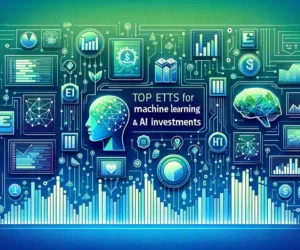
Optimizing Performance: AI Feedback and Reinforcement Learning
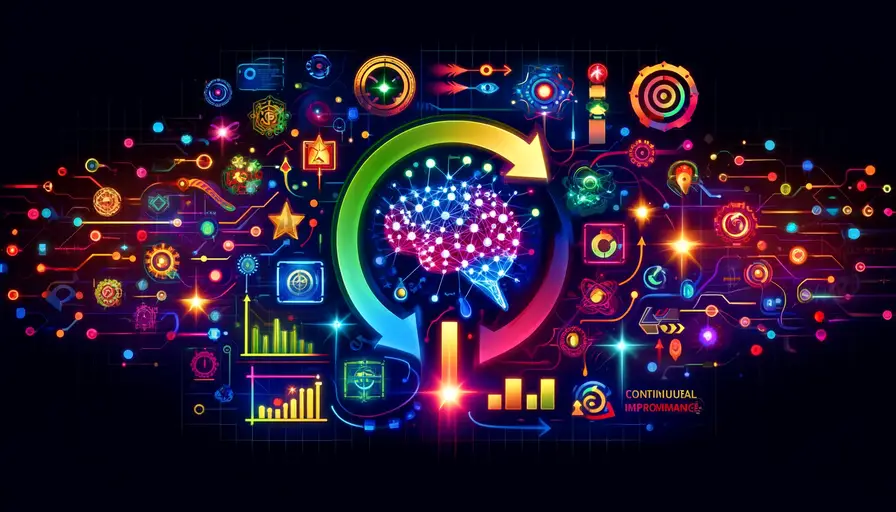
Artificial Intelligence (AI) and Reinforcement Learning (RL) are revolutionizing the way systems optimize performance and make decisions. By leveraging feedback mechanisms and iterative learning, AI systems can enhance their capabilities, leading to improved outcomes in various domains. This article delves into the concepts of AI feedback and reinforcement learning, exploring their significance, applications, and strategies for implementation.
Foundations of AI Feedback
Understanding AI Feedback Mechanisms
AI feedback mechanisms are essential for refining and improving AI models. Feedback involves using information from previous actions or outputs to make adjustments and enhance future performance. This iterative process is fundamental to machine learning, allowing models to learn from their mistakes and successes.
In supervised learning, feedback is provided through labeled data, where the model's predictions are compared against the true labels to calculate errors. These errors are then used to update the model parameters through techniques like gradient descent. This continuous adjustment process helps the model improve its accuracy over time.
In reinforcement learning, feedback is provided through rewards or penalties based on the agent's actions. The agent uses this feedback to adjust its policy, striving to maximize cumulative rewards. Feedback in RL can be immediate or delayed, making it a complex yet powerful mechanism for learning optimal strategies.
PCA: An Unsupervised Dimensionality Reduction TechniqueImportance of Feedback in AI Systems
The importance of feedback in AI systems cannot be overstated. Feedback mechanisms enable models to adapt to changing environments, learn from new data, and correct errors. This adaptability is crucial for developing robust and reliable AI systems that can perform well in diverse and dynamic settings.
Feedback helps in identifying and mitigating biases in AI models. By continuously monitoring and evaluating model performance, feedback mechanisms can detect biased outcomes and trigger corrective actions. This ensures that AI systems make fair and equitable decisions, promoting ethical AI practices.
Moreover, feedback is vital for improving user experience in AI applications. For instance, in recommendation systems, user feedback on recommended items helps refine the recommendations, making them more relevant and personalized. This iterative refinement process enhances user satisfaction and engagement.
Examples of AI Feedback Systems
Various AI applications utilize feedback mechanisms to optimize performance. In natural language processing (NLP), language models like GPT-3 use feedback from human reviewers to improve their responses and reduce biases. This feedback loop ensures that the models generate more accurate and contextually appropriate text.
Exploring Non-Machine Learning Approaches to ClusteringIn computer vision, feedback mechanisms are used in object detection and recognition systems. For example, autonomous vehicles rely on feedback from sensors and cameras to identify and respond to obstacles and road conditions. This continuous feedback loop is crucial for ensuring safe and reliable navigation.
Here’s an example of a simple feedback loop in a machine learning model using Scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Sample data
data = {'feature1': [1, 2, 3, 4, 5],
'feature2': [5, 6, 7, 8, 9],
'target': [1, 2, 3, 4, 5]}
df = pd.DataFrame(data)
# Features and target variable
X = df[['feature1', 'feature2']]
y = df['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict on test data
y_pred = model.predict(X_test)
# Calculate and print mean squared error
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
# Feedback loop: Adjust model if error is high
if mse > 1.0:
print("Adjusting model...")
# Example adjustment: retrain with different parameters or data preprocessing
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f'New Mean Squared Error: {mse}')
Principles of Reinforcement Learning
Basics of Reinforcement Learning
Reinforcement learning (RL) is a type of machine learning where an agent learns to make decisions by interacting with an environment. The agent takes actions to maximize cumulative rewards based on feedback from the environment. RL is inspired by behavioral psychology, where learning occurs through trial and error and reinforcement of positive outcomes.
In RL, the agent, environment, states, actions, and rewards are the key components. The agent observes the current state of the environment, takes an action, and receives a reward or penalty based on the action's outcome. This process continues over multiple iterations, with the agent learning to choose actions that maximize long-term rewards.
Decision Trees in Machine LearningKey Concepts in Reinforcement Learning
Several key concepts underpin reinforcement learning, including policies, value functions, and Q-learning. Policies define the agent's strategy for selecting actions based on the current state. Policies can be deterministic, where a specific action is chosen, or stochastic, where actions are chosen probabilistically.
Value functions estimate the expected return (cumulative reward) of states or state-action pairs. The value function helps the agent evaluate the desirability of different states and actions. The two main types of value functions are the state-value function (V) and the action-value function (Q).
Q-learning is a popular RL algorithm that learns the optimal action-value function (Q). The Q function estimates the expected return of taking a specific action in a given state and following the optimal policy thereafter. Q-learning updates the Q values iteratively based on the agent's experiences, leading to the optimal policy.
Implementing Reinforcement Learning Algorithms
Implementing RL algorithms involves defining the environment, states, actions, rewards, and the learning process. Various libraries and tools, such as OpenAI Gym and Stable Baselines, provide frameworks for developing and testing RL algorithms.
Strategies to Improve Accuracy in ML Classification: Minimizing ErrorsHere’s an example of implementing Q-learning for a simple gridworld environment using OpenAI Gym:
import numpy as np
import gym
# Create a simple gridworld environment
env = gym.make('FrozenLake-v0')
# Initialize Q-table with zeros
Q = np.zeros((env.observation_space.n, env.action_space.n))
# Set hyperparameters
alpha = 0.1 # Learning rate
gamma = 0.99 # Discount factor
epsilon = 0.1 # Exploration rate
# Train the agent
num_episodes = 1000
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
# Choose action using epsilon-greedy policy
if np.random.uniform(0, 1) < epsilon:
action = env.action_space.sample()
else:
action = np.argmax(Q[state, :])
# Take action and observe the result
next_state, reward, done, _ = env.step(action)
# Update Q-table
Q[state, action] = Q[state, action] + alpha * (reward + gamma * np.max(Q[next_state, :]) - Q[state, action])
state = next_state
# Print the learned Q-table
print(Q)
Applications of Reinforcement Learning
Reinforcement Learning in Robotics
Reinforcement learning in robotics has led to significant advancements in autonomous systems and robotic control. RL enables robots to learn complex tasks through interaction with their environment, improving their ability to adapt to new situations and perform tasks autonomously.
For example, RL is used in robotic manipulation, where robots learn to grasp and manipulate objects with precision. By receiving feedback from sensors and cameras, robots adjust their actions to achieve desired outcomes, such as assembling components or sorting items.
RL is also applied in robotic navigation, enabling robots to explore and navigate unknown environments. Autonomous vehicles use RL to learn safe and efficient driving strategies, considering various factors such as traffic conditions, obstacles, and road rules. This enhances the reliability and safety of autonomous systems.
Logistic Regression for Categorical Variables in Machine LearningReinforcement Learning in Gaming
Reinforcement learning in gaming has demonstrated the potential of AI to achieve superhuman performance in complex games. RL agents have been trained to play and excel in games such as chess, Go, and video games, showcasing the ability to learn and adapt to diverse gaming environments.
One notable example is AlphaGo, developed by DeepMind, which used RL to defeat world champions in the game of Go. AlphaGo combined deep neural networks with RL techniques to learn optimal strategies and make decisions that surpassed human capabilities.
In video games, RL agents are trained to navigate and complete game levels by maximizing rewards, such as points or in-game achievements. This involves learning strategies to overcome challenges, defeat opponents, and achieve objectives. RL in gaming not only demonstrates AI's capabilities but also provides a platform for testing and refining RL algorithms.
Reinforcement Learning in Finance
Reinforcement learning in finance is transforming the way financial institutions manage investments, trading, and risk. RL algorithms are used to optimize trading strategies, manage portfolios, and develop automated trading systems that adapt to market conditions.
Unveiling Decision Tree-based Ensemble MethodsIn trading, RL agents learn to make buy, sell, or hold decisions based on historical and real-time market data. By maximizing cumulative returns and minimizing risks, RL agents enhance the efficiency and profitability of trading activities. These algorithms can adapt to changing market trends and adjust strategies accordingly.
RL is also used in portfolio management, where agents optimize asset allocation to achieve specific investment goals. By considering factors such as risk tolerance, market volatility, and investment horizon, RL agents develop strategies that balance returns and risks, enhancing long-term portfolio performance.
Here’s an example of using RL for portfolio management with OpenAI Gym:
import gym
import numpy as np
# Create a custom trading environment
class TradingEnv(gym.Env):
def __init__(self, data):
self.data = data
self.current_step = 0
self.action_space = gym.spaces.Discrete(3) # Buy, Hold, Sell
self.observation_space = gym.spaces.Box(low=0, high=1, shape=(len(data),), dtype=np.float32)
self.reset()
def reset(self):
self.current_step = 0
self.state = self.data[self.current_step]
return self.state
def step(self, action):
self.current_step += 1
if self.current_step >= len(self.data):
done = True
reward = 0
else:
done = False
reward = self.data[self.current_step] - self.data[self.current_step - 1]
if action == 0: # Buy
reward *= 1.1
elif action == 2: # Sell
reward *= 0.9
self.state = self.data[self.current_step]
return self.state, reward, done, {}
# Sample market data
data = np.random.rand(100)
# Create and train the RL agent
env = TradingEnv(data)
Q = np.zeros((env.observation_space.shape[0], env.action_space.n))
alpha = 0.1 # Learning rate
gamma = 0.99 # Discount factor
epsilon = 0.1 # Exploration rate
for episode in range(1000):
state = env.reset()
done = False
while not done:
if np.random.uniform(0, 1) < epsilon:
action = env.action_space.sample()
else:
action = np.argmax(Q[state, :])
next_state, reward, done, _ = env.step(action)
Q[state, action] = Q[state, action] + alpha * (reward + gamma * np.max(Q[next_state, :]) - Q[state, action])
state = next_state
# Print the learned Q-table
print(Q)
Strategies for Enhancing RL Performance
Reward Engineering
Reward engineering is a critical aspect of reinforcement learning that involves designing appropriate reward functions to guide the agent's learning process. The reward function defines the feedback the agent receives for its actions, directly influencing the learning outcome and performance.
A well-designed reward function aligns the agent's objectives with the desired outcomes, encouraging behaviors that lead to optimal performance. For example, in a robotic arm task, rewards can be assigned for successfully grasping objects, while penalties can be given for collisions or failures.
Reward engineering requires a deep understanding of the problem domain and careful consideration of potential trade-offs. Overly simplistic rewards may not capture the complexity of the task, while overly complex rewards can make learning difficult. Iterative refinement and experimentation are often necessary to achieve the best results.
Exploration vs. Exploitation
Exploration vs. exploitation is a fundamental dilemma in reinforcement learning, where the agent must balance exploring new actions to discover better strategies and exploiting known actions to maximize rewards. Effective strategies for balancing exploration and exploitation are essential for optimal learning.
Exploration involves trying out new actions and states, which helps the agent discover potentially better strategies and improve its understanding of the environment. Exploitation involves leveraging the current knowledge to make decisions that maximize immediate rewards.
Common techniques for balancing exploration and exploitation include epsilon-greedy, where the agent chooses a random action with probability epsilon and the best-known action with probability 1-epsilon, and softmax exploration, where actions are chosen probabilistically based on their estimated values. Adaptive methods, such as Upper Confidence Bound (UCB), dynamically adjust the balance based on the agent's confidence in its knowledge.
Leveraging Transfer Learning
Transfer learning in reinforcement learning involves using knowledge gained from one task to improve learning performance in a related task. By leveraging pre-trained models or policies, transfer learning can accelerate learning and enhance performance in new environments.
Transfer learning is particularly useful when the new task shares similarities with previously learned tasks, such as similar state or action spaces. This allows the agent to build on existing knowledge and avoid starting from scratch, reducing the time and computational resources required for learning.
One approach to transfer learning is to initialize the agent's policy or value function with the parameters of a pre-trained model. Another approach is to use hierarchical RL, where high-level policies guide low-level policies based on the agent's experience in related tasks.
Here’s an example of using transfer learning in RL with Stable Baselines:
import gym
from stable_baselines3 import PPO
# Load a pre-trained model
pretrained_model = PPO.load("pretrained_model.zip")
# Create a new environment
env = gym.make('CartPole-v1')
# Fine-tune the pre-trained model on the new environment
pretrained_model.set_env(env)
pretrained_model.learn(total_timesteps=10000)
# Save the fine-tuned model
pretrained_model.save("fine_tuned_model.zip")
Ethical Considerations in RL
Ensuring Fairness and Avoiding Bias
Ensuring fairness and avoiding bias in reinforcement learning is crucial to developing ethical and equitable AI systems. Bias can arise from various sources, including biased training data, reward structures, and environment interactions. Addressing these biases is essential for promoting fairness and inclusivity.
One approach to ensuring fairness is to incorporate fairness constraints into the reward function, ensuring that the agent's actions do not disproportionately benefit or harm specific groups. Additionally, diverse and representative training data can help mitigate biases by providing a comprehensive view of the environment.
Regular monitoring and evaluation of the agent's performance across different demographic groups can help identify and address biases. Transparency in the design and deployment of RL systems is also important, enabling stakeholders to understand and mitigate potential biases.
Addressing Safety Concerns
Addressing safety concerns is critical for deploying reinforcement learning systems in real-world applications. Ensuring that RL agents operate safely and do not cause harm to humans, property, or the environment is paramount.
Safety concerns can be addressed through various techniques, including safe exploration, where the agent is constrained to explore only safe actions and states, and risk-sensitive RL, where the agent considers the potential risks and uncertainties associated with its actions.
Formal verification methods can also be used to prove the safety properties of RL systems, ensuring that they operate within predefined safety boundaries. Additionally, human oversight and intervention mechanisms can enhance the safety and reliability of RL systems.
Promoting Transparency and Accountability
Promoting transparency and accountability in reinforcement learning involves providing clear and understandable explanations of the agent's behavior and decision-making process. This helps build trust and ensures that stakeholders can hold the developers and operators of RL systems accountable.
Explainable AI (XAI) techniques can be used to provide insights into the agent's decisions, highlighting the factors that influenced specific actions. This transparency is particularly important in high-stakes applications, such as healthcare and finance, where the consequences of decisions can be significant.
Accountability can be promoted by establishing clear guidelines and standards for the development and deployment of RL systems. Regular audits and assessments can ensure compliance with ethical principles and regulatory requirements, fostering responsible AI practices.
Future Directions in RL and AI Feedback
Advancements in RL Algorithms
Advancements in RL algorithms are driving the next generation of intelligent systems, with researchers continuously developing new techniques to enhance learning efficiency, scalability, and robustness. Innovations such as deep reinforcement learning, meta-learning, and multi-agent RL are expanding the capabilities and applications of RL.
Deep reinforcement learning combines deep neural networks with RL techniques, enabling agents to learn complex tasks with high-dimensional state and action spaces. Meta-learning focuses on developing agents that can learn to learn, adapting quickly to new tasks with minimal data.
Multi-agent RL involves multiple agents learning and interacting within a shared environment, enabling collaborative and competitive behaviors. This approach is particularly useful in applications such as autonomous driving, where multiple vehicles must coordinate their actions for safe and efficient navigation.
Integrating RL with Other AI Technologies
Integrating RL with other AI technologies is opening new possibilities for developing intelligent systems that leverage the strengths of different approaches. Combining RL with supervised learning, unsupervised learning, and natural language processing can enhance the capabilities and versatility of AI systems.
For instance, integrating RL with supervised learning can improve the efficiency of learning by using labeled data to guide the agent's exploration. Combining RL with unsupervised learning can enable the agent to discover useful representations and patterns in the environment, enhancing its decision-making capabilities.
Natural language processing can be integrated with RL to enable agents to understand and generate human language, facilitating more natural and intuitive interactions. This integration is particularly valuable in applications such as virtual assistants and customer service, where effective communication is essential.
Expanding RL Applications
Expanding RL applications is a key focus for researchers and practitioners, with RL being applied to a growing range of domains and industries. From healthcare and finance to robotics and gaming, RL is transforming the way systems optimize performance and make decisions.
In healthcare, RL is used to develop personalized treatment plans, optimize clinical workflows, and manage chronic conditions. In finance, RL enhances trading strategies, portfolio management, and risk assessment. In robotics, RL improves robotic control, navigation, and manipulation.
Emerging applications of RL include smart grid management, where RL optimizes energy distribution and consumption, and climate modeling, where RL enhances the accuracy and reliability of climate predictions. As RL continues to evolve, its impact on various fields will only grow, driving innovation and progress.
If you want to read more articles similar to Optimizing Performance: AI Feedback and Reinforcement Learning, you can visit the Algorithms category.
You Must Read