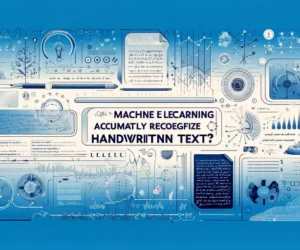
ROC and Precision-Recall Curves in Python
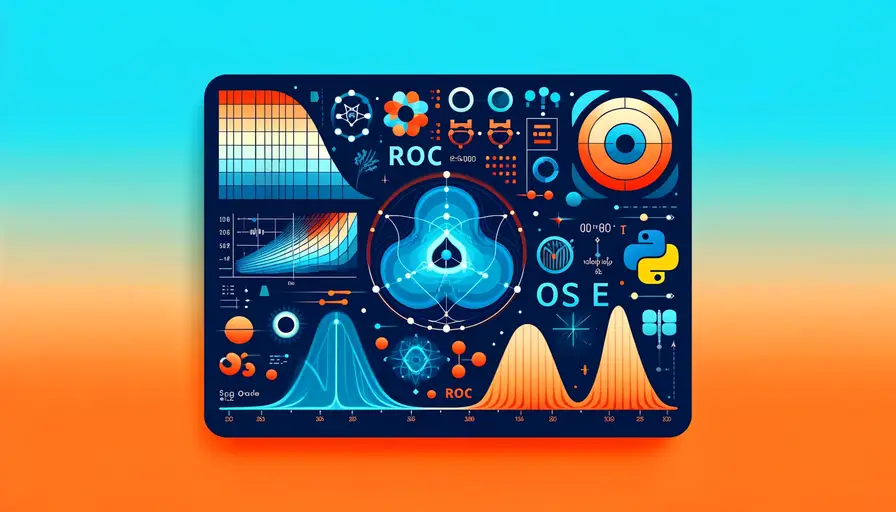
Effective classification is essential for many machine learning applications, from spam detection to medical diagnoses. Evaluating the performance of these models is crucial, and ROC and Precision-Recall curves are two powerful tools for this purpose. This article delves into using these curves in Python, providing insights and practical examples to enhance your classification models.
Understanding ROC and Precision-Recall Curves
Importance of ROC and AUC
The ROC curve (Receiver Operating Characteristic curve) is a graphical representation of a classifier's performance across various threshold settings. It plots the True Positive Rate (TPR) against the False Positive Rate (FPR), helping to visualize the trade-offs between sensitivity and specificity.
The AUC ROC curve (Area Under the ROC Curve) is a single metric summarizing the classifier's performance. A higher AUC indicates a better-performing model. This metric is especially useful when comparing multiple models, as it provides a clear and concise measure of their effectiveness.
In many cases, relying solely on accuracy can be misleading, particularly with imbalanced datasets. The roc function and the auc roc curve help address this issue by focusing on the trade-offs between different types of errors, offering a more nuanced evaluation of the model's performance.
Comparing ML and Statistical Models: Effectiveness and PerformancePrecision-Recall Curves Explained
The Precision-Recall curve is another essential tool for evaluating classification models, especially when dealing with imbalanced data. It plots Precision (the ratio of true positive predictions to the total positive predictions) against Recall (the ratio of true positives to the total actual positives).
Precision-Recall curves are particularly useful when the positive class is rare or when the cost of false positives and false negatives is significantly different. These curves provide insights into the balance between Precision and Recall, allowing you to choose the optimal threshold for your specific application.
Comparing roc and auc with Precision-Recall curves highlights their different strengths. While roc auc curve is useful for overall model performance, Precision-Recall curves excel in highlighting performance for the positive class.
Key Differences and Use Cases
Understanding when to use ROC curves versus Precision-Recall curves is vital. ROC curves are generally preferred when the negative and positive classes are roughly equal in size, as they provide a comprehensive view of the model's performance.
Maximizing Deep Learning Performance: Optimization TechniquesIn contrast, Precision-Recall curves are more informative when dealing with imbalanced datasets. They focus on the performance concerning the positive class, making them ideal for applications like fraud detection or medical screening, where the positive cases are rare but critical.
Choosing the appropriate curve based on your dataset and application ensures a more accurate evaluation of your classification models. Both curves, when used effectively, can significantly enhance your model's performance.
Implementing ROC Curves in Python
Loading and Preprocessing Data
To illustrate the use of ROC and AUC, we'll start with loading and preprocessing data. For this example, we'll use the popular Breast Cancer Wisconsin dataset, available in the sklearn.datasets module.
Here's how to load and preprocess the data:
Error Analysis to Evaluate Machine Learning Modelsfrom sklearn.datasets import load_breast_cancer
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Load the dataset
data = load_breast_cancer()
X = data.data
y = data.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
This code snippet demonstrates the process of loading the dataset, splitting it into training and testing sets, and standardizing the features. Standardization ensures that all features contribute equally to the model, improving its performance.
Training the Model
Next, we'll train a logistic regression model on the training data. Logistic regression is a simple yet powerful classification algorithm that is well-suited for binary classification tasks like this one.
from sklearn.linear_model import LogisticRegression
# Train a logistic regression model
model = LogisticRegression(max_iter=200)
model.fit(X_train, y_train)
This code snippet trains the logistic regression model on the standardized training data. Logistic regression works by fitting a linear decision boundary between the two classes, making it easy to interpret and evaluate.
Plotting the ROC Curve
Once the model is trained, we can plot the ROC curve to evaluate its performance. Scikit-learn provides a convenient function for this purpose:
Interpreting Machine Learning Model Results: A Guidefrom sklearn.metrics import roc_curve, roc_auc_score
import matplotlib.pyplot as plt
# Predict probabilities for the test set
y_probs = model.predict_proba(X_test)[:, 1]
# Compute the ROC curve
fpr, tpr, thresholds = roc_curve(y_test, y_probs)
# Compute the AUC
auc = roc_auc_score(y_test, y_probs)
# Plot the ROC curve
plt.figure()
plt.plot(fpr, tpr, label=f'ROC curve (AUC = {auc:.2f})')
plt.plot([0, 1], [0, 1], 'k--')
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC Curve')
plt.legend(loc='lower right')
plt.show()
This code snippet demonstrates how to plot the ROC curve and calculate the AUC. The roc function in scikit-learn computes the false positive and true positive rates for different thresholds, allowing us to visualize the trade-offs between sensitivity and specificity.
Implementing Precision-Recall Curves in Python
Calculating Precision and Recall
To plot the Precision-Recall curve, we first need to calculate Precision and Recall for different thresholds. Scikit-learn provides functions for this as well:
from sklearn.metrics import precision_recall_curve
# Compute precision and recall
precision, recall, thresholds = precision_recall_curve(y_test, y_probs)
This code snippet calculates Precision and Recall values for different thresholds, which are necessary for plotting the Precision-Recall curve.
Plotting the Precision-Recall Curve
With Precision and Recall values computed, we can now plot the Precision-Recall curve:
Can Machine Learning Classification Be Validated for Accuracy?# Plot the Precision-Recall curve
plt.figure()
plt.plot(recall, precision, label='Precision-Recall curve')
plt.xlabel('Recall')
plt.ylabel('Precision')
plt.title('Precision-Recall Curve')
plt.legend(loc='lower left')
plt.show()
This code snippet plots the Precision-Recall curve, providing insights into the trade-offs between Precision and Recall. This curve is particularly useful for evaluating models on imbalanced datasets.
Comparing Models with Precision-Recall Curves
Precision-Recall curves can also be used to compare the performance of multiple models. By plotting the curves for different models on the same graph, you can easily see which model performs better in terms of Precision and Recall.
# Plot Precision-Recall curves for multiple models
plt.figure()
plt.plot(recall, precision, label='Logistic Regression')
# Add more models here for comparison
plt.xlabel('Recall')
plt.ylabel('Precision')
plt.title('Precision-Recall Curve Comparison')
plt.legend(loc='lower left')
plt.show()
This code snippet provides a template for comparing multiple models using Precision-Recall curves. By evaluating the curves side by side, you can choose the model that best balances Precision and Recall for your specific application.
Advanced Techniques and Considerations
Handling Imbalanced Datasets
When dealing with imbalanced datasets, standard metrics like accuracy can be misleading. ROC and Precision-Recall curves offer a more nuanced evaluation of model performance. Additionally, techniques like SMOTE (Synthetic Minority Over-sampling Technique) can be used to balance the dataset.
Key Evaluation Scenarios for Machine Learning ModelsHere is an example of using SMOTE with scikit-learn:
from imblearn.over_sampling import SMOTE
# Apply SMOTE to balance the dataset
smote = SMOTE(random_state=42)
X_resampled, y_resampled = smote.fit_resample(X_train, y_train)
This code snippet demonstrates how to apply SMOTE to oversample the minority class, resulting in a more balanced dataset. Balancing the dataset can improve the performance of classification models, particularly when using metrics like roc auc curve and Precision-Recall curves.
Threshold Selection and Optimization
Selecting the optimal threshold for classification is crucial for maximizing model performance. Both ROC and Precision-Recall curves can help identify the best threshold by highlighting the trade-offs between different metrics.
Here is an example of threshold selection using the ROC curve:
# Find the optimal threshold
optimal_idx = np.argmax(tpr - fpr)
optimal_threshold = thresholds[optimal_idx]
print(f'Optimal Threshold: {optimal_threshold}')
This code snippet identifies the optimal threshold based on the ROC curve by maximizing the difference between the true positive rate and false positive rate. Selecting the right threshold can significantly impact the model's performance, making it a critical step in the evaluation process.
Combining Multiple Metrics
Using multiple metrics, such as roc auc curve and Precision-Recall curves, provides a comprehensive evaluation of your model's performance. By considering various aspects of the model, you can make more informed decisions about its effectiveness and areas for improvement.
Here is an example of combining multiple metrics:
from sklearn.metrics import f1_score
# Compute the F1 score
f1 = f1_score(y_test, y_pred)
print(f'F1 Score: {f1}')
This code snippet calculates the F1 score, a metric that combines Precision and Recall into a single value. By using multiple metrics, you can gain a deeper understanding of your model's strengths and weaknesses.
Practical Applications of ROC and Precision-Recall Curves
Fraud Detection
In fraud detection, identifying fraudulent transactions is critical. ROC and Precision-Recall curves help evaluate the performance of fraud detection models, ensuring they effectively distinguish between fraudulent and legitimate transactions.
For example, using Precision-Recall curves can highlight the trade-offs between false positives and false negatives, allowing you to choose a model that minimizes the cost of fraud while maintaining a high level of accuracy.
Medical Diagnoses
In medical diagnoses, accurate classification models can save lives. ROC and Precision-Recall curves provide essential insights into the performance of diagnostic models, helping healthcare professionals make informed decisions.
By evaluating models using these curves, you can ensure that the models are sensitive enough to detect true positives while maintaining a low rate of false positives, improving patient outcomes.
Spam Detection
Spam detection is another practical application where ROC and Precision-Recall curves play a crucial role. These curves help evaluate spam filters, ensuring they effectively identify spam emails while minimizing false positives.
Using roc auc curve and Precision-Recall curves, you can optimize your spam detection models to balance the trade-offs between different types of errors, improving the overall performance of your spam filter.
ROC and Precision-Recall curves are powerful tools for evaluating classification models. By understanding and applying these curves in Python, you can boost your classification models' performance and make more informed decisions. Whether you're working on fraud detection, medical diagnoses, or spam detection, these curves provide invaluable insights into your models' strengths and weaknesses.
If you want to read more articles similar to ROC and Precision-Recall Curves in Python, you can visit the Performance category.
You Must Read