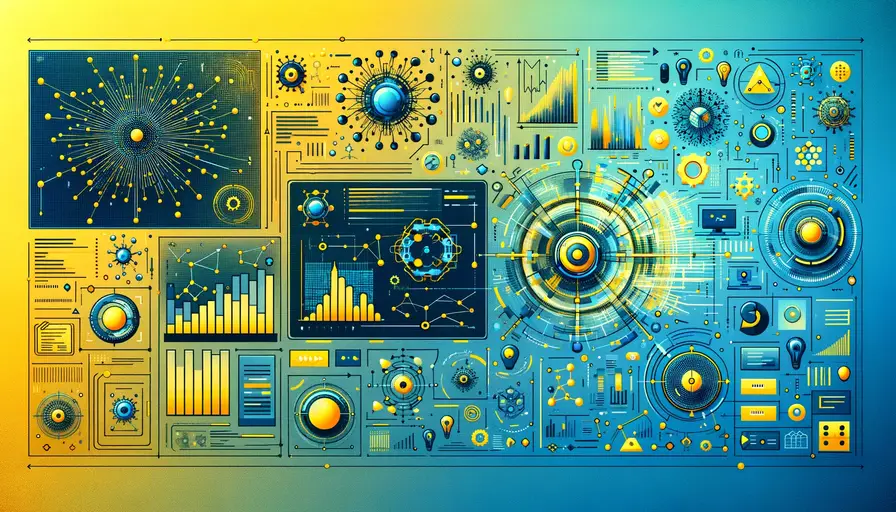
Python for Machine Learning and Data Analysis
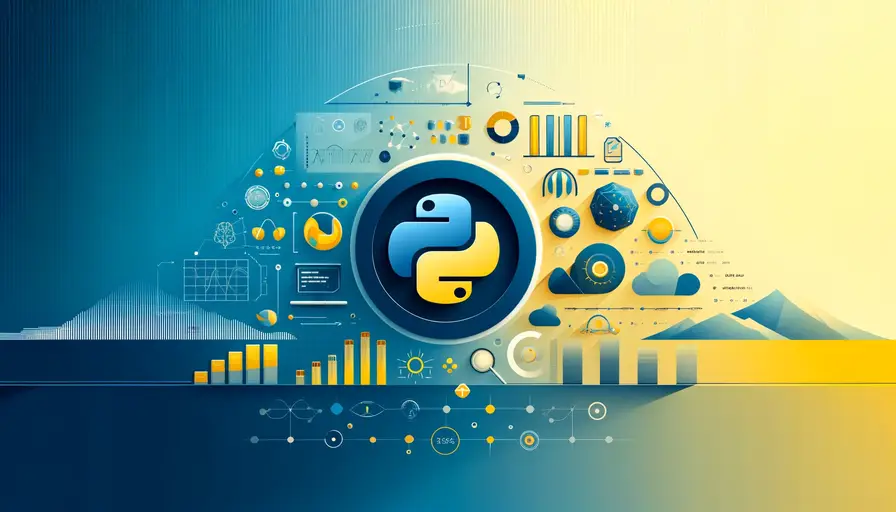
Python has become one of the most popular programming languages for machine learning and data analysis, thanks to its simplicity, readability, extensive libraries, and strong community support. This comprehensive guide delves into the various aspects of Python that make it an ideal choice for these fields, highlighting key libraries, benefits, and practical applications.
- Libraries for Machine Learning
- Libraries for Data Analysis
- Python's Simplicity and Readability Make It Easy
- Python's Extensive Documentation and Large Online Community
- Python's Scalability Allows for Handling Large Datasets and Complex Machine Learning Algorithms
- Python's Integration with Machine Learning Libraries
- Python's Data Analysis Capabilities
- Python's Cross-platform Capabilities
Libraries for Machine Learning
Python's machine learning ecosystem is rich with libraries that cater to different aspects of the machine learning workflow. One of the most prominent libraries is Scikit-learn, which provides simple and efficient tools for data mining and data analysis. Scikit-learn supports various supervised and unsupervised learning algorithms, including regression, classification, clustering, and dimensionality reduction.
TensorFlow and PyTorch are two other significant libraries that have revolutionized the field of deep learning. TensorFlow, developed by Google, is known for its robust performance and flexibility, allowing developers to build and deploy machine learning models on various platforms. PyTorch, developed by Facebook, is favored for its dynamic computation graph and ease of use, making it a preferred choice for research and development.
Keras is a high-level neural networks API, written in Python and capable of running on top of TensorFlow, CNTK, or Theano. It is designed for enabling fast experimentation with deep neural networks and offers a simple and consistent interface for building and training models.

from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train a model
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Evaluate the model
accuracy = clf.score(X_test, y_test)
print(f"Model accuracy: {accuracy}")
Libraries for Data Analysis
Python excels in data analysis through its powerful libraries such as Pandas, NumPy, and SciPy. Pandas provides data structures and functions needed to manipulate structured data seamlessly. It is especially useful for data cleaning, transformation, and analysis, offering a data frame object similar to R's data frames.
NumPy is the fundamental package for numerical computing in Python. It provides support for arrays, matrices, and a large collection of mathematical functions to operate on these data structures. NumPy's efficient handling of numerical operations makes it a cornerstone for scientific computing and data analysis.
SciPy builds on NumPy by adding a collection of algorithms and high-level commands for data manipulation and analysis. It includes modules for optimization, integration, interpolation, eigenvalue problems, and other advanced mathematical computations. Together, Pandas, NumPy, and SciPy form the backbone of Python's data analysis capabilities.
import pandas as pd
import numpy as np
# Create a sample DataFrame
data = {
'A': [1, 2, np.nan, 4],
'B': [5, 6, np.nan, 8],
'C': [9, 10, 11, 12]
}
df = pd.DataFrame(data)
# Fill missing values with the mean
df.fillna(df.mean(), inplace=True)
print(df)
Python's Simplicity and Readability Make It Easy
Python's simplicity and readability are significant advantages that make it an excellent choice for machine learning and data analysis. The language's clean syntax allows developers to write and understand code more efficiently, reducing the learning curve for beginners and increasing productivity for experienced programmers. This simplicity extends to complex machine learning algorithms and data manipulation tasks, making Python accessible for a wide range of users.

Readability is a core philosophy of Python, promoting the use of clear and concise code. This emphasis on readability not only makes the codebase easier to maintain but also facilitates collaboration among teams. When multiple data scientists and machine learning engineers work together, having a common language that is easy to read and understand is invaluable.
The extensive use of Python in academia and industry further underscores its simplicity and readability. Many educational institutions use Python as the primary language for teaching programming, data science, and machine learning. This widespread adoption ensures a steady stream of skilled Python developers entering the workforce, contributing to the language's popularity and growth.
Python's Extensive Documentation and Large Online Community
Python's extensive documentation is a crucial resource for developers at all levels. The official Python documentation provides comprehensive guides, tutorials, and reference materials that cover all aspects of the language. Additionally, most Python libraries, including those for machine learning and data analysis, come with detailed documentation that helps users understand and implement various functionalities.
The large online community surrounding Python is another significant benefit. Platforms like Stack Overflow, GitHub, and various forums host an active community of Python developers who share their knowledge, answer questions, and contribute to open-source projects. This vibrant community support ensures that help is readily available for any issues or questions that may arise, accelerating the development process.

Moreover, numerous online courses and tutorials are available for learning Python and its applications in machine learning and data analysis. Websites like Coursera, edX, and Udacity offer courses by industry experts, while platforms like YouTube provide countless tutorials that cater to different learning styles and levels. This abundance of resources makes it easier for anyone to learn and master Python.
import numpy as np
# Sample array
array = np.array([1, 2, 3, 4, 5])
# Calculate mean and standard deviation
mean = np.mean(array)
std_dev = np.std(array)
print(f"Mean: {mean}, Standard Deviation: {std_dev}")
Python's Scalability Allows for Handling Large Datasets and Complex Machine Learning Algorithms
Scalability is a key strength of Python, allowing it to handle large datasets and complex machine learning algorithms efficiently. Libraries like Dask and Apache Spark provide scalable solutions for parallel computing and distributed data processing, enabling Python to manage big data tasks that would be infeasible with standard computing resources.
Dask extends the capabilities of Pandas and NumPy by enabling parallel computing and handling larger-than-memory datasets. It provides a familiar interface, allowing users to leverage their existing knowledge of Pandas while scaling their operations to multiple cores or distributed clusters. This makes Dask an excellent tool for scaling data analysis workflows in Python.
Apache Spark, through its PySpark API, integrates seamlessly with Python, offering a powerful engine for big data processing. PySpark allows Python developers to write code that can be executed on large clusters, harnessing the full power of distributed computing. This capability is particularly useful for machine learning tasks that involve massive datasets and require significant computational resources.

import dask.dataframe as dd
# Load a large CSV file into a Dask DataFrame
df = dd.read_csv('large_dataset.csv')
# Perform a groupby operation and compute the result
result = df.groupby('category').mean().compute()
print(result)
Python's Integration with Machine Learning Libraries
Python's integration with machine learning libraries is seamless, providing a comprehensive ecosystem for developing and deploying machine learning models. The language's flexibility and compatibility with various libraries allow developers to build complex machine learning pipelines that incorporate data preprocessing, model training, evaluation, and deployment.
Integration with TensorFlow and PyTorch enables Python developers to leverage state-of-the-art deep learning frameworks. TensorFlow's Keras API provides a high-level interface for building and training deep neural networks, while PyTorch's dynamic computation graph allows for more intuitive model development and debugging. These integrations simplify the implementation of advanced machine learning models.
Additionally, Scikit-learn's integration with other data analysis libraries, such as Pandas and NumPy, facilitates the seamless flow of data through different stages of the machine learning pipeline. This integration ensures that data can be easily manipulated, transformed, and fed into machine learning models without the need for cumbersome data conversion processes.
import tensorflow as tf
from tensorflow.keras import layers, models
# Build a simple neural network model
model = models.Sequential()
model.add(layers.Dense(64, activation='relu', input_shape=(10,)))
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(1, activation='linear'))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Print the model summary
model.summary()
Python's Data Analysis Capabilities
Python's data analysis capabilities are unparalleled, making it the go-to language for data scientists and analysts. Libraries like Pandas and NumPy provide powerful tools for data manipulation, transformation, and analysis, enabling users to handle complex datasets with ease. These libraries offer a wide range of functions for tasks such as data cleaning, aggregation, and statistical analysis.

Pandas' DataFrame object is particularly useful for handling structured data. It provides a flexible and intuitive interface for performing operations on tabular data, making it easy to load, filter, group, and analyze datasets. The DataFrame's ability to handle missing data, perform efficient joins, and apply complex transformations makes it an indispensable tool for data analysis in Python.
NumPy's array operations and mathematical functions are essential for numerical computing. NumPy provides efficient storage and computation capabilities, allowing users to perform vectorized operations on large datasets. This efficiency is crucial for machine learning and data analysis tasks that involve large-scale numerical computations.
import pandas as pd
# Load a dataset into a Pandas DataFrame
df = pd.read_csv('data.csv')
# Perform basic data analysis
print(df.describe())
print(df.info())
# Handle missing values
df
.fillna(df.mean(), inplace=True)
Python's Cross-platform Capabilities
Python's cross-platform capabilities ensure that code written on one operating system can be executed on another without modification. This portability is a significant advantage for machine learning and data analysis projects, as it allows developers to collaborate and share code across different environments seamlessly.
The cross-platform nature of Python is supported by its extensive standard library and compatibility with major operating systems, including Windows, macOS, and Linux. This compatibility ensures that Python scripts and applications can be run on various platforms, facilitating the deployment of machine learning models and data analysis tools in diverse environments.

Additionally, Python's support for virtual environments allows developers to create isolated environments for their projects. This isolation ensures that dependencies and libraries specific to a project do not interfere with other projects or the system's global environment. Virtual environments enhance the portability and reproducibility of machine learning and data analysis workflows.
# Create a virtual environment
python -m venv myenv
# Activate the virtual environment
# On Windows
myenv\Scripts\activate
# On macOS/Linux
source myenv/bin/activate
# Install required packages
pip install numpy pandas scikit-learn
Python's versatility, simplicity, and powerful libraries make it an ideal choice for machine learning and data analysis. Its extensive ecosystem supports a wide range of tasks, from data manipulation and analysis to building and deploying complex machine learning models. With its strong community support, comprehensive documentation, and cross-platform capabilities, Python continues to be a leading language in the fields of data science and machine learning.
If you want to read more articles similar to Python for Machine Learning and Data Analysis, you can visit the Education category.
You Must Read