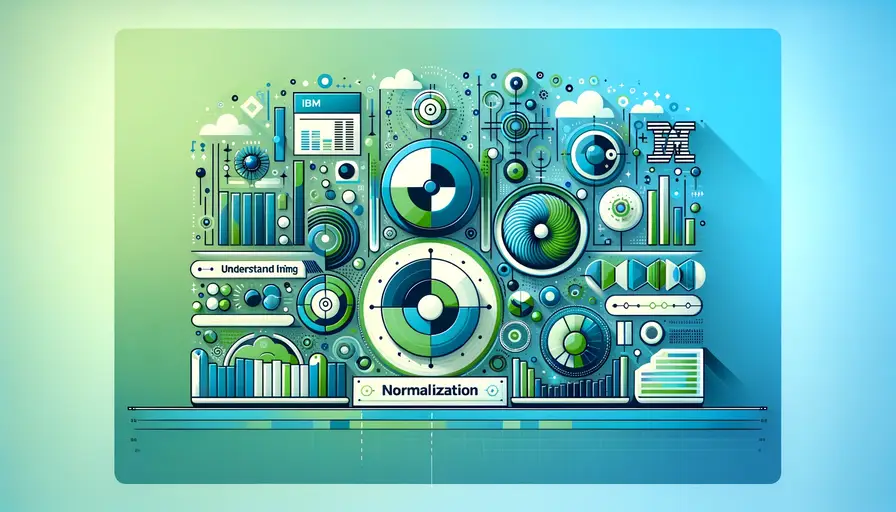
Optimizing Nested Data in Machine Learning Models

In machine learning, dealing with nested data can be challenging but rewarding when done correctly. Optimizing nested data requires a combination of feature engineering, memory optimization, algorithm selection, and parallel processing techniques. This guide provides an in-depth look at methods for handling nested data in machine learning models.
Feature Engineering Techniques
Feature engineering is crucial when working with nested data. It involves transforming raw data into features that better represent the underlying problem to predictive models, leading to improved model performance.
Flatten Nested Data
Flattening nested data involves transforming hierarchical structures into a flat format that can be easily processed by machine learning algorithms. This can be achieved through various methods, such as concatenating nested attributes or aggregating values.
Encode Categorical Variables
Encoding categorical variables is essential for machine learning models to process nested data. Techniques such as one-hot encoding, label encoding, and target encoding can transform categorical data into numerical values that models can understand.

Use Hierarchical Models
Hierarchical models are designed to handle nested data by explicitly modeling the hierarchical structure. These models consider the nested nature of the data, making them suitable for tasks involving grouped or multi-level data.
Consider Using Tree-based Algorithms
Tree-based algorithms like decision trees, random forests, and gradient boosting can naturally handle nested data structures. These algorithms can capture complex interactions within nested data without extensive preprocessing.
Flatten Nested Data Structure
Flattening nested data is a common preprocessing step that simplifies the data structure, making it easier to apply standard machine learning algorithms.
Strategies for Flattening Nested Data
Strategies for flattening nested data include using pandas' json_normalize
function to convert nested JSON objects into a flat table, or manually extracting nested attributes into separate columns. Another approach is to aggregate nested values, such as calculating statistical summaries (mean, median) for nested attributes.

import pandas as pd
import json
# Example nested data
nested_data = '{"id": 1, "name": "John", "address": {"city": "New York", "zip": "10001"}}'
data = json.loads(nested_data)
# Flatten nested data
df = pd.json_normalize(data)
print(df)
Best Practices for Flattening Nested Data
Best practices for flattening nested data involve ensuring that important information is not lost during the flattening process. Maintain a balance between flattening the data and preserving its hierarchical structure. Use meaningful column names to retain context and make the data more interpretable.
Tree-based Algorithms to Handle Nested Data
Tree-based algorithms are particularly effective for handling nested data due to their ability to model complex relationships without extensive preprocessing.
Strategies for Handling Nested Data With Tree-based Algorithms
Strategies for handling nested data with tree-based algorithms include directly feeding flattened data into the models or using feature engineering techniques to create meaningful features from nested attributes. Algorithms like random forests and gradient boosting can effectively handle the complexity of nested data.
from sklearn.ensemble import RandomForestClassifier
# Example flattened data
data = {'feature1': [1, 2, 3], 'feature2': [4, 5, 6], 'nested_feature': [7, 8, 9]}
df = pd.DataFrame(data)
# Train a random forest model
clf = RandomForestClassifier()
clf.fit(df[['feature1', 'feature2']], df['nested_feature'])
Optimize Memory Usage
Optimizing memory usage is critical when working with large nested datasets to prevent out-of-memory errors and ensure efficient processing.

Convert Nested Lists to Arrays
Converting nested lists to arrays can improve memory usage and computational efficiency. NumPy arrays consume less memory and provide faster operations compared to Python lists.
Utilize Sparse Representations
Utilizing sparse representations can significantly reduce memory usage for datasets with many zero or missing values. Sparse matrices store only the non-zero elements, which can be advantageous for certain types of nested data.
from scipy.sparse import csr_matrix
# Example sparse matrix
data = [[0, 0, 1], [1, 0, 0], [0, 1, 0]]
sparse_matrix = csr_matrix(data)
print(sparse_matrix)
Use Compressed Data Formats
Using compressed data formats like Parquet or Feather can reduce storage requirements and improve data loading times. These formats are particularly useful for large nested datasets.
Employ Dimensionality Reduction Techniques
Dimensionality reduction techniques such as PCA (Principal Component Analysis) and t-SNE (t-Distributed Stochastic Neighbor Embedding) can reduce the number of features while preserving important information, making the dataset more manageable.

Parallel Processing Techniques
Parallel processing can accelerate the handling of nested data by distributing computational tasks across multiple processors.
What is Parallel Processing?
Parallel processing involves dividing a computational task into smaller sub-tasks that are processed simultaneously on multiple processors. This approach can significantly reduce processing time for large and complex datasets.
Benefits of Parallel Processing for Nested Data
The benefits of parallel processing for nested data include faster data processing, improved model training times, and the ability to handle larger datasets. Parallel processing leverages modern multi-core processors to enhance computational efficiency.
Best Practices for Implementing Parallel Processing With Nested Data
Best practices for parallel processing with nested data include using libraries like Dask and Apache Spark, which provide high-level interfaces for parallel computing. Ensure that the data is partitioned effectively and that tasks are balanced across processors to maximize performance.

from dask import dataframe as dd
# Example using Dask for parallel processing
df = dd.read_csv('large_nested_data.csv')
df = df.compute()
print(df.head())
Regularize and Normalize Nested Data
Regularization and normalization are essential techniques to improve the performance and generalizability of machine learning models dealing with nested data.
Regularization Techniques for Nested Data
Regularization techniques such as L1 (Lasso) and L2 (Ridge) regularization can prevent overfitting by penalizing large coefficients in the model. These techniques ensure that the model generalizes well to new data.
Normalization Techniques for Nested Data
Normalization techniques like min-max scaling and z-score standardization transform features to a similar scale, improving model convergence and performance. Normalizing nested data ensures that all features contribute equally to the model.
Dimensionality Reduction Techniques
Dimensionality reduction helps in simplifying nested data by reducing the number of features while retaining significant information. Techniques like PCA, t-SNE, and UMAP (Uniform Manifold Approximation and Projection) are commonly used.

Incorporate Domain Knowledge
Incorporating domain knowledge can significantly enhance the handling of nested data by informing feature engineering, model selection, and data preprocessing.
Understand the Structure of the Nested Data
Understanding the structure of nested data involves exploring and visualizing the data to identify key relationships and hierarchies. This understanding guides the feature engineering and model selection process.
Preprocess and Clean the Nested Data
Preprocessing and cleaning nested data involves handling missing values, outliers, and inconsistencies. Effective preprocessing ensures that the data is in a suitable format for modeling.
Feature Engineering for Nested Data
Feature engineering for nested data includes creating new features that capture important relationships and patterns within the data. This step is critical for improving model performance.
Choose the Right Model Architecture
Choosing the right model architecture involves selecting algorithms and model structures that can effectively handle the complexity of nested data. Tree-based algorithms, hierarchical models, and deep learning architectures are common choices.
Cross-Validation and Evaluation
Cross-validation and evaluation are essential for assessing the performance of models trained on nested data. Techniques like k-fold cross-validation provide robust estimates of model performance and help in fine-tuning the model.
from sklearn.model_selection import cross_val_score
# Example cross-validation
model = RandomForestClassifier()
scores = cross_val_score(model, df[['feature1', 'feature2']], df['nested_feature'], cv=5)
print(f'Cross-validation scores: {scores}')
Optimizing nested data in machine learning models requires a combination of effective feature engineering, memory optimization, algorithm selection, and parallel processing techniques. By incorporating domain knowledge and using appropriate tools and libraries, practitioners can handle nested data efficiently and improve model performance.
If you want to read more articles similar to Optimizing Nested Data in Machine Learning Models, you can visit the Artificial Intelligence category.
You Must Read