
Support Vector Machines for Machine Learning
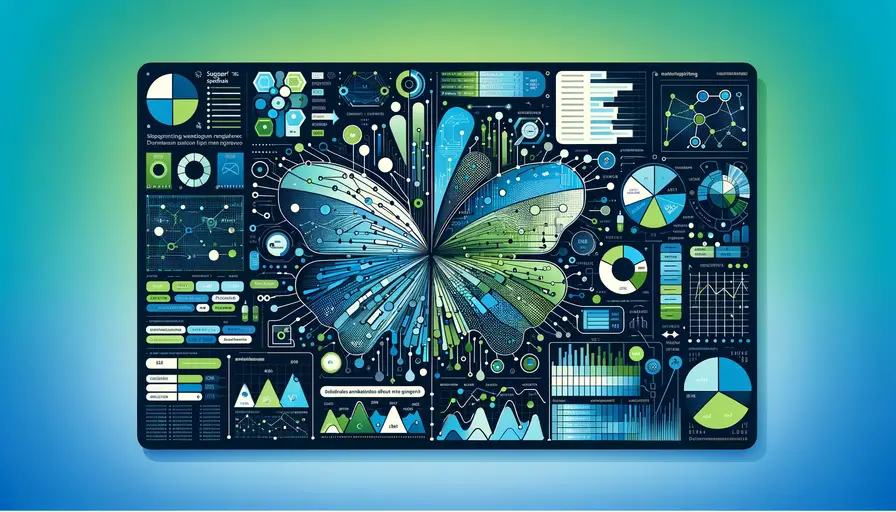
Support Vector Machines (SVMs) are a powerful set of supervised learning algorithms used for classification, regression, and outlier detection. Known for their effectiveness in high-dimensional spaces and versatility, SVMs have become a popular choice for machine learning practitioners. This article explores the fundamentals, implementation, and advanced techniques of Support Vector Machines, providing practical examples and insights to help you harness their potential.
Content
Basics of Support Vector Machines
Concept and Working Principle
Support Vector Machines (SVM) are based on the concept of finding a hyperplane that best separates the data points of different classes. This hyperplane is chosen to maximize the margin between the closest points of the classes, known as support vectors. The goal is to find the hyperplane that offers the largest margin, ensuring better generalization to unseen data.
The SVM algorithm works by transforming the original data into a higher-dimensional space where it becomes easier to find a hyperplane that separates the classes. This transformation is achieved through the use of kernel functions, which map the input features into a higher-dimensional space. Common kernel functions include linear, polynomial, and radial basis function (RBF) kernels.
By focusing on the data points closest to the decision boundary, Support Vector Machines (SVM) are less sensitive to outliers and noise. This characteristic makes them particularly useful for classification tasks where the data may not be perfectly separable in its original form.
Particle Swarm OptimizationAdvantages and Applications
One of the main advantages of Support Vector Machines (SVM) is their ability to handle high-dimensional data effectively. This makes them suitable for applications such as text classification, image recognition, and bioinformatics, where the feature space can be large and complex. Additionally, SVMs are versatile and can be adapted for various tasks, including classification, regression, and outlier detection.
Support Vector Machines (SVM) are also known for their robust performance and ability to find global optima. Unlike some other algorithms, SVMs are less likely to get stuck in local optima, ensuring a more reliable and consistent performance. Their regularization parameter, which controls the trade-off between maximizing the margin and minimizing the classification error, allows for fine-tuning and improving the model's performance.
In practical applications, Support Vector Machines (SVM) have been used in numerous fields, including finance, healthcare, and marketing. For instance, they are employed in stock market prediction, disease diagnosis, and customer segmentation. Their ability to handle complex and high-dimensional data makes them a valuable tool in a data scientist's toolkit.
Key Terminology and Concepts
Several key terms and concepts are essential for understanding Support Vector Machines (SVM). The support vectors are the data points closest to the hyperplane and are critical in defining the decision boundary. The margin refers to the distance between the hyperplane and the nearest support vectors. A larger margin indicates a better separation of the classes.
What is Long Short-Term Memory?The kernel trick is a fundamental concept in SVM. It involves using a kernel function to transform the input data into a higher-dimensional space, making it easier to find a hyperplane that separates the classes. Common kernel functions include linear, polynomial, and radial basis function (RBF) kernels. Each kernel function has its strengths and is chosen based on the nature of the data and the problem at hand.
The regularization parameter (C) controls the trade-off between maximizing the margin and minimizing the classification error. A smaller value of C creates a wider margin but allows for some misclassifications, while a larger value of C creates a narrower margin with fewer misclassifications. Fine-tuning this parameter is crucial for achieving optimal model performance.
Implementing Support Vector Machines in Python
Loading and Preprocessing Data
To implement Support Vector Machines (SVM) in Python, we can use the scikit-learn library, which provides a robust and easy-to-use implementation of SVM. We'll start by loading and preprocessing the data. For this example, we'll use the famous Iris dataset.
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Load the dataset
data = load_iris()
X = data.data
y = data.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
This code snippet demonstrates the process of loading the dataset, splitting it into training and testing sets, and standardizing the features. Standardization ensures that all features contribute equally to the model, improving its performance.
Choosing the Right ML Classification Algorithm: Decision TreeTraining the SVM Model
Next, we'll train a Support Vector Machine (SVM) model on the training data. For this example, we'll use a linear kernel. The linear kernel is suitable for data that is linearly separable, providing a straightforward decision boundary.
from sklearn.svm import SVC
# Train a linear SVM model
model = SVC(kernel='linear', C=1.0)
model.fit(X_train, y_train)
This code snippet trains a Support Vector Machine (SVM) model with a linear kernel on the standardized training data. The C
parameter is set to 1.0, balancing the trade-off between margin width and classification error.
Evaluating the SVM Model
Once the model is trained, we can evaluate its performance on the testing data. We'll use accuracy as the evaluation metric, but other metrics such as precision, recall, and F1-score can also be used for a more comprehensive evaluation.
from sklearn.metrics import accuracy_score
# Make predictions on the testing data
y_pred = model.predict(X_test)
# Evaluate the model's accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
This code snippet evaluates the Support Vector Machine (SVM) model's accuracy on the testing data, providing a measure of its performance. Accuracy is a useful metric, but it is important to consider other metrics depending on the specific application and data characteristics.
Normalization Techniques for Deep Learning Regression ModelsAdvanced Techniques with Support Vector Machines
Kernel Trick and Non-Linear SVM
One of the powerful features of Support Vector Machines (SVM) is the ability to handle non-linear data through the use of kernel functions. The kernel trick allows SVM to transform the input features into a higher-dimensional space, where it becomes easier to find a hyperplane that separates the classes.
For instance, the Radial Basis Function (RBF) kernel is commonly used for non-linear data. It maps the input features into an infinite-dimensional space, enabling the model to capture complex relationships between the data points.
# Train an SVM model with RBF kernel
rbf_model = SVC(kernel='rbf', C=1.0, gamma='scale')
rbf_model.fit(X_train, y_train)
# Evaluate the RBF SVM model
rbf_y_pred = rbf_model.predict(X_test)
rbf_accuracy = accuracy_score(y_test, rbf_y_pred)
print(f"RBF SVM Accuracy: {rbf_accuracy}")
This code snippet demonstrates how to train a Support Vector Machine (SVM) model with an RBF kernel and evaluate its performance. The gamma
parameter controls the influence of each training example, with a higher value leading to a more complex model.
Regularization and Hyperparameter Tuning
Hyperparameter tuning is crucial for optimizing the performance of Support Vector Machines (SVM). The regularization parameter C and the kernel-specific parameters, such as gamma for the RBF kernel, need to be carefully selected to balance the trade-off between margin width and classification error.
XGBoost: A Powerful ML Model for Classification and RegressionGrid search and cross-validation are common techniques for hyperparameter tuning. Scikit-learn provides a convenient implementation of grid search with cross-validation.
from sklearn.model_selection import GridSearchCV
# Define the parameter grid
param_grid = {
'C': [0.1, 1, 10, 100],
'gamma': ['scale', 'auto'],
'kernel': ['rbf']
}
# Perform grid search with cross-validation
grid_search = GridSearchCV(SVC(), param_grid, cv=5)
grid_search.fit(X_train, y_train)
# Get the best parameters
best_params = grid_search.best_params_
print(f"Best Parameters: {best_params}")
This code snippet demonstrates how to perform grid search with cross-validation to find the optimal hyperparameters for a Support Vector Machine (SVM) model. By tuning these parameters, you can significantly improve the model's performance.
Handling Imbalanced Data
Imbalanced data is a common challenge in many real-world applications. Support Vector Machines (SVM) can be adapted to handle imbalanced data by adjusting the class weights or using techniques like SMOTE (Synthetic Minority Over-sampling Technique) to balance the dataset.
Adjusting the class weights involves assigning a higher penalty to misclassifications of the minority class, encouraging the model to pay more attention to the minority class.
Bootstrapping: Training Deep Neural Networks on Noisy Labels# Train an SVM model with adjusted class weights
weighted_model = SVC(kernel='linear', class_weight='balanced', C=1.0)
weighted_model.fit(X_train, y_train)
# Evaluate the weighted SVM model
weighted_y_pred = weighted_model.predict(X_test)
weighted_accuracy = accuracy_score(y_test, weighted_y_pred)
print(f"Weighted SVM Accuracy: {weighted_accuracy}")
This code snippet demonstrates how to train a Support Vector Machine (SVM) model with adjusted class weights to handle imbalanced data. The class_weight
parameter is set to 'balanced'
, which automatically adjusts the weights inversely proportional to the class frequencies.
Practical Applications of Support Vector Machines
Text Classification
Support Vector Machines (SVM) are widely used for text classification tasks, such as spam detection, sentiment analysis, and document categorization. Their ability to handle high-dimensional data makes them well-suited for these applications, where the feature space can be vast due to the large number of unique words or terms.
For text classification, features are typically extracted using techniques like TF-IDF (Term Frequency-Inverse Document Frequency) or word embeddings. The extracted features are then fed into an SVM model for training and classification.
from sklearn.feature_extraction.text import TfidfVectorizer
# Sample text data
texts = ["I love machine learning", "Support Vector Machines are great", "I hate spam emails"]
# Convert text data to TF-IDF features
vectorizer = TfidfVectorizer()
X_text = vectorizer.fit_transform(texts)
# Train an SVM model on the text data
text_model = SVC(kernel='linear', C=1.0)
text_model.fit(X_text, [1, 1, 0])
This code snippet demonstrates how to convert text data to TF-IDF features and train a Support Vector Machine (SVM) model for text classification. The trained model can then be used to classify new text data.
Image Recognition
Support Vector Machines (SVM) are also used for image recognition tasks, such as object detection and facial recognition. In these applications, features are typically extracted using techniques like HOG (Histogram of Oriented Gradients) or SIFT (Scale-Invariant Feature Transform). The extracted features are then used to train an SVM model.
For instance, in facial recognition, features are extracted from face images and used to train an SVM model to distinguish between different individuals.
from skimage.feature import hog
from skimage import data, color, exposure
# Load a sample image
image = color.rgb2gray(data.astronaut())
# Extract HOG features
features, hog_image = hog(image, pixels_per_cell=(8, 8), cells_per_block=(2, 2), visualize=True)
# Train an SVM model on the HOG features
image_model = SVC(kernel='linear', C=1.0)
image_model.fit([features], [1])
This code snippet demonstrates how to extract HOG features from an image and train a Support Vector Machine (SVM) model for image recognition. The trained model can be used to recognize objects or individuals in new images.
Bioinformatics
In bioinformatics, Support Vector Machines (SVM) are used for tasks such as gene expression analysis, protein classification, and disease prediction. These applications often involve high-dimensional data, making SVMs a suitable choice.
For example, in gene expression analysis, SVMs can be used to classify samples based on their gene expression profiles, helping to identify disease subtypes or predict patient outcomes.
# Sample gene expression data
X_genes = [[1.2, 3.4, 2.1], [0.9, 1.7, 3.3], [1.5, 2.8, 2.0]]
y_genes = [0, 1, 0]
# Train an SVM model on the gene expression data
gene_model = SVC(kernel='linear', C=1.0)
gene_model.fit(X_genes, y_genes)
This code snippet demonstrates how to train a Support Vector Machine (SVM) model on gene expression data. The trained model can be used to classify new samples based on their gene expression profiles.
Tips and Best Practices for Using Support Vector Machines
Feature Scaling
Feature scaling is essential when using Support Vector Machines (SVM), as the algorithm's performance can be significantly impacted by the scale of the features. Standardizing the features to have a mean of zero and a standard deviation of one ensures that all features contribute equally to the model.
from sklearn.preprocessing import StandardScaler
# Standardize the features
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
This code snippet demonstrates how to standardize features using StandardScaler from scikit-learn. Feature scaling helps improve the performance and convergence of the SVM model.
Choosing the Right Kernel
Selecting the appropriate kernel function is crucial for the success of an SVM model. The choice of kernel depends on the nature of the data and the specific application. Linear kernels are suitable for linearly separable data, while non-linear kernels like RBF and polynomial are used for more complex data structures.
Experimenting with different kernels and using techniques like cross-validation can help identify the best kernel for your data.
Interpreting Results
Interpreting the results of an SVM model involves understanding the support vectors, the decision boundary, and the model's performance metrics. Analyzing the support vectors can provide insights into the critical data points that define the decision boundary.
Evaluating the model's performance using metrics such as accuracy, precision, recall, and F1-score helps ensure that the model is effective and reliable for the given application.
Support Vector Machines (SVM) are a versatile and powerful tool for various machine learning tasks. By understanding their working principles, implementing them effectively in Python, and applying advanced techniques, you can harness the full potential of SVMs for your data science projects. Whether you're working on text classification, image recognition, or bioinformatics, Support Vector Machines offer robust and reliable solutions for your machine learning needs.
If you want to read more articles similar to Support Vector Machines for Machine Learning, you can visit the Algorithms category.
You Must Read