
Setting Up Your First Speech Synthesis Project: A Step-by-Step Guide

Introduction
Speech synthesis is a fascinating field within artificial intelligence that allows machines to generate human-like speech. This technology has gained immense popularity due to advancements in machine learning and natural language processing. From virtual assistants like Siri and Alexa to tools for text-to-speech applications, speech synthesis is transforming how we interact with machines and access information. Understanding the foundational aspects of speech synthesis can empower you to harness this technology, whether for personal projects, educational purposes, or professional development.
In this article, we will guide you through the essential steps needed to set up your very first speech synthesis project. We will explore the tools, libraries, and best practices to ensure you start on firm ground. Although the technical details may seem daunting at first, our step-by-step format will simplify the process, allowing you to grasp the concepts easily and implement them with confidence.
Understanding Speech Synthesis
Speech synthesis, commonly referred to as text-to-speech (TTS), converts written text into spoken words. This process involves several stages, including text normalization, prosody generation, and audio rendering. Each of these stages plays a crucial role in determining the naturalness and clarity of the generated speech. For instance, text normalization deals with converting numbers, abbreviations, and other non-standard text into easily readable formats. Prosody generation involves adding rhythm and inflection to the speech, while audio rendering focuses on producing the final sound output.
Different methods of speech synthesis have evolved over the years. Early approaches used concatenative synthesis, which combines recorded snippets of speech to form sentences. However, this technique lacks the flexibility and expressive potential found in modern parametric synthesis methods, which utilize statistical models to generate speech. The most current trend is the use of deep learning, especially recurrent and convolutional neural networks, which can produce highly realistic voices and mimic various speaking styles.
Designing Interactive Voice Response Systems with AI AlgorithmsBefore diving into implementation, it is essential to consider the intended application of your speech synthesis project. Are you creating an assistive technology for individuals with disabilities? Perhaps you want to develop a chatbot that can converse naturally with users. These considerations will influence your choice of tools and libraries and help you better tailor the speech synthesis experience to meet your users' needs.
Setting Up Your Development Environment
Choosing the Right Tools
The first step in setting up your speech synthesis project is selecting the appropriate tools. The field of speech synthesis is rich with options, depending on your needs and expertise. Python is a popular choice due to its versatility and the wide range of libraries available for TTS. Libraries like gTTS (Google Text-to-Speech), pyttsx3, and Coqui TTS offer excellent starting points, each with its unique advantages.
For beginners, gTTS is particularly user-friendly. It leverages Google’s TTS API, which means you benefit from high-quality speech synthesis without extensive setup. Alternatively, if you prefer to have a local solution that does not rely on the internet, pyttsx3 serves this purpose well and works even while offline. Coqui TTS, on the other hand, offers more advanced capabilities, making it ideal for those interested in experimenting with neural network-based speech synthesis.
Installing Libraries and Dependencies
Once you have chosen your library, the next step is to install the required dependencies. If you’re using Python, you’ll most likely need to set up a virtual environment to manage your project’s dependencies effectively. This can be done using venv
or conda
. Here’s how to set it up:
Create a new virtual environment:
bash
python -m venv my_speech_synthesis_env
Activate the virtual environment:
- On Windows:
bash
my_speech_synthesis_envScriptsactivate
- On macOS or Linux:
bash
source my_speech_synthesis_env/bin/activate
- On Windows:
Install the chosen TTS library using pip:
bash
pip install gTTS
This installation process will ensure that you have all necessary packages required for your TTS project. Remember that you may need to install additional dependencies later based on the complexity of your project.
The Impact of AI on the Future of Speech Synthesis TechnologyUnderstanding Basic Configuration
After installation, it’s crucial to become familiar with the basic configuration of your TTS library. For gTTS, for instance, you need to specify the language and the text you wish to convert into speech. Here’s an example of how to perform a simple synthesis operation:
```python
from gtts import gTTS
import os
text = "Hello! Welcome to your first speech synthesis project."
language = 'en'
speech = gTTS(text=text, lang=language, slow=False)
speech.save("welcome.mp3")
os.system("start welcome.mp3") # This line plays the audio file.
```
In this example, we create a simple script that takes a text string and synthesizes it into an audio file. Exploring the documentation of the chosen library will yield even more functionality, such as adjusting the speed of speech, selecting different voices, and handling multiple languages.
Enhancing Your Speech Synthesis Project
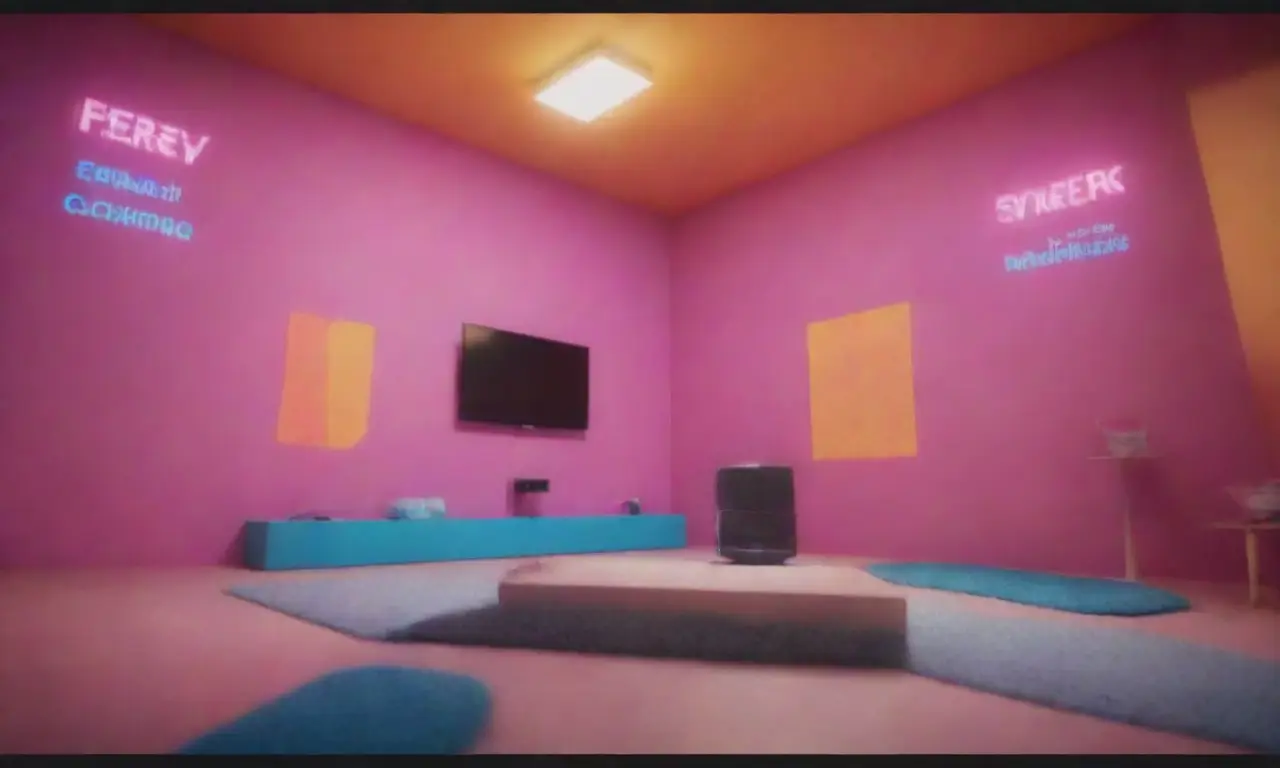
Experimenting with Different Voices
One of the most interesting aspects of modern speech synthesis technology is the ability to experiment with various voices. Many TTS libraries, including Coqui TTS, provide multiple voice models that cater to different styles and accents. You can use different voice parameters to create a more engaging and personalized listening experience. The following is a brief overview of how you can achieve this with Coqui TTS:
Install Coqui TTS:
Speech Synthesis Techniques for Multilingual Applicationsbash
pip install TTS
Choose a voice: After installation, you can download different voice models as specified in their GitHub repository.
Run a synthesis command:
python
from TTS.utils.synthesizer import Synthesizer
synthesizer = Synthesizer()
synthesizer.load_model("path/to/model")
wav = synthesizer.tts("Hello! I am using a different voice model.")
synthesizer.save_wav(wav, "output.wav")
Choosing the right voice can significantly influence user interaction, making the experience more relatable and enjoyable. This is particularly valuable for applications aimed at education or assistance, where a friendly and engaging voice can enhance user engagement and comprehension.
Adding Background Music and Effects
To elevate your speech synthesis project further, consider enhancing it with background music or sound effects. This approach can create more immersive experiences, particularly in applications like games, tutorials, or audiobooks. While many TTS libraries are primarily focused on voice generation, integrating audio libraries like Pydub or pygame can prove beneficial.
Challenges and Solutions in Speech Synthesis Technology DevelopmentUsing Pydub, you could easily blend narrations with music tracks:
```python
from pydub import AudioSegment
from pydub.playback import play
speech = AudioSegment.fromfile("welcome.mp3")
backgroundmusic = AudioSegment.from_file("background.mp3")
finaloutput = speech.overlay(backgroundmusic)
finaloutput.export("finaloutput.mp3", format="mp3")
play(final_output)
```
In the above example, we create a final audio track by overlaying synthesized speech with background music, making your project feel professional and polished.
Building a Frontend Interface
As your project evolves, you may want to consider creating a frontend interface where users can input text and control playback options. Tools like Flask or Django for web applications, and Tkinter for GUI applications, can help you create interactive interfaces. A simple Flask application could look something like this:
```python
from flask import Flask, request, render_template
from gtts import gTTS
import os
app = Flask(name)
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
text = request.form['text']
language = 'en'
speech = gTTS(text=text, lang=language, slow=False)
speech.save("output.mp3")
return rendertemplate('index.html', audiofile='output.mp3')
return render_template('index.html')
if name == 'main':
app.run(debug=True)
```
In this example, we set up a basic web application where users can enter text that they want to be synthesized. The resulting audio file is then saved and can be easily played back through the interface. Building such interfaces makes your application more user-centric and enhances usability.
Conclusion
Undertaking your first speech synthesis project can be both an exciting and educational experience that combines the intricacies of technology with creativity. From understanding the foundational aspects of speech synthesis to setting up your development environment and experimenting with various features, each step offers valuable learning opportunities.
Reflecting on the importance of selecting the right tools and libraries, it becomes clear that these foundational choices significantly impact the overall success of your project. Furthermore, experimenting with different voices, incorporating background music, and building a frontend interface can lead to a more engaging user experience, showcasing the endless possibilities within this field.
As you embark on your journey in speech synthesis, remember that continuous experimentation and learning are key to mastering this complex technology. Stay updated on the latest developments and best practices, as this area is rapidly evolving, and new tools and functionalities are regularly introduced. Whether for fun, educational, or professional projects, the skills you acquire in speech synthesis will undoubtedly serve you well and expand your horizons in the world of artificial intelligence and beyond. Happy synthesizing!
If you want to read more articles similar to Setting Up Your First Speech Synthesis Project: A Step-by-Step Guide, you can visit the Speech Synthesis Applications category.
You Must Read