing. Automated tests can be run more frequently without significantly impacting the development timeline. Additionally, by using tools that support parallel execution of tests, you can further reduce the time required for regression testing, allowing for more frequent testing cycles without overburdening the testing team.
Implementing Effective Regression Testing Strategies
Prioritizing Test Cases
Effective regression testing requires prioritizing test cases based on various factors such as feature criticality, usage frequency, and historical defect patterns. Prioritizing test cases ensures that the most critical and impactful areas of the application are tested first, maximizing the effectiveness of the regression testing process.
Feature criticality refers to the importance of a feature to the overall functionality and user experience of the application. Critical features that directly impact the core functionality should be tested more frequently and thoroughly. Usage frequency considers how often a feature is used by the end-users; frequently used features are more likely to encounter defects and should be prioritized in the testing process.
Historical defect patterns provide insights into areas of the application that have previously encountered issues. By analyzing past defect data, you can identify high-risk areas that require more rigorous testing. This data-driven approach to prioritizing test cases ensures that resources are focused on areas that are most likely to have defects, improving the efficiency and effectiveness of the regression testing process.
Using Python for Time Series Machine Learning: A Comprehensive GuideLeveraging Automated Testing
Automated testing is a powerful strategy for implementing effective regression testing. Automated tests can be run frequently and consistently, providing immediate feedback on code changes. This approach significantly reduces the time and effort required for manual testing, allowing the testing team to focus on more complex and exploratory testing activities.
Automated testing tools such as Selenium, JUnit, and TestNG provide robust frameworks for creating and executing regression tests. These tools support a wide range of testing activities, including functional testing, performance testing, and integration testing. By integrating automated testing into the CI pipeline, you can ensure that regression tests are run with every code commit, providing continuous validation of the application.
In addition to traditional automated testing tools, modern solutions such as Cypress and Playwright offer advanced features for end-to-end testing. These tools provide capabilities such as real-time testing, parallel test execution, and integration with CI/CD pipelines. By leveraging these advanced tools, you can enhance the efficiency and effectiveness of your regression testing process, ensuring that your application remains stable and reliable.
Example: Automating Regression Tests with Cypress
// cypress/integration/regression_test.js
describe('Regression Test Suite', () => {
it('should load the home page', () => {
cy.visit('https://www.example.com');
cy.contains('Welcome to Example');
});
it('should navigate to the about page', () => {
cy.get('a[href="/about"]').click();
cy.contains('About Us');
});
it('should submit the contact form', () => {
cy.get('a[href="/contact"]').click();
cy.get('input[name="name"]').type('John Doe');
cy.get('input[name="email"]').type('john.doe@example.com');
cy.get('textarea[name="message"]').type('Hello, this is a test message.');
cy.get('button[type="submit"]').click();
cy.contains('Thank you for your message');
});
});
In this example, Cypress is used to automate regression tests for a web application. The script includes tests for loading the home page, navigating to the about page, and submitting the contact form. By automating these tests, you can ensure that the core functionality of the application is continuously validated with every code change.
Comprehensive Guide to Machine Learning PipelinesMonitoring and Maintenance
Continuous monitoring and maintenance of regression tests are essential to ensure their effectiveness over time. As the application evolves, regression tests need to be updated to reflect new features, changes in functionality, and bug fixes. Regularly reviewing and updating test cases helps maintain their relevance and accuracy, ensuring that they continue to provide reliable validation.
Monitoring the results of regression tests is also crucial for identifying patterns and trends in defects. By analyzing test results, you can gain insights into areas of the application that are prone to issues and take proactive measures to address them. This data-driven approach to monitoring helps in improving the overall quality and stability of the application.
Maintenance of regression tests also involves managing test data and environments. Ensuring that test data is representative of real-world scenarios and that test environments are consistent and reliable is critical for accurate test results. Regularly cleaning and updating test data, as well as maintaining the integrity of test environments, helps in achieving accurate and meaningful regression testing outcomes.
Real-World Examples and Case Studies
E-Commerce Platforms
E-commerce platforms are complex systems that require frequent updates and changes to stay competitive. Regression testing is crucial for ensuring that new features, promotions, and changes do not disrupt the user experience. For instance, adding a new payment gateway or updating the product catalog can have wide-reaching impacts on the platform's functionality.
In e-commerce, automated regression testing can be employed to validate critical workflows such as user registration, product search, checkout process, and payment transactions. By integrating regression tests into the CI pipeline, e-commerce companies can ensure that these workflows remain functional and efficient, even with frequent updates.
Companies like Amazon and eBay use automated regression testing extensively to maintain the quality and reliability of their platforms. These companies leverage advanced testing tools and techniques to continuously validate their systems, ensuring that their customers have a seamless shopping experience.
Healthcare Applications
Healthcare applications handle sensitive and critical data, making regression testing essential for ensuring their accuracy and reliability. Changes to these applications, whether they involve new features, bug fixes, or security updates, must be thoroughly tested to prevent any adverse effects on patient data and functionality.
Automated regression testing can be used to validate key functionalities such as patient data entry, appointment scheduling, medication tracking, and report generation. These tests help ensure that the application remains functional and secure, even as new updates are introduced.
Healthcare providers and companies like Epic Systems and Cerner rely on rigorous regression testing to maintain the integrity of their applications. By continuously validating their systems through automated tests, these organizations can provide reliable and secure healthcare solutions to their users.
Example: Regression Testing in Healthcare Applications
import unittest
from healthcare_app import app, db
class RegressionTest(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
self.db = db
def test_patient_registration(self):
response = self.app.post('/register', data=dict(
name='John Doe',
age=30,
gender='Male',
email='john.doe@example.com'
))
self.assertEqual(response.status_code, 200)
self.assertIn(b'Registration successful', response.data)
def test_appointment_scheduling(self):
response = self.app.post('/schedule', data=dict(
patient_id=1,
doctor_id=2,
date='2023-05-10',
time='10:00 AM'
))
self.assertEqual(response.status_code, 200)
self.assertIn(b'Appointment scheduled', response.data)
def tearDown(self):
pass
if __name__ == '__main__':
unittest.main()
In this example, unittest is used to perform regression tests on a healthcare application. The tests validate the patient registration and appointment scheduling functionalities, ensuring that these critical features work correctly after recent updates.
Financial Services
Financial services applications deal with transactions, customer data, and regulatory compliance, making regression testing a vital part of their development process. Ensuring that changes do not affect transaction accuracy, security, or compliance is crucial for maintaining trust and reliability.
Automated regression testing can be employed to validate transactions, account management, reporting, and compliance checks. By continuously testing these functionalities, financial services companies can ensure the integrity and security of their applications.
Banks and financial institutions like JPMorgan Chase and Goldman Sachs use automated regression testing to maintain the quality of their software systems. These organizations leverage advanced testing frameworks and tools to ensure that their applications meet the highest standards of reliability and security.
Determining the optimal frequency for regression testing involves balancing the need for thorough testing with the available resources. By prioritizing test cases, leveraging automated testing tools, and integrating regression tests into the CI pipeline, organizations can achieve efficient and effective regression testing. Regular monitoring and maintenance of regression tests ensure their continued relevance and accuracy, helping organizations deliver high-quality software consistently. Through real-world examples and case studies, it is evident that effective regression testing is crucial for maintaining the quality and reliability of complex software systems across various industries.
If you want to read more articles similar to Optimal Frequency for Regression Testing: How Often is Ideal?, you can visit the Algorithms category.
You Must Read