
Optimal Machine Learning Algorithms for Training AI in Games
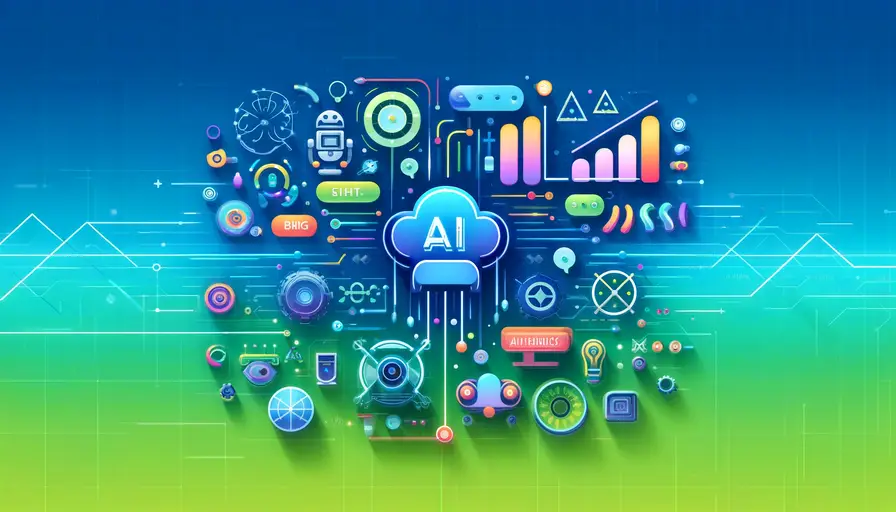
Machine Learning in Gaming
Machine learning has revolutionized the gaming industry, enabling the creation of sophisticated AI that can learn, adapt, and provide immersive experiences. From simple rule-based systems to complex neural networks, various machine learning algorithms are used to train AI in games.
Importance of AI in Gaming
AI in gaming enhances the player's experience by providing intelligent behavior for non-player characters (NPCs). It creates dynamic environments where NPCs can adapt to player actions, making games more challenging and engaging.
Evolution of Game AI
Game AI has evolved from basic scripts to advanced machine learning algorithms. Early games used simple if-then rules, while modern games employ complex models that can learn from data and improve over time.
Example: Evolution of AI in Games
Here’s a historical perspective on AI evolution in gaming:
- Early Games: Rule-based AI (e.g., Pac-Man)
- Mid-1990s: Pathfinding and finite state machines (e.g., Command & Conquer)
- 2000s: Behavior trees and decision trees (e.g., Halo)
- Present: Machine learning and deep learning (e.g., AlphaGo, OpenAI Five)
Reinforcement Learning
Reinforcement Learning (RL) is a powerful machine learning approach used extensively in game AI. RL enables agents to learn optimal strategies through trial and error.
What is Reinforcement Learning?
Reinforcement Learning involves training an agent to make decisions by rewarding desired behaviors and penalizing undesired ones. The agent learns to maximize cumulative rewards through exploration and exploitation.
Applications in Gaming
RL is used in gaming for tasks such as pathfinding, strategy optimization, and adaptive AI behaviors. It allows game AI to learn complex strategies that adapt to player actions.
Example: Q-Learning in R
Here’s an example of implementing Q-learning for game AI in R:
# Define environment
states <- c("Start", "End")
actions <- c("Left", "Right")
rewards <- matrix(c(-1, 0, 0, 1), nrow=2)
# Initialize Q-table
Q <- matrix(0, nrow=length(states), ncol=length(actions))
# Q-learning parameters
alpha <- 0.1 # Learning rate
gamma <- 0.9 # Discount factor
# Training loop
for (episode in 1:100) {
state <- "Start"
while (state != "End") {
action <- sample(actions, 1)
reward <- rewards[which(states == state), which(actions == action)]
next_state <- ifelse(action == "Right", "End", "Start")
Q[which(states == state), which(actions == action)] <- Q[which(states == state), which(actions == action)] + alpha * (reward + gamma * max(Q[which(states == next_state), ]) - Q[which(states == state), which(actions == action)])
state <- next_state
}
}
# Display Q-table
print(Q)
Deep Q-Networks (DQN)
Deep Q-Networks (DQN) combine the power of deep learning with Q-learning, enabling the training of AI in complex environments with high-dimensional state spaces.
Introduction to DQN
Deep Q-Networks (DQN) use neural networks to approximate the Q-values in reinforcement learning. This approach allows the agent to handle environments with large state and action spaces effectively.
Benefits of DQN
DQN can learn to play complex games by observing raw pixels and rewards, making it suitable for tasks such as game playing, robotics, and autonomous driving.
Example: DQN Implementation in R
Here’s an example of implementing DQN for game AI in R:
# Load necessary libraries
library(keras)
library(tensorflow)
# Define neural network model
model <- keras_model_sequential() %>%
layer_dense(units = 24, activation = 'relu', input_shape = c(4)) %>%
layer_dense(units = 24, activation = 'relu') %>%
layer_dense(units = 2, activation = 'linear')
# Compile model
model %>% compile(
loss = 'mse',
optimizer = optimizer_adam(lr = 0.001)
)
# DQN parameters
epsilon <- 1.0 # Exploration rate
gamma <- 0.95 # Discount factor
alpha <- 0.01 # Learning rate
# Training loop
for (episode in 1:1000) {
state <- env_reset()
done <- FALSE
while (!done) {
action <- ifelse(runif(1) < epsilon, sample(0:1, 1), which.max(predict(model, state)))
next_state, reward, done <- env_step(action)
target <- reward + gamma * max(predict(model, next_state))
target_f <- predict(model, state)
target_f[1, action + 1] <- target
model %>% fit(state, target_f, epochs = 1, verbose = 0)
state <- next_state
}
if (epsilon > 0.1) epsilon <- epsilon * 0.995
}
Genetic Algorithms
Genetic Algorithms (GAs) are inspired by natural selection and are used in games to evolve optimal strategies and behaviors.
What are Genetic Algorithms?
Genetic Algorithms use mechanisms inspired by biological evolution, such as selection, crossover, and mutation. They are used to find approximate solutions to optimization and search problems.
Applications in Gaming
GAs are used in gaming for optimizing game parameters, evolving NPC behaviors, and procedural content generation. They enable the creation of diverse and adaptive game experiences.
Example: Genetic Algorithm in R
Here’s an example of implementing a genetic algorithm for game AI in R:
# Load necessary library
library(GA)
# Define fitness function
fitness_function <- function(x) {
# Example fitness calculation
return(sum(x^2))
}
# GA parameters
pop_size <- 50
num_genes <- 10
mutation_rate <- 0.1
# Run GA
ga_result <- ga(
type = "real-valued",
fitness = fitness_function,
lower = -10,
upper = 10,
popSize = pop_size,
maxiter = 100,
pmutation = mutation_rate
)
# Display best solution
print(ga_result@solution)
Monte Carlo Tree Search (MCTS)
Monte Carlo Tree Search (MCTS) is a heuristic search algorithm used for decision-making in game AI, particularly in games with large search spaces.
Introduction to MCTS
Monte Carlo Tree Search builds a search tree incrementally and uses random sampling to simulate possible future states of the game. It balances exploration and exploitation to find the optimal strategy.
Benefits of MCTS
MCTS is effective in games with large state spaces, such as board games and real-time strategy games. It can handle complex decision-making processes and adapt to dynamic environments.
Example: MCTS in R
Here’s an example of implementing MCTS for game AI in R:
# Define game environment
states <- c("State1", "State2", "State3")
actions <- c("Action1", "Action2")
rewards <- matrix(c(1, -1, 0, 1, -1, 0), nrow = 3)
# MCTS parameters
num_simulations <- 100
# MCTS function
mcts <- function(state) {
for (sim in 1:num_simulations) {
current_state <- state
while (TRUE) {
action <- sample(actions, 1)
reward <- rewards[which(states == current_state), which(actions == action)]
next_state <- ifelse(action == "Action1", "State2", "State3")
current_state <- next_state
if (current_state == "State3") break
}
}
return(action)
}
# Run MCTS
best_action <- mcts("State1")
print(best_action)
Neural Networks
Neural Networks (NN) are used in game AI to model complex relationships and behaviors. They are particularly effective in pattern recognition tasks.
What are Neural Networks?
Neural Networks are computational models inspired by the human brain. They consist of interconnected nodes (neurons) that process information in layers, enabling the learning of complex patterns.
Applications in Gaming
NNs are used in gaming for tasks such as image recognition, behavior modeling, and natural language processing. They can learn from large datasets and generalize to new situations.
Example: Neural Network in R
Here’s an example of implementing a neural network for game AI in R using the keras
package:
# Load necessary libraries
library(keras)
library(tensorflow)
# Define neural network model
model <- keras_model_sequential() %>%
layer_dense(units = 128, activation = 'relu', input_shape = c(10)) %>%
layer_dropout(rate = 0.2) %>%
layer_dense(units = 64, activation = 'relu') %>%
layer_dense(units = 2, activation = 'softmax')
# Compile model
model %>% compile(
loss = 'categorical_crossentropy',
optimizer = optimizer_adam(lr = 0.001),
metrics = c('accuracy')
)
# Example training data
x_train <- matrix(runif(1000), nrow = 100, ncol = 10)
y_train <- to_categorical(sample(0:1, 100, replace = TRUE))
# Train the model
model %>% fit(x_train, y_train, epochs = 20, batch_size = 16)
# Predict on new data
x_test <- matrix(runif(100), nrow = 10, ncol = 10)
predictions <- model %>% predict(x_test)
print(predictions)
Transfer Learning
Transfer Learning leverages pre-trained models to solve new but related tasks, reducing the amount of data and training time required.
What is Transfer Learning?
Transfer Learning involves using a pre-trained model on a new task, allowing the model to transfer knowledge from one domain to another. This is particularly useful when data is scarce.
Applications in Gaming
Transfer Learning is used in gaming to transfer knowledge from one game to another, enabling rapid development of game AI. It is also used for enhancing NPC behaviors and improving game design.
Example: Transfer Learning in R
Here’s an example of implementing transfer learning for game AI in R using the keras
package:
# Load necessary libraries
library(keras)
library(tensorflow)
# Load pre-trained model
base_model <- application_vgg16(weights = 'imagenet', include_top = FALSE, input_shape = c(224, 224, 3))
# Add custom layers
model <- keras_model_sequential() %>%
base_model %>%
layer_flatten() %>%
layer_dense(units = 256, activation = 'relu') %>%
layer_dense(units = 2, activation = 'softmax')
# Freeze base model layers
freeze_weights(base_model)
# Compile model
model %>% compile(
loss = 'categorical_crossentropy',
optimizer = optimizer_adam(lr = 0.001),
metrics = c('accuracy')
)
# Example training data
x_train <- array(runif(1000 * 224 * 224 * 3), dim = c(1000, 224, 224, 3))
y_train <- to_categorical(sample(0:1, 1000, replace = TRUE))
# Train the model
model %>% fit(x_train, y_train, epochs = 10, batch_size = 32)
# Predict on new data
x_test <- array(runif(100 * 224 * 224 * 3), dim = c(10, 224, 224, 3))
predictions <- model %>% predict(x_test)
print(predictions)
Reinforcement Learning with Deep Learning
Combining reinforcement learning with deep learning, also known as Deep Reinforcement Learning (DRL), allows for the creation of AI that can learn and make decisions in complex environments.
Introduction to Deep Reinforcement Learning
Deep Reinforcement Learning (DRL) combines the principles of reinforcement learning with deep learning. This approach enables the training of agents that can perceive the environment and take actions to maximize cumulative rewards.
Benefits of DRL in Gaming
DRL is particularly powerful for tasks such as game playing, where the environment is complex and dynamic. It allows AI to learn from raw sensory inputs and improve over time through self-play and experience.
Example: Deep Reinforcement Learning in R
Here’s an example of implementing a simple DRL model in R:
# Load necessary libraries
library(keras)
library(tensorflow)
# Define neural network model
model <- keras_model_sequential() %>%
layer_dense(units = 24, activation = 'relu', input_shape = c(4)) %>%
layer_dense(units = 24, activation = 'relu') %>%
layer_dense(units = 2, activation = 'linear')
# Compile model
model %>% compile(
loss = 'mse',
optimizer = optimizer_adam(lr = 0.001)
)
# Example environment (CartPole)
env <- make_cartpole()
# Training loop
for (episode in 1:1000) {
state <- env_reset(env)
done <- FALSE
while (!done) {
action <- ifelse(runif(1) < epsilon, sample(0:1, 1), which.max(predict(model, state)))
next_state, reward, done <- env_step(env, action)
target <- reward + gamma * max(predict(model, next_state))
target_f <- predict(model, state)
target_f[1, action + 1] <- target
model %>% fit(state, target_f, epochs = 1, verbose = 0)
state <- next_state
}
if (epsilon > 0.1) epsilon <- epsilon * 0.995
}
Machine learning algorithms have transformed the gaming industry, enabling the creation of intelligent and adaptive AI. From reinforcement learning and neural networks to genetic algorithms and Monte Carlo Tree Search, various algorithms are used to train AI for games. By understanding the principles and applications of these algorithms, game developers can create immersive and challenging experiences for players. Leveraging tools and frameworks in R, such as keras
, tensorflow
, and caret
, makes implementing these algorithms accessible and efficient. As technology continues to advance, the role of machine learning in gaming will only grow, opening up new possibilities for innovation and creativity in game development.
If you want to read more articles similar to Optimal Machine Learning Algorithms for Training AI in Games, you can visit the Artificial Intelligence category.
You Must Read