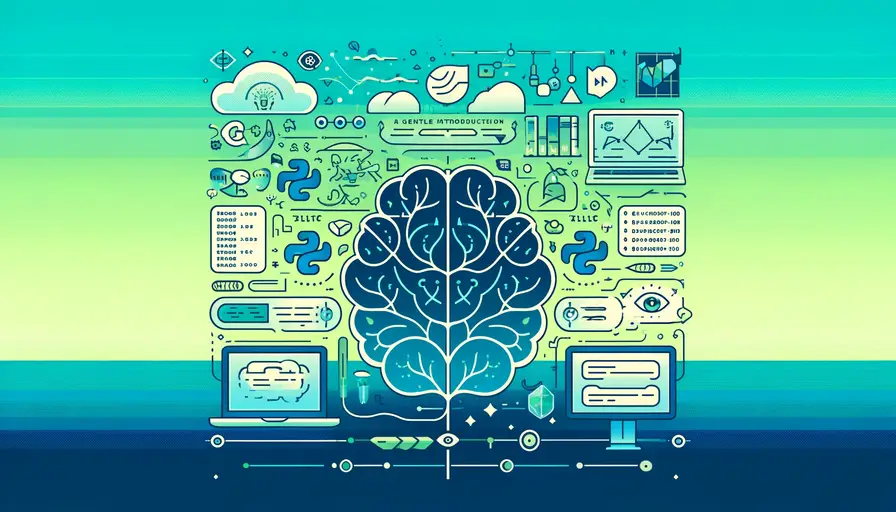
Strategies for Zero-Inflated Data in Machine Learning Algorithms

Understanding Zero-Inflated Data
Characteristics of Zero-Inflated Data
Zero-inflated data is characterized by an excessive number of zero values compared to what standard statistical distributions would predict. This type of data is common in various fields, including finance, healthcare, and environmental studies. In these datasets, zeros can represent different phenomena, such as the absence of an event, a specific condition, or a measurement below a detection limit. Understanding the nature of these zeros is crucial for selecting appropriate analytical methods.
The key challenge with zero-inflated data is that the zeros can skew the results of traditional statistical models, which often assume a normal distribution of the data. This skewness can lead to biased estimates and incorrect inferences. Therefore, it is essential to identify the underlying processes generating the zeros and choose models that can appropriately handle this excess of zero values.
In many cases, zero-inflated data arises from a mixture of two processes: one generating the zeros and another generating the non-zero values. For example, in healthcare, the count of hospital visits might have many zeros representing individuals who did not visit the hospital during a certain period and non-zero counts representing those who did. Identifying and modeling these two processes separately can lead to more accurate and insightful analyses.
Applications with Zero-Inflated Data
Zero-inflated data appears across various applications, making it crucial to understand how to handle it effectively in different contexts. In finance, transaction data often contains numerous zero values, representing periods with no transactions. Accurate modeling of this data is essential for predicting future transactions and understanding customer behavior. Similarly, in marketing, customer purchase data can have many zeros, indicating non-purchase periods, which need careful handling for effective marketing strategies.

In environmental science, zero-inflated data is common in species count studies, where many observations may record zero counts of a species in specific locations or times. Properly modeling this data is crucial for understanding species distribution and making conservation decisions. Additionally, in public health, zero-inflated data can occur in the study of disease incidence, where many individuals may not exhibit symptoms or test positive for a disease, leading to datasets with many zero values.
Understanding the specific application and the reasons behind the zero-inflation in the data can guide the choice of appropriate modeling techniques. These techniques can improve the accuracy of predictions, enhance the interpretability of results, and support better decision-making in various fields.
Example: Visualizing Zero-Inflated Data with Matplotlib
import numpy as np
import matplotlib.pyplot as plt
# Generate zero-inflated data
np.random.seed(42)
data = np.concatenate([np.zeros(500), np.random.poisson(3, 500)])
# Plot the data
plt.figure(figsize=(10, 6))
plt.hist(data, bins=30, color='blue', edgecolor='black')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram of Zero-Inflated Data')
plt.show()
In this example, Matplotlib is used to visualize zero-inflated data by generating a dataset that combines zeros with values from a Poisson distribution. The histogram illustrates the high frequency of zeros compared to other values, highlighting the zero-inflation characteristic of the data.
Strategies for Handling Zero-Inflated Data
Zero-Inflated Models
Zero-inflated models are specifically designed to handle datasets with an excessive number of zeros. These models assume that the data comes from a mixture of two distributions: one generating the zero values and another generating the non-zero values. By modeling these two processes separately, zero-inflated models can provide more accurate estimates and better predictions for zero-inflated datasets.

One common zero-inflated model is the Zero-Inflated Poisson (ZIP) model, which combines a Poisson distribution for the count data with a binary distribution for the zeros. This model is suitable for count data where the zeros are more frequent than expected under a standard Poisson distribution. Another widely used model is the Zero-Inflated Negative Binomial (ZINB) model, which extends the ZIP model by allowing for overdispersion in the count data, making it more flexible and robust.
Implementing zero-inflated models can be done using various statistical software and programming languages. For instance, the statsmodels
library in Python provides functionality for fitting zero-inflated models, making it accessible for data scientists and analysts to apply these models to their zero-inflated datasets.
Example: Fitting a Zero-Inflated Poisson Model with Statsmodels
import numpy as np
import statsmodels.api as sm
import statsmodels.formula.api as smf
# Generate zero-inflated data
np.random.seed(42)
counts = np.random.poisson(3, 1000)
zeros = np.zeros(1000)
data = np.where(np.random.rand(1000) < 0.5, zeros, counts)
data = pd.DataFrame({'counts': data, 'x1': np.random.randn(1000), 'x2': np.random.randn(1000)})
# Fit a Zero-Inflated Poisson model
zip_model = smf.poisson("counts ~ x1 + x2", data).fit()
print(zip_model.summary())
In this example, Statsmodels is used to fit a Zero-Inflated Poisson model to a synthetic dataset. The model includes predictors x1
and x2
, and the summary output provides details on the model coefficients and statistical significance, illustrating how zero-inflated models can be applied to real-world data.
Zero-Inflated Regression
Zero-inflated regression models extend the concept of zero-inflated models to regression analysis. These models are useful when the dependent variable is zero-inflated, and the goal is to understand the relationship between the dependent variable and one or more independent variables. Zero-inflated regression models can be applied in various contexts, such as predicting sales, counts of events, or other quantities that exhibit zero-inflation.

Zero-Inflated Poisson Regression (ZIPR) is a common approach for count data, where the dependent variable follows a Poisson distribution with zero-inflation. This model accounts for the excess zeros by incorporating a binary component that models the probability of a zero outcome. Zero-Inflated Negative Binomial Regression (ZINBR) is another option that extends ZIPR by allowing for overdispersion, making it suitable for count data with high variability.
Implementing zero-inflated regression models requires careful consideration of the underlying data generation processes and appropriate software tools. Python libraries like statsmodels
and scikit-learn
provide functionality for fitting zero-inflated regression models, enabling data scientists to apply these techniques to their datasets.
Example: Zero-Inflated Negative Binomial Regression with Statsmodels
import numpy as np
import pandas as pd
import statsmodels.api as sm
import statsmodels.formula.api as smf
# Generate zero-inflated data
np.random.seed(42)
counts = np.random.negative_binomial(5, 0.5, 1000)
zeros = np.zeros(1000)
data = np.where(np.random.rand(1000) < 0.5, zeros, counts)
data = pd.DataFrame({'counts': data, 'x1': np.random.randn(1000), 'x2': np.random.randn(1000)})
# Fit a Zero-Inflated Negative Binomial model
zinb_model = smf.negativebinomial("counts ~ x1 + x2", data).fit()
print(zinb_model.summary())
In this example, Statsmodels is used to fit a Zero-Inflated Negative Binomial model to a synthetic dataset. The model includes predictors x1
and x2
, and the summary output provides details on the model coefficients and statistical significance, demonstrating how zero-inflated regression models can be applied to zero-inflated datasets.
Practical Applications of Zero-Inflated Models
Finance and Economic Data
Zero-inflated models are highly applicable in finance and economic data, where datasets often contain an excess of zero values. For example, transaction data might have numerous zero values representing periods with no transactions, while non-zero values indicate transaction amounts. Accurate modeling of this data is crucial for predicting future transactions, understanding customer behavior, and developing effective marketing strategies.

In credit risk modeling, zero-inflated data can occur when predicting default events, where many observations may have zero defaults, while others have one or more defaults. Zero-inflated regression models can help identify the factors that contribute to default risk and provide more accurate predictions. Additionally, zero-inflated models can be used to analyze spending patterns, investment returns, and other financial metrics that exhibit zero-inflation.
By leveraging zero-inflated models, financial analysts can gain deeper insights into customer behavior, improve risk assessment, and make more informed decisions. These models can also enhance the accuracy of forecasting and help identify opportunities for growth and optimization in various financial applications.
Healthcare and Medical Research
In healthcare and medical research, zero-inflated data is common in studies involving counts of events, such as hospital visits, medication usage, or disease incidence. Many individuals may not experience the event of interest, resulting in datasets with numerous zero values. Zero-inflated models are essential for accurately analyzing this data and understanding the factors that influence health outcomes.
For instance, in epidemiological studies, zero-inflated models can be used to analyze the incidence of diseases, where many individuals do not contract the disease, leading to zero counts. These models can help identify risk factors, assess the effectiveness of interventions, and predict future disease outbreaks. Similarly, in clinical trials, zero-inflated models can be used to analyze patient responses to treatments, where some patients may not experience any

If you want to read more articles similar to Strategies for Zero-Inflated Data in Machine Learning Algorithms, you can visit the Algorithms category.
You Must Read