
Popular Pre-Trained Machine Learning Models
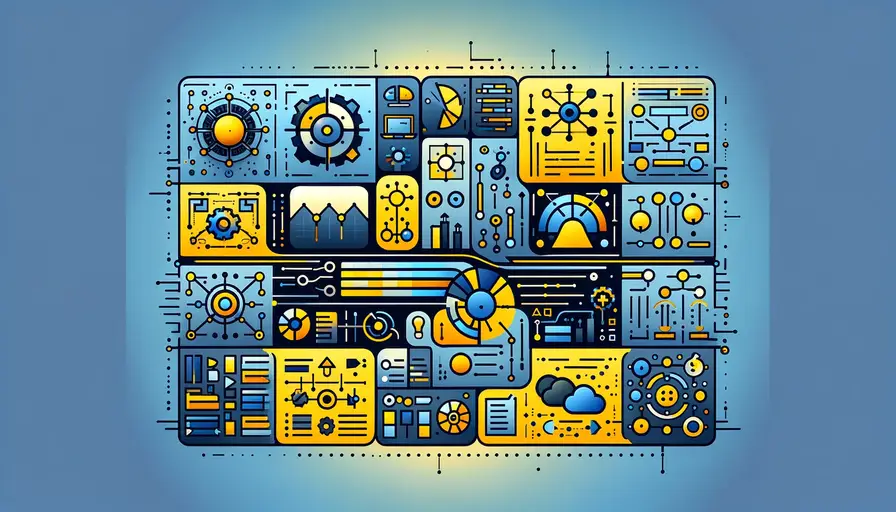
Pre-trained machine learning models have revolutionized the field by providing ready-made solutions that can be integrated into various applications with minimal effort. These models have been trained on extensive datasets, enabling them to perform complex tasks with high accuracy. They are widely used in image classification, object detection, text generation, sentiment analysis, and more.
Content
Image Classification
Image classification involves assigning a label to an image based on its content. Pre-trained models like VGG16, ResNet, and Inception have set benchmarks in this domain. These models can classify images into thousands of categories, making them ideal for applications in healthcare, security, and automated tagging systems.
VGG16 and ResNet are particularly popular due to their robust architecture and high performance on standard image classification benchmarks. VGG16 uses a deep convolutional network with small filters, while ResNet employs residual learning to address the vanishing gradient problem, allowing for very deep networks.
from tensorflow.keras.applications.vgg16 import VGG16
from tensorflow.keras.applications.vgg16 import preprocess_input, decode_predictions
from tensorflow.keras.preprocessing import image
import numpy as np
# Load pre-trained model
model = VGG16(weights='imagenet')
# Load and preprocess image
img_path = 'elephant.jpg'
img = image.load_img(img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
# Predict image classification
preds = model.predict(x)
print('Predicted:', decode_predictions(preds, top=3)[0])
Object Detection
Object detection identifies and localizes objects within an image. Models like YOLO (You Only Look Once) and Faster R-CNN are pre-trained to detect various objects and provide bounding boxes around them. These models are used in surveillance systems, autonomous vehicles, and retail analytics.
Is Machine Learning Necessary Before Deep Learning?YOLO is known for its speed and accuracy, making it suitable for real-time applications. Faster R-CNN provides high detection accuracy by combining region proposal networks with CNNs, making it effective for applications requiring precise object localization.
import cv2
import numpy as np
# Load YOLO model
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# Load image
img = cv2.imread("image.jpg")
height, width, channels = img.shape
# Prepare the image for YOLO
blob = cv2.dnn.blobFromImage(img, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# Process YOLO output
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# Apply Non-Max Suppression
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
for i in indices:
i = i[0]
box = boxes[i]
x, y, w, h = box[0], box[1], box[2], box[3]
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
text = str(class_ids[i]) + " " + str(round(confidences[i], 2))
cv2.putText(img, text, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Text Generation
Text generation uses models like GPT-3 (Generative Pre-trained Transformer 3) to create human-like text. These models can generate essays, articles, and even code based on a given prompt. They are used in content creation, automated reporting, and conversational agents.
GPT-3 is one of the most advanced models for text generation, capable of producing coherent and contextually relevant text. It leverages a massive amount of training data and a transformer architecture to generate high-quality text.
import openai
# Initialize GPT-3 API
openai.api_key = 'your-api-key'
# Generate text
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Once upon a time, in a land far, far away",
max_tokens=50
)
print(response.choices[0].text.strip())
Sentiment Analysis
Sentiment analysis involves determining the sentiment expressed in a piece of text. Pre-trained models like BERT (Bidirectional Encoder Representations from Transformers) are widely used for this purpose. These models can classify text as positive, negative, or neutral, making them useful for customer feedback analysis, social media monitoring, and market research.
The Role of Clustering in Machine LearningBERT excels at sentiment analysis due to its ability to understand context and disambiguate meanings. It uses a transformer architecture to capture the relationships between words in a sentence, providing accurate sentiment classification.
from transformers import pipeline
# Load pre-trained sentiment analysis model
classifier = pipeline('sentiment-analysis')
# Analyze sentiment
result = classifier("I love using pre-trained machine learning models!")
print(result)
Benefits of Using Pre-trained Machine Learning Models
Using pre-trained machine learning models offers several benefits, including saving time and computational resources. These models have already been trained on large datasets, allowing practitioners to leverage their capabilities without the need for extensive training.
Pre-trained models provide high accuracy and reliability, as they have been fine-tuned and validated on diverse datasets. This makes them suitable for a wide range of applications, from image recognition to natural language processing.
Specific Tasks
VGG16
VGG16 is a convolutional neural network known for its simplicity and effectiveness in image classification tasks. It has 16 layers and uses small convolution filters, making it suitable for applications requiring high accuracy and relatively low computational resources.
Unleashing Machine Learning AI: Explore Cutting-Edge ServicesResNet
ResNet (Residual Network) introduced residual learning to address the vanishing gradient problem, enabling the training of very deep networks. It is widely used for image classification and object detection due to its robustness and high performance.
BERT
BERT (Bidirectional Encoder Representations from Transformers) is a transformer-based model designed for NLP tasks. It captures context from both directions in a sentence, making it highly effective for tasks like sentiment analysis, question answering, and text classification.
GPT-3
GPT-3 is a state-of-the-art language model capable of generating human-like text. It is used for text generation, translation, and even coding, providing contextually relevant and coherent outputs based on input prompts.
Inception
Inception (GoogLeNet) is known for its inception modules, which allow the network to learn at multiple scales. It is used for image classification and object detection, offering high accuracy and efficiency.
Is Cheap Machine Learning AI Effective for Small Businesses?Integrated Into Existing Machine Learning Pipelines
Integrating pre-trained models into existing machine learning pipelines can significantly enhance performance and efficiency. This process involves selecting the appropriate model, loading it, fine-tuning if necessary, and integrating it into the application.
Choose the Appropriate Pre-trained Model
Choosing the appropriate pre-trained model depends on the specific task and the requirements of the application. Factors to consider include model accuracy, computational efficiency, and the nature of the input data.
Load the Pre-trained Model
Loading the pre-trained model involves importing it from a library or framework. Most pre-trained models are available in popular libraries like TensorFlow, PyTorch, and Hugging Face Transformers, making it easy to incorporate them into projects.
from transformers import BertTokenizer, BertForSequenceClassification
# Load pre-trained BERT model and tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertForSequenceClassification.from_pretrained('bert-base-uncased')
# Tokenize input text
inputs = tokenizer("Pre-trained models are very useful for NLP tasks.", return_tensors='pt')
# Predict
outputs = model(**inputs)
print(outputs)
Fine-tune the Pre-trained Model (Optional)
Fine-tuning the pre-trained model can improve its performance on specific tasks. This involves training the model on a smaller, task-specific dataset while retaining the knowledge from the original training. Fine-tuning adjusts the model's weights to better suit the new data.
Understanding Long Short Term Memory (LSTM) in Machine Learningfrom transformers import Trainer, TrainingArguments
# Define training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
)
# Define
Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=eval_dataset
)
# Train the model
trainer.train()
Integrate the Pre-trained Model Into Your Pipeline or Application
Integrating the pre-trained model into your pipeline or application involves embedding the model within your existing infrastructure. This could mean incorporating the model into a web service, deploying it on edge devices, or using it within data processing workflows.
By integrating pre-trained models, businesses can leverage advanced machine learning capabilities without the need for extensive training and development. This approach accelerates deployment and reduces costs, allowing for rapid innovation and improved application performance.
Pre-trained machine learning models offer powerful tools for various tasks, including image classification, object detection, text generation, and sentiment analysis. By leveraging these models, practitioners can achieve high accuracy and efficiency, integrating advanced capabilities into their existing pipelines and applications. The continued evolution of pre-trained models promises even greater potential for solving complex problems and driving innovation across industries.
Choosing Reinforcement Learning Models: A Comprehensive GuideIf you want to read more articles similar to Popular Pre-Trained Machine Learning Models, you can visit the Artificial Intelligence category.
You Must Read